STM32H743I_EVAL BSP User Manual
|
stm32h743i_eval_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h743i_eval_ts.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file provides a set of functions needed to manage the Touch 00008 * Screen on STM32H743I-EVAL evaluation boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the touch screen module of the STM32H743I-EVAL 00013 evaluation board on the AMPIRE 640x480 LCD mounted on MB1063 or AMPIRE 00014 480x272 LCD mounted on MB1046 daughter board. 00015 - If the AMPIRE 640x480 LCD is used, the TS3510 or EXC7200 component driver 00016 must be included according to the touch screen driver present on this board. 00017 - If the AMPIRE 480x272 LCD is used, the STMPE811 IO expander device component 00018 driver must be included in order to run the TS module commanded by the IO 00019 expander device, the MFXSTM32L152 IO expander device component driver must be 00020 also included in case of interrupt mode use of the TS. 00021 00022 Driver description: 00023 ------------------ 00024 + Initialization steps: 00025 o Initialize the TS module using the BSP_TS_Init() function. This 00026 function includes the MSP layer hardware resources initialization and the 00027 communication layer configuration to start the TS use. The LCD size properties 00028 (x and y) are passed as parameters. 00029 o If TS interrupt mode is desired, you must configure the TS interrupt mode 00030 by calling the function BSP_TS_ITConfig(). The TS interrupt mode is generated 00031 as an external interrupt whenever a touch is detected. 00032 The interrupt mode internally uses the IO functionalities driver driven by 00033 the IO expander, to configure the IT line. 00034 00035 + Touch screen use 00036 o The touch screen state is captured whenever the function BSP_TS_GetState() is 00037 used. This function returns information about the last LCD touch occurred 00038 in the TS_StateTypeDef structure. 00039 o If TS interrupt mode is used, the function BSP_TS_ITGetStatus() is needed to get 00040 the interrupt status. To clear the IT pending bits, you should call the 00041 function BSP_TS_ITClear(). 00042 o The IT is handled using the corresponding external interrupt IRQ handler, 00043 the user IT callback treatment is implemented on the same external interrupt 00044 callback. 00045 @endverbatim 00046 ****************************************************************************** 00047 * @attention 00048 * 00049 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00050 * 00051 * Redistribution and use in source and binary forms, with or without modification, 00052 * are permitted provided that the following conditions are met: 00053 * 1. Redistributions of source code must retain the above copyright notice, 00054 * this list of conditions and the following disclaimer. 00055 * 2. Redistributions in binary form must reproduce the above copyright notice, 00056 * this list of conditions and the following disclaimer in the documentation 00057 * and/or other materials provided with the distribution. 00058 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00059 * may be used to endorse or promote products derived from this software 00060 * without specific prior written permission. 00061 * 00062 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00063 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00064 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00065 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00066 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00067 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00068 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00069 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00070 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00071 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00072 * 00073 ****************************************************************************** 00074 */ 00075 00076 /* Includes ------------------------------------------------------------------*/ 00077 #include "stm32h743i_eval_ts.h" 00078 #include "stm32h743i_eval_io.h" 00079 00080 /** @addtogroup BSP 00081 * @{ 00082 */ 00083 00084 /** @addtogroup STM32H743I_EVAL 00085 * @{ 00086 */ 00087 00088 /** @addtogroup STM32H743I_EVAL_TS 00089 * @{ 00090 */ 00091 00092 /** @defgroup STM32H743I_EVAL_TS_Private_Types_Definitions TS Private Types Definitions 00093 * @{ 00094 */ 00095 /** 00096 * @} 00097 */ 00098 00099 /** @defgroup STM32H743I_EVAL_TS_Private_Defines TS Private Defines 00100 * @{ 00101 */ 00102 /** 00103 * @} 00104 */ 00105 00106 /** @defgroup STM32H743I_EVAL_TS_Private_Macros TS Private Macros 00107 * @{ 00108 */ 00109 /** 00110 * @} 00111 */ 00112 00113 /** @defgroup STM32H743I_EVAL_TS_Private_Variables TS Private Variables 00114 * @{ 00115 */ 00116 static TS_DrvTypeDef *tsDriver; 00117 static uint16_t tsXBoundary, tsYBoundary; 00118 static uint8_t tsOrientation; 00119 static uint8_t I2cAddress; 00120 /** 00121 * @} 00122 */ 00123 00124 /** @defgroup STM32H743I_EVAL_TS_Private_Function_Prototypes TS Private Function Prototypes 00125 * @{ 00126 */ 00127 /** 00128 * @} 00129 */ 00130 00131 /** addtogroup STM32H743I_EVAL_TS_Exported_Functions 00132 * @{ 00133 */ 00134 00135 /** 00136 * @brief Initializes and configures the touch screen functionalities and 00137 * configures all necessary hardware resources (GPIOs, clocks..). 00138 * @param xSize: Maximum X size of the TS area on LCD 00139 * @param ySize: Maximum Y size of the TS area on LCD 00140 * @retval TS_OK if all initializations are OK. Other value if error. 00141 */ 00142 uint8_t BSP_TS_Init(uint16_t xSize, uint16_t ySize) 00143 { 00144 uint8_t status = TS_OK; 00145 tsXBoundary = xSize; 00146 tsYBoundary = ySize; 00147 00148 /* Read ID and verify if the IO expander is ready */ 00149 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 00150 { 00151 /* Initialize the TS driver structure */ 00152 tsDriver = &stmpe811_ts_drv; 00153 I2cAddress = TS_I2C_ADDRESS; 00154 tsOrientation = TS_SWAP_Y; 00155 } 00156 else 00157 { 00158 IOE_Init(); 00159 00160 /* Check TS3510 touch screen driver presence to determine if TS3510 or 00161 * EXC7200 driver will be used */ 00162 if(BSP_TS3510_IsDetected() == 0) 00163 { 00164 /* Initialize the TS driver structure */ 00165 tsDriver = &ts3510_ts_drv; 00166 I2cAddress = TS3510_I2C_ADDRESS; 00167 } 00168 else 00169 { 00170 /* Initialize the TS driver structure */ 00171 tsDriver = &exc7200_ts_drv; 00172 I2cAddress = EXC7200_I2C_ADDRESS; 00173 } 00174 tsOrientation = TS_SWAP_NONE; 00175 } 00176 00177 /* Initialize the TS driver */ 00178 tsDriver->Init(I2cAddress); 00179 tsDriver->Start(I2cAddress); 00180 00181 return status; 00182 } 00183 00184 /** 00185 * @brief DeInitializes the TouchScreen. 00186 * @retval TS state 00187 */ 00188 uint8_t BSP_TS_DeInit(void) 00189 { 00190 /* Actually ts_driver does not provide a DeInit function */ 00191 return TS_OK; 00192 } 00193 00194 /** 00195 * @brief Configures and enables the touch screen interrupts. 00196 * @retval TS_OK if all initializations are OK. Other value if error. 00197 */ 00198 uint8_t BSP_TS_ITConfig(void) 00199 { 00200 uint8_t ts_status = TS_ERROR; 00201 uint8_t io_status = IO_ERROR; 00202 00203 /* Initialize the IO */ 00204 io_status = BSP_IO_Init(); 00205 if(io_status != IO_OK) 00206 { 00207 return (ts_status); 00208 } 00209 00210 /* Configure TS IT line IO : is active low on FT6206 (see data sheet) */ 00211 /* Configure TS_INT_PIN (MFX_IO_14) low level to generate MFX_IRQ_OUT in EXTI on MCU */ 00212 io_status = BSP_IO_ConfigPin(TS_INT_PIN, IO_MODE_IT_LOW_LEVEL_PU); 00213 if(io_status != IO_OK) 00214 { 00215 return (ts_status); 00216 } 00217 00218 /* Enable the TS in interrupt mode */ 00219 /* In that case the INT output of FT6206 when new touch is available */ 00220 /* is active low and directed on MFX IO14 */ 00221 tsDriver->EnableIT(I2cAddress); 00222 00223 /* If arrived here : set good status on exit */ 00224 ts_status = TS_OK; 00225 00226 return (ts_status); 00227 } 00228 00229 /** 00230 * @brief Gets the touch screen interrupt status. 00231 * @retval TS_OK if all initializations are OK. Other value if error. 00232 */ 00233 uint8_t BSP_TS_ITGetStatus(void) 00234 { 00235 /* Return the TS IT status */ 00236 return (tsDriver->GetITStatus(I2cAddress)); 00237 } 00238 00239 /** 00240 * @brief Returns status and positions of the touch screen. 00241 * @param TS_State: Pointer to touch screen current state structure 00242 * @retval TS_OK if all initializations are OK. Other value if error. 00243 */ 00244 uint8_t BSP_TS_GetState(TS_StateTypeDef *TS_State) 00245 { 00246 static uint32_t _x = 0, _y = 0; 00247 uint16_t x_diff, y_diff , x , y; 00248 uint16_t swap; 00249 00250 TS_State->TouchDetected = tsDriver->DetectTouch(I2cAddress); 00251 00252 if(TS_State->TouchDetected) 00253 { 00254 tsDriver->GetXY(I2cAddress, &x, &y); 00255 00256 if(tsOrientation & TS_SWAP_X) 00257 { 00258 x = 4096 - x; 00259 } 00260 00261 if(tsOrientation & TS_SWAP_Y) 00262 { 00263 y = 4096 - y; 00264 } 00265 00266 if(tsOrientation & TS_SWAP_XY) 00267 { 00268 swap = y; 00269 y = x; 00270 x = swap; 00271 } 00272 00273 x_diff = x > _x? (x - _x): (_x - x); 00274 y_diff = y > _y? (y - _y): (_y - y); 00275 00276 if (x_diff + y_diff > 5) 00277 { 00278 _x = x; 00279 _y = y; 00280 } 00281 00282 TS_State->x = (tsXBoundary * _x) >> 12; 00283 TS_State->y = (tsYBoundary * _y) >> 12; 00284 } 00285 return TS_OK; 00286 } 00287 00288 /** 00289 * @brief Clears all touch screen interrupts. 00290 * @retval None 00291 */ 00292 void BSP_TS_ITClear(void) 00293 { 00294 /* Clear all IO IT pin */ 00295 BSP_IO_ITClear(); 00296 00297 /* Clear TS IT pending bits */ 00298 tsDriver->ClearIT(I2cAddress); 00299 } 00300 00301 /** 00302 * @} 00303 */ 00304 00305 /** 00306 * @} 00307 */ 00308 00309 /** 00310 * @} 00311 */ 00312 00313 /** 00314 * @} 00315 */ 00316 00317 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Aug 23 2017 17:45:13 for STM32H743I_EVAL BSP User Manual by
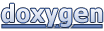