STM32H743I_EVAL BSP User Manual
|
stm32h743i_eval_sram.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h743i_eval_sram.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file includes the SRAM driver for the IS61WV102416BLL-10M memory 00008 * device mounted on STM32H743I-EVAL evaluation boards. 00009 @verbatim 00010 How To use this driver: 00011 ----------------------- 00012 - This driver is used to drive the IS61WV102416BLL-10M SRAM external memory mounted 00013 on STM32H743I-EVAL evaluation board. 00014 - This driver does not need a specific component driver for the SRAM device 00015 to be included with. 00016 00017 Driver description: 00018 ------------------ 00019 + Initialization steps: 00020 o Initialize the SRAM external memory using the BSP_SRAM_Init() function. This 00021 function includes the MSP layer hardware resources initialization and the 00022 FMC controller configuration to interface with the external SRAM memory. 00023 00024 + SRAM read/write operations 00025 o SRAM external memory can be accessed with read/write operations once it is 00026 initialized. 00027 Read/write operation can be performed with AHB access using the functions 00028 BSP_SRAM_ReadData()/BSP_SRAM_WriteData(), or by DMA transfer using the functions 00029 BSP_SRAM_ReadData_DMA()/BSP_SRAM_WriteData_DMA(). 00030 o The AHB access is performed with 16-bit width transaction, the DMA transfer 00031 configuration is fixed at single (no burst) halfword transfer 00032 (see the SRAM_MspInit() static function). 00033 o User can implement his own functions for read/write access with his desired 00034 configurations. 00035 o If interrupt mode is used for DMA transfer, the function BSP_SRAM_MDMA_IRQHandler() 00036 is called in IRQ handler file, to serve the generated interrupt once the DMA 00037 transfer is complete. 00038 @endverbatim 00039 ****************************************************************************** 00040 * @attention 00041 * 00042 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00043 * 00044 * Redistribution and use in source and binary forms, with or without modification, 00045 * are permitted provided that the following conditions are met: 00046 * 1. Redistributions of source code must retain the above copyright notice, 00047 * this list of conditions and the following disclaimer. 00048 * 2. Redistributions in binary form must reproduce the above copyright notice, 00049 * this list of conditions and the following disclaimer in the documentation 00050 * and/or other materials provided with the distribution. 00051 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00052 * may be used to endorse or promote products derived from this software 00053 * without specific prior written permission. 00054 * 00055 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00056 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00057 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00058 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00059 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00060 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00061 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00062 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00063 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00064 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00065 * 00066 ****************************************************************************** 00067 */ 00068 00069 /* Includes ------------------------------------------------------------------*/ 00070 #include "stm32h743i_eval_sram.h" 00071 00072 /** @addtogroup BSP 00073 * @{ 00074 */ 00075 00076 /** @addtogroup STM32H743I_EVAL 00077 * @{ 00078 */ 00079 00080 /** @addtogroup STM32H743I_EVAL_SRAM 00081 * @{ 00082 */ 00083 00084 /** @defgroup STM32H743I_EVAL_SRAM_Private_Types_Definitions SRAM Private Types Definitions 00085 * @{ 00086 */ 00087 /** 00088 * @} 00089 */ 00090 00091 /** @defgroup STM32H743I_EVAL_SRAM_Private_Defines SRAM Private Defines 00092 * @{ 00093 */ 00094 /** 00095 * @} 00096 */ 00097 00098 /** @defgroup STM32H743I_EVAL_SRAM_Private_Macros SRAM Private Macros 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32H743I_EVAL_SRAM_Private_Variables SRAM Private Variables 00106 * @{ 00107 */ 00108 SRAM_HandleTypeDef sramHandle; 00109 static FMC_NORSRAM_TimingTypeDef Timing; 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32H743I_EVAL_SRAM_Private_Function_Prototypes SRAM Private Function Prototypes 00115 * @{ 00116 */ 00117 /** 00118 * @} 00119 */ 00120 00121 /** @addtogroup STM32H743I_EVAL_SRAM_Exported_Functions 00122 * @{ 00123 */ 00124 00125 /** 00126 * @brief Initializes the SRAM device. 00127 * @retval SRAM status 00128 */ 00129 uint8_t BSP_SRAM_Init(void) 00130 { 00131 static uint8_t sram_status = SRAM_ERROR; 00132 /* SRAM device configuration */ 00133 sramHandle.Instance = FMC_NORSRAM_DEVICE; 00134 sramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00135 00136 /* SRAM device configuration */ 00137 /* Timing configuration derived from system clock (up to 400Mhz) 00138 for 100Mhz as SRAM clock frequency */ 00139 Timing.AddressSetupTime = 2; 00140 Timing.AddressHoldTime = 1; 00141 Timing.DataSetupTime = 2; 00142 Timing.BusTurnAroundDuration = 1; 00143 Timing.CLKDivision = 2; 00144 Timing.DataLatency = 2; 00145 Timing.AccessMode = FMC_ACCESS_MODE_A; 00146 00147 sramHandle.Init.NSBank = FMC_NORSRAM_BANK3; 00148 sramHandle.Init.DataAddressMux = FMC_DATA_ADDRESS_MUX_DISABLE; 00149 sramHandle.Init.MemoryType = FMC_MEMORY_TYPE_SRAM; 00150 sramHandle.Init.MemoryDataWidth = SRAM_MEMORY_WIDTH; 00151 sramHandle.Init.BurstAccessMode = SRAM_BURSTACCESS; 00152 sramHandle.Init.WaitSignalPolarity = FMC_WAIT_SIGNAL_POLARITY_LOW; 00153 sramHandle.Init.WaitSignalActive = FMC_WAIT_TIMING_BEFORE_WS; 00154 sramHandle.Init.WriteOperation = FMC_WRITE_OPERATION_ENABLE; 00155 sramHandle.Init.WaitSignal = FMC_WAIT_SIGNAL_DISABLE; 00156 sramHandle.Init.ExtendedMode = FMC_EXTENDED_MODE_DISABLE; 00157 sramHandle.Init.AsynchronousWait = FMC_ASYNCHRONOUS_WAIT_DISABLE; 00158 sramHandle.Init.WriteBurst = SRAM_WRITEBURST; 00159 sramHandle.Init.ContinuousClock = CONTINUOUSCLOCK_FEATURE; 00160 00161 /* SRAM controller initialization */ 00162 BSP_SRAM_MspInit(&sramHandle, NULL); /* __weak function can be rewritten by the application */ 00163 if(HAL_SRAM_Init(&sramHandle, &Timing, &Timing) != HAL_OK) 00164 { 00165 sram_status = SRAM_ERROR; 00166 } 00167 else 00168 { 00169 sram_status = SRAM_OK; 00170 } 00171 return sram_status; 00172 } 00173 00174 /** 00175 * @brief DeInitializes the SRAM device. 00176 * @retval SRAM status 00177 */ 00178 uint8_t BSP_SRAM_DeInit(void) 00179 { 00180 static uint8_t sram_status = SRAM_ERROR; 00181 /* SRAM device de-initialization */ 00182 sramHandle.Instance = FMC_NORSRAM_DEVICE; 00183 sramHandle.Extended = FMC_NORSRAM_EXTENDED_DEVICE; 00184 00185 if(HAL_SRAM_DeInit(&sramHandle) != HAL_OK) 00186 { 00187 sram_status = SRAM_ERROR; 00188 } 00189 else 00190 { 00191 sram_status = SRAM_OK; 00192 } 00193 00194 /* SRAM controller de-initialization */ 00195 BSP_SRAM_MspDeInit(&sramHandle, NULL); 00196 00197 return sram_status; 00198 } 00199 00200 /** 00201 * @brief Reads an amount of data from the SRAM device in polling mode. 00202 * @param uwStartAddress: Read start address 00203 * @param pData: Pointer to data to be read 00204 * @param uwDataSize: Size of read data from the memory 00205 * @retval SRAM status 00206 */ 00207 uint8_t BSP_SRAM_ReadData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00208 { 00209 if(HAL_SRAM_Read_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00210 { 00211 return SRAM_ERROR; 00212 } 00213 else 00214 { 00215 return SRAM_OK; 00216 } 00217 } 00218 00219 /** 00220 * @brief Reads an amount of data from the SRAM device in DMA mode. 00221 * @param uwStartAddress: Read start address 00222 * @param pData: Pointer to data to be read 00223 * @param uwDataSize: Size of read data from the memory 00224 * @retval SRAM status 00225 */ 00226 uint8_t BSP_SRAM_ReadData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00227 { 00228 if(HAL_SRAM_Read_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, (uint32_t)(uwDataSize/2)) != HAL_OK) 00229 { 00230 return SRAM_ERROR; 00231 } 00232 else 00233 { 00234 return SRAM_OK; 00235 } 00236 } 00237 00238 /** 00239 * @brief Writes an amount of data from the SRAM device in polling mode. 00240 * @param uwStartAddress: Write start address 00241 * @param pData: Pointer to data to be written 00242 * @param uwDataSize: Size of written data from the memory 00243 * @retval SRAM status 00244 */ 00245 uint8_t BSP_SRAM_WriteData(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00246 { 00247 if(HAL_SRAM_Write_16b(&sramHandle, (uint32_t *)uwStartAddress, pData, uwDataSize) != HAL_OK) 00248 { 00249 return SRAM_ERROR; 00250 } 00251 else 00252 { 00253 return SRAM_OK; 00254 } 00255 } 00256 00257 /** 00258 * @brief Writes an amount of data from the SRAM device in DMA mode. 00259 * @param uwStartAddress: Write start address 00260 * @param pData: Pointer to data to be written 00261 * @param uwDataSize: Size of written data from the memory 00262 * @retval SRAM status 00263 */ 00264 uint8_t BSP_SRAM_WriteData_DMA(uint32_t uwStartAddress, uint16_t *pData, uint32_t uwDataSize) 00265 { 00266 if(HAL_SRAM_Write_DMA(&sramHandle, (uint32_t *)uwStartAddress, (uint32_t *)pData, (uint32_t)(uwDataSize/2)) != HAL_OK) 00267 { 00268 return SRAM_ERROR; 00269 } 00270 else 00271 { 00272 return SRAM_OK; 00273 } 00274 } 00275 00276 /** 00277 * @brief Initializes SRAM MSP. 00278 * @param hsram: SRAM handle 00279 * @param Params: Pointer to void 00280 * @retval None 00281 */ 00282 __weak void BSP_SRAM_MspInit(SRAM_HandleTypeDef *hsram, void *Params) 00283 { 00284 static MDMA_HandleTypeDef mdma_handle; 00285 GPIO_InitTypeDef gpio_init_structure; 00286 00287 /* Enable FMC clock */ 00288 __HAL_RCC_FMC_CLK_ENABLE(); 00289 00290 /* Enable chosen DMAx clock */ 00291 __SRAM_MDMAx_CLK_ENABLE(); 00292 00293 /* Enable GPIOs clock */ 00294 __HAL_RCC_GPIOD_CLK_ENABLE(); 00295 __HAL_RCC_GPIOE_CLK_ENABLE(); 00296 __HAL_RCC_GPIOF_CLK_ENABLE(); 00297 __HAL_RCC_GPIOG_CLK_ENABLE(); 00298 00299 /* Common GPIO configuration */ 00300 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00301 gpio_init_structure.Pull = GPIO_PULLUP; 00302 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00303 gpio_init_structure.Alternate = GPIO_AF12_FMC; 00304 00305 /* GPIOD configuration */ 00306 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3| GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 |\ 00307 GPIO_PIN_7 | GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00308 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00309 HAL_GPIO_Init(GPIOD, &gpio_init_structure); 00310 00311 /* GPIOE configuration */ 00312 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_3| GPIO_PIN_4 | GPIO_PIN_7 |\ 00313 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 |\ 00314 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00315 HAL_GPIO_Init(GPIOE, &gpio_init_structure); 00316 00317 /* GPIOF configuration */ 00318 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 |\ 00319 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00320 00321 HAL_GPIO_Init(GPIOF, &gpio_init_structure); 00322 00323 /* GPIOG configuration */ 00324 gpio_init_structure.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2| GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 |\ 00325 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14; 00326 HAL_GPIO_Init(GPIOG, &gpio_init_structure); 00327 00328 00329 /* Configure common MDMA parameters */ 00330 mdma_handle.Init.Request = MDMA_REQUEST_SW; 00331 mdma_handle.Init.TransferTriggerMode = MDMA_BLOCK_TRANSFER; 00332 mdma_handle.Init.Priority = MDMA_PRIORITY_HIGH; 00333 mdma_handle.Init.Endianness = MDMA_LITTLE_ENDIANNESS_PRESERVE; 00334 mdma_handle.Init.SourceInc = MDMA_SRC_INC_WORD; 00335 mdma_handle.Init.DestinationInc = MDMA_DEST_INC_WORD; 00336 mdma_handle.Init.SourceDataSize = MDMA_SRC_DATASIZE_WORD; 00337 mdma_handle.Init.DestDataSize = MDMA_DEST_DATASIZE_WORD; 00338 mdma_handle.Init.DataAlignment = MDMA_DATAALIGN_PACKENABLE; 00339 mdma_handle.Init.SourceBurst = MDMA_SOURCE_BURST_SINGLE; 00340 mdma_handle.Init.DestBurst = MDMA_DEST_BURST_SINGLE; 00341 mdma_handle.Init.BufferTransferLength = 128; 00342 mdma_handle.Init.SourceBlockAddressOffset = 0; 00343 mdma_handle.Init.DestBlockAddressOffset = 0; 00344 00345 mdma_handle.Instance = SRAM_MDMAx_CHANNEL; 00346 00347 /* Associate the DMA handle */ 00348 __HAL_LINKDMA(hsram, hmdma, mdma_handle); 00349 00350 /* Deinitialize the Stream for new transfer */ 00351 HAL_MDMA_DeInit(&mdma_handle); 00352 00353 /* Configure the DMA Stream */ 00354 HAL_MDMA_Init(&mdma_handle); 00355 00356 /* NVIC configuration for DMA transfer complete interrupt */ 00357 HAL_NVIC_SetPriority(SRAM_MDMAx_IRQn, 0x0F, 0); 00358 HAL_NVIC_EnableIRQ(SRAM_MDMAx_IRQn); 00359 } 00360 00361 00362 /** 00363 * @brief DeInitializes SRAM MSP. 00364 * @param hsram: SRAM handle 00365 * @param Params: Pointer to void 00366 * @retval None 00367 */ 00368 __weak void BSP_SRAM_MspDeInit(SRAM_HandleTypeDef *hsram, void *Params) 00369 { 00370 static MDMA_HandleTypeDef mdma_handle; 00371 00372 /* Disable NVIC configuration for DMA interrupt */ 00373 HAL_NVIC_DisableIRQ(SRAM_MDMAx_IRQn); 00374 00375 /* Deinitialize the stream for new transfer */ 00376 mdma_handle.Instance = SRAM_MDMAx_CHANNEL; 00377 HAL_MDMA_DeInit(&mdma_handle); 00378 00379 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the applications 00380 by surcharging this __weak function */ 00381 } 00382 00383 /** 00384 * @} 00385 */ 00386 00387 /** 00388 * @} 00389 */ 00390 00391 /** 00392 * @} 00393 */ 00394 00395 /** 00396 * @} 00397 */ 00398 00399 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Aug 23 2017 17:45:13 for STM32H743I_EVAL BSP User Manual by
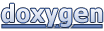