STM32H743I_EVAL BSP User Manual
|
stm32h743i_eval_qspi.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h743i_eval_qspi.h 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm32h743i_eval_qspi.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /** @addtogroup BSP 00040 * @{ 00041 */ 00042 00043 /** @addtogroup STM32H743I_EVAL 00044 * @{ 00045 */ 00046 00047 /* Define to prevent recursive inclusion -------------------------------------*/ 00048 #ifndef __STM32H743I_EVAL_QSPI_H 00049 #define __STM32H743I_EVAL_QSPI_H 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 /* Includes ------------------------------------------------------------------*/ 00056 #include "stm32h7xx_hal.h" 00057 #include "../Components/mt25tl01g/mt25tl01g.h" 00058 00059 /** @defgroup STM32H743I_EVAL_QSPI QSPI 00060 * @{ 00061 */ 00062 00063 00064 /* Exported constants --------------------------------------------------------*/ 00065 /** @defgroup STM32H743I_EVAL_QSPI_Exported_Constants QSPI Exported Constants 00066 * @{ 00067 */ 00068 /* QSPI Error codes */ 00069 #define QSPI_OK ((uint8_t)0x00) 00070 #define QSPI_ERROR ((uint8_t)0x01) 00071 #define QSPI_BUSY ((uint8_t)0x02) 00072 #define QSPI_NOT_SUPPORTED ((uint8_t)0x04) 00073 #define QSPI_SUSPENDED ((uint8_t)0x08) 00074 00075 00076 /* Definition for QSPI clock resources */ 00077 #define QSPI_CLK_ENABLE() __HAL_RCC_QSPI_CLK_ENABLE() 00078 #define QSPI_CLK_DISABLE() __HAL_RCC_QSPI_CLK_DISABLE() 00079 #define QSPI_CLK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00080 #define QSPI_BK1_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00081 #define QSPI_BK1_D0_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00082 #define QSPI_BK1_D1_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00083 #define QSPI_BK1_D2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00084 #define QSPI_BK1_D3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00085 #define QSPI_BK2_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00086 #define QSPI_BK2_D0_GPIO_CLK_ENABLE() __HAL_RCC_GPIOH_CLK_ENABLE() 00087 #define QSPI_BK2_D1_GPIO_CLK_ENABLE() __HAL_RCC_GPIOH_CLK_ENABLE() 00088 #define QSPI_BK2_D2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00089 #define QSPI_BK2_D3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00090 00091 00092 #define QSPI_FORCE_RESET() __HAL_RCC_QSPI_FORCE_RESET() 00093 #define QSPI_RELEASE_RESET() __HAL_RCC_QSPI_RELEASE_RESET() 00094 00095 /* Definition for QSPI Pins */ 00096 #define QSPI_CLK_PIN GPIO_PIN_2 00097 #define QSPI_CLK_GPIO_PORT GPIOB 00098 /* Bank 1 */ 00099 #define QSPI_BK1_CS_PIN GPIO_PIN_6 00100 #define QSPI_BK1_CS_GPIO_PORT GPIOG 00101 #define QSPI_BK1_D0_PIN GPIO_PIN_8 00102 #define QSPI_BK1_D0_GPIO_PORT GPIOF 00103 #define QSPI_BK1_D1_PIN GPIO_PIN_9 00104 #define QSPI_BK1_D1_GPIO_PORT GPIOF 00105 #define QSPI_BK1_D2_PIN GPIO_PIN_7 00106 #define QSPI_BK1_D2_GPIO_PORT GPIOF 00107 #define QSPI_BK1_D3_PIN GPIO_PIN_6 00108 #define QSPI_BK1_D3_GPIO_PORT GPIOF 00109 00110 /* Bank 2 */ 00111 #define QSPI_BK2_CS_PIN GPIO_PIN_11 00112 #define QSPI_BK2_CS_GPIO_PORT GPIOC 00113 #define QSPI_BK2_D0_PIN GPIO_PIN_2 00114 #define QSPI_BK2_D0_GPIO_PORT GPIOH 00115 #define QSPI_BK2_D1_PIN GPIO_PIN_3 00116 #define QSPI_BK2_D1_GPIO_PORT GPIOH 00117 #define QSPI_BK2_D2_PIN GPIO_PIN_9 00118 #define QSPI_BK2_D2_GPIO_PORT GPIOG 00119 #define QSPI_BK2_D3_PIN GPIO_PIN_14 00120 #define QSPI_BK2_D3_GPIO_PORT GPIOG 00121 00122 00123 /* MT25TL01G Micron memory */ 00124 /* Size of the flash */ 00125 #define QSPI_FLASH_SIZE 26 /* Address bus width to access whole memory space */ 00126 #define QSPI_PAGE_SIZE 256 00127 00128 /* QSPI Base Address */ 00129 #define QSPI_BASE_ADDRESS 0x90000000 00130 00131 /** 00132 * @} 00133 */ 00134 00135 /* Exported types ------------------------------------------------------------*/ 00136 /** @defgroup STM32H743I_EVAL_QSPI_Exported_Types QSPI Exported Types 00137 * @{ 00138 */ 00139 /* QSPI Info */ 00140 typedef struct { 00141 uint32_t FlashSize; /*!< Size of the flash */ 00142 uint32_t EraseSectorSize; /*!< Size of sectors for the erase operation */ 00143 uint32_t EraseSectorsNumber; /*!< Number of sectors for the erase operation */ 00144 uint32_t ProgPageSize; /*!< Size of pages for the program operation */ 00145 uint32_t ProgPagesNumber; /*!< Number of pages for the program operation */ 00146 } QSPI_Info; 00147 00148 /** 00149 * @} 00150 */ 00151 00152 00153 /* Exported functions --------------------------------------------------------*/ 00154 /** @defgroup STM32H743I_EVAL_QSPI_Exported_Functions QSPI Exported Functions 00155 * @{ 00156 */ 00157 uint8_t BSP_QSPI_Init (void); 00158 uint8_t BSP_QSPI_DeInit (void); 00159 uint8_t BSP_QSPI_Read (uint8_t* pData, uint32_t ReadAddr, uint32_t Size); 00160 uint8_t BSP_QSPI_Write (uint8_t* pData, uint32_t WriteAddr, uint32_t Size); 00161 uint8_t BSP_QSPI_Erase_Block(uint32_t BlockAddress); 00162 uint8_t BSP_QSPI_Erase_Chip (void); 00163 uint8_t BSP_QSPI_GetStatus (void); 00164 uint8_t BSP_QSPI_GetInfo (QSPI_Info* pInfo); 00165 uint8_t BSP_QSPI_EnableMemoryMappedMode(void); 00166 00167 /* These functions can be modified in case the current settings 00168 need to be changed for specific application needs */ 00169 void BSP_QSPI_MspInit(QSPI_HandleTypeDef *hqspi, void *Params); 00170 void BSP_QSPI_MspDeInit(QSPI_HandleTypeDef *hqspi, void *Params); 00171 00172 /** 00173 * @} 00174 */ 00175 00176 /** 00177 * @} 00178 */ 00179 00180 #ifdef __cplusplus 00181 } 00182 #endif 00183 00184 #endif /* __STM32H743I_EVAL_QSPI_H */ 00185 /** 00186 * @} 00187 */ 00188 00189 /** 00190 * @} 00191 */ 00192 00193 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Aug 23 2017 17:45:13 for STM32H743I_EVAL BSP User Manual by
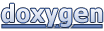