STM32H743I_EVAL BSP User Manual
|
stm32h743i_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32h743i_eval.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 21-April-2017 00007 * @brief This file provides a set of firmware functions to manage LEDs, 00008 * push-buttons and COM ports available on STM32H743I-EVAL 00009 * evaluation board(MB1219) from STMicroelectronics. 00010 * 00011 @verbatim 00012 This driver requires the stm32h743i_eval_io.c/.h files to manage the 00013 IO module resources mapped on the MFX IO expander. 00014 These resources are mainly LEDs, Joystick push buttons, SD detect pin, 00015 USB OTG power switch/over current drive pins, Audio 00016 INT pin 00017 @endverbatim 00018 ****************************************************************************** 00019 * @attention 00020 * 00021 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00022 * 00023 * Redistribution and use in source and binary forms, with or without modification, 00024 * are permitted provided that the following conditions are met: 00025 * 1. Redistributions of source code must retain the above copyright notice, 00026 * this list of conditions and the following disclaimer. 00027 * 2. Redistributions in binary form must reproduce the above copyright notice, 00028 * this list of conditions and the following disclaimer in the documentation 00029 * and/or other materials provided with the distribution. 00030 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00031 * may be used to endorse or promote products derived from this software 00032 * without specific prior written permission. 00033 * 00034 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00035 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00036 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00037 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00038 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00039 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00040 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00041 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00042 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00043 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00044 * 00045 ****************************************************************************** 00046 */ 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32h743i_eval.h" 00050 #if defined(USE_IOEXPANDER) 00051 #include "stm32h743i_eval_io.h" 00052 #endif /* USE_IOEXPANDER */ 00053 00054 /** @addtogroup BSP 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM32H743I_EVAL 00059 * @{ 00060 */ 00061 00062 /** @addtogroup STM32H743I_EVAL_LOW_LEVEL 00063 * @{ 00064 */ 00065 00066 /** @defgroup STM32H743I_EVAL_LOW_LEVEL_Private_TypesDefinitions STM32H743I-EVAL LOW LEVEL Private Types Definitions 00067 * @{ 00068 */ 00069 /** 00070 * @} 00071 */ 00072 00073 /** @defgroup STM32H743I_EVAL_LOW_LEVEL_Private_Defines STM32H743I-EVAL LOW LEVEL Private Defines 00074 * @{ 00075 */ 00076 /** 00077 * @brief STM32H743I EVAL BSP Driver version number V1.0.0 00078 */ 00079 #define __STM32H743I_EVAL_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00080 #define __STM32H743I_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00081 #define __STM32H743I_EVAL_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00082 #define __STM32H743I_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00083 #define __STM32H743I_EVAL_BSP_VERSION ((__STM32H743I_EVAL_BSP_VERSION_MAIN << 24)\ 00084 |(__STM32H743I_EVAL_BSP_VERSION_SUB1 << 16)\ 00085 |(__STM32H743I_EVAL_BSP_VERSION_SUB2 << 8 )\ 00086 |(__STM32H743I_EVAL_BSP_VERSION_RC)) 00087 /** 00088 * @} 00089 */ 00090 00091 /** @defgroup STM32H743I_EVAL_LOW_LEVEL_Private_Macros STM32H743I-EVAL LOW LEVEL Private Macros 00092 * @{ 00093 */ 00094 /** 00095 * @} 00096 */ 00097 00098 /** @defgroup STM32H743I_EVAL_LOW_LEVEL_Private_Variables STM32H743I-EVAL LOW LEVEL Private Variables 00099 * @{ 00100 */ 00101 00102 #if defined(USE_IOEXPANDER) 00103 00104 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00105 LED2_PIN, 00106 LED3_PIN, 00107 LED4_PIN}; 00108 #else 00109 00110 const uint32_t GPIO_PIN[LEDn] = {LED1_PIN, 00111 LED3_PIN}; 00112 #endif /* USE_IOEXPANDER */ 00113 00114 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00115 TAMPER_BUTTON_GPIO_PORT, 00116 KEY_BUTTON_GPIO_PORT}; 00117 00118 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00119 TAMPER_BUTTON_PIN, 00120 KEY_BUTTON_PIN}; 00121 00122 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00123 TAMPER_BUTTON_EXTI_IRQn, 00124 KEY_BUTTON_EXTI_IRQn}; 00125 00126 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00127 00128 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00129 00130 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00131 00132 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00133 00134 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00135 00136 const uint16_t COM_TX_AF[COMn] = {EVAL_COM1_TX_AF}; 00137 00138 const uint16_t COM_RX_AF[COMn] = {EVAL_COM1_RX_AF}; 00139 00140 static I2C_HandleTypeDef heval_I2c; 00141 static ADC_HandleTypeDef heval_ADC; 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /** @defgroup STM32H743I_EVAL_LOW_LEVEL_Private_FunctionPrototypes STM32H743I_EVAL LOW LEVEL Private Function Prototypes 00148 * @{ 00149 */ 00150 static void I2Cx_MspInit(void); 00151 static void I2Cx_Init(void); 00152 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00153 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg); 00154 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00155 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00156 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00157 static void I2Cx_Error(uint8_t Addr); 00158 00159 #if defined(USE_IOEXPANDER) 00160 /* IOExpander IO functions */ 00161 void IOE_Init(void); 00162 void IOE_ITConfig(void); 00163 void IOE_Delay(uint32_t Delay); 00164 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00165 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00166 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00167 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00168 00169 /* MFX IO functions */ 00170 void MFX_IO_Init(void); 00171 void MFX_IO_DeInit(void); 00172 void MFX_IO_ITConfig(void); 00173 void MFX_IO_Delay(uint32_t Delay); 00174 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value); 00175 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg); 00176 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00177 void MFX_IO_Wakeup(void); 00178 void MFX_IO_EnableWakeupPin(void); 00179 #endif /* USE_IOEXPANDER */ 00180 00181 /* AUDIO IO functions */ 00182 void AUDIO_IO_Init(void); 00183 void AUDIO_IO_DeInit(void); 00184 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value); 00185 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg); 00186 void AUDIO_IO_Delay(uint32_t Delay); 00187 00188 /* I2C EEPROM IO function */ 00189 void EEPROM_IO_Init(void); 00190 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00191 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00192 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00193 00194 /* TouchScreen (TS) IO functions */ 00195 void TS_IO_Init(void); 00196 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00197 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg); 00198 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00199 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00200 void TS_IO_Delay(uint32_t Delay); 00201 00202 void OTM8009A_IO_Delay(uint32_t Delay); 00203 /** 00204 * @} 00205 */ 00206 00207 /** @addtogroup STM32H743I_EVAL_LOW_LEVEL_Exported_Functions 00208 * @{ 00209 */ 00210 00211 /** 00212 * @brief This method returns the STM32H743I EVAL BSP Driver revision 00213 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00214 */ 00215 uint32_t BSP_GetVersion(void) 00216 { 00217 return __STM32H743I_EVAL_BSP_VERSION; 00218 } 00219 00220 /** 00221 * @brief Configures LED on GPIO and/or on MFX. 00222 * @param Led: LED to be configured. 00223 * This parameter can be one of the following values: 00224 * @arg LED1 00225 * @arg LED2 00226 * @arg LED3 00227 * @arg LED4 00228 * @retval None 00229 */ 00230 void BSP_LED_Init(Led_TypeDef Led) 00231 { 00232 /* On RevB board, LED1 and LED3 are connected to GPIOs */ 00233 00234 GPIO_InitTypeDef gpio_initstruct; 00235 GPIO_TypeDef* gpio_led; 00236 00237 if ((Led == LED1) || (Led == LED3)) 00238 { 00239 if (Led == LED1) 00240 { 00241 gpio_led = LED1_GPIO_PORT; 00242 /* Enable the GPIO_LED clock */ 00243 LED1_GPIO_CLK_ENABLE(); 00244 } 00245 else 00246 { 00247 gpio_led = LED3_GPIO_PORT; 00248 /* Enable the GPIO_LED clock */ 00249 LED3_GPIO_CLK_ENABLE(); 00250 } 00251 00252 /* Configure the GPIO_LED pin */ 00253 gpio_initstruct.Pin = GPIO_PIN[Led]; 00254 gpio_initstruct.Mode = GPIO_MODE_OUTPUT_PP; 00255 gpio_initstruct.Pull = GPIO_PULLUP; 00256 gpio_initstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00257 00258 HAL_GPIO_Init(gpio_led, &gpio_initstruct); 00259 00260 /* By default, turn off LED */ 00261 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_SET); 00262 } 00263 else 00264 { 00265 #if defined(USE_IOEXPANDER) 00266 /* On RevB and above eval board, LED2 and LED4 are connected to MFX */ 00267 BSP_IO_Init(); /* Initialize MFX */ 00268 BSP_IO_ConfigPin(GPIO_PIN[Led], IO_MODE_OUTPUT_PP_PU); 00269 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_SET); 00270 #endif /* USE_IOEXPANDER */ 00271 00272 } 00273 } 00274 00275 00276 /** 00277 * @brief DeInit LEDs. 00278 * @param Led: LED to be configured. 00279 * This parameter can be one of the following values: 00280 * @arg LED1 00281 * @arg LED2 00282 * @arg LED3 00283 * @arg LED4 00284 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00285 * @retval None 00286 */ 00287 void BSP_LED_DeInit(Led_TypeDef Led) 00288 { 00289 GPIO_InitTypeDef gpio_init_structure; 00290 GPIO_TypeDef* gpio_led; 00291 00292 /* On RevB led1 and Led3 are on GPIO while Led2 and Led4 on Mfx*/ 00293 if ((Led == LED1) || (Led == LED3)) 00294 { 00295 if (Led == LED1) 00296 { 00297 gpio_led = LED1_GPIO_PORT; 00298 } 00299 else 00300 { 00301 gpio_led = LED3_GPIO_PORT; 00302 } 00303 /* Turn off LED */ 00304 HAL_GPIO_WritePin(gpio_led, GPIO_PIN[Led], GPIO_PIN_RESET); 00305 /* Configure the GPIO_LED pin */ 00306 gpio_init_structure.Pin = GPIO_PIN[Led]; 00307 HAL_GPIO_DeInit(gpio_led, gpio_init_structure.Pin); 00308 } 00309 else 00310 { 00311 #if defined(USE_IOEXPANDER) /* (USE_IOEXPANDER always defined for RevA) */ 00312 /* GPIO_PIN[Led] depends on the board revision: */ 00313 /* - in case of RevA all leds are deinit */ 00314 /* - in case of RevB just led 2 and led4 are deinit */ 00315 BSP_IO_ConfigPin(GPIO_PIN[Led], IO_MODE_OFF); 00316 #endif /* USE_IOEXPANDER */ 00317 } 00318 } 00319 00320 /** 00321 * @brief Turns selected LED On. 00322 * @param Led: LED to be set on 00323 * This parameter can be one of the following values: 00324 * @arg LED1 00325 * @arg LED2 00326 * @arg LED3 00327 * @arg LED4 00328 * @retval None 00329 */ 00330 void BSP_LED_On(Led_TypeDef Led) 00331 { 00332 00333 if ((Led == LED1) || (Led == LED3)) /* Switch On LED connected to GPIO */ 00334 { 00335 if (Led == LED1) 00336 { 00337 HAL_GPIO_WritePin(LED1_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_RESET); 00338 } 00339 else 00340 { 00341 HAL_GPIO_WritePin(LED3_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_RESET); 00342 } 00343 } 00344 else 00345 { 00346 #if defined(USE_IOEXPANDER) /* Switch On LED connected to MFX */ 00347 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_RESET); 00348 #endif /* USE_IOEXPANDER */ 00349 } 00350 } 00351 00352 /** 00353 * @brief Turns selected LED Off. 00354 * @param Led: LED to be set off 00355 * This parameter can be one of the following values: 00356 * @arg LED1 00357 * @arg LED2 00358 * @arg LED3 00359 * @arg LED4 00360 * @retval None 00361 */ 00362 void BSP_LED_Off(Led_TypeDef Led) 00363 { 00364 if ((Led == LED1) || (Led == LED3)) /* Switch Off LED connected to GPIO */ 00365 { 00366 if (Led == LED1) 00367 { 00368 HAL_GPIO_WritePin(LED1_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_SET); 00369 } 00370 else 00371 { 00372 HAL_GPIO_WritePin(LED3_GPIO_PORT, GPIO_PIN[Led], GPIO_PIN_SET); 00373 } 00374 } 00375 else 00376 { 00377 #if defined(USE_IOEXPANDER) /* Switch Off LED connected to MFX */ 00378 BSP_IO_WritePin(GPIO_PIN[Led], BSP_IO_PIN_SET); 00379 #endif /* USE_IOEXPANDER */ 00380 } 00381 } 00382 00383 /** 00384 * @brief Toggles the selected LED. 00385 * @param Led: LED to be toggled 00386 * This parameter can be one of the following values: 00387 * @arg LED1 00388 * @arg LED2 00389 * @arg LED3 00390 * @arg LED4 00391 * @retval None 00392 */ 00393 void BSP_LED_Toggle(Led_TypeDef Led) 00394 { 00395 if ((Led == LED1) || (Led == LED3)) /* Toggle LED connected to GPIO */ 00396 { 00397 if (Led == LED1) 00398 { 00399 HAL_GPIO_TogglePin(LED1_GPIO_PORT, GPIO_PIN[Led]); 00400 } 00401 else 00402 { 00403 HAL_GPIO_TogglePin(LED3_GPIO_PORT, GPIO_PIN[Led]); 00404 } 00405 } 00406 else 00407 { 00408 #if defined(USE_IOEXPANDER) /* Toggle LED connected to MFX */ 00409 BSP_IO_TogglePin(GPIO_PIN[Led]); 00410 #endif /* USE_IOEXPANDER */ 00411 } 00412 } 00413 00414 /** 00415 * @brief Configures button GPIO and EXTI Line. 00416 * @param Button: Button to be configured 00417 * This parameter can be one of the following values: 00418 * @arg BUTTON_WAKEUP: Wakeup Push Button 00419 * @arg BUTTON_TAMPER: Tamper Push Button 00420 * @arg BUTTON_KEY: Key Push Button 00421 * @param ButtonMode: Button mode 00422 * This parameter can be one of the following values: 00423 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00424 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00425 * with interrupt generation capability 00426 * @note On STM32H743I-EVAL evaluation board, the three buttons (Wakeup, Tamper 00427 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00428 * on the board serigraphy. 00429 * @retval None 00430 */ 00431 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00432 { 00433 GPIO_InitTypeDef GPIO_InitStruct; 00434 00435 /* Enable the BUTTON clock */ 00436 BUTTONx_GPIO_CLK_ENABLE(Button); 00437 00438 if(ButtonMode == BUTTON_MODE_GPIO) 00439 { 00440 /* Configure Button pin as input */ 00441 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00442 GPIO_InitStruct.Mode = GPIO_MODE_INPUT; 00443 GPIO_InitStruct.Pull = GPIO_NOPULL; 00444 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00445 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00446 } 00447 00448 if(ButtonMode == BUTTON_MODE_EXTI) 00449 { 00450 /* Configure Button pin as input with External interrupt */ 00451 GPIO_InitStruct.Pin = BUTTON_PIN[Button]; 00452 GPIO_InitStruct.Pull = GPIO_NOPULL; 00453 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00454 00455 if(Button != BUTTON_WAKEUP) 00456 { 00457 GPIO_InitStruct.Mode = GPIO_MODE_IT_FALLING; 00458 } 00459 else 00460 { 00461 GPIO_InitStruct.Mode = GPIO_MODE_IT_RISING; 00462 } 00463 00464 HAL_GPIO_Init(BUTTON_PORT[Button], &GPIO_InitStruct); 00465 00466 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00467 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00468 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00469 } 00470 } 00471 00472 /** 00473 * @brief Push Button DeInit. 00474 * @param Button: Button to be configured 00475 * This parameter can be one of the following values: 00476 * @arg BUTTON_WAKEUP: Wakeup Push Button 00477 * @arg BUTTON_TAMPER: Tamper Push Button 00478 * @arg BUTTON_KEY: Key Push Button 00479 * @note On STM32H743I-EVAL evaluation board, the three buttons (Wakeup, Tamper 00480 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00481 * on the board serigraphy. 00482 * @note PB DeInit does not disable the GPIO clock 00483 * @retval None 00484 */ 00485 void BSP_PB_DeInit(Button_TypeDef Button) 00486 { 00487 GPIO_InitTypeDef gpio_init_structure; 00488 00489 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00490 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00491 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00492 } 00493 00494 00495 /** 00496 * @brief Returns the selected button state. 00497 * @param Button: Button to be checked 00498 * This parameter can be one of the following values: 00499 * @arg BUTTON_WAKEUP: Wakeup Push Button 00500 * @arg BUTTON_TAMPER: Tamper Push Button 00501 * @arg BUTTON_KEY: Key Push Button 00502 * @note On STM32H743I-EVAL evaluation board, the three buttons (Wakeup, Tamper 00503 * and key buttons) are mapped on the same push button named "Wakeup/Tamper" 00504 * on the board serigraphy. 00505 * @retval The Button GPIO pin value 00506 */ 00507 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00508 { 00509 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00510 } 00511 00512 /** 00513 * @brief Configures COM port. 00514 * @param COM: COM port to be configured. 00515 * This parameter can be one of the following values: 00516 * @arg COM1 00517 * @arg COM2 00518 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00519 * configuration information for the specified USART peripheral. 00520 * @retval None 00521 */ 00522 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00523 { 00524 GPIO_InitTypeDef gpio_init_structure; 00525 00526 /* Enable GPIO clock */ 00527 EVAL_COMx_TX_GPIO_CLK_ENABLE(COM); 00528 EVAL_COMx_RX_GPIO_CLK_ENABLE(COM); 00529 00530 /* Enable USART clock */ 00531 EVAL_COMx_CLK_ENABLE(COM); 00532 00533 /* Configure USART Tx as alternate function */ 00534 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00535 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00536 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00537 gpio_init_structure.Pull = GPIO_PULLUP; 00538 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00539 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00540 00541 /* Configure USART Rx as alternate function */ 00542 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00543 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00544 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00545 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00546 00547 /* USART configuration */ 00548 huart->Instance = COM_USART[COM]; 00549 HAL_UART_Init(huart); 00550 } 00551 00552 /** 00553 * @brief DeInit COM port. 00554 * @param COM: COM port to be configured. 00555 * This parameter can be one of the following values: 00556 * @arg COM1 00557 * @arg COM2 00558 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00559 * configuration information for the specified USART peripheral. 00560 * @retval None 00561 */ 00562 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00563 { 00564 /* USART configuration */ 00565 huart->Instance = COM_USART[COM]; 00566 HAL_UART_DeInit(huart); 00567 00568 /* Enable USART clock */ 00569 EVAL_COMx_CLK_DISABLE(COM); 00570 00571 /* DeInit GPIO pins can be done in the application 00572 (by surcharging this __weak function) */ 00573 00574 /* GPIO pins clock, DMA clock can be shut down in the application 00575 by surcharging this __weak function */ 00576 } 00577 00578 /** 00579 * @brief Init Potentiometer. 00580 * @retval None 00581 */ 00582 void BSP_POTENTIOMETER_Init(void) 00583 { 00584 GPIO_InitTypeDef GPIO_InitStruct; 00585 ADC_ChannelConfTypeDef ADC_Config; 00586 00587 /* ADC an GPIO Periph clock enable */ 00588 ADCx_CLK_ENABLE(); 00589 ADCx_CHANNEL_GPIO_CLK_ENABLE(); 00590 00591 /* ADC Channel GPIO pin configuration */ 00592 GPIO_InitStruct.Pin = ADCx_CHANNEL_PIN; 00593 GPIO_InitStruct.Mode = GPIO_MODE_ANALOG; 00594 GPIO_InitStruct.Pull = GPIO_NOPULL; 00595 HAL_GPIO_Init(ADCx_CHANNEL_GPIO_PORT, &GPIO_InitStruct); 00596 00597 /* Configure the ADC peripheral */ 00598 heval_ADC.Instance = ADCx; 00599 00600 HAL_ADC_DeInit(&heval_ADC); 00601 00602 heval_ADC.Init.ClockPrescaler = ADC_CLOCKPRESCALER_PCLK_DIV4; /* Asynchronous clock mode, input ADC clock not divided */ 00603 heval_ADC.Init.Resolution = ADC_RESOLUTION_12B; /* 12-bit resolution for converted data */ 00604 heval_ADC.Init.ScanConvMode = DISABLE; /* Sequencer disabled (ADC conversion on only 1 channel: channel set on rank 1) */ 00605 heval_ADC.Init.EOCSelection = ADC_EOC_SINGLE_CONV; /* EOC flag picked-up to indicate conversion end */ 00606 heval_ADC.Init.ContinuousConvMode = DISABLE; /* Continuous mode disabled to have only 1 conversion at each conversion trig */ 00607 heval_ADC.Init.NbrOfConversion = 1; /* Parameter discarded because sequencer is disabled */ 00608 heval_ADC.Init.DiscontinuousConvMode = DISABLE; /* Parameter discarded because sequencer is disabled */ 00609 heval_ADC.Init.NbrOfDiscConversion = 0; /* Parameter discarded because sequencer is disabled */ 00610 heval_ADC.Init.ExternalTrigConv = ADC_EXTERNALTRIG_T1_CC1; /* Software start to trig the 1st conversion manually, without external event */ 00611 heval_ADC.Init.ExternalTrigConvEdge = ADC_EXTERNALTRIGCONVEDGE_NONE; /* Parameter discarded because software trigger chosen */ 00612 00613 HAL_ADC_Init(&heval_ADC); 00614 00615 /* Configure ADC regular channel */ 00616 ADC_Config.Channel = ADCx_CHANNEL; /* Sampled channel number */ 00617 ADC_Config.Rank = 1; /* Rank of sampled channel number ADCx_CHANNEL */ 00618 ADC_Config.SamplingTime = ADC_SAMPLETIME_2CYCLES_5; /* Sampling time (number of clock cycles unit) */ 00619 ADC_Config.Offset = 0; /* Parameter discarded because offset correction is disabled */ 00620 00621 HAL_ADC_ConfigChannel(&heval_ADC, &ADC_Config); 00622 } 00623 00624 /** 00625 * @brief Get Potentiometer level in 12 bits. 00626 * @retval Potentiometer level(0..0xFFF) / 0xFFFFFFFF : Error 00627 */ 00628 uint32_t BSP_POTENTIOMETER_GetLevel(void) 00629 { 00630 if(HAL_ADC_Start(&heval_ADC) == HAL_OK) 00631 { 00632 if(HAL_ADC_PollForConversion(&heval_ADC, ADCx_POLL_TIMEOUT)== HAL_OK) 00633 { 00634 return (HAL_ADC_GetValue(&heval_ADC)); 00635 } 00636 } 00637 return 0xFFFFFFFF; 00638 } 00639 00640 #if defined(USE_IOEXPANDER) 00641 /** 00642 * @brief Configures joystick GPIO and EXTI modes. 00643 * @param JoyMode: Button mode. 00644 * This parameter can be one of the following values: 00645 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00646 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00647 * with interrupt generation capability 00648 * @retval IO_OK: if all initializations are OK. Other value if error. 00649 */ 00650 uint8_t BSP_JOY_Init(JOYMode_TypeDef JoyMode) 00651 { 00652 uint8_t ret = 0; 00653 00654 /* Initialize the IO functionalities */ 00655 ret = BSP_IO_Init(); 00656 00657 /* Configure joystick pins in IT mode */ 00658 if(JoyMode == JOY_MODE_EXTI) 00659 { 00660 /* Configure IO interrupt acquisition mode */ 00661 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_LOW_LEVEL_PU); 00662 } 00663 else 00664 { 00665 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_INPUT_PU); 00666 } 00667 00668 return ret; 00669 } 00670 00671 00672 /** 00673 * @brief DeInit joystick GPIOs. 00674 * @note JOY DeInit does not disable the MFX, just set the MFX pins in Off mode 00675 * @retval None. 00676 */ 00677 void BSP_JOY_DeInit() 00678 { 00679 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_OFF); 00680 } 00681 00682 /** 00683 * @brief Returns the current joystick status. 00684 * @retval Code of the joystick key pressed 00685 * This code can be one of the following values: 00686 * @arg JOY_NONE 00687 * @arg JOY_SEL 00688 * @arg JOY_DOWN 00689 * @arg JOY_LEFT 00690 * @arg JOY_RIGHT 00691 * @arg JOY_UP 00692 */ 00693 JOYState_TypeDef BSP_JOY_GetState(void) 00694 { 00695 uint16_t pin_status = 0; 00696 00697 /* Read the status joystick pins */ 00698 pin_status = BSP_IO_ReadPin(JOY_ALL_PINS); 00699 00700 /* Check the pressed keys */ 00701 if((pin_status & JOY_NONE_PIN) == JOY_NONE) 00702 { 00703 return(JOYState_TypeDef) JOY_NONE; 00704 } 00705 else if(!(pin_status & JOY_SEL_PIN)) 00706 { 00707 return(JOYState_TypeDef) JOY_SEL; 00708 } 00709 else if(!(pin_status & JOY_DOWN_PIN)) 00710 { 00711 return(JOYState_TypeDef) JOY_DOWN; 00712 } 00713 else if(!(pin_status & JOY_LEFT_PIN)) 00714 { 00715 return(JOYState_TypeDef) JOY_LEFT; 00716 } 00717 else if(!(pin_status & JOY_RIGHT_PIN)) 00718 { 00719 return(JOYState_TypeDef) JOY_RIGHT; 00720 } 00721 else if(!(pin_status & JOY_UP_PIN)) 00722 { 00723 return(JOYState_TypeDef) JOY_UP; 00724 } 00725 else 00726 { 00727 return(JOYState_TypeDef) JOY_NONE; 00728 } 00729 } 00730 00731 /** 00732 * @brief Check TS3510 touch screen presence 00733 * @retval Return 0 if TS3510 is detected, return 1 if not detected 00734 */ 00735 uint8_t BSP_TS3510_IsDetected(void) 00736 { 00737 HAL_StatusTypeDef status = HAL_OK; 00738 uint32_t error = 0; 00739 uint8_t a_buffer; 00740 00741 uint8_t tmp_buffer[2] = {0x81, 0x08}; 00742 00743 /* Prepare for LCD read data */ 00744 IOE_WriteMultiple(TS3510_I2C_ADDRESS, 0x8A, tmp_buffer, 2); 00745 00746 status = HAL_I2C_Mem_Read(&heval_I2c, TS3510_I2C_ADDRESS, 0x8A, I2C_MEMADD_SIZE_8BIT, &a_buffer, 1, 1000); 00747 00748 /* Check the communication status */ 00749 if(status != HAL_OK) 00750 { 00751 error = (uint32_t)HAL_I2C_GetError(&heval_I2c); 00752 00753 /* I2C error occurred */ 00754 I2Cx_Error(TS3510_I2C_ADDRESS); 00755 00756 if(error == HAL_I2C_ERROR_AF) 00757 { 00758 return 1; 00759 } 00760 } 00761 return 0; 00762 } 00763 00764 #endif /* USE_IOEXPANDER */ 00765 00766 /******************************************************************************* 00767 BUS OPERATIONS 00768 *******************************************************************************/ 00769 00770 /******************************* I2C Routines *********************************/ 00771 /** 00772 * @brief Initializes I2C MSP. 00773 * @retval None 00774 */ 00775 static void I2Cx_MspInit(void) 00776 { 00777 GPIO_InitTypeDef gpio_init_structure; 00778 RCC_PeriphCLKInitTypeDef RCC_PeriphClkInit; 00779 00780 /* Configure the I2C clock source */ 00781 RCC_PeriphClkInit.PeriphClockSelection = RCC_PERIPHCLK_I2C123; 00782 RCC_PeriphClkInit.I2c123ClockSelection = RCC_I2C123CLKSOURCE_D2PCLK1; 00783 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphClkInit); 00784 00785 /* set STOPWUCK in RCC_CFGR */ 00786 __HAL_RCC_WAKEUPSTOP_CLK_CONFIG(RCC_STOP_WAKEUPCLOCK_HSI); 00787 00788 /*** Configure the GPIOs ***/ 00789 /* Enable GPIO clock */ 00790 EVAL_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00791 00792 /* Configure I2C Tx as alternate function */ 00793 gpio_init_structure.Pin = EVAL_I2Cx_SCL_PIN; 00794 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00795 gpio_init_structure.Pull = GPIO_NOPULL; 00796 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00797 gpio_init_structure.Alternate = EVAL_I2Cx_SCL_SDA_AF; 00798 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00799 00800 /* Configure I2C Rx as alternate function */ 00801 gpio_init_structure.Pin = EVAL_I2Cx_SDA_PIN; 00802 HAL_GPIO_Init(EVAL_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00803 00804 /*** Configure the I2C peripheral ***/ 00805 /* Enable I2C clock */ 00806 EVAL_I2Cx_CLK_ENABLE(); 00807 00808 /* Force the I2C peripheral clock reset */ 00809 EVAL_I2Cx_FORCE_RESET(); 00810 00811 /* Release the I2C peripheral clock reset */ 00812 EVAL_I2Cx_RELEASE_RESET(); 00813 00814 /* Enable and set I2Cx Interrupt to a lower priority */ 00815 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 0x0F, 0); 00816 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00817 00818 /* Enable and set I2Cx Interrupt to a lower priority */ 00819 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 0x0F, 0); 00820 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00821 } 00822 00823 /** 00824 * @brief Initializes I2C HAL. 00825 * @retval None 00826 */ 00827 static void I2Cx_Init(void) 00828 { 00829 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00830 { 00831 heval_I2c.Instance = EVAL_I2Cx; 00832 heval_I2c.Init.Timing = EVAL_I2Cx_TIMING; 00833 heval_I2c.Init.OwnAddress1 = 0x72; 00834 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00835 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_ENABLE; 00836 heval_I2c.Init.OwnAddress2 = 0; 00837 heval_I2c.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00838 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_ENABLE; 00839 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00840 00841 /* Init the I2C */ 00842 I2Cx_MspInit(); 00843 HAL_I2C_Init(&heval_I2c); 00844 } 00845 } 00846 00847 /** 00848 * @brief Writes a single data. 00849 * @param Addr: I2C address 00850 * @param Reg: Register address 00851 * @param Value: Data to be written 00852 * @retval None 00853 */ 00854 static void I2Cx_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00855 { 00856 HAL_StatusTypeDef status = HAL_OK; 00857 00858 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00859 00860 /* Check the communication status */ 00861 if(status != HAL_OK) 00862 { 00863 /* Execute user timeout callback */ 00864 I2Cx_Error(Addr); 00865 } 00866 } 00867 00868 /** 00869 * @brief Reads a single data. 00870 * @param Addr: I2C address 00871 * @param Reg: Register address 00872 * @retval Read data 00873 */ 00874 static uint8_t I2Cx_Read(uint8_t Addr, uint8_t Reg) 00875 { 00876 HAL_StatusTypeDef status = HAL_OK; 00877 uint8_t Value = 0; 00878 00879 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00880 00881 /* Check the communication status */ 00882 if(status != HAL_OK) 00883 { 00884 /* Execute user timeout callback */ 00885 I2Cx_Error(Addr); 00886 } 00887 return Value; 00888 } 00889 00890 /** 00891 * @brief Reads multiple data. 00892 * @param Addr: I2C address 00893 * @param Reg: Reg address 00894 * @param MemAddress: memory address to be read 00895 * @param Buffer: Pointer to data buffer 00896 * @param Length: Length of the data 00897 * @retval Number of read data 00898 */ 00899 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00900 { 00901 HAL_StatusTypeDef status = HAL_OK; 00902 00903 if(Addr == EXC7200_I2C_ADDRESS) 00904 { 00905 status = HAL_I2C_Master_Receive(&heval_I2c, Addr, Buffer, Length, 1000); 00906 } 00907 else 00908 { 00909 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00910 } 00911 00912 /* Check the communication status */ 00913 if(status != HAL_OK) 00914 { 00915 /* I2C error occurred */ 00916 I2Cx_Error(Addr); 00917 } 00918 return status; 00919 } 00920 00921 /** 00922 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00923 * @param Addr: Device address on BUS Bus. 00924 * @param Reg: The target register address to write 00925 * @param MemAddress: memory address to be written 00926 * @param Buffer: The target register value to be written 00927 * @param Length: buffer size to be written 00928 * @retval HAL status 00929 */ 00930 static HAL_StatusTypeDef I2Cx_WriteMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00931 { 00932 HAL_StatusTypeDef status = HAL_OK; 00933 00934 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00935 00936 /* Check the communication status */ 00937 if(status != HAL_OK) 00938 { 00939 /* Re-Initiaize the I2C Bus */ 00940 I2Cx_Error(Addr); 00941 } 00942 return status; 00943 } 00944 00945 /** 00946 * @brief Checks if target device is ready for communication. 00947 * @note This function is used with Memory devices 00948 * @param DevAddress: Target device address 00949 * @param Trials: Number of trials 00950 * @retval HAL status 00951 */ 00952 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00953 { 00954 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, 1000)); 00955 } 00956 00957 /** 00958 * @brief Manages error callback by re-initializing I2C. 00959 * @param Addr: I2C Address 00960 * @retval None 00961 */ 00962 static void I2Cx_Error(uint8_t Addr) 00963 { 00964 /* De-initialize the I2C comunication bus */ 00965 HAL_I2C_DeInit(&heval_I2c); 00966 00967 /* Re-Initialize the I2C communication bus */ 00968 I2Cx_Init(); 00969 } 00970 00971 /******************************************************************************* 00972 LINK OPERATIONS 00973 *******************************************************************************/ 00974 00975 /********************************* LINK IOE ***********************************/ 00976 #if defined(USE_IOEXPANDER) 00977 /** 00978 * @brief Initializes IOE low level. 00979 * @retval None 00980 */ 00981 void IOE_Init(void) 00982 { 00983 I2Cx_Init(); 00984 } 00985 00986 /** 00987 * @brief Configures IOE low level interrupt. 00988 * @retval None 00989 */ 00990 void IOE_ITConfig(void) 00991 { 00992 /* IO expander IT config done by BSP_TS_ITConfig function */ 00993 } 00994 00995 /** 00996 * @brief IOE writes single data. 00997 * @param Addr: I2C address 00998 * @param Reg: Register address 00999 * @param Value: Data to be written 01000 * @retval None 01001 */ 01002 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01003 { 01004 I2Cx_Write(Addr, Reg, Value); 01005 } 01006 01007 /** 01008 * @brief IOE reads single data. 01009 * @param Addr: I2C address 01010 * @param Reg: Register address 01011 * @retval Read data 01012 */ 01013 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 01014 { 01015 return I2Cx_Read(Addr, Reg); 01016 } 01017 01018 /** 01019 * @brief IOE reads multiple data. 01020 * @param Addr: I2C address 01021 * @param Reg: Register address 01022 * @param Buffer: Pointer to data buffer 01023 * @param Length: Length of the data 01024 * @retval Number of read data 01025 */ 01026 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01027 { 01028 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01029 } 01030 01031 /** 01032 * @brief IOE writes multiple data. 01033 * @param Addr: I2C address 01034 * @param Reg: Register address 01035 * @param Buffer: Pointer to data buffer 01036 * @param Length: Length of the data 01037 * @retval None 01038 */ 01039 void IOE_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01040 { 01041 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01042 } 01043 01044 /** 01045 * @brief IOE delay 01046 * @param Delay: Delay in ms 01047 * @retval None 01048 */ 01049 void IOE_Delay(uint32_t Delay) 01050 { 01051 HAL_Delay(Delay); 01052 } 01053 01054 01055 /********************************* LINK MFX ***********************************/ 01056 01057 /** 01058 * @brief Initializes MFX low level. 01059 * @retval None 01060 */ 01061 void MFX_IO_Init(void) 01062 { 01063 I2Cx_Init(); 01064 } 01065 01066 /** 01067 * @brief DeInitializes MFX low level. 01068 * @retval None 01069 */ 01070 void MFX_IO_DeInit(void) 01071 { 01072 } 01073 01074 /** 01075 * @brief Configures MFX low level interrupt. 01076 * @retval None 01077 */ 01078 void MFX_IO_ITConfig(void) 01079 { 01080 static uint8_t mfx_io_it_enabled = 0; 01081 GPIO_InitTypeDef gpio_init_structure; 01082 01083 if(mfx_io_it_enabled == 0) 01084 { 01085 mfx_io_it_enabled = 1; 01086 /* Enable the GPIO EXTI clock */ 01087 __HAL_RCC_GPIOI_CLK_ENABLE(); 01088 __HAL_RCC_SYSCFG_CLK_ENABLE(); 01089 01090 gpio_init_structure.Pin = GPIO_PIN_8; 01091 gpio_init_structure.Pull = GPIO_NOPULL; 01092 gpio_init_structure.Speed = GPIO_SPEED_FREQ_LOW; 01093 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 01094 HAL_GPIO_Init(GPIOI, &gpio_init_structure); 01095 01096 /* Enable and set GPIO EXTI Interrupt to the lowest priority */ 01097 HAL_NVIC_SetPriority((IRQn_Type)(EXTI9_5_IRQn), 0x0F, 0x0F); 01098 HAL_NVIC_EnableIRQ((IRQn_Type)(EXTI9_5_IRQn)); 01099 } 01100 } 01101 01102 /** 01103 * @brief MFX writes single data. 01104 * @param Addr: I2C address 01105 * @param Reg: Register address 01106 * @param Value: Data to be written 01107 * @retval None 01108 */ 01109 void MFX_IO_Write(uint16_t Addr, uint8_t Reg, uint8_t Value) 01110 { 01111 I2Cx_Write((uint8_t) Addr, Reg, Value); 01112 } 01113 01114 /** 01115 * @brief MFX reads single data. 01116 * @param Addr: I2C address 01117 * @param Reg: Register address 01118 * @retval Read data 01119 */ 01120 uint8_t MFX_IO_Read(uint16_t Addr, uint8_t Reg) 01121 { 01122 return I2Cx_Read((uint8_t) Addr, Reg); 01123 } 01124 01125 /** 01126 * @brief MFX reads multiple data. 01127 * @param Addr: I2C address 01128 * @param Reg: Register address 01129 * @param Buffer: Pointer to data buffer 01130 * @param Length: Length of the data 01131 * @retval Number of read data 01132 */ 01133 uint16_t MFX_IO_ReadMultiple(uint16_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01134 { 01135 return I2Cx_ReadMultiple((uint8_t)Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01136 } 01137 01138 /** 01139 * @brief MFX delay 01140 * @param Delay: Delay in ms 01141 * @retval None 01142 */ 01143 void MFX_IO_Delay(uint32_t Delay) 01144 { 01145 HAL_Delay(Delay); 01146 } 01147 01148 /** 01149 * @brief Used by Lx family but requested for MFX component compatibility. 01150 * @retval None 01151 */ 01152 void MFX_IO_Wakeup(void) 01153 { 01154 } 01155 01156 /** 01157 * @brief Used by Lx family but requested for MXF component compatibility. 01158 * @retval None 01159 */ 01160 void MFX_IO_EnableWakeupPin(void) 01161 { 01162 } 01163 01164 #endif /* USE_IOEXPANDER */ 01165 01166 /********************************* LINK AUDIO *********************************/ 01167 01168 /** 01169 * @brief Initializes Audio low level. 01170 * @retval None 01171 */ 01172 void AUDIO_IO_Init(void) 01173 { 01174 I2Cx_Init(); 01175 } 01176 01177 /** 01178 * @brief Deinitializes Audio low level. 01179 * @retval None 01180 */ 01181 void AUDIO_IO_DeInit(void) 01182 { 01183 } 01184 01185 /** 01186 * @brief Writes a single data. 01187 * @param Addr: I2C address 01188 * @param Reg: Reg address 01189 * @param Value: Data to be written 01190 * @retval None 01191 */ 01192 void AUDIO_IO_Write(uint8_t Addr, uint16_t Reg, uint16_t Value) 01193 { 01194 uint16_t tmp = Value; 01195 01196 Value = ((uint16_t)(tmp >> 8) & 0x00FF); 01197 01198 Value |= ((uint16_t)(tmp << 8)& 0xFF00); 01199 01200 I2Cx_WriteMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT,(uint8_t*)&Value, 2); 01201 } 01202 01203 /** 01204 * @brief Reads a single data. 01205 * @param Addr: I2C address 01206 * @param Reg: Reg address 01207 * @retval Data to be read 01208 */ 01209 uint16_t AUDIO_IO_Read(uint8_t Addr, uint16_t Reg) 01210 { 01211 uint16_t read_value = 0, tmp = 0; 01212 01213 I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_16BIT, (uint8_t*)&read_value, 2); 01214 01215 tmp = ((uint16_t)(read_value >> 8) & 0x00FF); 01216 01217 tmp |= ((uint16_t)(read_value << 8)& 0xFF00); 01218 01219 read_value = tmp; 01220 01221 return read_value; 01222 } 01223 01224 /** 01225 * @brief AUDIO Codec delay 01226 * @param Delay: Delay in ms 01227 * @retval None 01228 */ 01229 void AUDIO_IO_Delay(uint32_t Delay) 01230 { 01231 HAL_Delay(Delay); 01232 } 01233 01234 /******************************** LINK I2C EEPROM *****************************/ 01235 01236 /** 01237 * @brief Initializes peripherals used by the I2C EEPROM driver. 01238 * @retval None 01239 */ 01240 void EEPROM_IO_Init(void) 01241 { 01242 I2Cx_Init(); 01243 } 01244 01245 /** 01246 * @brief Write data to I2C EEPROM driver in using DMA channel. 01247 * @param DevAddress: Target device address 01248 * @param MemAddress: Internal memory address 01249 * @param pBuffer: Pointer to data buffer 01250 * @param BufferSize: Amount of data to be sent 01251 * @retval HAL status 01252 */ 01253 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01254 { 01255 return (I2Cx_WriteMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01256 } 01257 01258 /** 01259 * @brief Read data from I2C EEPROM driver in using DMA channel. 01260 * @param DevAddress: Target device address 01261 * @param MemAddress: Internal memory address 01262 * @param pBuffer: Pointer to data buffer 01263 * @param BufferSize: Amount of data to be read 01264 * @retval HAL status 01265 */ 01266 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01267 { 01268 return (I2Cx_ReadMultiple(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01269 } 01270 01271 /** 01272 * @brief Checks if target device is ready for communication. 01273 * @note This function is used with Memory devices 01274 * @param DevAddress: Target device address 01275 * @param Trials: Number of trials 01276 * @retval HAL status 01277 */ 01278 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01279 { 01280 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01281 } 01282 01283 /******************************** LINK TS (TouchScreen) *****************************/ 01284 01285 /** 01286 * @brief Initialize I2C communication 01287 * channel from MCU to TouchScreen (TS). 01288 */ 01289 void TS_IO_Init(void) 01290 { 01291 I2Cx_Init(); 01292 } 01293 01294 /** 01295 * @brief Writes single data with I2C communication 01296 * channel from MCU to TouchScreen. 01297 * @param Addr: I2C address 01298 * @param Reg: Register address 01299 * @param Value: Data to be written 01300 */ 01301 void TS_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 01302 { 01303 I2Cx_Write(Addr, Reg, Value); 01304 } 01305 01306 /** 01307 * @brief Reads single data with I2C communication 01308 * channel from TouchScreen. 01309 * @param Addr: I2C address 01310 * @param Reg: Register address 01311 * @retval Read data 01312 */ 01313 uint8_t TS_IO_Read(uint8_t Addr, uint8_t Reg) 01314 { 01315 return I2Cx_Read(Addr, Reg); 01316 } 01317 01318 /** 01319 * @brief Reads multiple data with I2C communication 01320 * channel from TouchScreen. 01321 * @param Addr: I2C address 01322 * @param Reg: Register address 01323 * @param Buffer: Pointer to data buffer 01324 * @param Length: Length of the data 01325 * @retval Number of read data 01326 */ 01327 uint16_t TS_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01328 { 01329 return I2Cx_ReadMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01330 } 01331 01332 /** 01333 * @brief Writes multiple data with I2C communication 01334 * channel from MCU to TouchScreen. 01335 * @param Addr: I2C address 01336 * @param Reg: Register address 01337 * @param Buffer: Pointer to data buffer 01338 * @param Length: Length of the data 01339 * @retval None 01340 */ 01341 void TS_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 01342 { 01343 I2Cx_WriteMultiple(Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 01344 } 01345 01346 /** 01347 * @brief Delay function used in TouchScreen low level driver. 01348 * @param Delay: Delay in ms 01349 * @retval None 01350 */ 01351 void TS_IO_Delay(uint32_t Delay) 01352 { 01353 HAL_Delay(Delay); 01354 } 01355 01356 /**************************** LINK OTM8009A (Display driver) ******************/ 01357 /** 01358 * @brief OTM8009A delay 01359 * @param Delay: Delay in ms 01360 */ 01361 void OTM8009A_IO_Delay(uint32_t Delay) 01362 { 01363 HAL_Delay(Delay); 01364 } 01365 01366 /** 01367 * @} 01368 */ 01369 01370 /** 01371 * @} 01372 */ 01373 01374 /** 01375 * @} 01376 */ 01377 01378 /** 01379 * @} 01380 */ 01381 01382 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Aug 23 2017 17:45:13 for STM32H743I_EVAL BSP User Manual by
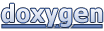