STM32F429I-Discovery BSP User Manual
|
stm32f429i_discovery_sdram.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f429i_discovery_sdram.h 00004 * @author MCD Application Team 00005 * @version V2.1.3 00006 * @date 13-January-2016 00007 * @brief This file contains all the functions prototypes for the 00008 * stm32f429i_discovery_sdram.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32F429I_DISCOVERY_SDRAM_H 00041 #define __STM32F429I_DISCOVERY_SDRAM_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32f429i_discovery.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32F429I_DISCOVERY 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM32F429I_DISCOVERY_SDRAM 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32F429I_DISCOVERY_SDRAM_Exported_Types STM32F429I DISCOVERY SDRAM Exported Types 00063 * @{ 00064 */ 00065 /** 00066 * @} 00067 */ 00068 00069 /** @defgroup STM32F429I_DISCOVERY_SDRAM_Exported_Constants STM32F429I DISCOVERY SDRAM Exported Constants 00070 * @{ 00071 */ 00072 /** 00073 * @brief FMC SDRAM Bank address 00074 */ 00075 #define SDRAM_DEVICE_ADDR ((uint32_t)0xD0000000) 00076 #define SDRAM_DEVICE_SIZE ((uint32_t)0x800000) /* SDRAM device size in MBytes */ 00077 00078 /** 00079 * @brief FMC SDRAM Memory Width 00080 */ 00081 /* #define SDRAM_MEMORY_WIDTH FMC_SDRAM_MEM_BUS_WIDTH_8 */ 00082 #define SDRAM_MEMORY_WIDTH FMC_SDRAM_MEM_BUS_WIDTH_16 00083 00084 /** 00085 * @brief FMC SDRAM CAS Latency 00086 */ 00087 /* #define SDRAM_CAS_LATENCY FMC_SDRAM_CAS_LATENCY_2 */ 00088 #define SDRAM_CAS_LATENCY FMC_SDRAM_CAS_LATENCY_3 00089 00090 /** 00091 * @brief FMC SDRAM Memory clock period 00092 */ 00093 #define SDCLOCK_PERIOD FMC_SDRAM_CLOCK_PERIOD_2 /* Default configuration used with LCD */ 00094 /* #define SDCLOCK_PERIOD FMC_SDRAM_CLOCK_PERIOD_3 */ 00095 00096 /** 00097 * @brief FMC SDRAM Memory Read Burst feature 00098 */ 00099 #define SDRAM_READBURST FMC_SDRAM_RBURST_DISABLE /* Default configuration used with LCD */ 00100 /* #define SDRAM_READBURST FMC_SDRAM_RBURST_ENABLE */ 00101 00102 /** 00103 * @brief FMC SDRAM Bank Remap 00104 */ 00105 /* #define SDRAM_BANK_REMAP */ 00106 00107 /* Set the refresh rate counter */ 00108 /* (15.62 us x Freq) - 20 */ 00109 #define REFRESH_COUNT ((uint32_t)1386) /* SDRAM refresh counter */ 00110 #define SDRAM_TIMEOUT ((uint32_t)0xFFFF) 00111 00112 /* DMA definitions for SDRAM DMA transfer */ 00113 #define __DMAx_CLK_ENABLE __DMA2_CLK_ENABLE 00114 #define SDRAM_DMAx_CHANNEL DMA_CHANNEL_0 00115 #define SDRAM_DMAx_STREAM DMA2_Stream0 00116 #define SDRAM_DMAx_IRQn DMA2_Stream0_IRQn 00117 #define SDRAM_DMAx_IRQHandler DMA2_Stream0_IRQHandler 00118 00119 /** 00120 * @brief FMC SDRAM Mode definition register defines 00121 */ 00122 #define SDRAM_MODEREG_BURST_LENGTH_1 ((uint16_t)0x0000) 00123 #define SDRAM_MODEREG_BURST_LENGTH_2 ((uint16_t)0x0001) 00124 #define SDRAM_MODEREG_BURST_LENGTH_4 ((uint16_t)0x0002) 00125 #define SDRAM_MODEREG_BURST_LENGTH_8 ((uint16_t)0x0004) 00126 #define SDRAM_MODEREG_BURST_TYPE_SEQUENTIAL ((uint16_t)0x0000) 00127 #define SDRAM_MODEREG_BURST_TYPE_INTERLEAVED ((uint16_t)0x0008) 00128 #define SDRAM_MODEREG_CAS_LATENCY_2 ((uint16_t)0x0020) 00129 #define SDRAM_MODEREG_CAS_LATENCY_3 ((uint16_t)0x0030) 00130 #define SDRAM_MODEREG_OPERATING_MODE_STANDARD ((uint16_t)0x0000) 00131 #define SDRAM_MODEREG_WRITEBURST_MODE_PROGRAMMED ((uint16_t)0x0000) 00132 #define SDRAM_MODEREG_WRITEBURST_MODE_SINGLE ((uint16_t)0x0200) 00133 /** 00134 * @} 00135 */ 00136 00137 /** @defgroup STM32F429I_DISCOVERY_SDRAM_Exported_Macro STM32F429I DISCOVERY SDRAM Exported Macro 00138 * @{ 00139 */ 00140 /** 00141 * @} 00142 */ 00143 00144 /** @defgroup STM32F429I_DISCOVERY_SDRAM_Exported_Functions STM32F429I DISCOVERY SDRAM Exported Functions 00145 * @{ 00146 */ 00147 void BSP_SDRAM_Init(void); 00148 void BSP_SDRAM_Initialization_sequence(uint32_t RefreshCount); 00149 void BSP_SDRAM_ReadData(uint32_t uwStartAddress, uint32_t* pData, uint32_t uwDataSize); 00150 void BSP_SDRAM_ReadData_DMA(uint32_t uwStartAddress, uint32_t* pData, uint32_t uwDataSize); 00151 void BSP_SDRAM_WriteData(uint32_t uwStartAddress, uint32_t* pData, uint32_t uwDataSize); 00152 void BSP_SDRAM_WriteData_DMA(uint32_t uwStartAddress, uint32_t* pData, uint32_t uwDataSize); 00153 HAL_StatusTypeDef BSP_SDRAM_Sendcmd(FMC_SDRAM_CommandTypeDef *SdramCmd); 00154 void BSP_SDRAM_DMA_IRQHandler(void); 00155 00156 /** 00157 * @} 00158 */ 00159 00160 /** 00161 * @} 00162 */ 00163 00164 /** 00165 * @} 00166 */ 00167 00168 /** 00169 * @} 00170 */ 00171 00172 #ifdef __cplusplus 00173 } 00174 #endif 00175 00176 #endif /* __STM32F429I_DISCOVERY_SDRAM_H */ 00177 00178 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 13:22:14 for STM32F429I-Discovery BSP User Manual by
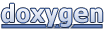