STM32F429I-Discovery BSP User Manual
|
Functions | |
uint32_t | BSP_GetVersion (void) |
This method returns the STM32F429I DISCO BSP Driver revision. | |
void | BSP_LED_Init (Led_TypeDef Led) |
Configures LED GPIO. | |
void | BSP_LED_On (Led_TypeDef Led) |
Turns selected LED On. | |
void | BSP_LED_Off (Led_TypeDef Led) |
Turns selected LED Off. | |
void | BSP_LED_Toggle (Led_TypeDef Led) |
Toggles the selected LED. | |
void | BSP_PB_Init (Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) |
Configures Button GPIO and EXTI Line. | |
uint32_t | BSP_PB_GetState (Button_TypeDef Button) |
Returns the selected Button state. | |
static void | I2Cx_MspInit (I2C_HandleTypeDef *hi2c) |
I2Cx MSP Initialization. | |
static void | I2Cx_WriteData (uint8_t Addr, uint8_t Reg, uint8_t Value) |
Writes a value in a register of the device through BUS. | |
static void | I2Cx_WriteBuffer (uint8_t Addr, uint8_t Reg, uint8_t *pBuffer, uint16_t Length) |
Writes a value in a register of the device through BUS. | |
static uint8_t | I2Cx_ReadData (uint8_t Addr, uint8_t Reg) |
Reads a register of the device through BUS. | |
static uint8_t | I2Cx_ReadBuffer (uint8_t Addr, uint8_t Reg, uint8_t *pBuffer, uint16_t Length) |
Reads multiple data on the BUS. | |
static uint32_t | SPIx_Read (uint8_t ReadSize) |
Reads 4 bytes from device. | |
static void | SPIx_Write (uint16_t Value) |
Writes a byte to device. | |
static uint8_t | SPIx_WriteRead (uint8_t Byte) |
Sends a Byte through the SPI interface and return the Byte received from the SPI bus. | |
static void | SPIx_MspInit (SPI_HandleTypeDef *hspi) |
SPI MSP Init. | |
uint32_t | LCD_IO_ReadData (uint16_t RegValue, uint8_t ReadSize) |
Reads register value. | |
void | LCD_Delay (uint32_t Delay) |
Wait for loop in ms. | |
void | IOE_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
IOE Writes single data operation. | |
uint8_t | IOE_Read (uint8_t Addr, uint8_t Reg) |
IOE Reads single data. | |
void | IOE_WriteMultiple (uint8_t Addr, uint8_t Reg, uint8_t *pBuffer, uint16_t Length) |
IOE Writes multiple data. | |
uint16_t | IOE_ReadMultiple (uint8_t Addr, uint8_t Reg, uint8_t *pBuffer, uint16_t Length) |
IOE Reads multiple data. | |
void | IOE_Delay (uint32_t Delay) |
IOE Delay. | |
void | GYRO_IO_Write (uint8_t *pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite) |
Writes one byte to the Gyroscope. | |
void | GYRO_IO_Read (uint8_t *pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead) |
Reads a block of data from the Gyroscope. |
Function Documentation
uint32_t BSP_GetVersion | ( | void | ) |
This method returns the STM32F429I DISCO BSP Driver revision.
- Return values:
-
version,: 0xXYZR (8bits for each decimal, R for RC)
Definition at line 180 of file stm32f429i_discovery.c.
References __STM32F429I_DISCO_BSP_VERSION.
void BSP_LED_Init | ( | Led_TypeDef | Led | ) |
Configures LED GPIO.
- Parameters:
-
Led,: Specifies the Led to be configured. This parameter can be one of following parameters: - LED3
- LED4
Definition at line 192 of file stm32f429i_discovery.c.
References GPIO_PIN, GPIO_PORT, and LEDx_GPIO_CLK_ENABLE.
void BSP_LED_Off | ( | Led_TypeDef | Led | ) |
Turns selected LED Off.
- Parameters:
-
Led,: Specifies the Led to be set off. This parameter can be one of following parameters: - LED3
- LED4
Definition at line 229 of file stm32f429i_discovery.c.
void BSP_LED_On | ( | Led_TypeDef | Led | ) |
Turns selected LED On.
- Parameters:
-
Led,: Specifies the Led to be set on. This parameter can be one of following parameters: - LED3
- LED4
Definition at line 217 of file stm32f429i_discovery.c.
void BSP_LED_Toggle | ( | Led_TypeDef | Led | ) |
Toggles the selected LED.
- Parameters:
-
Led,: Specifies the Led to be toggled. This parameter can be one of following parameters: - LED3
- LED4
Definition at line 241 of file stm32f429i_discovery.c.
uint32_t BSP_PB_GetState | ( | Button_TypeDef | Button | ) |
Returns the selected Button state.
- Parameters:
-
Button,: Specifies the Button to be checked. This parameter should be: BUTTON_KEY
- Return values:
-
The Button GPIO pin value.
Definition at line 293 of file stm32f429i_discovery.c.
References BUTTON_PIN, and BUTTON_PORT.
void BSP_PB_Init | ( | Button_TypeDef | Button, |
ButtonMode_TypeDef | ButtonMode | ||
) |
Configures Button GPIO and EXTI Line.
- Parameters:
-
Button,: Specifies the Button to be configured. This parameter should be: BUTTON_KEY ButtonMode,: Specifies Button mode. This parameter can be one of following parameters: - BUTTON_MODE_GPIO: Button will be used as simple IO
- BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt generation capability
Definition at line 256 of file stm32f429i_discovery.c.
References BUTTON_IRQn, BUTTON_MODE_EXTI, BUTTON_MODE_GPIO, BUTTON_PIN, BUTTON_PORT, and BUTTONx_GPIO_CLK_ENABLE.
void GYRO_IO_Read | ( | uint8_t * | pBuffer, |
uint8_t | ReadAddr, | ||
uint16_t | NumByteToRead | ||
) |
Reads a block of data from the Gyroscope.
- Parameters:
-
pBuffer,: Pointer to the buffer that receives the data read from the Gyroscope. ReadAddr,: Gyroscope's internal address to read from. NumByteToRead,: Number of bytes to read from the Gyroscope.
Definition at line 1028 of file stm32f429i_discovery.c.
References DUMMY_BYTE, GYRO_CS_HIGH, GYRO_CS_LOW, MULTIPLEBYTE_CMD, READWRITE_CMD, and SPIx_WriteRead().
void GYRO_IO_Write | ( | uint8_t * | pBuffer, |
uint8_t | WriteAddr, | ||
uint16_t | NumByteToWrite | ||
) |
Writes one byte to the Gyroscope.
- Parameters:
-
pBuffer,: Pointer to the buffer containing the data to be written to the Gyroscope. WriteAddr,: Gyroscope's internal address to write to. NumByteToWrite,: Number of bytes to write.
Definition at line 994 of file stm32f429i_discovery.c.
References GYRO_CS_HIGH, GYRO_CS_LOW, MULTIPLEBYTE_CMD, and SPIx_WriteRead().
static void I2Cx_MspInit | ( | I2C_HandleTypeDef * | hi2c | ) | [static] |
I2Cx MSP Initialization.
- Parameters:
-
hi2c,: I2C handle
Definition at line 308 of file stm32f429i_discovery.c.
References DISCOVERY_I2Cx, DISCOVERY_I2Cx_CLOCK_ENABLE, DISCOVERY_I2Cx_ER_IRQn, DISCOVERY_I2Cx_EV_IRQn, DISCOVERY_I2Cx_FORCE_RESET, DISCOVERY_I2Cx_RELEASE_RESET, DISCOVERY_I2Cx_SCL_GPIO_CLK_ENABLE, DISCOVERY_I2Cx_SCL_GPIO_PORT, DISCOVERY_I2Cx_SCL_PIN, DISCOVERY_I2Cx_SCL_SDA_AF, DISCOVERY_I2Cx_SDA_GPIO_CLK_ENABLE, DISCOVERY_I2Cx_SDA_GPIO_PORT, DISCOVERY_I2Cx_SDA_PIN, and I2cHandle.
Referenced by I2Cx_Init().
static uint8_t I2Cx_ReadBuffer | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Reads multiple data on the BUS.
- Parameters:
-
Addr,: I2C Address Reg,: Reg Address pBuffer,: pointer to read data buffer Length,: length of the data
- Return values:
-
0 if no problems to read multiple data
Definition at line 535 of file stm32f429i_discovery.c.
References I2cHandle, I2Cx_Error(), and I2cxTimeout.
Referenced by IOE_ReadMultiple().
static uint8_t I2Cx_ReadData | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) | [static] |
Reads a register of the device through BUS.
- Parameters:
-
Addr,: Device address on BUS Bus. Reg,: The target register address to write
- Return values:
-
Data read at register address
Definition at line 510 of file stm32f429i_discovery.c.
References I2cHandle, I2Cx_Error(), and I2cxTimeout.
Referenced by IOE_Read().
static void I2Cx_WriteBuffer | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) | [static] |
Writes a value in a register of the device through BUS.
- Parameters:
-
Addr,: Device address on BUS Bus. Reg,: The target register address to write pBuffer,: The target register value to be written Length,: buffer size to be written
Definition at line 490 of file stm32f429i_discovery.c.
References I2cHandle, I2Cx_Error(), and I2cxTimeout.
Referenced by IOE_WriteMultiple().
static void I2Cx_WriteData | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) | [static] |
Writes a value in a register of the device through BUS.
- Parameters:
-
Addr,: Device address on BUS Bus. Reg,: The target register address to write Value,: The target register value to be written
Definition at line 469 of file stm32f429i_discovery.c.
References I2cHandle, I2Cx_Error(), and I2cxTimeout.
Referenced by IOE_Write().
void IOE_Delay | ( | uint32_t | Delay | ) |
uint8_t IOE_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) |
IOE Reads single data.
- Parameters:
-
Addr,: I2C Address Reg,: Reg Address
- Return values:
-
The read data
Definition at line 917 of file stm32f429i_discovery.c.
References I2Cx_ReadData().
uint16_t IOE_ReadMultiple | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) |
IOE Reads multiple data.
- Parameters:
-
Addr,: I2C Address Reg,: Reg Address pBuffer,: pointer to data buffer Length,: length of the data
- Return values:
-
0 if no problems to read multiple data
Definition at line 942 of file stm32f429i_discovery.c.
References I2Cx_ReadBuffer().
void IOE_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
IOE Writes single data operation.
- Parameters:
-
Addr,: I2C Address Reg,: Reg Address Value,: Data to be written
Definition at line 906 of file stm32f429i_discovery.c.
References I2Cx_WriteData().
void IOE_WriteMultiple | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) |
IOE Writes multiple data.
- Parameters:
-
Addr,: I2C Address Reg,: Reg Address pBuffer,: pointer to data buffer Length,: length of the data
Definition at line 929 of file stm32f429i_discovery.c.
References I2Cx_WriteBuffer().
void LCD_Delay | ( | uint32_t | Delay | ) |
uint32_t LCD_IO_ReadData | ( | uint16_t | RegValue, |
uint8_t | ReadSize | ||
) |
Reads register value.
- Parameters:
-
RegValue Address of the register to read ReadSize Number of bytes to read
- Return values:
-
Content of the register value
Definition at line 846 of file stm32f429i_discovery.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_WRX_HIGH, LCD_WRX_LOW, SPIx_Read(), and SPIx_Write().
static void SPIx_MspInit | ( | SPI_HandleTypeDef * | hspi | ) | [static] |
SPI MSP Init.
- Parameters:
-
hspi,: SPI handle
Definition at line 743 of file stm32f429i_discovery.c.
References DISCOVERY_SPIx_AF, DISCOVERY_SPIx_CLK_ENABLE, DISCOVERY_SPIx_GPIO_CLK_ENABLE, DISCOVERY_SPIx_GPIO_PORT, DISCOVERY_SPIx_MISO_PIN, DISCOVERY_SPIx_MOSI_PIN, and DISCOVERY_SPIx_SCK_PIN.
Referenced by SPIx_Init().
static uint32_t SPIx_Read | ( | uint8_t | ReadSize | ) | [static] |
Reads 4 bytes from device.
- Parameters:
-
ReadSize,: Number of bytes to read (max 4 bytes)
- Return values:
-
Value read on the SPI
Definition at line 672 of file stm32f429i_discovery.c.
References SpiHandle, SPIx_Error(), and SpixTimeout.
Referenced by LCD_IO_ReadData().
static void SPIx_Write | ( | uint16_t | Value | ) | [static] |
Writes a byte to device.
- Parameters:
-
Value,: value to be written
Definition at line 693 of file stm32f429i_discovery.c.
References SpiHandle, SPIx_Error(), and SpixTimeout.
Referenced by LCD_IO_ReadData(), LCD_IO_WriteData(), and LCD_IO_WriteReg().
static uint8_t SPIx_WriteRead | ( | uint8_t | Byte | ) | [static] |
Sends a Byte through the SPI interface and return the Byte received from the SPI bus.
- Parameters:
-
Byte,: Byte send.
- Return values:
-
The received byte value
Definition at line 713 of file stm32f429i_discovery.c.
References SpiHandle, SPIx_Error(), and SpixTimeout.
Referenced by GYRO_IO_Read(), and GYRO_IO_Write().
Generated on Wed Jan 13 2016 13:22:14 for STM32F429I-Discovery BSP User Manual by
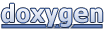