STM32F429I-Discovery BSP User Manual
|
stm32f429i_discovery_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f429i_discovery_lcd.c 00004 * @author MCD Application Team 00005 * @version V2.1.3 00006 * @date 13-January-2016 00007 * @brief This file includes the LCD driver for ILI9341 Liquid Crystal 00008 * Display Modules of STM32F429I-Discovery kit (MB1075). 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* File Info : ----------------------------------------------------------------- 00040 User NOTES 00041 1. How To use this driver: 00042 -------------------------- 00043 - This driver is used to drive directly an LCD TFT using LTDC controller. 00044 - This driver select dynamically the mounted LCD, ILI9341 240x320 LCD mounted 00045 on MB1075B discovery board, and use the adequate timing and setting for 00046 the specified LCD using device ID of the ILI9341 mounted on MB1075B discovery board 00047 00048 2. Driver description: 00049 --------------------- 00050 + Initialization steps : 00051 o Initialize the LCD using the LCD_Init() function. 00052 o Apply the Layer configuration using LCD_LayerDefaultInit() function 00053 o Select the LCD layer to be used using LCD_SelectLayer() function. 00054 o Enable the LCD display using LCD_DisplayOn() function. 00055 00056 + Options 00057 o Configure and enable the color keying functionality using LCD_SetColorKeying() 00058 function. 00059 o Modify in the fly the transparency and/or the frame buffer address 00060 using the following functions : 00061 - LCD_SetTransparency() 00062 - LCD_SetLayerAddress() 00063 00064 + Display on LCD 00065 o Clear the hole LCD using LCD_Clear() function or only one specified string 00066 line using LCD_ClearStringLine() function. 00067 o Display a character on the specified line and column using LCD_DisplayChar() 00068 function or a complete string line using LCD_DisplayStringAtLine() function. 00069 o Display a string line on the specified position (x,y in pixel) and align mode 00070 using LCD_DisplayStringAtLine() function. 00071 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00072 on LCD using the available set of functions 00073 00074 ------------------------------------------------------------------------------*/ 00075 00076 /* Includes ------------------------------------------------------------------*/ 00077 #include "stm32f429i_discovery_lcd.h" 00078 #include "../../../Utilities/Fonts/fonts.h" 00079 #include "../../../Utilities/Fonts/font24.c" 00080 #include "../../../Utilities/Fonts/font20.c" 00081 #include "../../../Utilities/Fonts/font16.c" 00082 #include "../../../Utilities/Fonts/font12.c" 00083 #include "../../../Utilities/Fonts/font8.c" 00084 00085 /** @addtogroup BSP 00086 * @{ 00087 */ 00088 00089 /** @addtogroup STM32F429I_DISCOVERY 00090 * @{ 00091 */ 00092 00093 /** @defgroup STM32F429I_DISCOVERY_LCD STM32F429I DISCOVERY LCD 00094 * @brief This file includes the LCD driver for (ILI9341) 00095 * @{ 00096 */ 00097 00098 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_TypesDefinitions STM32F429I DISCOVERY LCD Private TypesDefinitions 00099 * @{ 00100 */ 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_Defines STM32F429I DISCOVERY LCD Private Defines 00106 * @{ 00107 */ 00108 #define POLY_X(Z) ((int32_t)((Points + Z)->X)) 00109 #define POLY_Y(Z) ((int32_t)((Points + Z)->Y)) 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_Macros STM32F429I DISCOVERY LCD Private Macros 00115 * @{ 00116 */ 00117 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_Variables STM32F429I DISCOVERY LCD Private Variables 00123 * @{ 00124 */ 00125 static LTDC_HandleTypeDef LtdcHandler; 00126 static DMA2D_HandleTypeDef Dma2dHandler; 00127 static RCC_PeriphCLKInitTypeDef PeriphClkInitStruct; 00128 00129 /* Default LCD configuration with LCD Layer 1 */ 00130 static uint32_t ActiveLayer = 0; 00131 static LCD_DrawPropTypeDef DrawProp[MAX_LAYER_NUMBER]; 00132 LCD_DrvTypeDef *LcdDrv; 00133 /** 00134 * @} 00135 */ 00136 00137 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_FunctionPrototypes STM32F429I DISCOVERY LCD Private FunctionPrototypes 00138 * @{ 00139 */ 00140 static void MspInit(void); 00141 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c); 00142 static void FillBuffer(uint32_t LayerIndex, void *pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex); 00143 static void ConvertLineToARGB8888(void *pSrc, void *pDst, uint32_t xSize, uint32_t ColorMode); 00144 /** 00145 * @} 00146 */ 00147 00148 /** @defgroup STM32F429I_DISCOVERY_LCD_Private_Functions STM32F429I DISCOVERY LCD Private Functions 00149 * @{ 00150 */ 00151 00152 /** 00153 * @brief Initializes the LCD. 00154 * @retval LCD state 00155 */ 00156 uint8_t BSP_LCD_Init(void) 00157 { 00158 /* On STM32F429I-DISCO, it is not possible to read ILI9341 ID because */ 00159 /* PIN EXTC is not connected to VDD and then LCD_READ_ID4 is not accessible. */ 00160 /* In this case, ReadID function is bypassed.*/ 00161 /*if(ili9341_drv.ReadID() == ILI9341_ID)*/ 00162 00163 /* LTDC Configuration ----------------------------------------------------*/ 00164 LtdcHandler.Instance = LTDC; 00165 00166 /* Timing configuration (Typical configuration from ILI9341 datasheet) 00167 HSYNC=10 (9+1) 00168 HBP=20 (29-10+1) 00169 ActiveW=240 (269-20-10+1) 00170 HFP=10 (279-240-20-10+1) 00171 00172 VSYNC=2 (1+1) 00173 VBP=2 (3-2+1) 00174 ActiveH=320 (323-2-2+1) 00175 VFP=4 (327-320-2-2+1) 00176 */ 00177 00178 /* Configure horizontal synchronization width */ 00179 LtdcHandler.Init.HorizontalSync = ILI9341_HSYNC; 00180 /* Configure vertical synchronization height */ 00181 LtdcHandler.Init.VerticalSync = ILI9341_VSYNC; 00182 /* Configure accumulated horizontal back porch */ 00183 LtdcHandler.Init.AccumulatedHBP = ILI9341_HBP; 00184 /* Configure accumulated vertical back porch */ 00185 LtdcHandler.Init.AccumulatedVBP = ILI9341_VBP; 00186 /* Configure accumulated active width */ 00187 LtdcHandler.Init.AccumulatedActiveW = 269; 00188 /* Configure accumulated active height */ 00189 LtdcHandler.Init.AccumulatedActiveH = 323; 00190 /* Configure total width */ 00191 LtdcHandler.Init.TotalWidth = 279; 00192 /* Configure total height */ 00193 LtdcHandler.Init.TotalHeigh = 327; 00194 00195 /* Configure R,G,B component values for LCD background color */ 00196 LtdcHandler.Init.Backcolor.Red= 0; 00197 LtdcHandler.Init.Backcolor.Blue= 0; 00198 LtdcHandler.Init.Backcolor.Green= 0; 00199 00200 /* LCD clock configuration */ 00201 /* PLLSAI_VCO Input = HSE_VALUE/PLL_M = 1 Mhz */ 00202 /* PLLSAI_VCO Output = PLLSAI_VCO Input * PLLSAIN = 192 Mhz */ 00203 /* PLLLCDCLK = PLLSAI_VCO Output/PLLSAIR = 192/4 = 48 Mhz */ 00204 /* LTDC clock frequency = PLLLCDCLK / LTDC_PLLSAI_DIVR_8 = 48/4 = 6Mhz */ 00205 PeriphClkInitStruct.PeriphClockSelection = RCC_PERIPHCLK_LTDC; 00206 PeriphClkInitStruct.PLLSAI.PLLSAIN = 192; 00207 PeriphClkInitStruct.PLLSAI.PLLSAIR = 4; 00208 PeriphClkInitStruct.PLLSAIDivR = RCC_PLLSAIDIVR_8; 00209 HAL_RCCEx_PeriphCLKConfig(&PeriphClkInitStruct); 00210 00211 /* Polarity */ 00212 LtdcHandler.Init.HSPolarity = LTDC_HSPOLARITY_AL; 00213 LtdcHandler.Init.VSPolarity = LTDC_VSPOLARITY_AL; 00214 LtdcHandler.Init.DEPolarity = LTDC_DEPOLARITY_AL; 00215 LtdcHandler.Init.PCPolarity = LTDC_PCPOLARITY_IPC; 00216 00217 MspInit(); 00218 HAL_LTDC_Init(&LtdcHandler); 00219 00220 /* Select the device */ 00221 LcdDrv = &ili9341_drv; 00222 00223 /* LCD Init */ 00224 LcdDrv->Init(); 00225 00226 /* Initialize the SDRAM */ 00227 BSP_SDRAM_Init(); 00228 00229 /* Initialize the font */ 00230 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00231 00232 return LCD_OK; 00233 } 00234 00235 /** 00236 * @brief Gets the LCD X size. 00237 * @retval The used LCD X size 00238 */ 00239 uint32_t BSP_LCD_GetXSize(void) 00240 { 00241 return LcdDrv->GetLcdPixelWidth(); 00242 } 00243 00244 /** 00245 * @brief Gets the LCD Y size. 00246 * @retval The used LCD Y size 00247 */ 00248 uint32_t BSP_LCD_GetYSize(void) 00249 { 00250 return LcdDrv->GetLcdPixelHeight(); 00251 } 00252 00253 /** 00254 * @brief Initializes the LCD layers. 00255 * @param LayerIndex: the layer foreground or background. 00256 * @param FB_Address: the layer frame buffer. 00257 */ 00258 void BSP_LCD_LayerDefaultInit(uint16_t LayerIndex, uint32_t FB_Address) 00259 { 00260 LCD_LayerCfgTypeDef Layercfg; 00261 00262 /* Layer Init */ 00263 Layercfg.WindowX0 = 0; 00264 Layercfg.WindowX1 = BSP_LCD_GetXSize(); 00265 Layercfg.WindowY0 = 0; 00266 Layercfg.WindowY1 = BSP_LCD_GetYSize(); 00267 Layercfg.PixelFormat = LTDC_PIXEL_FORMAT_ARGB8888; 00268 Layercfg.FBStartAdress = FB_Address; 00269 Layercfg.Alpha = 255; 00270 Layercfg.Alpha0 = 0; 00271 Layercfg.Backcolor.Blue = 0; 00272 Layercfg.Backcolor.Green = 0; 00273 Layercfg.Backcolor.Red = 0; 00274 Layercfg.BlendingFactor1 = LTDC_BLENDING_FACTOR1_PAxCA; 00275 Layercfg.BlendingFactor2 = LTDC_BLENDING_FACTOR2_PAxCA; 00276 Layercfg.ImageWidth = BSP_LCD_GetXSize(); 00277 Layercfg.ImageHeight = BSP_LCD_GetYSize(); 00278 00279 HAL_LTDC_ConfigLayer(&LtdcHandler, &Layercfg, LayerIndex); 00280 00281 DrawProp[LayerIndex].BackColor = LCD_COLOR_WHITE; 00282 DrawProp[LayerIndex].pFont = &Font24; 00283 DrawProp[LayerIndex].TextColor = LCD_COLOR_BLACK; 00284 00285 /* Dithering activation */ 00286 HAL_LTDC_EnableDither(&LtdcHandler); 00287 } 00288 00289 /** 00290 * @brief Selects the LCD Layer. 00291 * @param LayerIndex: the Layer foreground or background. 00292 */ 00293 void BSP_LCD_SelectLayer(uint32_t LayerIndex) 00294 { 00295 ActiveLayer = LayerIndex; 00296 } 00297 00298 /** 00299 * @brief Sets a LCD Layer visible. 00300 * @param LayerIndex: the visible Layer. 00301 * @param state: new state of the specified layer. 00302 * This parameter can be: ENABLE or DISABLE. 00303 */ 00304 void BSP_LCD_SetLayerVisible(uint32_t LayerIndex, FunctionalState state) 00305 { 00306 if(state == ENABLE) 00307 { 00308 __HAL_LTDC_LAYER_ENABLE(&LtdcHandler, LayerIndex); 00309 } 00310 else 00311 { 00312 __HAL_LTDC_LAYER_DISABLE(&LtdcHandler, LayerIndex); 00313 } 00314 __HAL_LTDC_RELOAD_CONFIG(&LtdcHandler); 00315 } 00316 00317 /** 00318 * @brief Configures the Transparency. 00319 * @param LayerIndex: the Layer foreground or background. 00320 * @param Transparency: the Transparency, 00321 * This parameter must range from 0x00 to 0xFF. 00322 */ 00323 void BSP_LCD_SetTransparency(uint32_t LayerIndex, uint8_t Transparency) 00324 { 00325 HAL_LTDC_SetAlpha(&LtdcHandler, Transparency, LayerIndex); 00326 } 00327 00328 /** 00329 * @brief Sets a LCD layer frame buffer address. 00330 * @param LayerIndex: specifies the Layer foreground or background 00331 * @param Address: new LCD frame buffer value 00332 */ 00333 void BSP_LCD_SetLayerAddress(uint32_t LayerIndex, uint32_t Address) 00334 { 00335 HAL_LTDC_SetAddress(&LtdcHandler, Address, LayerIndex); 00336 } 00337 00338 /** 00339 * @brief Sets the Display window. 00340 * @param LayerIndex: layer index 00341 * @param Xpos: LCD X position 00342 * @param Ypos: LCD Y position 00343 * @param Width: LCD window width 00344 * @param Height: LCD window height 00345 */ 00346 void BSP_LCD_SetLayerWindow(uint16_t LayerIndex, uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00347 { 00348 /* reconfigure the layer size */ 00349 HAL_LTDC_SetWindowSize(&LtdcHandler, Width, Height, LayerIndex); 00350 00351 /* reconfigure the layer position */ 00352 HAL_LTDC_SetWindowPosition(&LtdcHandler, Xpos, Ypos, LayerIndex); 00353 } 00354 00355 /** 00356 * @brief Configures and sets the color Keying. 00357 * @param LayerIndex: the Layer foreground or background 00358 * @param RGBValue: the Color reference 00359 */ 00360 void BSP_LCD_SetColorKeying(uint32_t LayerIndex, uint32_t RGBValue) 00361 { 00362 /* Configure and Enable the color Keying for LCD Layer */ 00363 HAL_LTDC_ConfigColorKeying(&LtdcHandler, RGBValue, LayerIndex); 00364 HAL_LTDC_EnableColorKeying(&LtdcHandler, LayerIndex); 00365 } 00366 00367 /** 00368 * @brief Disables the color Keying. 00369 * @param LayerIndex: the Layer foreground or background 00370 */ 00371 void BSP_LCD_ResetColorKeying(uint32_t LayerIndex) 00372 { 00373 /* Disable the color Keying for LCD Layer */ 00374 HAL_LTDC_DisableColorKeying(&LtdcHandler, LayerIndex); 00375 } 00376 00377 /** 00378 * @brief Gets the LCD Text color. 00379 * @retval Text color 00380 */ 00381 uint32_t BSP_LCD_GetTextColor(void) 00382 { 00383 return DrawProp[ActiveLayer].TextColor; 00384 } 00385 00386 /** 00387 * @brief Gets the LCD Background color. 00388 * @retval Background color 00389 */ 00390 uint32_t BSP_LCD_GetBackColor(void) 00391 { 00392 return DrawProp[ActiveLayer].BackColor; 00393 } 00394 00395 /** 00396 * @brief Sets the Text color. 00397 * @param Color: the Text color code ARGB(8-8-8-8) 00398 */ 00399 void BSP_LCD_SetTextColor(uint32_t Color) 00400 { 00401 DrawProp[ActiveLayer].TextColor = Color; 00402 } 00403 00404 /** 00405 * @brief Sets the Background color. 00406 * @param Color: the layer Background color code ARGB(8-8-8-8) 00407 */ 00408 void BSP_LCD_SetBackColor(uint32_t Color) 00409 { 00410 DrawProp[ActiveLayer].BackColor = Color; 00411 } 00412 00413 /** 00414 * @brief Sets the Text Font. 00415 * @param pFonts: the layer font to be used 00416 */ 00417 void BSP_LCD_SetFont(sFONT *pFonts) 00418 { 00419 DrawProp[ActiveLayer].pFont = pFonts; 00420 } 00421 00422 /** 00423 * @brief Gets the Text Font. 00424 * @retval Layer font 00425 */ 00426 sFONT *BSP_LCD_GetFont(void) 00427 { 00428 return DrawProp[ActiveLayer].pFont; 00429 } 00430 00431 /** 00432 * @brief Reads Pixel. 00433 * @param Xpos: the X position 00434 * @param Ypos: the Y position 00435 * @retval RGB pixel color 00436 */ 00437 uint32_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00438 { 00439 uint32_t ret = 0; 00440 00441 if(LtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB8888) 00442 { 00443 /* Read data value from SDRAM memory */ 00444 ret = *(__IO uint32_t*) (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00445 } 00446 else if(LtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB888) 00447 { 00448 /* Read data value from SDRAM memory */ 00449 ret = (*(__IO uint32_t*) (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))) & 0x00FFFFFF); 00450 } 00451 else if((LtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_RGB565) || \ 00452 (LtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_ARGB4444) || \ 00453 (LtdcHandler.LayerCfg[ActiveLayer].PixelFormat == LTDC_PIXEL_FORMAT_AL88)) 00454 { 00455 /* Read data value from SDRAM memory */ 00456 ret = *(__IO uint16_t*) (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00457 } 00458 else 00459 { 00460 /* Read data value from SDRAM memory */ 00461 ret = *(__IO uint8_t*) (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (2*(Ypos*BSP_LCD_GetXSize() + Xpos))); 00462 } 00463 00464 return ret; 00465 } 00466 00467 /** 00468 * @brief Clears the hole LCD. 00469 * @param Color: the color of the background 00470 */ 00471 void BSP_LCD_Clear(uint32_t Color) 00472 { 00473 /* Clear the LCD */ 00474 FillBuffer(ActiveLayer, (uint32_t *)(LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress), BSP_LCD_GetXSize(), BSP_LCD_GetYSize(), 0, Color); 00475 } 00476 00477 /** 00478 * @brief Clears the selected line. 00479 * @param Line: the line to be cleared 00480 */ 00481 void BSP_LCD_ClearStringLine(uint32_t Line) 00482 { 00483 uint32_t colorbackup = DrawProp[ActiveLayer].TextColor; 00484 DrawProp[ActiveLayer].TextColor = DrawProp[ActiveLayer].BackColor; 00485 00486 /* Draw rectangle with background color */ 00487 BSP_LCD_FillRect(0, (Line * DrawProp[ActiveLayer].pFont->Height), BSP_LCD_GetXSize(), DrawProp[ActiveLayer].pFont->Height); 00488 00489 DrawProp[ActiveLayer].TextColor = colorbackup; 00490 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00491 } 00492 00493 /** 00494 * @brief Displays one character. 00495 * @param Xpos: start column address 00496 * @param Ypos: the Line where to display the character shape 00497 * @param Ascii: character ascii code, must be between 0x20 and 0x7E 00498 */ 00499 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00500 { 00501 DrawChar(Xpos, Ypos, &DrawProp[ActiveLayer].pFont->table[(Ascii-' ') *\ 00502 DrawProp[ActiveLayer].pFont->Height * ((DrawProp[ActiveLayer].pFont->Width + 7) / 8)]); 00503 } 00504 00505 /** 00506 * @brief Displays a maximum of 60 char on the LCD. 00507 * @param X: pointer to x position (in pixel) 00508 * @param Y: pointer to y position (in pixel) 00509 * @param pText: pointer to string to display on LCD 00510 * @param mode: The display mode 00511 * This parameter can be one of the following values: 00512 * @arg CENTER_MODE 00513 * @arg RIGHT_MODE 00514 * @arg LEFT_MODE 00515 */ 00516 void BSP_LCD_DisplayStringAt(uint16_t X, uint16_t Y, uint8_t *pText, Text_AlignModeTypdef mode) 00517 { 00518 uint16_t refcolumn = 1, i = 0; 00519 uint32_t size = 0, xsize = 0; 00520 uint8_t *ptr = pText; 00521 00522 /* Get the text size */ 00523 while (*ptr++) size ++ ; 00524 00525 /* Characters number per line */ 00526 xsize = (BSP_LCD_GetXSize()/DrawProp[ActiveLayer].pFont->Width); 00527 00528 switch (mode) 00529 { 00530 case CENTER_MODE: 00531 { 00532 refcolumn = X+ ((xsize - size)* DrawProp[ActiveLayer].pFont->Width) / 2; 00533 break; 00534 } 00535 case LEFT_MODE: 00536 { 00537 refcolumn = X; 00538 break; 00539 } 00540 case RIGHT_MODE: 00541 { 00542 refcolumn = X + ((xsize - size)*DrawProp[ActiveLayer].pFont->Width); 00543 break; 00544 } 00545 default: 00546 { 00547 refcolumn = X; 00548 break; 00549 } 00550 } 00551 00552 /* Send the string character by character on LCD */ 00553 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (i*DrawProp[ActiveLayer].pFont->Width)) & 0xFFFF) >= DrawProp[ActiveLayer].pFont->Width)) 00554 { 00555 /* Display one character on LCD */ 00556 BSP_LCD_DisplayChar(refcolumn, Y, *pText); 00557 /* Decrement the column position by 16 */ 00558 refcolumn += DrawProp[ActiveLayer].pFont->Width; 00559 /* Point on the next character */ 00560 pText++; 00561 i++; 00562 } 00563 } 00564 00565 /** 00566 * @brief Displays a maximum of 20 char on the LCD. 00567 * @param Line: the Line where to display the character shape 00568 * @param ptr: pointer to string to display on LCD 00569 */ 00570 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr) 00571 { 00572 BSP_LCD_DisplayStringAt(0, LINE(Line), ptr, LEFT_MODE); 00573 } 00574 00575 /** 00576 * @brief Displays an horizontal line. 00577 * @param Xpos: the X position 00578 * @param Ypos: the Y position 00579 * @param Length: line length 00580 */ 00581 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00582 { 00583 uint32_t xaddress = 0; 00584 00585 /* Get the line address */ 00586 xaddress = (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00587 00588 /* Write line */ 00589 FillBuffer(ActiveLayer, (uint32_t *)xaddress, Length, 1, 0, DrawProp[ActiveLayer].TextColor); 00590 } 00591 00592 /** 00593 * @brief Displays a vertical line. 00594 * @param Xpos: the X position 00595 * @param Ypos: the Y position 00596 * @param Length: line length 00597 */ 00598 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00599 { 00600 uint32_t xaddress = 0; 00601 00602 /* Get the line address */ 00603 xaddress = (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00604 00605 /* Write line */ 00606 FillBuffer(ActiveLayer, (uint32_t *)xaddress, 1, Length, (BSP_LCD_GetXSize() - 1), DrawProp[ActiveLayer].TextColor); 00607 } 00608 00609 /** 00610 * @brief Displays an uni-line (between two points). 00611 * @param X1: the point 1 X position 00612 * @param Y1: the point 1 Y position 00613 * @param X2: the point 2 X position 00614 * @param Y2: the point 2 Y position 00615 */ 00616 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2) 00617 { 00618 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00619 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00620 curpixel = 0; 00621 00622 deltax = ABS(X2 - X1); /* The difference between the x's */ 00623 deltay = ABS(Y2 - Y1); /* The difference between the y's */ 00624 x = X1; /* Start x off at the first pixel */ 00625 y = Y1; /* Start y off at the first pixel */ 00626 00627 if (X2 >= X1) /* The x-values are increasing */ 00628 { 00629 xinc1 = 1; 00630 xinc2 = 1; 00631 } 00632 else /* The x-values are decreasing */ 00633 { 00634 xinc1 = -1; 00635 xinc2 = -1; 00636 } 00637 00638 if (Y2 >= Y1) /* The y-values are increasing */ 00639 { 00640 yinc1 = 1; 00641 yinc2 = 1; 00642 } 00643 else /* The y-values are decreasing */ 00644 { 00645 yinc1 = -1; 00646 yinc2 = -1; 00647 } 00648 00649 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00650 { 00651 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00652 yinc2 = 0; /* Don't change the y for every iteration */ 00653 den = deltax; 00654 num = deltax / 2; 00655 numadd = deltay; 00656 numpixels = deltax; /* There are more x-values than y-values */ 00657 } 00658 else /* There is at least one y-value for every x-value */ 00659 { 00660 xinc2 = 0; /* Don't change the x for every iteration */ 00661 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00662 den = deltay; 00663 num = deltay / 2; 00664 numadd = deltax; 00665 numpixels = deltay; /* There are more y-values than x-values */ 00666 } 00667 00668 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00669 { 00670 BSP_LCD_DrawPixel(x, y, DrawProp[ActiveLayer].TextColor); /* Draw the current pixel */ 00671 num += numadd; /* Increase the numerator by the top of the fraction */ 00672 if (num >= den) /* Check if numerator >= denominator */ 00673 { 00674 num -= den; /* Calculate the new numerator value */ 00675 x += xinc1; /* Change the x as appropriate */ 00676 y += yinc1; /* Change the y as appropriate */ 00677 } 00678 x += xinc2; /* Change the x as appropriate */ 00679 y += yinc2; /* Change the y as appropriate */ 00680 } 00681 } 00682 00683 /** 00684 * @brief Displays a rectangle. 00685 * @param Xpos: the X position 00686 * @param Ypos: the Y position 00687 * @param Height: display rectangle height 00688 * @param Width: display rectangle width 00689 */ 00690 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00691 { 00692 /* Draw horizontal lines */ 00693 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00694 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00695 00696 /* Draw vertical lines */ 00697 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00698 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00699 } 00700 00701 /** 00702 * @brief Displays a circle. 00703 * @param Xpos: the X position 00704 * @param Ypos: the Y position 00705 * @param Radius: the circle radius 00706 */ 00707 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00708 { 00709 int32_t d;/* Decision Variable */ 00710 uint32_t curx;/* Current X Value */ 00711 uint32_t cury;/* Current Y Value */ 00712 00713 d = 3 - (Radius << 1); 00714 curx = 0; 00715 cury = Radius; 00716 00717 while (curx <= cury) 00718 { 00719 BSP_LCD_DrawPixel((Xpos + curx), (Ypos - cury), DrawProp[ActiveLayer].TextColor); 00720 BSP_LCD_DrawPixel((Xpos - curx), (Ypos - cury), DrawProp[ActiveLayer].TextColor); 00721 BSP_LCD_DrawPixel((Xpos + cury), (Ypos - curx), DrawProp[ActiveLayer].TextColor); 00722 BSP_LCD_DrawPixel((Xpos - cury), (Ypos - curx), DrawProp[ActiveLayer].TextColor); 00723 BSP_LCD_DrawPixel((Xpos + curx), (Ypos + cury), DrawProp[ActiveLayer].TextColor); 00724 BSP_LCD_DrawPixel((Xpos - curx), (Ypos + cury), DrawProp[ActiveLayer].TextColor); 00725 BSP_LCD_DrawPixel((Xpos + cury), (Ypos + curx), DrawProp[ActiveLayer].TextColor); 00726 BSP_LCD_DrawPixel((Xpos - cury), (Ypos + curx), DrawProp[ActiveLayer].TextColor); 00727 00728 if (d < 0) 00729 { 00730 d += (curx << 2) + 6; 00731 } 00732 else 00733 { 00734 d += ((curx - cury) << 2) + 10; 00735 cury--; 00736 } 00737 curx++; 00738 } 00739 } 00740 00741 /** 00742 * @brief Displays an poly-line (between many points). 00743 * @param Points: pointer to the points array 00744 * @param PointCount: Number of points 00745 */ 00746 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount) 00747 { 00748 int16_t x = 0, y = 0; 00749 00750 if(PointCount < 2) 00751 { 00752 return; 00753 } 00754 00755 BSP_LCD_DrawLine(Points->X, Points->Y, (Points+PointCount-1)->X, (Points+PointCount-1)->Y); 00756 00757 while(--PointCount) 00758 { 00759 x = Points->X; 00760 y = Points->Y; 00761 Points++; 00762 BSP_LCD_DrawLine(x, y, Points->X, Points->Y); 00763 } 00764 } 00765 00766 /** 00767 * @brief Displays an Ellipse. 00768 * @param Xpos: the X position 00769 * @param Ypos: the Y position 00770 * @param XRadius: the X radius of ellipse 00771 * @param YRadius: the Y radius of ellipse 00772 */ 00773 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00774 { 00775 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 00776 float k = 0, rad1 = 0, rad2 = 0; 00777 00778 rad1 = XRadius; 00779 rad2 = YRadius; 00780 00781 k = (float)(rad2/rad1); 00782 00783 do { 00784 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00785 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos+y), DrawProp[ActiveLayer].TextColor); 00786 BSP_LCD_DrawPixel((Xpos+(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00787 BSP_LCD_DrawPixel((Xpos-(uint16_t)(x/k)), (Ypos-y), DrawProp[ActiveLayer].TextColor); 00788 00789 e2 = err; 00790 if (e2 <= x) { 00791 err += ++x*2+1; 00792 if (-y == x && e2 <= y) e2 = 0; 00793 } 00794 if (e2 > y) err += ++y*2+1; 00795 } 00796 while (y <= 0); 00797 } 00798 00799 /** 00800 * @brief Displays a bitmap picture loaded in the internal Flash (32 bpp). 00801 * @param X: the bmp x position in the LCD 00802 * @param Y: the bmp Y position in the LCD 00803 * @param pBmp: Bmp picture address in the internal Flash 00804 */ 00805 void BSP_LCD_DrawBitmap(uint32_t X, uint32_t Y, uint8_t *pBmp) 00806 { 00807 uint32_t index = 0, width = 0, height = 0, bitpixel = 0; 00808 uint32_t address; 00809 uint32_t inputcolormode = 0; 00810 00811 /* Get bitmap data address offset */ 00812 index = *(__IO uint16_t *) (pBmp + 10); 00813 index |= (*(__IO uint16_t *) (pBmp + 12)) << 16; 00814 00815 /* Read bitmap width */ 00816 width = *(uint16_t *) (pBmp + 18); 00817 width |= (*(uint16_t *) (pBmp + 20)) << 16; 00818 00819 /* Read bitmap height */ 00820 height = *(uint16_t *) (pBmp + 22); 00821 height |= (*(uint16_t *) (pBmp + 24)) << 16; 00822 00823 /* Read bit/pixel */ 00824 bitpixel = *(uint16_t *) (pBmp + 28); 00825 00826 /* Set Address */ 00827 address = LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (((BSP_LCD_GetXSize()*Y) + X)*(4)); 00828 00829 /* Get the Layer pixel format */ 00830 if ((bitpixel/8) == 4) 00831 { 00832 inputcolormode = CM_ARGB8888; 00833 } 00834 else if ((bitpixel/8) == 2) 00835 { 00836 inputcolormode = CM_RGB565; 00837 } 00838 else 00839 { 00840 inputcolormode = CM_RGB888; 00841 } 00842 00843 /* bypass the bitmap header */ 00844 pBmp += (index + (width * (height - 1) * (bitpixel/8))); 00845 00846 /* Convert picture to ARGB8888 pixel format */ 00847 for(index=0; index < height; index++) 00848 { 00849 /* Pixel format conversion */ 00850 ConvertLineToARGB8888((uint32_t *)pBmp, (uint32_t *)address, width, inputcolormode); 00851 00852 /* Increment the source and destination buffers */ 00853 address+= ((BSP_LCD_GetXSize() - width + width)*4); 00854 pBmp -= width*(bitpixel/8); 00855 } 00856 } 00857 00858 /** 00859 * @brief Displays a full rectangle. 00860 * @param Xpos: the X position 00861 * @param Ypos: the Y position 00862 * @param Height: rectangle height 00863 * @param Width: rectangle width 00864 */ 00865 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00866 { 00867 uint32_t xaddress = 0; 00868 00869 /* Set the text color */ 00870 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00871 00872 /* Get the rectangle start address */ 00873 xaddress = (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress) + 4*(BSP_LCD_GetXSize()*Ypos + Xpos); 00874 00875 /* Fill the rectangle */ 00876 FillBuffer(ActiveLayer, (uint32_t *)xaddress, Width, Height, (BSP_LCD_GetXSize() - Width), DrawProp[ActiveLayer].TextColor); 00877 } 00878 00879 /** 00880 * @brief Displays a full circle. 00881 * @param Xpos: the X position 00882 * @param Ypos: the Y position 00883 * @param Radius: the circle radius 00884 */ 00885 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00886 { 00887 int32_t d; /* Decision Variable */ 00888 uint32_t curx;/* Current X Value */ 00889 uint32_t cury;/* Current Y Value */ 00890 00891 d = 3 - (Radius << 1); 00892 00893 curx = 0; 00894 cury = Radius; 00895 00896 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00897 00898 while (curx <= cury) 00899 { 00900 if(cury > 0) 00901 { 00902 BSP_LCD_DrawHLine(Xpos - cury, Ypos + curx, 2*cury); 00903 BSP_LCD_DrawHLine(Xpos - cury, Ypos - curx, 2*cury); 00904 } 00905 00906 if(curx > 0) 00907 { 00908 BSP_LCD_DrawHLine(Xpos - curx, Ypos - cury, 2*curx); 00909 BSP_LCD_DrawHLine(Xpos - curx, Ypos + cury, 2*curx); 00910 } 00911 if (d < 0) 00912 { 00913 d += (curx << 2) + 6; 00914 } 00915 else 00916 { 00917 d += ((curx - cury) << 2) + 10; 00918 cury--; 00919 } 00920 curx++; 00921 } 00922 00923 BSP_LCD_SetTextColor(DrawProp[ActiveLayer].TextColor); 00924 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00925 } 00926 00927 /** 00928 * @brief Fill triangle. 00929 * @param X1: the point 1 x position 00930 * @param Y1: the point 1 y position 00931 * @param X2: the point 2 x position 00932 * @param Y2: the point 2 y position 00933 * @param X3: the point 3 x position 00934 * @param Y3: the point 3 y position 00935 */ 00936 void BSP_LCD_FillTriangle(uint16_t X1, uint16_t X2, uint16_t X3, uint16_t Y1, uint16_t Y2, uint16_t Y3) 00937 { 00938 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00939 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00940 curpixel = 0; 00941 00942 deltax = ABS(X2 - X1); /* The difference between the x's */ 00943 deltay = ABS(Y2 - Y1); /* The difference between the y's */ 00944 x = X1; /* Start x off at the first pixel */ 00945 y = Y1; /* Start y off at the first pixel */ 00946 00947 if (X2 >= X1) /* The x-values are increasing */ 00948 { 00949 xinc1 = 1; 00950 xinc2 = 1; 00951 } 00952 else /* The x-values are decreasing */ 00953 { 00954 xinc1 = -1; 00955 xinc2 = -1; 00956 } 00957 00958 if (Y2 >= Y1) /* The y-values are increasing */ 00959 { 00960 yinc1 = 1; 00961 yinc2 = 1; 00962 } 00963 else /* The y-values are decreasing */ 00964 { 00965 yinc1 = -1; 00966 yinc2 = -1; 00967 } 00968 00969 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00970 { 00971 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00972 yinc2 = 0; /* Don't change the y for every iteration */ 00973 den = deltax; 00974 num = deltax / 2; 00975 numadd = deltay; 00976 numpixels = deltax; /* There are more x-values than y-values */ 00977 } 00978 else /* There is at least one y-value for every x-value */ 00979 { 00980 xinc2 = 0; /* Don't change the x for every iteration */ 00981 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00982 den = deltay; 00983 num = deltay / 2; 00984 numadd = deltax; 00985 numpixels = deltay; /* There are more y-values than x-values */ 00986 } 00987 00988 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00989 { 00990 BSP_LCD_DrawLine(x, y, X3, Y3); 00991 00992 num += numadd; /* Increase the numerator by the top of the fraction */ 00993 if (num >= den) /* Check if numerator >= denominator */ 00994 { 00995 num -= den; /* Calculate the new numerator value */ 00996 x += xinc1; /* Change the x as appropriate */ 00997 y += yinc1; /* Change the y as appropriate */ 00998 } 00999 x += xinc2; /* Change the x as appropriate */ 01000 y += yinc2; /* Change the y as appropriate */ 01001 } 01002 } 01003 01004 /** 01005 * @brief Displays a full poly-line (between many points). 01006 * @param Points: pointer to the points array 01007 * @param PointCount: Number of points 01008 */ 01009 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount) 01010 { 01011 01012 int16_t x = 0, y = 0, x2 = 0, y2 = 0, xcenter = 0, ycenter = 0, xfirst = 0, yfirst = 0, pixelx = 0, pixely = 0, counter = 0; 01013 uint16_t imageleft = 0, imageright = 0, imagetop = 0, imagebottom = 0; 01014 01015 imageleft = imageright = Points->X; 01016 imagetop= imagebottom = Points->Y; 01017 01018 for(counter = 1; counter < PointCount; counter++) 01019 { 01020 pixelx = POLY_X(counter); 01021 if(pixelx < imageleft) 01022 { 01023 imageleft = pixelx; 01024 } 01025 if(pixelx > imageright) 01026 { 01027 imageright = pixelx; 01028 } 01029 01030 pixely = POLY_Y(counter); 01031 if(pixely < imagetop) 01032 { 01033 imagetop = pixely; 01034 } 01035 if(pixely > imagebottom) 01036 { 01037 imagebottom = pixely; 01038 } 01039 } 01040 01041 if(PointCount < 2) 01042 { 01043 return; 01044 } 01045 01046 xcenter = (imageleft + imageright)/2; 01047 ycenter = (imagebottom + imagetop)/2; 01048 01049 xfirst = Points->X; 01050 yfirst = Points->Y; 01051 01052 while(--PointCount) 01053 { 01054 x = Points->X; 01055 y = Points->Y; 01056 Points++; 01057 x2 = Points->X; 01058 y2 = Points->Y; 01059 01060 BSP_LCD_FillTriangle(x, x2, xcenter, y, y2, ycenter); 01061 BSP_LCD_FillTriangle(x, xcenter, x2, y, ycenter, y2); 01062 BSP_LCD_FillTriangle(xcenter, x2, x, ycenter, y2, y); 01063 } 01064 01065 BSP_LCD_FillTriangle(xfirst, x2, xcenter, yfirst, y2, ycenter); 01066 BSP_LCD_FillTriangle(xfirst, xcenter, x2, yfirst, ycenter, y2); 01067 BSP_LCD_FillTriangle(xcenter, x2, xfirst, ycenter, y2, yfirst); 01068 } 01069 01070 /** 01071 * @brief Draw a full ellipse. 01072 * @param Xpos: the X position 01073 * @param Ypos: the Y position 01074 * @param XRadius: X radius of ellipse 01075 * @param YRadius: Y radius of ellipse. 01076 */ 01077 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 01078 { 01079 int x = 0, y = -YRadius, err = 2-2*XRadius, e2; 01080 float K = 0, rad1 = 0, rad2 = 0; 01081 01082 rad1 = XRadius; 01083 rad2 = YRadius; 01084 K = (float)(rad2/rad1); 01085 01086 do 01087 { 01088 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos+y), (2*(uint16_t)(x/K) + 1)); 01089 BSP_LCD_DrawHLine((Xpos-(uint16_t)(x/K)), (Ypos-y), (2*(uint16_t)(x/K) + 1)); 01090 01091 e2 = err; 01092 if (e2 <= x) 01093 { 01094 err += ++x*2+1; 01095 if (-y == x && e2 <= y) e2 = 0; 01096 } 01097 if (e2 > y) err += ++y*2+1; 01098 } 01099 while (y <= 0); 01100 } 01101 01102 /** 01103 * @brief Enables the Display. 01104 */ 01105 void BSP_LCD_DisplayOn(void) 01106 { 01107 if(LcdDrv->DisplayOn != NULL) 01108 { 01109 LcdDrv->DisplayOn(); 01110 } 01111 } 01112 01113 /** 01114 * @brief Disables the Display. 01115 */ 01116 void BSP_LCD_DisplayOff(void) 01117 { 01118 if(LcdDrv->DisplayOff != NULL) 01119 { 01120 LcdDrv->DisplayOff(); 01121 } 01122 } 01123 01124 /******************************************************************************* 01125 LTDC and DMA2D BSP Routines 01126 *******************************************************************************/ 01127 01128 /** 01129 * @brief Initializes the LTDC MSP. 01130 */ 01131 static void MspInit(void) 01132 { 01133 GPIO_InitTypeDef GPIO_InitStructure; 01134 01135 /* Enable the LTDC and DMA2D Clock */ 01136 __LTDC_CLK_ENABLE(); 01137 __DMA2D_CLK_ENABLE(); 01138 01139 /* Enable GPIOs clock */ 01140 __GPIOA_CLK_ENABLE(); 01141 __GPIOB_CLK_ENABLE(); 01142 __GPIOC_CLK_ENABLE(); 01143 __GPIOD_CLK_ENABLE(); 01144 __GPIOF_CLK_ENABLE(); 01145 __GPIOG_CLK_ENABLE(); 01146 01147 /* GPIOs Configuration */ 01148 /* 01149 +------------------------+-----------------------+----------------------------+ 01150 + LCD pins assignment + 01151 +------------------------+-----------------------+----------------------------+ 01152 | LCD_TFT R2 <-> PC.10 | LCD_TFT G2 <-> PA.06 | LCD_TFT B2 <-> PD.06 | 01153 | LCD_TFT R3 <-> PB.00 | LCD_TFT G3 <-> PG.10 | LCD_TFT B3 <-> PG.11 | 01154 | LCD_TFT R4 <-> PA.11 | LCD_TFT G4 <-> PB.10 | LCD_TFT B4 <-> PG.12 | 01155 | LCD_TFT R5 <-> PA.12 | LCD_TFT G5 <-> PB.11 | LCD_TFT B5 <-> PA.03 | 01156 | LCD_TFT R6 <-> PB.01 | LCD_TFT G6 <-> PC.07 | LCD_TFT B6 <-> PB.08 | 01157 | LCD_TFT R7 <-> PG.06 | LCD_TFT G7 <-> PD.03 | LCD_TFT B7 <-> PB.09 | 01158 ------------------------------------------------------------------------------- 01159 | LCD_TFT HSYNC <-> PC.06 | LCDTFT VSYNC <-> PA.04 | 01160 | LCD_TFT CLK <-> PG.07 | LCD_TFT DE <-> PF.10 | 01161 ----------------------------------------------------- 01162 */ 01163 01164 /* GPIOA configuration */ 01165 GPIO_InitStructure.Pin = GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_6 | 01166 GPIO_PIN_11 | GPIO_PIN_12; 01167 GPIO_InitStructure.Mode = GPIO_MODE_AF_PP; 01168 GPIO_InitStructure.Pull = GPIO_NOPULL; 01169 GPIO_InitStructure.Speed = GPIO_SPEED_FAST; 01170 GPIO_InitStructure.Alternate= GPIO_AF14_LTDC; 01171 HAL_GPIO_Init(GPIOA, &GPIO_InitStructure); 01172 01173 /* GPIOB configuration */ 01174 GPIO_InitStructure.Pin = GPIO_PIN_8 | \ 01175 GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11; 01176 HAL_GPIO_Init(GPIOB, &GPIO_InitStructure); 01177 01178 /* GPIOC configuration */ 01179 GPIO_InitStructure.Pin = GPIO_PIN_6 | GPIO_PIN_7 | GPIO_PIN_10; 01180 HAL_GPIO_Init(GPIOC, &GPIO_InitStructure); 01181 01182 /* GPIOD configuration */ 01183 GPIO_InitStructure.Pin = GPIO_PIN_3 | GPIO_PIN_6; 01184 HAL_GPIO_Init(GPIOD, &GPIO_InitStructure); 01185 01186 /* GPIOF configuration */ 01187 GPIO_InitStructure.Pin = GPIO_PIN_10; 01188 HAL_GPIO_Init(GPIOF, &GPIO_InitStructure); 01189 01190 /* GPIOG configuration */ 01191 GPIO_InitStructure.Pin = GPIO_PIN_6 | GPIO_PIN_7 | \ 01192 GPIO_PIN_11; 01193 HAL_GPIO_Init(GPIOG, &GPIO_InitStructure); 01194 01195 /* GPIOB configuration */ 01196 GPIO_InitStructure.Pin = GPIO_PIN_0 | GPIO_PIN_1; 01197 GPIO_InitStructure.Alternate= GPIO_AF9_LTDC; 01198 HAL_GPIO_Init(GPIOB, &GPIO_InitStructure); 01199 01200 /* GPIOG configuration */ 01201 GPIO_InitStructure.Pin = GPIO_PIN_10 | GPIO_PIN_12; 01202 HAL_GPIO_Init(GPIOG, &GPIO_InitStructure); 01203 } 01204 01205 /******************************************************************************* 01206 Static Functions 01207 *******************************************************************************/ 01208 01209 /** 01210 * @brief Writes Pixel. 01211 * @param Xpos: the X position 01212 * @param Ypos: the Y position 01213 * @param RGB_Code: the pixel color in ARGB mode (8-8-8-8) 01214 */ 01215 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint32_t RGB_Code) 01216 { 01217 /* Write data value to all SDRAM memory */ 01218 *(__IO uint32_t*) (LtdcHandler.LayerCfg[ActiveLayer].FBStartAdress + (4*(Ypos*BSP_LCD_GetXSize() + Xpos))) = RGB_Code; 01219 } 01220 01221 /** 01222 * @brief Draws a character on LCD. 01223 * @param Xpos: the Line where to display the character shape 01224 * @param Ypos: start column address 01225 * @param c: pointer to the character data 01226 */ 01227 static void DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *c) 01228 { 01229 uint32_t i = 0, j = 0; 01230 uint16_t height, width; 01231 uint8_t offset; 01232 uint8_t *pchar; 01233 uint32_t line=0; 01234 01235 height = DrawProp[ActiveLayer].pFont->Height; 01236 width = DrawProp[ActiveLayer].pFont->Width; 01237 01238 offset = 8 *((width + 7)/8) - width ; 01239 01240 for(i = 0; i < height; i++) 01241 { 01242 pchar = ((uint8_t *)c + (width + 7)/8 * i); 01243 01244 switch(((width + 7)/8)) 01245 { 01246 case 1: 01247 line = pchar[0]; 01248 break; 01249 01250 case 2: 01251 line = (pchar[0]<< 8) | pchar[1]; 01252 break; 01253 01254 case 3: 01255 default: 01256 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01257 break; 01258 } 01259 01260 for (j = 0; j < width; j++) 01261 { 01262 if(line & (1 << (width- j + offset- 1))) 01263 { 01264 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].TextColor); 01265 } 01266 else 01267 { 01268 BSP_LCD_DrawPixel((Xpos + j), Ypos, DrawProp[ActiveLayer].BackColor); 01269 } 01270 } 01271 Ypos++; 01272 } 01273 } 01274 01275 /** 01276 * @brief Fills buffer. 01277 * @param LayerIndex: layer index 01278 * @param pDst: output color 01279 * @param xSize: buffer width 01280 * @param ySize: buffer height 01281 * @param OffLine: offset 01282 * @param ColorIndex: color Index 01283 */ 01284 static void FillBuffer(uint32_t LayerIndex, void * pDst, uint32_t xSize, uint32_t ySize, uint32_t OffLine, uint32_t ColorIndex) 01285 { 01286 01287 /* Register to memory mode with ARGB8888 as color Mode */ 01288 Dma2dHandler.Init.Mode = DMA2D_R2M; 01289 Dma2dHandler.Init.ColorMode = DMA2D_ARGB8888; 01290 Dma2dHandler.Init.OutputOffset = OffLine; 01291 01292 Dma2dHandler.Instance = DMA2D; 01293 01294 /* DMA2D Initialization */ 01295 if(HAL_DMA2D_Init(&Dma2dHandler) == HAL_OK) 01296 { 01297 if(HAL_DMA2D_ConfigLayer(&Dma2dHandler, LayerIndex) == HAL_OK) 01298 { 01299 if (HAL_DMA2D_Start(&Dma2dHandler, ColorIndex, (uint32_t)pDst, xSize, ySize) == HAL_OK) 01300 { 01301 /* Polling For DMA transfer */ 01302 HAL_DMA2D_PollForTransfer(&Dma2dHandler, 10); 01303 } 01304 } 01305 } 01306 } 01307 01308 /** 01309 * @brief Converts Line to ARGB8888 pixel format. 01310 * @param pSrc: pointer to source buffer 01311 * @param pDst: output color 01312 * @param xSize: buffer width 01313 * @param ColorMode: input color mode 01314 */ 01315 static void ConvertLineToARGB8888(void * pSrc, void * pDst, uint32_t xSize, uint32_t ColorMode) 01316 { 01317 /* Configure the DMA2D Mode, Color Mode and output offset */ 01318 Dma2dHandler.Init.Mode = DMA2D_M2M_PFC; 01319 Dma2dHandler.Init.ColorMode = DMA2D_ARGB8888; 01320 Dma2dHandler.Init.OutputOffset = 0; 01321 01322 /* Foreground Configuration */ 01323 Dma2dHandler.LayerCfg[1].AlphaMode = DMA2D_NO_MODIF_ALPHA; 01324 Dma2dHandler.LayerCfg[1].InputAlpha = 0xFF; 01325 Dma2dHandler.LayerCfg[1].InputColorMode = ColorMode; 01326 Dma2dHandler.LayerCfg[1].InputOffset = 0; 01327 01328 Dma2dHandler.Instance = DMA2D; 01329 01330 /* DMA2D Initialization */ 01331 if(HAL_DMA2D_Init(&Dma2dHandler) == HAL_OK) 01332 { 01333 if(HAL_DMA2D_ConfigLayer(&Dma2dHandler, 1) == HAL_OK) 01334 { 01335 if (HAL_DMA2D_Start(&Dma2dHandler, (uint32_t)pSrc, (uint32_t)pDst, xSize, 1) == HAL_OK) 01336 { 01337 /* Polling For DMA transfer */ 01338 HAL_DMA2D_PollForTransfer(&Dma2dHandler, 10); 01339 } 01340 } 01341 } 01342 } 01343 01344 /** 01345 * @} 01346 */ 01347 01348 /** 01349 * @} 01350 */ 01351 01352 /** 01353 * @} 01354 */ 01355 01356 /** 01357 * @} 01358 */ 01359 01360 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 13:22:14 for STM32F429I-Discovery BSP User Manual by
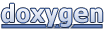