STM32F429I-Discovery BSP User Manual
|
stm32f429i_discovery_eeprom.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f429i_discovery_eeprom.h 00004 * @author MCD Application Team 00005 * @version V2.1.3 00006 * @date 13-January-2016 00007 * @brief This file contains all the functions prototypes for 00008 * the stm32f429i_discovery_eeprom.c firmware driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2015 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32F429I_DISCOVERY_EEPROM_H 00041 #define __STM32F429I_DISCOVERY_EEPROM_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32f429i_discovery.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32F429I_DISCOVERY 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM32F429I_DISCOVERY_EEPROM STM32F429I DISCOVERY EEPROM 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32F429I_DISCOVERY_EEPROM_Exported_Types STM32F429I DISCOVERY EEPROM Exported Types 00063 * @{ 00064 */ 00065 /** 00066 * @} 00067 */ 00068 00069 /** @defgroup STM32F429I_DISCOVERY_EEPROM_Exported_Constants STM32F429I DISCOVERY EEPROM Exported Constants 00070 * @{ 00071 */ 00072 /* EEPROM hardware address and page size */ 00073 #define EEPROM_PAGESIZE 4 00074 #define EEPROM_MAX_SIZE 0x2000 /* 64Kbit*/ 00075 00076 /* Maximum Timeout values for flags and events waiting loops. 00077 This timeout is based on systick set to 1ms*/ 00078 /* Timeout for read based if read all the EEPROM : EEPROM_MAX_SIZE * BSP_I2C_SPEED (640ms)*/ 00079 #define EEPROM_READ_TIMEOUT ((uint32_t)(1000)) 00080 /* Timeout for write based on max write which is EEPROM_PAGESIZE bytes: EEPROM_PAGESIZE * BSP_I2C_SPEED (320us)*/ 00081 #define EEPROM_WRITE_TIMEOUT ((uint32_t)(1)) 00082 00083 /* Maximum number of trials for EEPROM_WaitEepromStandbyState() function */ 00084 #define EEPROM_MAX_TRIALS 300 00085 00086 #define EEPROM_OK 0 00087 #define EEPROM_FAIL 1 00088 #define EEPROM_TIMEOUT 2 00089 /** 00090 * @} 00091 */ 00092 00093 /** @defgroup STM32F429I_DISCOVERY_EEPROM_Exported_Macros STM32F429I DISCOVERY EEPROM Exported Macros 00094 * @{ 00095 */ 00096 /** 00097 * @} 00098 */ 00099 00100 /** @defgroup STM32F429I_DISCOVERY_EEPROM_Exported_Functions STM32F429I DISCOVERY EEPROM Exported Functions 00101 * @{ 00102 */ 00103 uint32_t BSP_EEPROM_Init(void); 00104 uint32_t BSP_EEPROM_ReadBuffer(uint8_t *pBuffer, uint16_t ReadAddr, uint16_t *NumByteToRead); 00105 uint32_t BSP_EEPROM_WritePage(uint8_t *pBuffer, uint16_t WriteAddr, uint8_t *NumByteToWrite); 00106 uint32_t BSP_EEPROM_WriteBuffer(uint8_t *pBuffer, uint16_t WriteAddr, uint16_t NumByteToWrite); 00107 uint32_t BSP_EEPROM_WaitEepromStandbyState(void); 00108 00109 /* USER Callbacks: This function is declared as __weak in EEPROM driver and 00110 should be implemented into user application. 00111 BSP_EEPROM_TIMEOUT_UserCallback() function is called whenever a timeout condition 00112 occurs during communication (waiting on an event that doesn't occur, bus 00113 errors, busy devices ...). */ 00114 void BSP_EEPROM_TIMEOUT_UserCallback(void); 00115 00116 00117 /* Link function for I2C EEPROM peripheral */ 00118 void EEPROM_IO_Init(void); 00119 HAL_StatusTypeDef EEPROM_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize); 00120 HAL_StatusTypeDef EEPROM_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize); 00121 HAL_StatusTypeDef EEPROM_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00122 00123 #ifdef __cplusplus 00124 } 00125 #endif 00126 00127 #endif /* __STM32F429I_DISCOVERY_EEPROM_H */ 00128 00129 /** 00130 * @} 00131 */ 00132 00133 /** 00134 * @} 00135 */ 00136 00137 /** 00138 * @} 00139 */ 00140 00141 /** 00142 * @} 00143 */ 00144 00145 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jan 13 2016 13:22:14 for STM32F429I-Discovery BSP User Manual by
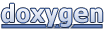