STM32F429I-Discovery BSP User Manual
|
stm32f429i_discovery_ts.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f429i_discovery_ts.c 00004 * @author MCD Application Team 00005 * @version V2.1.5 00006 * @date 27-January-2017 00007 * @brief This file provides a set of functions needed to manage Touch 00008 * screen available with STMPE811 IO Expander device mounted on 00009 * STM32F429I-Discovery Kit. 00010 ****************************************************************************** 00011 * @attention 00012 * 00013 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00014 * 00015 * Redistribution and use in source and binary forms, with or without modification, 00016 * are permitted provided that the following conditions are met: 00017 * 1. Redistributions of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00023 * may be used to endorse or promote products derived from this software 00024 * without specific prior written permission. 00025 * 00026 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00027 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00028 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00029 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00030 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00031 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00032 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00033 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00034 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00035 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00036 * 00037 ****************************************************************************** 00038 */ 00039 00040 /* Includes ------------------------------------------------------------------*/ 00041 #include "stm32f429i_discovery_ts.h" 00042 #include "stm32f429i_discovery_io.h" 00043 00044 /** @addtogroup BSP 00045 * @{ 00046 */ 00047 00048 /** @addtogroup STM32F429I_DISCOVERY 00049 * @{ 00050 */ 00051 00052 /** @defgroup STM32F429I_DISCOVERY_TS STM32F429I DISCOVERY TS 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32F429I_DISCOVERY_TS_Private_Types_Definitions STM32F429I DISCOVERY TS Private Types Definitions 00057 * @{ 00058 */ 00059 /** 00060 * @} 00061 */ 00062 00063 /** @defgroup STM32F429I_DISCOVERY_TS_Private_Defines STM32F429I DISCOVERY TS Private Defines 00064 * @{ 00065 */ 00066 /** 00067 * @} 00068 */ 00069 00070 /** @defgroup STM32F429I_DISCOVERY_TS_Private_Macros STM32F429I DISCOVERY TS Private Macros 00071 * @{ 00072 */ 00073 /** 00074 * @} 00075 */ 00076 00077 /** @defgroup STM32F429I_DISCOVERY_TS_Private_Variables STM32F429I DISCOVERY TS Private Variables 00078 * @{ 00079 */ 00080 static TS_DrvTypeDef *TsDrv; 00081 static uint16_t TsXBoundary, TsYBoundary; 00082 /** 00083 * @} 00084 */ 00085 00086 /** @defgroup STM32F429I_DISCOVERY_TS_Private_Function_Prototypes STM32F429I DISCOVERY TS Private Function Prototypes 00087 * @{ 00088 */ 00089 /** 00090 * @} 00091 */ 00092 00093 /** @defgroup STM32F429I_DISCOVERY_TS_Private_Functions STM32F429I DISCOVERY TS Private Functions 00094 * @{ 00095 */ 00096 00097 /** 00098 * @brief Initializes and configures the touch screen functionalities and 00099 * configures all necessary hardware resources (GPIOs, clocks..). 00100 * @param XSize: The maximum X size of the TS area on LCD 00101 * @param YSize: The maximum Y size of the TS area on LCD 00102 * @retval TS_OK: if all initializations are OK. Other value if error. 00103 */ 00104 uint8_t BSP_TS_Init(uint16_t XSize, uint16_t YSize) 00105 { 00106 uint8_t ret = TS_ERROR; 00107 00108 /* Initialize x and y positions boundaries */ 00109 TsXBoundary = XSize; 00110 TsYBoundary = YSize; 00111 00112 /* Read ID and verify if the IO expander is ready */ 00113 if(stmpe811_ts_drv.ReadID(TS_I2C_ADDRESS) == STMPE811_ID) 00114 { 00115 /* Initialize the TS driver structure */ 00116 TsDrv = &stmpe811_ts_drv; 00117 00118 ret = TS_OK; 00119 } 00120 00121 if(ret == TS_OK) 00122 { 00123 /* Initialize the LL TS Driver */ 00124 TsDrv->Init(TS_I2C_ADDRESS); 00125 TsDrv->Start(TS_I2C_ADDRESS); 00126 } 00127 00128 return ret; 00129 } 00130 00131 /** 00132 * @brief Configures and enables the touch screen interrupts. 00133 * @retval TS_OK: if ITconfig is OK. Other value if error. 00134 */ 00135 uint8_t BSP_TS_ITConfig(void) 00136 { 00137 /* Enable the TS ITs */ 00138 TsDrv->EnableIT(TS_I2C_ADDRESS); 00139 00140 return TS_OK; 00141 } 00142 00143 /** 00144 * @brief Gets the TS IT status. 00145 * @retval Interrupt status. 00146 */ 00147 uint8_t BSP_TS_ITGetStatus(void) 00148 { 00149 /* Return the TS IT status */ 00150 return (TsDrv->GetITStatus(TS_I2C_ADDRESS)); 00151 } 00152 00153 /** 00154 * @brief Returns status and positions of the touch screen. 00155 * @param TsState: Pointer to touch screen current state structure 00156 */ 00157 void BSP_TS_GetState(TS_StateTypeDef* TsState) 00158 { 00159 static uint32_t _x = 0, _y = 0; 00160 uint16_t xDiff, yDiff , x , y, xr, yr; 00161 00162 TsState->TouchDetected = TsDrv->DetectTouch(TS_I2C_ADDRESS); 00163 00164 if(TsState->TouchDetected) 00165 { 00166 TsDrv->GetXY(TS_I2C_ADDRESS, &x, &y); 00167 00168 /* Y value first correction */ 00169 y -= 360; 00170 00171 /* Y value second correction */ 00172 yr = y / 11; 00173 00174 /* Return y position value */ 00175 if(yr <= 0) 00176 { 00177 yr = 0; 00178 } 00179 else if (yr > TsYBoundary) 00180 { 00181 yr = TsYBoundary - 1; 00182 } 00183 else 00184 {} 00185 y = yr; 00186 00187 /* X value first correction */ 00188 if(x <= 3000) 00189 { 00190 x = 3870 - x; 00191 } 00192 else 00193 { 00194 x = 3800 - x; 00195 } 00196 00197 /* X value second correction */ 00198 xr = x / 15; 00199 00200 /* Return X position value */ 00201 if(xr <= 0) 00202 { 00203 xr = 0; 00204 } 00205 else if (xr > TsXBoundary) 00206 { 00207 xr = TsXBoundary - 1; 00208 } 00209 else 00210 {} 00211 00212 x = xr; 00213 xDiff = x > _x? (x - _x): (_x - x); 00214 yDiff = y > _y? (y - _y): (_y - y); 00215 00216 if (xDiff + yDiff > 5) 00217 { 00218 _x = x; 00219 _y = y; 00220 } 00221 00222 /* Update the X position */ 00223 TsState->X = _x; 00224 00225 /* Update the Y position */ 00226 TsState->Y = _y; 00227 } 00228 } 00229 00230 /** 00231 * @brief Clears all touch screen interrupts. 00232 */ 00233 void BSP_TS_ITClear(void) 00234 { 00235 /* Clear TS IT pending bits */ 00236 TsDrv->ClearIT(TS_I2C_ADDRESS); 00237 } 00238 00239 /** 00240 * @} 00241 */ 00242 00243 /** 00244 * @} 00245 */ 00246 00247 /** 00248 * @} 00249 */ 00250 00251 /** 00252 * @} 00253 */ 00254 00255 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Feb 17 2017 12:10:38 for STM32F429I-Discovery BSP User Manual by
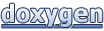