STM32F429I-Discovery BSP User Manual
|
stm32f429i_discovery_io.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f429i_discovery_io.c 00004 * @author MCD Application Team 00005 * @version V2.1.5 00006 * @date 27-January-2017 00007 * @brief This file provides a set of functions needed to manage the STMPE811 00008 * IO Expander device mounted on STM32F429I-Discovery Kit. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32f429i_discovery_io.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @addtogroup STM32F429I_DISCOVERY 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32F429I_DISCOVERY_IO STM32F429I DISCOVERY IO 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32F429I_DISCOVERY_IO_Private_Types_Definitions STM32F429I DISCOVERY IO Private Types Definitions 00055 * @{ 00056 */ 00057 /** 00058 * @} 00059 */ 00060 00061 /** @defgroup STM32F429I_DISCOVERY_IO_Private_Defines STM32F429I DISCOVERY IO Private Defines 00062 * @{ 00063 */ 00064 /** 00065 * @} 00066 */ 00067 00068 /** @defgroup STM32F429I_DISCOVERY_IO_Private_Macros STM32F429I DISCOVERY IO Private Macros 00069 * @{ 00070 */ 00071 /** 00072 * @} 00073 */ 00074 00075 00076 /** @defgroup STM32F429I_DISCOVERY_IO_Private_Variables STM32F429I DISCOVERY IO Private Variables 00077 * @{ 00078 */ 00079 static IO_DrvTypeDef *IoDrv; 00080 00081 /** 00082 * @} 00083 */ 00084 00085 00086 /** @defgroup STM32F429I_DISCOVERY_IO_Private_Function_Prototypes STM32F429I DISCOVERY IO Private Function Prototypes 00087 * @{ 00088 */ 00089 /** 00090 * @} 00091 */ 00092 00093 /** @defgroup STM32F429I_DISCOVERY_IO_Private_Functions STM32F429I DISCOVERY IO Private Functions 00094 * @{ 00095 */ 00096 00097 /** 00098 * @brief Initializes and configures the IO functionalities and configures all 00099 * necessary hardware resources (GPIOs, clocks..). 00100 * @note BSP_IO_Init() is using HAL_Delay() function to ensure that stmpe811 00101 * IO Expander is correctly reset. HAL_Delay() function provides accurate 00102 * delay (in milliseconds) based on variable incremented in SysTick ISR. 00103 * This implies that if BSP_IO_Init() is called from a peripheral ISR process, 00104 * then the SysTick interrupt must have higher priority (numerically lower) 00105 * than the peripheral interrupt. Otherwise the caller ISR process will be blocked. 00106 * @retval IO_OK if all initializations done correctly. Other value if error. 00107 */ 00108 uint8_t BSP_IO_Init(void) 00109 { 00110 uint8_t ret = IO_ERROR; 00111 00112 /* Read ID and verify the IO expander is ready */ 00113 if(stmpe811_io_drv.ReadID(IO_I2C_ADDRESS) == STMPE811_ID) 00114 { 00115 /* Initialize the IO driver structure */ 00116 IoDrv = &stmpe811_io_drv; 00117 ret = IO_OK; 00118 } 00119 00120 if(ret == IO_OK) 00121 { 00122 IoDrv->Init(IO_I2C_ADDRESS); 00123 IoDrv->Start(IO_I2C_ADDRESS, IO_PIN_ALL); 00124 } 00125 return ret; 00126 } 00127 00128 /** 00129 * @brief Gets the selected pins IT status. 00130 * @param IoPin: The selected pins to check the status. 00131 * This parameter could be any combination of the IO pins. 00132 * @retval Status of IO Pin checked. 00133 */ 00134 uint8_t BSP_IO_ITGetStatus(uint16_t IoPin) 00135 { 00136 /* Return the IO Pin IT status */ 00137 return (IoDrv->ITStatus(IO_I2C_ADDRESS, IoPin)); 00138 } 00139 00140 /** 00141 * @brief Clears all the IO IT pending bits 00142 */ 00143 void BSP_IO_ITClear(void) 00144 { 00145 /* Clear all IO IT pending bits */ 00146 IoDrv->ClearIT(IO_I2C_ADDRESS, IO_PIN_ALL); 00147 } 00148 00149 /** 00150 * @brief Configures the IO pin(s) according to IO mode structure value. 00151 * @param IoPin: IO pin(s) to be configured. 00152 * This parameter could be any combination of the following values: 00153 * @arg STMPE811_PIN_x: where x can be from 0 to 7. 00154 * @param IoMode: The IO pin mode to configure, could be one of the following values: 00155 * @arg IO_MODE_INPUT 00156 * @arg IO_MODE_OUTPUT 00157 * @arg IO_MODE_IT_RISING_EDGE 00158 * @arg IO_MODE_IT_FALLING_EDGE 00159 * @arg IO_MODE_IT_LOW_LEVEL 00160 * @arg IO_MODE_IT_HIGH_LEVEL 00161 */ 00162 void BSP_IO_ConfigPin(uint16_t IoPin, IO_ModeTypedef IoMode) 00163 { 00164 /* Configure the selected IO pin(s) mode */ 00165 IoDrv->Config(IO_I2C_ADDRESS, IoPin, IoMode); 00166 } 00167 00168 /** 00169 * @brief Sets the selected pins state. 00170 * @param IoPin: The selected pins to write. 00171 * This parameter could be any combination of the IO pins. 00172 * @param PinState: the new pins state to write 00173 */ 00174 void BSP_IO_WritePin(uint16_t IoPin, uint8_t PinState) 00175 { 00176 /* Set the Pin state */ 00177 IoDrv->WritePin(IO_I2C_ADDRESS, IoPin, PinState); 00178 } 00179 00180 /** 00181 * @brief Gets the selected pins current state. 00182 * @param IoPin: The selected pins to read. 00183 * This parameter could be any combination of the IO pins. 00184 * @retval The current pins state 00185 */ 00186 uint16_t BSP_IO_ReadPin(uint16_t IoPin) 00187 { 00188 return(IoDrv->ReadPin(IO_I2C_ADDRESS, IoPin)); 00189 } 00190 00191 /** 00192 * @brief Toggles the selected pins state. 00193 * @param IoPin: The selected pins to toggle. 00194 * This parameter could be any combination of the IO pins. 00195 */ 00196 void BSP_IO_TogglePin(uint16_t IoPin) 00197 { 00198 /* Toggle the current pin state */ 00199 if(IoDrv->ReadPin(IO_I2C_ADDRESS, IoPin) == 1 /* Set */) 00200 { 00201 IoDrv->WritePin(IO_I2C_ADDRESS, IoPin, 0 /* Reset */); 00202 } 00203 else 00204 { 00205 IoDrv->WritePin(IO_I2C_ADDRESS, IoPin, 1 /* Set */); 00206 } 00207 } 00208 00209 /** 00210 * @} 00211 */ 00212 00213 /** 00214 * @} 00215 */ 00216 00217 /** 00218 * @} 00219 */ 00220 00221 /** 00222 * @} 00223 */ 00224 00225 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Feb 17 2017 12:10:38 for STM32F429I-Discovery BSP User Manual by
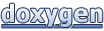