STM32F429I-Discovery BSP User Manual
|
stm32f429i_discovery.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f429i_discovery.h 00004 * @author MCD Application Team 00005 * @version V2.1.5 00006 * @date 27-January-2017 00007 * @brief This file contains definitions for STM32F429I-Discovery Kit LEDs, 00008 * push-buttons hardware resources. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32F429I_DISCOVERY_H 00041 #define __STM32F429I_DISCOVERY_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32f4xx_hal.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM32F429I_DISCOVERY 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM32F429I_DISCOVERY_LOW_LEVEL 00059 * @{ 00060 */ 00061 00062 /** @addtogroup STM32F429I_DISCOVERY_LOW_LEVEL_Exported_Types STM32F429I DISCOVERY LOW LEVEL Exported Types 00063 * @{ 00064 */ 00065 typedef enum 00066 { 00067 LED3 = 0, 00068 LED4 = 1 00069 }Led_TypeDef; 00070 00071 typedef enum 00072 { 00073 BUTTON_KEY = 0, 00074 }Button_TypeDef; 00075 00076 typedef enum 00077 { 00078 BUTTON_MODE_GPIO = 0, 00079 BUTTON_MODE_EXTI = 1 00080 }ButtonMode_TypeDef; 00081 00082 /** 00083 * @} 00084 */ 00085 00086 /** @defgroup STM32F429I_DISCOVERY_LOW_LEVEL_Exported_Constants STM32F429I DISCOVERY LOW LEVEL Exported Constants 00087 * @{ 00088 */ 00089 00090 /** 00091 * @brief Define for STM32F429I_DISCO board 00092 */ 00093 #if !defined (USE_STM32F429I_DISCO) 00094 #define USE_STM32F429I_DISCO 00095 #endif 00096 00097 /** @defgroup STM32F429I_DISCOVERY_LOW_LEVEL_LED STM32F429I DISCOVERY LOW LEVEL LED 00098 * @{ 00099 */ 00100 #define LEDn 2 00101 00102 #define LED3_PIN GPIO_PIN_13 00103 #define LED3_GPIO_PORT GPIOG 00104 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00105 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOG_CLK_DISABLE() 00106 00107 #define LED4_PIN GPIO_PIN_14 00108 #define LED4_GPIO_PORT GPIOG 00109 #define LED4_GPIO_CLK_ENABLE() __HAL_RCC_GPIOG_CLK_ENABLE() 00110 #define LED4_GPIO_CLK_DISABLE() __HAL_RCC_GPIOG_CLK_DISABLE() 00111 00112 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do{if((__INDEX__) == 0) LED3_GPIO_CLK_ENABLE(); else \ 00113 if((__INDEX__) == 1) LED4_GPIO_CLK_ENABLE(); \ 00114 }while(0) 00115 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) do{if((__INDEX__) == 0) LED3_GPIO_CLK_DISABLE(); else \ 00116 if((__INDEX__) == 1) LED4_GPIO_CLK_DISABLE(); \ 00117 }while(0) 00118 /** 00119 * @} 00120 */ 00121 00122 /** @defgroup STM32F429I_DISCOVERY_LOW_LEVEL_BUTTON STM32F429I DISCOVERY LOW LEVEL BUTTON 00123 * @{ 00124 */ 00125 #define BUTTONn 1 00126 00127 /** 00128 * @brief Wakeup push-button 00129 */ 00130 #define KEY_BUTTON_PIN GPIO_PIN_0 00131 #define KEY_BUTTON_GPIO_PORT GPIOA 00132 #define KEY_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00133 #define KEY_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00134 #define KEY_BUTTON_EXTI_IRQn EXTI0_IRQn 00135 00136 #define BUTTONx_GPIO_CLK_ENABLE(__INDEX__) do{if((__INDEX__) == 0) KEY_BUTTON_GPIO_CLK_ENABLE(); \ 00137 }while(0) 00138 #define BUTTONx_GPIO_CLK_DISABLE(__INDEX__) do{if((__INDEX__) == 0) KEY_BUTTON_GPIO_CLK_DISABLE(); \ 00139 }while(0) 00140 /** 00141 * @} 00142 */ 00143 00144 /** @defgroup STM32F429I_DISCOVERY_LOW_LEVEL_BUS STM32F429I DISCOVERY LOW LEVEL BUS 00145 * @{ 00146 */ 00147 /* Exported constanIO --------------------------------------------------------*/ 00148 #define IO_I2C_ADDRESS 0x82 00149 #define TS_I2C_ADDRESS 0x82 00150 00151 #ifdef EE_M24LR64 00152 #define EEPROM_I2C_ADDRESS_A01 0xA0 00153 #define EEPROM_I2C_ADDRESS_A02 0xA6 00154 #endif /* EE_M24LR64 */ 00155 00156 /*############################### I2Cx #######################################*/ 00157 /* User can use this section to tailor I2Cx instance used and associated 00158 resources */ 00159 #define DISCOVERY_I2Cx I2C3 00160 #define DISCOVERY_I2Cx_CLOCK_ENABLE() __HAL_RCC_I2C3_CLK_ENABLE() 00161 #define DISCOVERY_I2Cx_FORCE_RESET() __HAL_RCC_I2C3_FORCE_RESET() 00162 #define DISCOVERY_I2Cx_RELEASE_RESET() __HAL_RCC_I2C3_RELEASE_RESET() 00163 #define DISCOVERY_I2Cx_SDA_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00164 #define DISCOVERY_I2Cx_SCL_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00165 #define DISCOVERY_I2Cx_SDA_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00166 00167 /* Definition for DISCO I2Cx Pins */ 00168 #define DISCOVERY_I2Cx_SCL_PIN GPIO_PIN_8 00169 #define DISCOVERY_I2Cx_SCL_GPIO_PORT GPIOA 00170 #define DISCOVERY_I2Cx_SCL_SDA_AF GPIO_AF4_I2C3 00171 #define DISCOVERY_I2Cx_SDA_PIN GPIO_PIN_9 00172 #define DISCOVERY_I2Cx_SDA_GPIO_PORT GPIOC 00173 00174 /* Definition for IOE I2Cx's NVIC */ 00175 #define DISCOVERY_I2Cx_EV_IRQn I2C3_EV_IRQn 00176 #define DISCOVERY_I2Cx_ER_IRQn I2C3_ER_IRQn 00177 00178 /* I2C clock speed configuration (in Hz) 00179 WARNING: 00180 Make sure that this define is not already declared in other files. 00181 It can be used in parallel by other modules. */ 00182 #ifndef BSP_I2C_SPEED 00183 #define BSP_I2C_SPEED 100000 00184 #endif /* BSP_I2C_SPEED */ 00185 00186 #define I2Cx_TIMEOUT_MAX 0x3000 /*<! The value of the maximal timeout for I2C waiting loops */ 00187 00188 /*############################### SPIx #######################################*/ 00189 #define DISCOVERY_SPIx SPI5 00190 #define DISCOVERY_SPIx_CLK_ENABLE() __HAL_RCC_SPI5_CLK_ENABLE() 00191 #define DISCOVERY_SPIx_GPIO_PORT GPIOF /* GPIOF */ 00192 #define DISCOVERY_SPIx_AF GPIO_AF5_SPI5 00193 #define DISCOVERY_SPIx_GPIO_CLK_ENABLE() __HAL_RCC_GPIOF_CLK_ENABLE() 00194 #define DISCOVERY_SPIx_GPIO_CLK_DISABLE() __HAL_RCC_GPIOF_CLK_DISABLE() 00195 #define DISCOVERY_SPIx_SCK_PIN GPIO_PIN_7 /* PF.07 */ 00196 #define DISCOVERY_SPIx_MISO_PIN GPIO_PIN_8 /* PF.08 */ 00197 #define DISCOVERY_SPIx_MOSI_PIN GPIO_PIN_9 /* PF.09 */ 00198 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00199 on accurate values, they just guarantee that the application will not remain 00200 stuck if the SPI communication is corrupted. 00201 You may modify these timeout values depending on CPU frequency and application 00202 conditions (interrupts routines ...). */ 00203 #define SPIx_TIMEOUT_MAX ((uint32_t)0x1000) 00204 00205 00206 /*################################ IOE #######################################*/ 00207 /** 00208 * @brief IOE Control pin 00209 */ 00210 /* Definition for external IT for STMPE811 */ 00211 #define STMPE811_INT_PIN GPIO_PIN_15 00212 #define STMPE811_INT_GPIO_PORT GPIOA 00213 #define STMPE811_INT_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00214 #define STMPE811_INT_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00215 #define STMPE811_INT_EXTI EXTI15_10_IRQn 00216 #define STMPE811_INT_EXTIHandler EXTI15_10_IRQHandler 00217 00218 /*################################ LCD #######################################*/ 00219 /* Chip Select macro definition */ 00220 #define LCD_CS_LOW() HAL_GPIO_WritePin(LCD_NCS_GPIO_PORT, LCD_NCS_PIN, GPIO_PIN_RESET) 00221 #define LCD_CS_HIGH() HAL_GPIO_WritePin(LCD_NCS_GPIO_PORT, LCD_NCS_PIN, GPIO_PIN_SET) 00222 00223 /* Set WRX High to send data */ 00224 #define LCD_WRX_LOW() HAL_GPIO_WritePin(LCD_WRX_GPIO_PORT, LCD_WRX_PIN, GPIO_PIN_RESET) 00225 #define LCD_WRX_HIGH() HAL_GPIO_WritePin(LCD_WRX_GPIO_PORT, LCD_WRX_PIN, GPIO_PIN_SET) 00226 00227 /* Set WRX High to send data */ 00228 #define LCD_RDX_LOW() HAL_GPIO_WritePin(LCD_RDX_GPIO_PORT, LCD_RDX_PIN, GPIO_PIN_RESET) 00229 #define LCD_RDX_HIGH() HAL_GPIO_WritePin(LCD_RDX_GPIO_PORT, LCD_RDX_PIN, GPIO_PIN_SET) 00230 00231 /** 00232 * @brief LCD Control pin 00233 */ 00234 #define LCD_NCS_PIN GPIO_PIN_2 00235 #define LCD_NCS_GPIO_PORT GPIOC 00236 #define LCD_NCS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00237 #define LCD_NCS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00238 /** 00239 * @} 00240 */ 00241 /** 00242 * @brief LCD Command/data pin 00243 */ 00244 #define LCD_WRX_PIN GPIO_PIN_13 00245 #define LCD_WRX_GPIO_PORT GPIOD 00246 #define LCD_WRX_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00247 #define LCD_WRX_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00248 00249 #define LCD_RDX_PIN GPIO_PIN_12 00250 #define LCD_RDX_GPIO_PORT GPIOD 00251 #define LCD_RDX_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00252 #define LCD_RDX_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00253 00254 /*################################ GYROSCOPE #################################*/ 00255 /* Read/Write command */ 00256 #define READWRITE_CMD ((uint8_t)0x80) 00257 /* Multiple byte read/write command */ 00258 #define MULTIPLEBYTE_CMD ((uint8_t)0x40) 00259 /* Dummy Byte Send by the SPI Master device in order to generate the Clock to the Slave device */ 00260 #define DUMMY_BYTE ((uint8_t)0x00) 00261 00262 /* Chip Select macro definition */ 00263 #define GYRO_CS_LOW() HAL_GPIO_WritePin(GYRO_CS_GPIO_PORT, GYRO_CS_PIN, GPIO_PIN_RESET) 00264 #define GYRO_CS_HIGH() HAL_GPIO_WritePin(GYRO_CS_GPIO_PORT, GYRO_CS_PIN, GPIO_PIN_SET) 00265 00266 /** 00267 * @brief GYROSCOPE SPI Interface pins 00268 */ 00269 #define GYRO_CS_PIN GPIO_PIN_1 /* PC.01 */ 00270 #define GYRO_CS_GPIO_PORT GPIOC /* GPIOC */ 00271 #define GYRO_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00272 #define GYRO_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00273 00274 #define GYRO_INT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00275 #define GYRO_INT_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00276 #define GYRO_INT_GPIO_PORT GPIOA /* GPIOA */ 00277 #define GYRO_INT1_PIN GPIO_PIN_1 /* PA.01 */ 00278 #define GYRO_INT1_EXTI_IRQn EXTI1_IRQn 00279 #define GYRO_INT2_PIN GPIO_PIN_2 /* PA.02 */ 00280 #define GYRO_INT2_EXTI_IRQn EXTI2_IRQn 00281 /** 00282 * @} 00283 */ 00284 00285 #ifdef EE_M24LR64 00286 /** @defgroup STM32F429I_DISCOVERY_LOW_LEVEL_I2C_EEPROM STM32F429I DISCOVERY LOW LEVEL I2C EEPROM 00287 * @{ 00288 */ 00289 /** 00290 * @brief I2C EEPROM Interface pins 00291 */ 00292 #define EEPROM_I2C_DMA DMA1 00293 #define EEPROM_I2C_DMA_CHANNEL DMA_CHANNEL_3 00294 #define EEPROM_I2C_DMA_STREAM_TX DMA1_Stream4 00295 #define EEPROM_I2C_DMA_STREAM_RX DMA1_Stream2 00296 #define EEPROM_I2C_DMA_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00297 00298 #define EEPROM_I2C_DMA_TX_IRQn DMA1_Stream4_IRQn 00299 #define EEPROM_I2C_DMA_RX_IRQn DMA1_Stream2_IRQn 00300 #define EEPROM_I2C_DMA_TX_IRQHandler DMA1_Stream4_IRQHandler 00301 #define EEPROM_I2C_DMA_RX_IRQHandler DMA1_Stream2_IRQHandler 00302 #define EEPROM_I2C_DMA_PREPRIO 0x0F 00303 /** 00304 * @} 00305 */ 00306 00307 #endif /* EE_M24LR64 */ 00308 00309 /** @defgroup STM32F429I_DISCOVERY_LOW_LEVEL_Exported_Macros STM32F429I DISCOVERY LOW LEVEL Exported Macros 00310 * @{ 00311 */ 00312 /** 00313 * @} 00314 */ 00315 00316 /** @defgroup STM32F429I_DISCOVERY_LOW_LEVEL_Exported_Functions STM32F429I DISCOVERY LOW LEVEL Exported Functions 00317 * @{ 00318 */ 00319 uint32_t BSP_GetVersion(void); 00320 void BSP_LED_Init(Led_TypeDef Led); 00321 void BSP_LED_On(Led_TypeDef Led); 00322 void BSP_LED_Off(Led_TypeDef Led); 00323 void BSP_LED_Toggle(Led_TypeDef Led); 00324 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode); 00325 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00326 00327 /** 00328 * @} 00329 */ 00330 00331 /** 00332 * @} 00333 */ 00334 00335 /** 00336 * @} 00337 */ 00338 00339 /** 00340 * @} 00341 */ 00342 00343 #ifdef __cplusplus 00344 } 00345 #endif 00346 00347 #endif /* __STM32F429I_DISCOVERY_H */ 00348 00349 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Feb 17 2017 12:10:38 for STM32F429I-Discovery BSP User Manual by
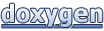