_BSP_User_Manual
|
stm3210e_eval_lcd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_lcd.h 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file contains the common defines and functions prototypes for 00008 * the stm3210e_eval_lcd.c driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /** @addtogroup BSP 00040 * @{ 00041 */ 00042 00043 /** @addtogroup STM3210E_EVAL 00044 * @{ 00045 */ 00046 00047 /* Define to prevent recursive inclusion -------------------------------------*/ 00048 #ifndef __STM3210E_EVAL_LCD_H 00049 #define __STM3210E_EVAL_LCD_H 00050 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 /* Includes ------------------------------------------------------------------*/ 00056 #include "stm3210e_eval.h" 00057 #include "../Components/hx8347d/hx8347d.h" 00058 #include "../Components/spfd5408/spfd5408.h" 00059 #include "../Components/ili9320/ili9320.h" 00060 #include "../../../Utilities/Fonts/fonts.h" 00061 00062 /** @addtogroup STM3210E_EVAL_LCD 00063 * @{ 00064 */ 00065 00066 00067 /** @defgroup STM3210E_EVAL_LCD_Exported_Types Exported Types 00068 * @{ 00069 */ 00070 typedef struct 00071 { 00072 uint32_t TextColor; 00073 uint32_t BackColor; 00074 sFONT *pFont; 00075 00076 }LCD_DrawPropTypeDef; 00077 /** 00078 * @} 00079 */ 00080 00081 /** @defgroup STM3210E_EVAL_LCD_Exported_Constants Exported Constants 00082 * @{ 00083 */ 00084 /** 00085 * @brief LCD status structure definition 00086 */ 00087 #define LCD_OK 0x00 00088 #define LCD_ERROR 0x01 00089 #define LCD_TIMEOUT 0x02 00090 00091 typedef struct 00092 { 00093 int16_t X; 00094 int16_t Y; 00095 00096 }Point, * pPoint; 00097 00098 /** 00099 * @brief Line mode structures definition 00100 */ 00101 typedef enum 00102 { 00103 CENTER_MODE = 0x01, /*!< Center mode */ 00104 RIGHT_MODE = 0x02, /*!< Right mode */ 00105 LEFT_MODE = 0x03 /*!< Left mode */ 00106 00107 }Line_ModeTypdef; 00108 00109 /** 00110 * @brief LCD color 00111 */ 00112 #define LCD_COLOR_BLUE (uint16_t)0x001F 00113 #define LCD_COLOR_GREEN (uint16_t)0x07E0 00114 #define LCD_COLOR_RED (uint16_t)0xF800 00115 #define LCD_COLOR_CYAN (uint16_t)0x07FF 00116 #define LCD_COLOR_MAGENTA (uint16_t)0xF81F 00117 #define LCD_COLOR_YELLOW (uint16_t)0xFFE0 00118 #define LCD_COLOR_LIGHTBLUE (uint16_t)0x841F 00119 #define LCD_COLOR_LIGHTGREEN (uint16_t)0x87F0 00120 #define LCD_COLOR_LIGHTRED (uint16_t)0xFC10 00121 #define LCD_COLOR_LIGHTCYAN (uint16_t)0x87FF 00122 #define LCD_COLOR_LIGHTMAGENTA (uint16_t)0xFC1F 00123 #define LCD_COLOR_LIGHTYELLOW (uint16_t)0xFFF0 00124 #define LCD_COLOR_DARKBLUE (uint16_t)0x0010 00125 #define LCD_COLOR_DARKGREEN (uint16_t)0x0400 00126 #define LCD_COLOR_DARKRED (uint16_t)0x8000 00127 #define LCD_COLOR_DARKCYAN (uint16_t)0x0410 00128 #define LCD_COLOR_DARKMAGENTA (uint16_t)0x8010 00129 #define LCD_COLOR_DARKYELLOW (uint16_t)0x8400 00130 #define LCD_COLOR_WHITE (uint16_t)0xFFFF 00131 #define LCD_COLOR_LIGHTGRAY (uint16_t)0xD69A 00132 #define LCD_COLOR_GRAY (uint16_t)0x8410 00133 #define LCD_COLOR_DARKGRAY (uint16_t)0x4208 00134 #define LCD_COLOR_BLACK (uint16_t)0x0000 00135 #define LCD_COLOR_BROWN (uint16_t)0xA145 00136 #define LCD_COLOR_ORANGE (uint16_t)0xFD20 00137 00138 /** 00139 * @brief LCD default font 00140 */ 00141 #define LCD_DEFAULT_FONT Font24 00142 00143 /** 00144 * @} 00145 */ 00146 00147 /** @addtogroup STM3210E_EVAL_LCD_Exported_Functions 00148 * @{ 00149 */ 00150 00151 uint8_t BSP_LCD_Init(void); 00152 uint32_t BSP_LCD_GetXSize(void); 00153 uint32_t BSP_LCD_GetYSize(void); 00154 00155 uint16_t BSP_LCD_GetTextColor(void); 00156 uint16_t BSP_LCD_GetBackColor(void); 00157 void BSP_LCD_SetTextColor(__IO uint16_t Color); 00158 void BSP_LCD_SetBackColor(__IO uint16_t Color); 00159 void BSP_LCD_SetFont(sFONT *fonts); 00160 sFONT *BSP_LCD_GetFont(void); 00161 00162 void BSP_LCD_Clear(uint16_t Color); 00163 void BSP_LCD_ClearStringLine(uint16_t Line); 00164 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *ptr); 00165 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *Text, Line_ModeTypdef Mode); 00166 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii); 00167 00168 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos); 00169 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGB_Code); 00170 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00171 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length); 00172 void BSP_LCD_DrawLine(uint16_t x1, uint16_t y1, uint16_t x2, uint16_t y2); 00173 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00174 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00175 void BSP_LCD_DrawPolygon(pPoint Points, uint16_t PointCount); 00176 void BSP_LCD_FillTriangle(uint16_t X1, uint16_t X2, uint16_t X3, uint16_t Y1, uint16_t Y2, uint16_t Y3); 00177 void BSP_LCD_FillPolygon(pPoint Points, uint16_t PointCount); 00178 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00179 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pbmp); 00180 void BSP_LCD_DrawRGBImage(uint16_t Xpos, uint16_t Ypos, uint16_t Xsize, uint16_t Ysize, uint8_t *pbmp); 00181 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00182 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius); 00183 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius); 00184 00185 void BSP_LCD_DisplayOff(void); 00186 void BSP_LCD_DisplayOn(void); 00187 00188 /** 00189 * @} 00190 */ 00191 00192 /** 00193 * @} 00194 */ 00195 00196 #ifdef __cplusplus 00197 } 00198 #endif 00199 00200 #endif /* __STM3210E_EVAL_LCD_H */ 00201 00202 /** 00203 * @} 00204 */ 00205 00206 /** 00207 * @} 00208 */ 00209 00210 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 11 2014 16:16:37 for _BSP_User_Manual by
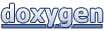