_BSP_User_Manual
|
stm3210e_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210e_eval_lcd.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file includes the driver for Liquid Crystal Display (LCD) module 00008 * mounted on STM3210E-EVAL evaluation board. 00009 @verbatim 00010 ============================================================================== 00011 ##### How to use this driver ##### 00012 ============================================================================== 00013 [..] 00014 (#) This driver is used to drive indirectly an LCD TFT. 00015 00016 (#) This driver supports the AM-240320L8TNQW00H (ILI9320), AM-240320LDTNQW-05H (ILI9325) 00017 AM-240320LDTNQW-02H (SPFD5408B) and AM240320LGTNQW-01H (HX8347D) LCD 00018 mounted on MB895 daughter board 00019 00020 (#) The ILI9320, ILI9325, SPFD5408B and HX8347D components driver MUST be included with this driver. 00021 00022 (#) Initialization steps: 00023 (++) Initialize the LCD using the BSP_LCD_Init() function. 00024 00025 (#) Display on LCD 00026 (++) Clear the hole LCD using yhe BSP_LCD_Clear() function or only one specified 00027 string line using the BSP_LCD_ClearStringLine() function. 00028 (++) Display a character on the specified line and column using the BSP_LCD_DisplayChar() 00029 function or a complete string line using the BSP_LCD_DisplayStringAtLine() function. 00030 (++) Display a string line on the specified position (x,y in pixel) and align mode 00031 using the BSP_LCD_DisplayStringAtLine() function. 00032 (++) Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap, raw picture) 00033 on LCD using a set of functions. 00034 @endverbatim 00035 ****************************************************************************** 00036 * @attention 00037 * 00038 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00039 * 00040 * Redistribution and use in source and binary forms, with or without modification, 00041 * are permitted provided that the following conditions are met: 00042 * 1. Redistributions of source code must retain the above copyright notice, 00043 * this list of conditions and the following disclaimer. 00044 * 2. Redistributions in binary form must reproduce the above copyright notice, 00045 * this list of conditions and the following disclaimer in the documentation 00046 * and/or other materials provided with the distribution. 00047 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00048 * may be used to endorse or promote products derived from this software 00049 * without specific prior written permission. 00050 * 00051 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00052 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00053 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00054 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00055 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00056 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00057 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00058 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00059 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00060 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00061 * 00062 ****************************************************************************** 00063 */ 00064 00065 /* Includes ------------------------------------------------------------------*/ 00066 #include "stm3210e_eval_lcd.h" 00067 #include "../../../Utilities/Fonts/fonts.h" 00068 #include "../../../Utilities/Fonts/font24.c" 00069 #include "../../../Utilities/Fonts/font20.c" 00070 #include "../../../Utilities/Fonts/font16.c" 00071 #include "../../../Utilities/Fonts/font12.c" 00072 #include "../../../Utilities/Fonts/font8.c" 00073 00074 /** @addtogroup BSP 00075 * @{ 00076 */ 00077 00078 /** @addtogroup STM3210E_EVAL 00079 * @{ 00080 */ 00081 00082 /** @defgroup STM3210E_EVAL_LCD STM3210E-EVAL LCD 00083 * @{ 00084 */ 00085 00086 00087 /** @defgroup STM3210E_EVAL_LCD_Private_Defines Private Defines 00088 * @{ 00089 */ 00090 #define POLY_X(Z) ((int32_t)((pPoints + (Z))->X)) 00091 #define POLY_Y(Z) ((int32_t)((pPoints + (Z))->Y)) 00092 00093 #define MAX_HEIGHT_FONT 17 00094 #define MAX_WIDTH_FONT 24 00095 #define OFFSET_BITMAP 54 00096 /** 00097 * @} 00098 */ 00099 00100 /** @defgroup STM3210E_EVAL_LCD_Private_Macros Private Macros 00101 * @{ 00102 */ 00103 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00104 00105 /** 00106 * @} 00107 */ 00108 00109 /** @defgroup STM3210E_EVAL_LCD_Private_Variables Private Variables 00110 * @{ 00111 */ 00112 LCD_DrawPropTypeDef DrawProp; 00113 00114 static LCD_DrvTypeDef *lcd_drv; 00115 00116 /* Max size of bitmap will based on a font24 (17x24) */ 00117 static uint8_t bitmap[MAX_HEIGHT_FONT*MAX_WIDTH_FONT*2+OFFSET_BITMAP] = {0}; 00118 00119 static uint32_t LCD_SwapXY = 0; 00120 /** 00121 * @} 00122 */ 00123 00124 /** @defgroup STM3210E_EVAL_LCD_Private_Functions Private Functions 00125 * @{ 00126 */ 00127 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode); 00128 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar); 00129 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00130 /** 00131 * @} 00132 */ 00133 00134 00135 /** @defgroup STM3210E_EVAL_LCD_Exported_Functions Exported Functions 00136 * @{ 00137 */ 00138 00139 /** 00140 * @brief Initializes the LCD. 00141 * @retval LCD state 00142 */ 00143 uint8_t BSP_LCD_Init(void) 00144 { 00145 uint8_t ret = LCD_ERROR; 00146 00147 /* Default value for draw propriety */ 00148 DrawProp.BackColor = 0xFFFF; 00149 DrawProp.pFont = &Font24; 00150 DrawProp.TextColor = 0x0000; 00151 00152 if(hx8347d_drv.ReadID() == HX8347D_ID) 00153 { 00154 lcd_drv = &hx8347d_drv; 00155 ret = LCD_OK; 00156 } 00157 else if(spfd5408_drv.ReadID() == SPFD5408_ID) 00158 { 00159 lcd_drv = &spfd5408_drv; 00160 ret = LCD_OK; 00161 } 00162 else if(ili9320_drv.ReadID() == ILI9320_ID) 00163 { 00164 lcd_drv = &ili9320_drv; 00165 LCD_SwapXY = 1; 00166 ret = LCD_OK; 00167 } 00168 00169 if(ret != LCD_ERROR) 00170 { 00171 /* LCD Init */ 00172 lcd_drv->Init(); 00173 00174 /* Initialize the font */ 00175 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00176 } 00177 00178 #if defined(AFIO_MAPR2_FSMC_NADV_REMAP) 00179 /* Disconnect FSMC pin NADV. */ 00180 /* FSMC and I2C share the same I/O pin: NADV/SDA (PB7). Since this pin */ 00181 /* is not used by FSMC on STM32F10E_EVAL board, disconnect it internaly */ 00182 /* from FSMC to let it dedicated to I2C1 without sharing constraints. */ 00183 __HAL_AFIO_FSMCNADV_DISCONNECTED(); 00184 #else 00185 #error "Warning: FSMC pin NADV cannot be disconnected by internal remap. \ 00186 Therefore, I2C1 will have a shared pin in conflict with FSMC. \ 00187 Use macro __HAL_RCC_FSMC_CLK_ENABLE() and \ 00188 __HAL_RCC_FSMC_CLK_DISABLE() to manage the FSMC and I2C1 usage \ 00189 alternatively." 00190 #endif 00191 00192 return ret; 00193 } 00194 00195 /** 00196 * @brief Gets the LCD X size. 00197 * @retval Used LCD X size 00198 */ 00199 uint32_t BSP_LCD_GetXSize(void) 00200 { 00201 return(lcd_drv->GetLcdPixelWidth()); 00202 } 00203 00204 /** 00205 * @brief Gets the LCD Y size. 00206 * @retval Used LCD Y size 00207 */ 00208 uint32_t BSP_LCD_GetYSize(void) 00209 { 00210 return(lcd_drv->GetLcdPixelHeight()); 00211 } 00212 00213 /** 00214 * @brief Gets the LCD text color. 00215 * @retval Used text color. 00216 */ 00217 uint16_t BSP_LCD_GetTextColor(void) 00218 { 00219 return DrawProp.TextColor; 00220 } 00221 00222 /** 00223 * @brief Gets the LCD background color. 00224 * @retval Used background color 00225 */ 00226 uint16_t BSP_LCD_GetBackColor(void) 00227 { 00228 return DrawProp.BackColor; 00229 } 00230 00231 /** 00232 * @brief Sets the LCD text color. 00233 * @param Color: Text color code RGB(5-6-5) 00234 * @retval None 00235 */ 00236 void BSP_LCD_SetTextColor(uint16_t Color) 00237 { 00238 DrawProp.TextColor = Color; 00239 } 00240 00241 /** 00242 * @brief Sets the LCD background color. 00243 * @param Color: Background color code RGB(5-6-5) 00244 * @retval None 00245 */ 00246 void BSP_LCD_SetBackColor(uint16_t Color) 00247 { 00248 DrawProp.BackColor = Color; 00249 } 00250 00251 /** 00252 * @brief Sets the LCD text font. 00253 * @param pFonts: Font to be used 00254 * @retval None 00255 */ 00256 void BSP_LCD_SetFont(sFONT *pFonts) 00257 { 00258 DrawProp.pFont = pFonts; 00259 } 00260 00261 /** 00262 * @brief Gets the LCD text font. 00263 * @retval Used font 00264 */ 00265 sFONT *BSP_LCD_GetFont(void) 00266 { 00267 return DrawProp.pFont; 00268 } 00269 00270 /** 00271 * @brief Clears the hole LCD. 00272 * @param Color: Color of the background 00273 * @retval None 00274 */ 00275 void BSP_LCD_Clear(uint16_t Color) 00276 { 00277 uint32_t counter = 0; 00278 00279 uint32_t color_backup = DrawProp.TextColor; 00280 DrawProp.TextColor = Color; 00281 00282 for(counter = 0; counter < BSP_LCD_GetYSize(); counter++) 00283 { 00284 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00285 } 00286 00287 DrawProp.TextColor = color_backup; 00288 BSP_LCD_SetTextColor(DrawProp.TextColor); 00289 } 00290 00291 /** 00292 * @brief Clears the selected line. 00293 * @param Line: Line to be cleared 00294 * This parameter can be one of the following values: 00295 * @arg 0..9: if the Current fonts is Font16x24 00296 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00297 * @arg 0..29: if the Current fonts is Font8x8 00298 * @retval None 00299 */ 00300 void BSP_LCD_ClearStringLine(uint16_t Line) 00301 { 00302 uint32_t colorbackup = DrawProp.TextColor; 00303 DrawProp.TextColor = DrawProp.BackColor;; 00304 00305 /* Draw a rectangle with background color */ 00306 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00307 00308 DrawProp.TextColor = colorbackup; 00309 BSP_LCD_SetTextColor(DrawProp.TextColor); 00310 } 00311 00312 /** 00313 * @brief Displays one character. 00314 * @param Xpos: Start column address 00315 * @param Ypos: Line where to display the character shape. 00316 * @param Ascii: Character ascii code 00317 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00318 * @retval None 00319 */ 00320 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00321 { 00322 LCD_DrawChar(Ypos, Xpos, &DrawProp.pFont->table[(Ascii-' ') *\ 00323 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00324 } 00325 00326 /** 00327 * @brief Displays characters on the LCD. 00328 * @param Xpos: X position (in pixel) 00329 * @param Ypos: Y position (in pixel) 00330 * @param pText: Pointer to string to display on LCD 00331 * @param Mode: Display mode 00332 * This parameter can be one of the following values: 00333 * @arg CENTER_MODE 00334 * @arg RIGHT_MODE 00335 * @arg LEFT_MODE 00336 * @retval None 00337 */ 00338 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *pText, Line_ModeTypdef Mode) 00339 { 00340 uint16_t refcolumn = 1, counter = 0; 00341 uint32_t size = 0, ysize = 0; 00342 uint8_t *ptr = pText; 00343 00344 /* Get the text size */ 00345 while (*ptr++) size ++ ; 00346 00347 /* Characters number per line */ 00348 ysize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00349 00350 switch (Mode) 00351 { 00352 case CENTER_MODE: 00353 { 00354 refcolumn = Xpos + ((ysize - size)* DrawProp.pFont->Width) / 2; 00355 break; 00356 } 00357 case LEFT_MODE: 00358 { 00359 refcolumn = Xpos; 00360 break; 00361 } 00362 case RIGHT_MODE: 00363 { 00364 refcolumn = -Xpos + ((ysize - size)*DrawProp.pFont->Width); 00365 break; 00366 } 00367 default: 00368 { 00369 refcolumn = Xpos; 00370 break; 00371 } 00372 } 00373 00374 /* Send the string character by character on lCD */ 00375 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (counter*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00376 { 00377 /* Display one character on LCD */ 00378 BSP_LCD_DisplayChar(refcolumn, Ypos, *pText); 00379 /* Decrement the column position by 16 */ 00380 refcolumn += DrawProp.pFont->Width; 00381 /* Point on the next character */ 00382 pText++; 00383 counter++; 00384 } 00385 } 00386 00387 /** 00388 * @brief Displays a character on the LCD. 00389 * @param Line: Line where to display the character shape 00390 * This parameter can be one of the following values: 00391 * @arg 0..9: if the Current fonts is Font16x24 00392 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00393 * @arg 0..29: if the Current fonts is Font8x8 00394 * @param pText: Pointer to string to display on LCD 00395 * @retval None 00396 */ 00397 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *pText) 00398 { 00399 BSP_LCD_DisplayStringAt(0, LINE(Line),pText, LEFT_MODE); 00400 } 00401 00402 /** 00403 * @brief Reads an LCD pixel. 00404 * @param Xpos: X position 00405 * @param Ypos: Y position 00406 * @retval RGB pixel color 00407 */ 00408 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00409 { 00410 uint16_t ret = 0; 00411 00412 if(lcd_drv->ReadPixel != NULL) 00413 { 00414 ret = lcd_drv->ReadPixel(Xpos, Ypos); 00415 } 00416 00417 return ret; 00418 } 00419 00420 /** 00421 * @brief Draws an horizontal line. 00422 * @param Xpos: X position 00423 * @param Ypos: Y position 00424 * @param Length: Line length 00425 * @retval None 00426 */ 00427 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00428 { 00429 uint32_t index = 0; 00430 00431 if(lcd_drv->DrawHLine != NULL) 00432 { 00433 if (LCD_SwapXY) 00434 { 00435 uint16_t tmp = Ypos; 00436 Ypos = Xpos; 00437 Xpos = tmp; 00438 } 00439 00440 lcd_drv->DrawHLine(DrawProp.TextColor, Ypos, Xpos, Length); 00441 } 00442 else 00443 { 00444 for(index = 0; index < Length; index++) 00445 { 00446 LCD_DrawPixel((Ypos + index), Xpos, DrawProp.TextColor); 00447 } 00448 } 00449 } 00450 00451 /** 00452 * @brief Draws a vertical line. 00453 * @param Xpos: X position 00454 * @param Ypos: Y position 00455 * @param Length: Line length 00456 * @retval None 00457 */ 00458 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00459 { 00460 uint32_t index = 0; 00461 00462 if(lcd_drv->DrawVLine != NULL) 00463 { 00464 if (LCD_SwapXY) 00465 { 00466 uint16_t tmp = Ypos; 00467 Ypos = Xpos; 00468 Xpos = tmp; 00469 } 00470 00471 LCD_SetDisplayWindow(Ypos, Xpos, 1, Length); 00472 lcd_drv->DrawVLine(DrawProp.TextColor, Ypos, Xpos, Length); 00473 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00474 } 00475 else 00476 { 00477 for(index = 0; index < Length; index++) 00478 { 00479 LCD_DrawPixel(Ypos, Xpos + index, DrawProp.TextColor); 00480 } 00481 } 00482 } 00483 00484 /** 00485 * @brief Draws an uni-line (between two points). 00486 * @param X1: Point 1 X position 00487 * @param Y1: Point 1 Y position 00488 * @param X2: Point 2 X position 00489 * @param Y2: Point 2 Y position 00490 * @retval None 00491 */ 00492 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2) 00493 { 00494 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00495 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00496 curpixel = 0; 00497 00498 deltax = ABS(Y2 - Y1); /* The difference between the x's */ 00499 deltay = ABS(X2 - X1); /* The difference between the y's */ 00500 x = Y1; /* Start x off at the first pixel */ 00501 y = X1; /* Start y off at the first pixel */ 00502 00503 if (Y2 >= Y1) /* The x-values are increasing */ 00504 { 00505 xinc1 = 1; 00506 xinc2 = 1; 00507 } 00508 else /* The x-values are decreasing */ 00509 { 00510 xinc1 = -1; 00511 xinc2 = -1; 00512 } 00513 00514 if (X2 >= X1) /* The y-values are increasing */ 00515 { 00516 yinc1 = 1; 00517 yinc2 = 1; 00518 } 00519 else /* The y-values are decreasing */ 00520 { 00521 yinc1 = -1; 00522 yinc2 = -1; 00523 } 00524 00525 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00526 { 00527 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00528 yinc2 = 0; /* Don't change the y for every iteration */ 00529 den = deltax; 00530 num = deltax / 2; 00531 numadd = deltay; 00532 numpixels = deltax; /* There are more x-values than y-values */ 00533 } 00534 else /* There is at least one y-value for every x-value */ 00535 { 00536 xinc2 = 0; /* Don't change the x for every iteration */ 00537 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00538 den = deltay; 00539 num = deltay / 2; 00540 numadd = deltax; 00541 numpixels = deltay; /* There are more y-values than x-values */ 00542 } 00543 00544 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00545 { 00546 LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00547 num += numadd; /* Increase the numerator by the top of the fraction */ 00548 if (num >= den) /* Check if numerator >= denominator */ 00549 { 00550 num -= den; /* Calculate the new numerator value */ 00551 x += xinc1; /* Change the x as appropriate */ 00552 y += yinc1; /* Change the y as appropriate */ 00553 } 00554 x += xinc2; /* Change the x as appropriate */ 00555 y += yinc2; /* Change the y as appropriate */ 00556 } 00557 } 00558 00559 /** 00560 * @brief Draws a rectangle. 00561 * @param Xpos: X position 00562 * @param Ypos: Y position 00563 * @param Width: Rectangle width 00564 * @param Height: Rectangle height 00565 * @retval None 00566 */ 00567 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00568 { 00569 /* Draw horizontal lines */ 00570 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00571 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00572 00573 /* Draw vertical lines */ 00574 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00575 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00576 } 00577 00578 /** 00579 * @brief Draws a circle. 00580 * @param Xpos: X position 00581 * @param Ypos: Y position 00582 * @param Radius: Circle radius 00583 * @retval None 00584 */ 00585 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00586 { 00587 int32_t decision; /* Decision Variable */ 00588 uint32_t curx; /* Current X Value */ 00589 uint32_t cury; /* Current Y Value */ 00590 00591 decision = 3 - (Radius << 1); 00592 curx = 0; 00593 cury = Radius; 00594 00595 while (curx <= cury) 00596 { 00597 LCD_DrawPixel((Ypos + curx), (Xpos - cury), DrawProp.TextColor); 00598 00599 LCD_DrawPixel((Ypos - curx), (Xpos - cury), DrawProp.TextColor); 00600 00601 LCD_DrawPixel((Ypos + cury), (Xpos - curx), DrawProp.TextColor); 00602 00603 LCD_DrawPixel((Ypos - cury), (Xpos - curx), DrawProp.TextColor); 00604 00605 LCD_DrawPixel((Ypos + curx), (Xpos + cury), DrawProp.TextColor); 00606 00607 LCD_DrawPixel((Ypos - curx), (Xpos + cury), DrawProp.TextColor); 00608 00609 LCD_DrawPixel((Ypos + cury), (Xpos + curx), DrawProp.TextColor); 00610 00611 LCD_DrawPixel((Ypos - cury), (Xpos + curx), DrawProp.TextColor); 00612 00613 /* Initialize the font */ 00614 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00615 00616 if (decision < 0) 00617 { 00618 decision += (curx << 2) + 6; 00619 } 00620 else 00621 { 00622 decision += ((curx - cury) << 2) + 10; 00623 cury--; 00624 } 00625 curx++; 00626 } 00627 } 00628 00629 /** 00630 * @brief Draws an poly-line (between many points). 00631 * @param pPoints: Pointer to the points array 00632 * @param PointCount: Number of points 00633 * @retval None 00634 */ 00635 void BSP_LCD_DrawPolygon(pPoint pPoints, uint16_t PointCount) 00636 { 00637 int16_t x = 0, y = 0; 00638 00639 if(PointCount < 2) 00640 { 00641 return; 00642 } 00643 00644 BSP_LCD_DrawLine(pPoints->X, pPoints->Y, (pPoints+PointCount-1)->X, (pPoints+PointCount-1)->Y); 00645 00646 while(--PointCount) 00647 { 00648 x = pPoints->X; 00649 y = pPoints->Y; 00650 pPoints++; 00651 BSP_LCD_DrawLine(x, y, pPoints->X, pPoints->Y); 00652 } 00653 00654 } 00655 00656 /** 00657 * @brief Draws an ellipse on LCD. 00658 * @param Xpos: X position 00659 * @param Ypos: Y position 00660 * @param XRadius: Ellipse X radius 00661 * @param YRadius: Ellipse Y radius 00662 * @retval None 00663 */ 00664 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00665 { 00666 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00667 float k = 0, rad1 = 0, rad2 = 0; 00668 00669 rad1 = YRadius; 00670 rad2 = XRadius; 00671 00672 k = (float)(rad2/rad1); 00673 00674 do { 00675 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00676 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00677 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00678 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00679 00680 e2 = err; 00681 if (e2 <= x) { 00682 err += ++x*2+1; 00683 if (-y == x && e2 <= y) e2 = 0; 00684 } 00685 if (e2 > y) err += ++y*2+1; 00686 } 00687 while (y <= 0); 00688 } 00689 00690 /** 00691 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 00692 * @param Xpos: Bmp X position in the LCD 00693 * @param Ypos: Bmp Y position in the LCD 00694 * @param pBmp: Pointer to Bmp picture address in the internal Flash 00695 * @retval None 00696 */ 00697 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pBmp) 00698 { 00699 uint32_t height = 0, width = 0; 00700 00701 if (LCD_SwapXY) 00702 { 00703 uint16_t tmp = Ypos; 00704 Ypos = Xpos; 00705 Xpos = tmp; 00706 } 00707 00708 /* Read bitmap width */ 00709 width = *(uint16_t *) (pBmp + 18); 00710 width |= (*(uint16_t *) (pBmp + 20)) << 16; 00711 00712 /* Read bitmap height */ 00713 height = *(uint16_t *) (pBmp + 22); 00714 height |= (*(uint16_t *) (pBmp + 24)) << 16; 00715 00716 /* Remap Ypos, hx8347d works with inverted X in case of bitmap */ 00717 /* X = 0, cursor is on Bottom corner */ 00718 if(lcd_drv == &hx8347d_drv) 00719 { 00720 Ypos = BSP_LCD_GetYSize() - Ypos - height; 00721 } 00722 00723 LCD_SetDisplayWindow(Ypos, Xpos, width, height); 00724 00725 if(lcd_drv->DrawBitmap != NULL) 00726 { 00727 lcd_drv->DrawBitmap(Ypos, Xpos, pBmp); 00728 } 00729 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00730 } 00731 00732 /** 00733 * @brief Draws a full rectangle. 00734 * @param Xpos: X position 00735 * @param Ypos: Y position 00736 * @param Width: Rectangle width 00737 * @param Height: Rectangle height 00738 * @retval None 00739 */ 00740 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00741 { 00742 BSP_LCD_SetTextColor(DrawProp.TextColor); 00743 do 00744 { 00745 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00746 } 00747 while(Height--); 00748 } 00749 00750 /** 00751 * @brief Draws a full circle. 00752 * @param Xpos: X position 00753 * @param Ypos: Y position 00754 * @param Radius: Circle radius 00755 * @retval None 00756 */ 00757 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00758 { 00759 int32_t decision; /* Decision Variable */ 00760 uint32_t curx; /* Current X Value */ 00761 uint32_t cury; /* Current Y Value */ 00762 00763 decision = 3 - (Radius << 1); 00764 00765 curx = 0; 00766 cury = Radius; 00767 00768 BSP_LCD_SetTextColor(DrawProp.TextColor); 00769 00770 while (curx <= cury) 00771 { 00772 if(cury > 0) 00773 { 00774 BSP_LCD_DrawVLine(Xpos + curx, Ypos - cury, 2*cury); 00775 BSP_LCD_DrawVLine(Xpos - curx, Ypos - cury, 2*cury); 00776 } 00777 00778 if(curx > 0) 00779 { 00780 BSP_LCD_DrawVLine(Xpos - cury, Ypos - curx, 2*curx); 00781 BSP_LCD_DrawVLine(Xpos + cury, Ypos - curx, 2*curx); 00782 } 00783 if (decision < 0) 00784 { 00785 decision += (curx << 2) + 6; 00786 } 00787 else 00788 { 00789 decision += ((curx - cury) << 2) + 10; 00790 cury--; 00791 } 00792 curx++; 00793 } 00794 00795 BSP_LCD_SetTextColor(DrawProp.TextColor); 00796 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00797 } 00798 00799 /** 00800 * @brief Fill triangle. 00801 * @param X1: specifies the point 1 x position. 00802 * @param Y1: specifies the point 1 y position. 00803 * @param X2: specifies the point 2 x position. 00804 * @param Y2: specifies the point 2 y position. 00805 * @param X3: specifies the point 3 x position. 00806 * @param Y3: specifies the point 3 y position. 00807 * @retval None 00808 */ 00809 void BSP_LCD_FillTriangle(uint16_t X1, uint16_t X2, uint16_t X3, uint16_t Y1, uint16_t Y2, uint16_t Y3) 00810 { 00811 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00812 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00813 curpixel = 0; 00814 00815 deltax = ABS(X2 - X1); /* The difference between the x's */ 00816 deltay = ABS(Y2 - Y1); /* The difference between the y's */ 00817 x = X1; /* Start x off at the first pixel */ 00818 y = Y1; /* Start y off at the first pixel */ 00819 00820 if (X2 >= X1) /* The x-values are increasing */ 00821 { 00822 xinc1 = 1; 00823 xinc2 = 1; 00824 } 00825 else /* The x-values are decreasing */ 00826 { 00827 xinc1 = -1; 00828 xinc2 = -1; 00829 } 00830 00831 if (Y2 >= Y1) /* The y-values are increasing */ 00832 { 00833 yinc1 = 1; 00834 yinc2 = 1; 00835 } 00836 else /* The y-values are decreasing */ 00837 { 00838 yinc1 = -1; 00839 yinc2 = -1; 00840 } 00841 00842 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00843 { 00844 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00845 yinc2 = 0; /* Don't change the y for every iteration */ 00846 den = deltax; 00847 num = deltax / 2; 00848 numadd = deltay; 00849 numpixels = deltax; /* There are more x-values than y-values */ 00850 } 00851 else /* There is at least one y-value for every x-value */ 00852 { 00853 xinc2 = 0; /* Don't change the x for every iteration */ 00854 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00855 den = deltay; 00856 num = deltay / 2; 00857 numadd = deltax; 00858 numpixels = deltay; /* There are more y-values than x-values */ 00859 } 00860 00861 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00862 { 00863 BSP_LCD_DrawLine(x, y, X3, Y3); 00864 00865 num += numadd; /* Increase the numerator by the top of the fraction */ 00866 if (num >= den) /* Check if numerator >= denominator */ 00867 { 00868 num -= den; /* Calculate the new numerator value */ 00869 x += xinc1; /* Change the x as appropriate */ 00870 y += yinc1; /* Change the y as appropriate */ 00871 } 00872 x += xinc2; /* Change the x as appropriate */ 00873 y += yinc2; /* Change the y as appropriate */ 00874 } 00875 } 00876 00877 /** 00878 * @brief Displays a full poly-line (between many points). 00879 * @param pPoints: pointer to the points array. 00880 * @param PointCount: Number of points. 00881 * @retval None 00882 */ 00883 void BSP_LCD_FillPolygon(pPoint pPoints, uint16_t PointCount) 00884 { 00885 00886 int16_t x = 0, y = 0, x2 = 0, y2 = 0, xcenter = 0, ycenter = 0, xfirst = 0, yfirst = 0, pixelx = 0, pixely = 0, counter = 0; 00887 uint16_t imageleft = 0, imageright = 0, imagetop = 0, imagebottom = 0; 00888 00889 imageleft = imageright = pPoints->X; 00890 imagetop= imagebottom = pPoints->Y; 00891 00892 for(counter = 1; counter < PointCount; counter++) 00893 { 00894 pixelx = POLY_X(counter); 00895 if(pixelx < imageleft) 00896 { 00897 imageleft = pixelx; 00898 } 00899 if(pixelx > imageright) 00900 { 00901 imageright = pixelx; 00902 } 00903 00904 pixely = POLY_Y(counter); 00905 if(pixely < imagetop) 00906 { 00907 imagetop = pixely; 00908 } 00909 if(pixely > imagebottom) 00910 { 00911 imagebottom = pixely; 00912 } 00913 } 00914 00915 if(PointCount < 2) 00916 { 00917 return; 00918 } 00919 00920 xcenter = (imageleft + imageright)/2; 00921 ycenter = (imagebottom + imagetop)/2; 00922 00923 xfirst = pPoints->X; 00924 yfirst = pPoints->Y; 00925 00926 while(--PointCount) 00927 { 00928 x = pPoints->X; 00929 y = pPoints->Y; 00930 pPoints++; 00931 x2 = pPoints->X; 00932 y2 = pPoints->Y; 00933 00934 BSP_LCD_FillTriangle(x, x2, xcenter, y, y2, ycenter); 00935 BSP_LCD_FillTriangle(x, xcenter, x2, y, ycenter, y2); 00936 BSP_LCD_FillTriangle(xcenter, x2, x, ycenter, y2, y); 00937 } 00938 00939 BSP_LCD_FillTriangle(xfirst, x2, xcenter, yfirst, y2, ycenter); 00940 BSP_LCD_FillTriangle(xfirst, xcenter, x2, yfirst, ycenter, y2); 00941 BSP_LCD_FillTriangle(xcenter, x2, xfirst, ycenter, y2, yfirst); 00942 } 00943 00944 /** 00945 * @brief Draws a full ellipse. 00946 * @param Xpos: X position 00947 * @param Ypos: Y position 00948 * @param XRadius: Ellipse X radius 00949 * @param YRadius: Ellipse Y radius 00950 * @retval None 00951 */ 00952 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00953 { 00954 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00955 float k = 0, rad1 = 0, rad2 = 0; 00956 00957 rad1 = YRadius; 00958 rad2 = XRadius; 00959 00960 k = (float)(rad2/rad1); 00961 00962 do 00963 { 00964 BSP_LCD_DrawVLine((Xpos+y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00965 BSP_LCD_DrawVLine((Xpos-y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00966 00967 e2 = err; 00968 if (e2 <= x) 00969 { 00970 err += ++x*2+1; 00971 if (-y == x && e2 <= y) e2 = 0; 00972 } 00973 if (e2 > y) err += ++y*2+1; 00974 } 00975 while (y <= 0); 00976 } 00977 00978 /** 00979 * @brief Enables the display. 00980 * @retval None 00981 */ 00982 void BSP_LCD_DisplayOn(void) 00983 { 00984 lcd_drv->DisplayOn(); 00985 } 00986 00987 /** 00988 * @brief Disables the display. 00989 * @retval None 00990 */ 00991 void BSP_LCD_DisplayOff(void) 00992 { 00993 lcd_drv->DisplayOff(); 00994 } 00995 00996 /** 00997 * @} 00998 */ 00999 01000 /** @addtogroup STM3210E_EVAL_LCD_Private_Functions 01001 * @{ 01002 */ 01003 01004 /****************************************************************************** 01005 Static Function 01006 *******************************************************************************/ 01007 /** 01008 * @brief Draws a pixel on LCD. 01009 * @param Xpos: X position 01010 * @param Ypos: Y position 01011 * @param RGBCode: Pixel color in RGB mode (5-6-5) 01012 * @retval None 01013 */ 01014 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode) 01015 { 01016 if (LCD_SwapXY) 01017 { 01018 uint16_t tmp = Ypos; 01019 Ypos = Xpos; 01020 Xpos = tmp; 01021 } 01022 01023 if(lcd_drv->WritePixel != NULL) 01024 { 01025 lcd_drv->WritePixel(Xpos, Ypos, RGBCode); 01026 } 01027 } 01028 01029 /** 01030 * @brief Draws a character on LCD. 01031 * @param Xpos: Line where to display the character shape 01032 * @param Ypos: Start column address 01033 * @param pChar: Pointer to the character data 01034 * @retval None 01035 */ 01036 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar) 01037 { 01038 uint32_t counterh = 0, counterw = 0, index = 0; 01039 uint16_t height = 0, width = 0; 01040 uint8_t offset = 0; 01041 uint8_t *pchar = NULL; 01042 uint32_t line = 0; 01043 01044 01045 height = DrawProp.pFont->Height; 01046 width = DrawProp.pFont->Width; 01047 01048 /* Fill bitmap header*/ 01049 *(uint16_t *) (bitmap + 2) = (uint16_t)(height*width*2+OFFSET_BITMAP); 01050 *(uint16_t *) (bitmap + 4) = (uint16_t)((height*width*2+OFFSET_BITMAP)>>16); 01051 *(uint16_t *) (bitmap + 10) = OFFSET_BITMAP; 01052 *(uint16_t *) (bitmap + 18) = (uint16_t)(width); 01053 *(uint16_t *) (bitmap + 20) = (uint16_t)((width)>>16); 01054 *(uint16_t *) (bitmap + 22) = (uint16_t)(height); 01055 *(uint16_t *) (bitmap + 24) = (uint16_t)((height)>>16); 01056 01057 offset = 8 *((width + 7)/8) - width ; 01058 01059 for(counterh = 0; counterh < height; counterh++) 01060 { 01061 pchar = ((uint8_t *)pChar + (width + 7)/8 * counterh); 01062 01063 if(((width + 7)/8) == 3) 01064 { 01065 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 01066 } 01067 01068 if(((width + 7)/8) == 2) 01069 { 01070 line = (pchar[0]<< 8) | pchar[1]; 01071 } 01072 01073 if(((width + 7)/8) == 1) 01074 { 01075 line = pchar[0]; 01076 } 01077 01078 for (counterw = 0; counterw < width; counterw++) 01079 { 01080 /* Image in the bitmap is written from the bottom to the top */ 01081 /* Need to invert image in the bitmap */ 01082 index = (((height-counterh-1)*width)+(counterw))*2+OFFSET_BITMAP; 01083 if(line & (1 << (width- counterw + offset- 1))) 01084 { 01085 bitmap[index] = (uint8_t)DrawProp.TextColor; 01086 bitmap[index+1] = (uint8_t)(DrawProp.TextColor >> 8); 01087 } 01088 else 01089 { 01090 bitmap[index] = (uint8_t)DrawProp.BackColor; 01091 bitmap[index+1] = (uint8_t)(DrawProp.BackColor >> 8); 01092 } 01093 } 01094 } 01095 01096 BSP_LCD_DrawBitmap(Ypos, Xpos, bitmap); 01097 } 01098 01099 /** 01100 * @brief Sets display window. 01101 * @param Xpos: LCD X position 01102 * @param Ypos: LCD Y position 01103 * @param Width: LCD window width 01104 * @param Height: LCD window height 01105 * @retval None 01106 */ 01107 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 01108 { 01109 if(lcd_drv->SetDisplayWindow != NULL) 01110 { 01111 lcd_drv->SetDisplayWindow(Xpos, Ypos, Width, Height); 01112 } 01113 } 01114 01115 /** 01116 * @} 01117 */ 01118 01119 /** 01120 * @} 01121 */ 01122 01123 /** 01124 * @} 01125 */ 01126 01127 /** 01128 * @} 01129 */ 01130 01131 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 11 2014 16:16:37 for _BSP_User_Manual by
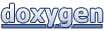