STM3210C_EVAL BSP User Manual
|
stm3210c_eval_eeprom.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval_eeprom.h 00004 * @author MCD Application Team 00005 * @version V7.0.0 00006 * @date 14-April-2017 00007 * @brief This file contains all the functions prototypes for 00008 * the stm3210c_eval_eeprom.c firmware driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM3210C_EVAL_EEPROM_H 00041 #define __STM3210C_EVAL_EEPROM_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm3210c_eval.h" 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @addtogroup STM3210C_EVAL 00055 * @{ 00056 */ 00057 00058 /** @addtogroup STM3210C_EVAL_EEPROM 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM3210C_EVAL_EEPROM_Exported_Types STM3210C EVAL EEPROM Exported Types 00063 * @{ 00064 */ 00065 typedef struct 00066 { 00067 uint32_t (*Init)(void); 00068 uint32_t (*ReadBuffer)(uint8_t* , uint16_t , uint32_t* ); 00069 uint32_t (*WritePage)(uint8_t* , uint16_t , uint32_t* ); 00070 }EEPROM_DrvTypeDef; 00071 /** 00072 * @} 00073 */ 00074 00075 /** @defgroup STM3210C_EVAL_EEPROM_Exported_Constants STM3210C EVAL EEPROM Exported Constants 00076 * @{ 00077 */ 00078 /* EEPROMs hardware address and page size */ 00079 #define EEPROM_ADDRESS_M24C64_32 0xA0 /* Support the devices: M24C32 and M24C64 */ 00080 /* The M24C08W contains 4 blocks (128byte each) with the adresses below: E2 = 0 00081 EEPROM Addresses defines */ 00082 #define EEPROM_ADDRESS_M24C08_BLOCK0 0xA0 00083 #define EEPROM_ADDRESS_M24C08_BLOCK1 0xA2 00084 #define EEPROM_ADDRESS_M24C08_BLOCK2 0xA4 00085 #define EEPROM_ADDRESS_M24C08_BLOCK3 0xA6 00086 00087 #define EEPROM_PAGESIZE_M24C64_32 32 /* Support the devices: M24C32 and M24C64 */ 00088 #define EEPROM_PAGESIZE_M24C08 16 /* Support the device: M24C08. */ 00089 00090 /* EEPROM BSP return values */ 00091 #define EEPROM_OK 0 00092 #define EEPROM_FAIL 1 00093 #define EEPROM_TIMEOUT 2 00094 00095 /* EEPROM BSP devices definition list supported */ 00096 #define BSP_EEPROM_M24C64_32 1 /* RF I2C EEPROM M24C32 and M24C64 */ 00097 #define BSP_EEPROM_M24C08 2 /* RF I2C EEPROM M24C08 */ 00098 00099 /* Maximum number of trials for EEPROM_I2C_WaitEepromStandbyState() function */ 00100 #define EEPROM_MAX_TRIALS 300 00101 /** 00102 * @} 00103 */ 00104 00105 /** @addtogroup STM3210C_EVAL_EEPROM_Exported_Functions 00106 * @{ 00107 */ 00108 uint32_t BSP_EEPROM_Init(void); 00109 void BSP_EEPROM_SelectDevice(uint8_t DeviceID); 00110 uint32_t BSP_EEPROM_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead); 00111 uint32_t BSP_EEPROM_WriteBuffer(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t NumByteToWrite); 00112 00113 /* USER Callbacks: This function is declared as __weak in EEPROM driver and 00114 should be implemented into user application. 00115 BSP_EEPROM_TIMEOUT_UserCallback() function is called whenever a timeout condition 00116 occure during communication (waiting on an event that doesn't occur, bus 00117 errors, busy devices ...). */ 00118 void BSP_EEPROM_TIMEOUT_UserCallback(void); 00119 00120 00121 /* Link functions for I2C EEPROM peripheral */ 00122 void EEPROM_I2C_IO_Init(void); 00123 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00124 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00125 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00126 00127 #ifdef __cplusplus 00128 } 00129 #endif 00130 00131 #endif /* __STM3210C_EVAL_EEPROM_H */ 00132 /** 00133 * @} 00134 */ 00135 00136 /** 00137 * @} 00138 */ 00139 00140 /** 00141 * @} 00142 */ 00143 00144 /** 00145 * @} 00146 */ 00147 00148 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Apr 14 2017 13:00:47 for STM3210C_EVAL BSP User Manual by
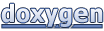