_BSP_User_Manual
|
Functions | |
uint8_t | BSP_SD_Init (void) |
Initializes the SD/SD communication. | |
uint8_t | BSP_SD_IsDetected (void) |
Detects if SD card is correctly plugged in the memory slot or not. | |
uint8_t | BSP_SD_GetCardInfo (SD_CardInfo *pCardInfo) |
Returns information about specific card. | |
uint8_t | BSP_SD_ReadBlocks (uint32_t *p32Data, uint64_t ReadAddr, uint16_t BlockSize, uint32_t NumberOfBlocks) |
Reads block(s) from a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocks (uint32_t *p32Data, uint64_t WriteAddr, uint16_t BlockSize, uint32_t NumberOfBlocks) |
Writes block(s) to a specified address in an SD card, in polling mode. | |
uint8_t | BSP_SD_GetStatus (void) |
Returns the SD status. | |
uint8_t | BSP_SD_Erase (uint32_t StartAddr, uint32_t EndAddr) |
Erases the specified memory area of the given SD card. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_WriteByte (uint8_t Data) |
Write a byte on the SD. | |
uint8_t | SD_IO_ReadByte (void) |
Read a byte from the SD. | |
HAL_StatusTypeDef | SD_IO_WriteCmd (uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) |
Send 5 bytes command to the SD card and get response. | |
HAL_StatusTypeDef | SD_IO_WaitResponse (uint8_t Response) |
Wait response from the SD card. | |
void | SD_IO_WriteDummy (void) |
Send dummy byte with CS High. | |
static uint8_t | SD_GetCSDRegister (SD_CSD *Csd) |
Read the CSD card register. | |
static uint8_t | SD_GetCIDRegister (SD_CID *Cid) |
Read the CID card register. | |
static uint8_t | SD_SendCmd (uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) |
Send 5 bytes command to the SD card and get response. | |
static SD_Info | SD_GetDataResponse (void) |
Get SD card data response. | |
static uint8_t | SD_GoIdleState (void) |
Put SD in Idle state. |
Function Documentation
uint8_t BSP_SD_Erase | ( | uint32_t | StartAddr, |
uint32_t | EndAddr | ||
) |
Erases the specified memory area of the given SD card.
Definition at line 684 of file stm3210c_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_CMD_ERASE, SD_CMD_SD_ERASE_GRP_END, SD_CMD_SD_ERASE_GRP_START, SD_RESPONSE_NO_ERROR, and SD_SendCmd().
uint8_t BSP_SD_GetCardInfo | ( | SD_CardInfo * | pCardInfo | ) |
Returns information about specific card.
Definition at line 217 of file stm3210c_eval_sd.c.
References SD_CardInfo::CardBlockSize, SD_CardInfo::CardCapacity, SD_CardInfo::Cid, SD_CardInfo::Csd, SD_CSD::DeviceSize, SD_CSD::DeviceSizeMul, MSD_ERROR, SD_CSD::RdBlockLen, SD_GetCIDRegister(), and SD_GetCSDRegister().
uint8_t BSP_SD_GetStatus | ( | void | ) |
uint8_t BSP_SD_Init | ( | void | ) |
Initializes the SD/SD communication.
Definition at line 172 of file stm3210c_eval_sd.c.
References BSP_SD_IsDetected(), MSD_ERROR, SD_GoIdleState(), SD_IO_Init(), SD_NOT_PRESENT, SD_PRESENT, and SdStatus.
uint8_t BSP_SD_IsDetected | ( | void | ) |
Detects if SD card is correctly plugged in the memory slot or not.
Definition at line 196 of file stm3210c_eval_sd.c.
References SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_NOT_PRESENT, and SD_PRESENT.
Referenced by BSP_SD_Init().
uint8_t BSP_SD_ReadBlocks | ( | uint32_t * | p32Data, |
uint64_t | ReadAddr, | ||
uint16_t | BlockSize, | ||
uint32_t | NumberOfBlocks | ||
) |
Reads block(s) from a specified address in an SD card, in polling mode.
Definition at line 240 of file stm3210c_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_CMD_READ_SINGLE_BLOCK, SD_CMD_SET_BLOCKLEN, SD_IO_ReadByte(), SD_IO_WaitResponse(), SD_IO_WriteCmd(), SD_IO_WriteDummy(), SD_RESPONSE_NO_ERROR, and SD_START_DATA_SINGLE_BLOCK_READ.
uint8_t BSP_SD_WriteBlocks | ( | uint32_t * | p32Data, |
uint64_t | WriteAddr, | ||
uint16_t | BlockSize, | ||
uint32_t | NumberOfBlocks | ||
) |
Writes block(s) to a specified address in an SD card, in polling mode.
Definition at line 306 of file stm3210c_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_CMD_WRITE_SINGLE_BLOCK, SD_DATA_OK, SD_DUMMY_BYTE, SD_GetDataResponse(), SD_IO_ReadByte(), SD_IO_WriteByte(), SD_IO_WriteCmd(), SD_IO_WriteDummy(), SD_RESPONSE_NO_ERROR, and SD_START_DATA_SINGLE_BLOCK_WRITE.
static uint8_t SD_GetCIDRegister | ( | SD_CID * | Cid | ) | [static] |
Read the CID card register.
Reading the contents of the CID register in SPI mode is a simple read-block transaction.
Definition at line 490 of file stm3210c_eval_sd.c.
References SD_CID::CID_CRC, SD_CID::ManufactDate, SD_CID::ManufacturerID, MSD_ERROR, MSD_OK, SD_CID::OEM_AppliID, SD_CID::ProdName1, SD_CID::ProdName2, SD_CID::ProdRev, SD_CID::ProdSN, SD_CID::Reserved1, SD_CID::Reserved2, SD_CMD_SEND_CID, SD_DUMMY_BYTE, SD_IO_ReadByte(), SD_IO_WaitResponse(), SD_IO_WriteByte(), SD_IO_WriteCmd(), SD_IO_WriteDummy(), SD_RESPONSE_NO_ERROR, and SD_START_DATA_SINGLE_BLOCK_READ.
Referenced by BSP_SD_GetCardInfo().
uint8_t SD_GetCSDRegister | ( | SD_CSD * | Csd | ) | [static] |
Read the CSD card register.
Reading the contents of the CSD register in SPI mode is a simple read-block transaction.
< Reserved
Definition at line 372 of file stm3210c_eval_sd.c.
References SD_CSD::CardComdClasses, SD_CSD::ContentProtectAppli, SD_CSD::CopyFlag, SD_CSD::CSD_CRC, SD_CSD::CSDStruct, SD_CSD::DeviceSize, SD_CSD::DeviceSizeMul, SD_CSD::DSRImpl, SD_CSD::ECC, SD_CSD::EraseGrMul, SD_CSD::EraseGrSize, SD_CSD::FileFormat, SD_CSD::FileFormatGrouop, SD_CSD::ManDeflECC, SD_CSD::MaxBusClkFrec, SD_CSD::MaxRdCurrentVDDMax, SD_CSD::MaxRdCurrentVDDMin, SD_CSD::MaxWrBlockLen, SD_CSD::MaxWrCurrentVDDMax, SD_CSD::MaxWrCurrentVDDMin, MSD_ERROR, MSD_OK, SD_CSD::NSAC, SD_CSD::PartBlockRead, SD_CSD::PermWrProtect, SD_CSD::RdBlockLen, SD_CSD::RdBlockMisalign, SD_CSD::Reserved1, SD_CSD::Reserved2, SD_CSD::Reserved3, SD_CSD::Reserved4, SD_CMD_SEND_CSD, SD_DUMMY_BYTE, SD_IO_ReadByte(), SD_IO_WaitResponse(), SD_IO_WriteByte(), SD_IO_WriteCmd(), SD_IO_WriteDummy(), SD_RESPONSE_NO_ERROR, SD_START_DATA_SINGLE_BLOCK_READ, SD_CSD::SysSpecVersion, SD_CSD::TAAC, SD_CSD::TempWrProtect, SD_CSD::WrBlockMisalign, SD_CSD::WriteBlockPaPartial, SD_CSD::WrProtectGrEnable, SD_CSD::WrProtectGrSize, and SD_CSD::WrSpeedFact.
Referenced by BSP_SD_GetCardInfo().
static SD_Info SD_GetDataResponse | ( | void | ) | [static] |
Get SD card data response.
Definition at line 606 of file stm3210c_eval_sd.c.
References SD_DATA_CRC_ERROR, SD_DATA_OK, SD_DATA_OTHER_ERROR, SD_DATA_WRITE_ERROR, and SD_IO_ReadByte().
Referenced by BSP_SD_WriteBlocks().
static uint8_t SD_GoIdleState | ( | void | ) | [static] |
Put SD in Idle state.
Definition at line 661 of file stm3210c_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_CMD_GO_IDLE_STATE, SD_CMD_SEND_OP_COND, SD_IN_IDLE_STATE, SD_RESPONSE_NO_ERROR, and SD_SendCmd().
Referenced by BSP_SD_Init().
void SD_IO_Init | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
Definition at line 1115 of file stm3210c_eval.c.
References SD_CS_GPIO_CLK_ENABLE, SD_CS_GPIO_PORT, SD_CS_HIGH, SD_CS_PIN, SD_DETECT_EXTI_IRQn, SD_DETECT_GPIO_CLK_ENABLE, SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_DUMMY_BYTE, SD_IO_WriteByte(), and SPIx_Init().
Referenced by BSP_SD_Init().
uint8_t SD_IO_ReadByte | ( | void | ) |
Read a byte from the SD.
Definition at line 1172 of file stm3210c_eval.c.
References SPIx_Read().
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_GetDataResponse(), and SD_IO_WaitResponse().
HAL_StatusTypeDef SD_IO_WaitResponse | ( | uint8_t | Response | ) |
Wait response from the SD card.
Definition at line 1226 of file stm3210c_eval.c.
References SD_IO_ReadByte().
Referenced by BSP_SD_ReadBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_IO_WriteCmd().
void SD_IO_WriteByte | ( | uint8_t | Data | ) |
Write a byte on the SD.
Definition at line 1162 of file stm3210c_eval.c.
References SPIx_Write().
Referenced by BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_IO_Init(), SD_IO_WriteCmd(), and SD_IO_WriteDummy().
HAL_StatusTypeDef SD_IO_WriteCmd | ( | uint8_t | Cmd, |
uint32_t | Arg, | ||
uint8_t | Crc, | ||
uint8_t | Response | ||
) |
Send 5 bytes command to the SD card and get response.
Definition at line 1191 of file stm3210c_eval.c.
References SD_CS_LOW, SD_IO_WaitResponse(), SD_IO_WriteByte(), and SD_NO_RESPONSE_EXPECTED.
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_SendCmd().
void SD_IO_WriteDummy | ( | void | ) |
Send dummy byte with CS High.
Definition at line 1254 of file stm3210c_eval.c.
References SD_CS_HIGH, SD_DUMMY_BYTE, and SD_IO_WriteByte().
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_SendCmd().
static uint8_t SD_SendCmd | ( | uint8_t | Cmd, |
uint32_t | Arg, | ||
uint8_t | Crc, | ||
uint8_t | Response | ||
) | [static] |
Send 5 bytes command to the SD card and get response.
Definition at line 583 of file stm3210c_eval_sd.c.
References MSD_ERROR, MSD_OK, SD_IO_WriteCmd(), and SD_IO_WriteDummy().
Referenced by BSP_SD_Erase(), and SD_GoIdleState().
Generated on Thu Dec 11 2014 15:38:29 for _BSP_User_Manual by
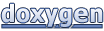