_BSP_User_Manual
|
Functions | |
void | IOE_Init (void) |
Initializes IOE low level. | |
void | IOE_ITConfig (void) |
Configures IOE low level Interrupt. | |
void | IOE_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
IOE writes single data. | |
uint8_t | IOE_Read (uint8_t Addr, uint8_t Reg) |
IOE reads single data. | |
uint16_t | IOE_ReadMultiple (uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) |
IOE reads multiple data. | |
void | IOE_Delay (uint32_t Delay) |
IOE delay. | |
void | LCD_IO_Init (void) |
Configures the LCD_SPI interface. | |
void | LCD_IO_WriteMultipleData (uint8_t *pData, uint32_t Size) |
Write register value. | |
void | LCD_IO_WriteReg (uint8_t Reg) |
register address. | |
uint16_t | LCD_IO_ReadData (uint16_t Reg) |
Read register value. | |
void | LCD_Delay (uint32_t Delay) |
Wait for loop in ms. | |
void | SD_IO_Init (void) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_WriteByte (uint8_t Data) |
Write a byte on the SD. | |
uint8_t | SD_IO_ReadByte (void) |
Read a byte from the SD. | |
HAL_StatusTypeDef | SD_IO_WriteCmd (uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) |
Send 5 bytes command to the SD card and get response. | |
HAL_StatusTypeDef | SD_IO_WaitResponse (uint8_t Response) |
Wait response from the SD card. | |
void | SD_IO_WriteDummy (void) |
Send dummy byte with CS High. | |
void | EEPROM_I2C_IO_Init (void) |
Initializes peripherals used by the I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_I2C_IO_WriteData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Write data to I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_I2C_IO_ReadData (uint16_t DevAddress, uint16_t MemAddress, uint8_t *pBuffer, uint32_t BufferSize) |
Read data from I2C EEPROM driver. | |
HAL_StatusTypeDef | EEPROM_I2C_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
void | TSENSOR_IO_Init (void) |
Initializes peripherals used by the I2C Temperature Sensor driver. | |
void | TSENSOR_IO_Write (uint16_t DevAddress, uint8_t *pBuffer, uint8_t WriteAddr, uint16_t Length) |
Writes one byte to the TSENSOR. | |
void | TSENSOR_IO_Read (uint16_t DevAddress, uint8_t *pBuffer, uint8_t ReadAddr, uint16_t Length) |
Reads one byte from the TSENSOR. | |
uint16_t | TSENSOR_IO_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if Temperature Sensor is ready for communication. | |
void | ACCELERO_IO_Init (void) |
Configures ACCELEROMETER SPI interface. | |
void | ACCELERO_IO_ITConfig (void) |
Configures ACCELERO INT2 config. | |
void | ACCELERO_IO_Write (uint8_t *pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite) |
Writes one byte to the ACCELEROMETER. | |
void | ACCELERO_IO_Read (uint8_t *pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead) |
Reads a block of data from the ACCELEROMETER. | |
void | AUDIO_IO_Init (void) |
Initializes Audio low level. | |
void | AUDIO_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
Writes a single data. | |
uint8_t | AUDIO_IO_Read (uint8_t Addr, uint8_t Reg) |
Reads a single data. |
Function Documentation
void ACCELERO_IO_Init | ( | void | ) |
Configures ACCELEROMETER SPI interface.
Definition at line 1366 of file stm3210c_eval.c.
References BSP_IO_Init().
void ACCELERO_IO_ITConfig | ( | void | ) |
Configures ACCELERO INT2 config.
EXTI0 is already used by user button so INT1 is configured here
Definition at line 1378 of file stm3210c_eval.c.
References BSP_IO_ConfigPin(), and MEMS_ALL_PINS.
void ACCELERO_IO_Read | ( | uint8_t * | pBuffer, |
uint8_t | ReadAddr, | ||
uint16_t | NumByteToRead | ||
) |
Reads a block of data from the ACCELEROMETER.
Definition at line 1402 of file stm3210c_eval.c.
References I2Cx_ReadBuffer(), and L1S302DL_I2C_ADDRESS.
void ACCELERO_IO_Write | ( | uint8_t * | pBuffer, |
uint8_t | WriteAddr, | ||
uint16_t | NumByteToWrite | ||
) |
Writes one byte to the ACCELEROMETER.
Definition at line 1390 of file stm3210c_eval.c.
References I2Cx_WriteBuffer(), and L1S302DL_I2C_ADDRESS.
void AUDIO_IO_Init | ( | void | ) |
Initializes Audio low level.
Definition at line 1413 of file stm3210c_eval.c.
References AUDIO_RESET_PIN, BSP_IO_ConfigPin(), BSP_IO_Init(), and BSP_IO_WritePin().
uint8_t AUDIO_IO_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) |
void AUDIO_IO_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
void EEPROM_I2C_IO_Init | ( | void | ) |
Initializes peripherals used by the I2C EEPROM driver.
Definition at line 1271 of file stm3210c_eval.c.
References I2Cx_Init().
Referenced by EEPROM_I2C_Init().
HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
Definition at line 1309 of file stm3210c_eval.c.
References I2Cx_IsDeviceReady().
Referenced by EEPROM_I2C_Init(), and EEPROM_I2C_WaitEepromStandbyState().
HAL_StatusTypeDef EEPROM_I2C_IO_ReadData | ( | uint16_t | DevAddress, |
uint16_t | MemAddress, | ||
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Read data from I2C EEPROM driver.
Definition at line 1297 of file stm3210c_eval.c.
References I2Cx_ReadBuffer().
Referenced by EEPROM_I2C_ReadBuffer().
HAL_StatusTypeDef EEPROM_I2C_IO_WriteData | ( | uint16_t | DevAddress, |
uint16_t | MemAddress, | ||
uint8_t * | pBuffer, | ||
uint32_t | BufferSize | ||
) |
Write data to I2C EEPROM driver.
Definition at line 1284 of file stm3210c_eval.c.
References I2Cx_WriteBuffer().
Referenced by EEPROM_I2C_WritePage().
void IOE_Delay | ( | uint32_t | Delay | ) |
IOE delay.
Definition at line 971 of file stm3210c_eval.c.
void IOE_Init | ( | void | ) |
void IOE_ITConfig | ( | void | ) |
Configures IOE low level Interrupt.
Definition at line 925 of file stm3210c_eval.c.
References I2Cx_ITConfig().
uint8_t IOE_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) |
uint16_t IOE_ReadMultiple | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t * | Buffer, | ||
uint16_t | Length | ||
) |
IOE reads multiple data.
Definition at line 961 of file stm3210c_eval.c.
References I2Cx_ReadMultiple().
void IOE_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
IOE writes single data.
Definition at line 937 of file stm3210c_eval.c.
References I2Cx_WriteData().
void LCD_Delay | ( | uint32_t | Delay | ) |
Wait for loop in ms.
Definition at line 1103 of file stm3210c_eval.c.
void LCD_IO_Init | ( | void | ) |
Configures the LCD_SPI interface.
Definition at line 985 of file stm3210c_eval.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_NCS_GPIO_CLK_ENABLE, LCD_NCS_GPIO_PORT, LCD_NCS_PIN, and SPIx_Init().
uint16_t LCD_IO_ReadData | ( | uint16_t | Reg | ) |
Read register value.
Definition at line 1073 of file stm3210c_eval.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_IO_WriteReg(), LCD_READ_REG, SPIx_Read(), SPIx_Write(), and START_BYTE.
void LCD_IO_WriteMultipleData | ( | uint8_t * | pData, |
uint32_t | Size | ||
) |
Write register value.
Definition at line 1012 of file stm3210c_eval.c.
References heval_Spi, LCD_CS_HIGH, LCD_CS_LOW, LCD_WRITE_REG, SPIx_Write(), and START_BYTE.
void LCD_IO_WriteReg | ( | uint8_t | Reg | ) |
register address.
Definition at line 1052 of file stm3210c_eval.c.
References LCD_CS_HIGH, LCD_CS_LOW, SET_INDEX, SPIx_Write(), and START_BYTE.
Referenced by LCD_IO_ReadData().
void SD_IO_Init | ( | void | ) |
Initializes the SD Card and put it into StandBy State (Ready for data transfer).
Definition at line 1115 of file stm3210c_eval.c.
References SD_CS_GPIO_CLK_ENABLE, SD_CS_GPIO_PORT, SD_CS_HIGH, SD_CS_PIN, SD_DETECT_EXTI_IRQn, SD_DETECT_GPIO_CLK_ENABLE, SD_DETECT_GPIO_PORT, SD_DETECT_PIN, SD_DUMMY_BYTE, SD_IO_WriteByte(), and SPIx_Init().
Referenced by BSP_SD_Init().
uint8_t SD_IO_ReadByte | ( | void | ) |
Read a byte from the SD.
Definition at line 1172 of file stm3210c_eval.c.
References SPIx_Read().
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_GetDataResponse(), and SD_IO_WaitResponse().
HAL_StatusTypeDef SD_IO_WaitResponse | ( | uint8_t | Response | ) |
Wait response from the SD card.
Definition at line 1226 of file stm3210c_eval.c.
References SD_IO_ReadByte().
Referenced by BSP_SD_ReadBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_IO_WriteCmd().
void SD_IO_WriteByte | ( | uint8_t | Data | ) |
Write a byte on the SD.
Definition at line 1162 of file stm3210c_eval.c.
References SPIx_Write().
Referenced by BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), SD_IO_Init(), SD_IO_WriteCmd(), and SD_IO_WriteDummy().
HAL_StatusTypeDef SD_IO_WriteCmd | ( | uint8_t | Cmd, |
uint32_t | Arg, | ||
uint8_t | Crc, | ||
uint8_t | Response | ||
) |
Send 5 bytes command to the SD card and get response.
Definition at line 1191 of file stm3210c_eval.c.
References SD_CS_LOW, SD_IO_WaitResponse(), SD_IO_WriteByte(), and SD_NO_RESPONSE_EXPECTED.
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_SendCmd().
void SD_IO_WriteDummy | ( | void | ) |
Send dummy byte with CS High.
Definition at line 1254 of file stm3210c_eval.c.
References SD_CS_HIGH, SD_DUMMY_BYTE, and SD_IO_WriteByte().
Referenced by BSP_SD_ReadBlocks(), BSP_SD_WriteBlocks(), SD_GetCIDRegister(), SD_GetCSDRegister(), and SD_SendCmd().
void TSENSOR_IO_Init | ( | void | ) |
Initializes peripherals used by the I2C Temperature Sensor driver.
Definition at line 1319 of file stm3210c_eval.c.
References I2Cx_Init().
uint16_t TSENSOR_IO_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) |
Checks if Temperature Sensor is ready for communication.
Definition at line 1356 of file stm3210c_eval.c.
References I2Cx_IsDeviceReady().
void TSENSOR_IO_Read | ( | uint16_t | DevAddress, |
uint8_t * | pBuffer, | ||
uint8_t | ReadAddr, | ||
uint16_t | Length | ||
) |
Reads one byte from the TSENSOR.
Definition at line 1345 of file stm3210c_eval.c.
References I2Cx_ReadBuffer().
void TSENSOR_IO_Write | ( | uint16_t | DevAddress, |
uint8_t * | pBuffer, | ||
uint8_t | WriteAddr, | ||
uint16_t | Length | ||
) |
Writes one byte to the TSENSOR.
Definition at line 1332 of file stm3210c_eval.c.
References I2Cx_WriteBuffer().
Generated on Thu Dec 11 2014 15:38:29 for _BSP_User_Manual by
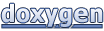