_BSP_User_Manual
|
stm3210c_eval.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval.h 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file contains definitions for STM3210C_EVAL's LEDs, 00008 * push-buttons and COM ports hardware resources. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /** @addtogroup BSP 00040 * @{ 00041 */ 00042 00043 /** @addtogroup STM3210C_EVAL 00044 * @{ 00045 */ 00046 00047 /** @addtogroup STM3210C_EVAL_COMMON 00048 * @{ 00049 */ 00050 00051 /* Define to prevent recursive inclusion -------------------------------------*/ 00052 #ifndef __STM3210C_EVAL_H 00053 #define __STM3210C_EVAL_H 00054 00055 #ifdef __cplusplus 00056 extern "C" { 00057 #endif 00058 00059 /* Includes ------------------------------------------------------------------*/ 00060 #include "stm32f1xx_hal.h" 00061 #ifdef HAL_I2C_MODULE_ENABLED 00062 #include "stm3210c_eval_io.h" 00063 #endif /* HAL_I2C_MODULE_ENABLED */ 00064 00065 /** @defgroup STM3210C_EVAL_Exported_Types Exported Types 00066 * @{ 00067 */ 00068 00069 /** 00070 * @brief LED Types Definition 00071 */ 00072 typedef enum 00073 { 00074 LED1 = 0, 00075 LED2 = 1, 00076 LED3 = 2, 00077 LED4 = 3, 00078 00079 LED_GREEN = LED1, 00080 LED_ORANGE = LED2, 00081 LED_RED = LED3, 00082 LED_BLUE = LED4 00083 00084 } Led_TypeDef; 00085 00086 /** 00087 * @brief BUTTON Types Definition 00088 */ 00089 typedef enum 00090 { 00091 BUTTON_WAKEUP = 0, 00092 BUTTON_TAMPER = 1, 00093 BUTTON_KEY = 2, 00094 00095 } Button_TypeDef; 00096 00097 typedef enum 00098 { 00099 BUTTON_MODE_GPIO = 0, 00100 BUTTON_MODE_EXTI = 1 00101 } ButtonMode_TypeDef; 00102 00103 /** 00104 * @brief JOYSTICK Types Definition 00105 */ 00106 typedef enum 00107 { 00108 JOY_SEL = 0, 00109 JOY_LEFT = 1, 00110 JOY_RIGHT = 2, 00111 JOY_DOWN = 3, 00112 JOY_UP = 4, 00113 JOY_NONE = 5 00114 00115 }JOYState_TypeDef; 00116 00117 typedef enum 00118 { 00119 JOY_MODE_GPIO = 0, 00120 JOY_MODE_EXTI = 1 00121 00122 }JOYMode_TypeDef; 00123 00124 /** 00125 * @brief COM Types Definition 00126 */ 00127 typedef enum 00128 { 00129 COM1 = 0, 00130 COM2 = 1 00131 } COM_TypeDef; 00132 /** 00133 * @} 00134 */ 00135 00136 /** @defgroup STM3210C_EVAL_Exported_Constants Exported Constants 00137 * @{ 00138 */ 00139 00140 /** 00141 * @brief Define for STM3210C_EVAL board 00142 */ 00143 #if !defined (USE_STM3210C_EVAL) 00144 #define USE_STM3210C_EVAL 00145 #endif 00146 00147 /** @addtogroup STM3210C_EVAL_LED 00148 * @{ 00149 */ 00150 #define LEDn 4 00151 00152 #define LED1_PIN GPIO_PIN_7 /* PD.07*/ 00153 #define LED1_GPIO_PORT GPIOD 00154 #define LED1_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00155 #define LED1_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00156 00157 #define LED2_PIN GPIO_PIN_13 /* PD.13*/ 00158 #define LED2_GPIO_PORT GPIOD 00159 #define LED2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00160 #define LED2_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00161 00162 00163 #define LED3_PIN GPIO_PIN_3 /* PD.03*/ 00164 #define LED3_GPIO_PORT GPIOD 00165 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00166 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00167 00168 00169 #define LED4_PIN GPIO_PIN_4 /* PD.04*/ 00170 #define LED4_GPIO_PORT GPIOD 00171 #define LED4_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00172 #define LED4_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00173 00174 #define LEDx_GPIO_CLK_ENABLE(__LED__) do { if ((__LED__) == LED1) LED1_GPIO_CLK_ENABLE(); else \ 00175 if ((__LED__) == LED2) LED2_GPIO_CLK_ENABLE(); else \ 00176 if ((__LED__) == LED3) LED3_GPIO_CLK_ENABLE(); else \ 00177 if ((__LED__) == LED4) LED4_GPIO_CLK_ENABLE();} while(0) 00178 00179 #define LEDx_GPIO_CLK_DISABLE(__LED__) (((__LED__) == LED1) ? LED1_GPIO_CLK_DISABLE() :\ 00180 ((__LED__) == LED2) ? LED2_GPIO_CLK_DISABLE() :\ 00181 ((__LED__) == LED3) ? LED3_GPIO_CLK_DISABLE() :\ 00182 ((__LED__) == LED4) ? LED4_GPIO_CLK_DISABLE() : 0 ) 00183 00184 /** 00185 * @} 00186 */ 00187 00188 /** @addtogroup STM3210C_EVAL_BUTTON 00189 * @{ 00190 */ 00191 #define BUTTONn 3 00192 00193 /** 00194 * @brief Tamper push-button 00195 */ 00196 #define TAMPER_BUTTON_PIN GPIO_PIN_13 /* PC.13*/ 00197 #define TAMPER_BUTTON_GPIO_PORT GPIOC 00198 #define TAMPER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00199 #define TAMPER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00200 #define TAMPER_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00201 00202 /** 00203 * @brief Key push-button 00204 */ 00205 #define KEY_BUTTON_PIN GPIO_PIN_9 /* PB.09*/ 00206 #define KEY_BUTTON_GPIO_PORT GPIOB 00207 #define KEY_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00208 #define KEY_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00209 #define KEY_BUTTON_EXTI_IRQn EXTI9_5_IRQn 00210 00211 /** 00212 * @brief Wake-up push-button 00213 */ 00214 #define WAKEUP_BUTTON_PIN GPIO_PIN_0 /* PA.00*/ 00215 #define WAKEUP_BUTTON_GPIO_PORT GPIOA 00216 #define WAKEUP_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00217 #define WAKEUP_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00218 #define WAKEUP_BUTTON_EXTI_IRQn EXTI0_IRQn 00219 00220 #define BUTTONx_GPIO_CLK_ENABLE(__BUTTON__) do { if ((__BUTTON__) == BUTTON_TAMPER) TAMPER_BUTTON_GPIO_CLK_ENABLE() ; else \ 00221 if ((__BUTTON__) == BUTTON_KEY) KEY_BUTTON_GPIO_CLK_ENABLE() ; else \ 00222 if ((__BUTTON__) == BUTTON_WAKEUP) WAKEUP_BUTTON_GPIO_CLK_ENABLE();} while(0) 00223 00224 #define BUTTONx_GPIO_CLK_DISABLE(__BUTTON__) (((__BUTTON__) == BUTTON_TAMPER) TAMPER_BUTTON_GPIO_CLK_DISABLE() :\ 00225 ((__BUTTON__) == BUTTON_KEY) KEY_BUTTON_GPIO_CLK_DISABLE() :\ 00226 ((__BUTTON__) == BUTTON_WAKEUP) WAKEUP_BUTTON_GPIO_CLK_DISABLE() : 0 ) 00227 00228 /** 00229 * @brief IO Pins definition 00230 */ 00231 /* Joystick */ 00232 #define JOY_SEL_PIN (IO2_PIN_7) /* IO_Expander_2 */ 00233 #define JOY_DOWN_PIN (IO2_PIN_6) /* IO_Expander_2 */ 00234 #define JOY_LEFT_PIN (IO2_PIN_5) /* IO_Expander_2 */ 00235 #define JOY_RIGHT_PIN (IO2_PIN_4) /* IO_Expander_2 */ 00236 #define JOY_UP_PIN (IO2_PIN_3) /* IO_Expander_2 */ 00237 #define JOY_NONE_PIN JOY_ALL_PINS 00238 #define JOY_ALL_PINS (JOY_SEL_PIN | JOY_DOWN_PIN | JOY_LEFT_PIN | JOY_RIGHT_PIN | JOY_UP_PIN) 00239 00240 /* MEMS */ 00241 #define MEMS_INT1_PIN (IO1_PIN_3) /* IO_Expander_1 */ /* Input */ 00242 #define MEMS_INT2_PIN (IO1_PIN_2) /* IO_Expander_1 */ /* Input */ 00243 #define MEMS_ALL_PINS (MEMS_INT1_PIN | MEMS_INT2_PIN) 00244 00245 #define AUDIO_RESET_PIN (IO2_PIN_2) /* IO_Expander_2 */ /* Output */ 00246 #define MII_INT_PIN (IO2_PIN_0) /* IO_Expander_2 */ /* Output */ 00247 #define VBAT_DIV_PIN (IO1_PIN_0) /* IO_Expander_1 */ /* Output */ 00248 00249 /** 00250 * @} 00251 */ 00252 00253 /** @addtogroup STM3210C_EVAL_COM 00254 * @{ 00255 */ 00256 #define COMn 1 00257 00258 /** 00259 * @brief Definition for COM port1, connected to USART2 00260 */ 00261 #define EVAL_COM1 USART2 00262 #define EVAL_COM1_CLK_ENABLE() __HAL_RCC_USART2_CLK_ENABLE() 00263 #define EVAL_COM1_CLK_DISABLE() __HAL_RCC_USART2_CLK_DISABLE() 00264 00265 #define AFIOCOM1_CLK_ENABLE() __HAL_RCC_AFIO_CLK_ENABLE() 00266 #define AFIOCOM1_CLK_DISABLE() __HAL_RCC_AFIO_CLK_DISABLE() 00267 00268 #define EVAL_COM1_TX_PIN GPIO_PIN_5 /* PD.05*/ 00269 #define EVAL_COM1_TX_GPIO_PORT GPIOD 00270 #define EVAL_COM1_TX_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00271 #define EVAL_COM1_TX_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00272 00273 #define EVAL_COM1_RX_PIN GPIO_PIN_6 /* PD.06*/ 00274 #define EVAL_COM1_RX_GPIO_PORT GPIOD 00275 #define EVAL_COM1_RX_GPIO_CLK_ENABLE() __HAL_RCC_GPIOD_CLK_ENABLE() 00276 #define EVAL_COM1_RX_GPIO_CLK_DISABLE() __HAL_RCC_GPIOD_CLK_DISABLE() 00277 00278 #define EVAL_COM1_IRQn USART2_IRQn 00279 00280 #define COMx_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == COM1) EVAL_COM1_CLK_ENABLE();} while(0) 00281 #define COMx_CLK_DISABLE(__INDEX__) (((__INDEX__) == COM1) ? EVAL_COM1_CLK_DISABLE() : 0) 00282 00283 #define AFIOCOMx_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == COM1) AFIOCOM1_CLK_ENABLE();} while(0) 00284 #define AFIOCOMx_CLK_DISABLE(__INDEX__) (((__INDEX__) == COM1) ? AFIOCOM1_CLK_DISABLE() : 0) 00285 00286 #define AFIOCOMx_REMAP(__INDEX__) (((__INDEX__) == COM1) ? (AFIO->MAPR |= (AFIO_MAPR_USART2_REMAP)) : 0) 00287 00288 #define COMx_TX_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == COM1) EVAL_COM1_TX_GPIO_CLK_ENABLE();} while(0) 00289 #define COMx_TX_GPIO_CLK_DISABLE(__INDEX__) (((__INDEX__) == COM1) ? EVAL_COM1_TX_GPIO_CLK_DISABLE() : 0) 00290 00291 #define COMx_RX_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == COM1) EVAL_COM1_RX_GPIO_CLK_ENABLE();} while(0) 00292 #define COMx_RX_GPIO_CLK_DISABLE(__INDEX__) (((__INDEX__) == COM1) ? EVAL_COM1_RX_GPIO_CLK_DISABLE() : 0) 00293 00294 /** 00295 * @} 00296 */ 00297 00298 /** @addtogroup STM3210C_EVAL_BUS 00299 * @{ 00300 */ 00301 00302 /** 00303 * @brief IO Expander Interrupt line on EXTI 00304 */ 00305 #define IOE_IT_PIN GPIO_PIN_14 00306 #define IOE_IT_GPIO_PORT GPIOB 00307 #define IOE_IT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00308 #define IOE_IT_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00309 #define IOE_IT_EXTI_IRQn EXTI15_10_IRQn 00310 #define IOE_IT_EXTI_IRQHANDLER EXTI15_10_IRQHandler 00311 00312 /* Exported constant IO ------------------------------------------------------*/ 00313 #define IO1_I2C_ADDRESS 0x82 00314 #define IO2_I2C_ADDRESS 0x88 00315 #define TS_I2C_ADDRESS 0x82 00316 00317 /*The Slave ADdress (SAD) associated to the LIS302DL is 001110xb. SDO pad can be used 00318 to modify less significant bit of the device address. If SDO pad is connected to voltage 00319 supply LSb is �1� (address 0011101b) else if SDO pad is connected to ground LSb value is 00320 �0� (address 0011100b).*/ 00321 #define L1S302DL_I2C_ADDRESS 0x38 00322 00323 00324 /*##################### ACCELEROMETER ##########################*/ 00325 /* Read/Write command */ 00326 #define READWRITE_CMD ((uint8_t)0x80) 00327 /* Multiple byte read/write command */ 00328 #define MULTIPLEBYTE_CMD ((uint8_t)0x40) 00329 00330 /*##################### I2Cx ###################################*/ 00331 /* User can use this section to tailor I2Cx instance used and associated 00332 resources */ 00333 /* Definition for I2Cx Pins */ 00334 #define EVAL_I2Cx_SCL_PIN GPIO_PIN_6 /* PB.06*/ 00335 #define EVAL_I2Cx_SCL_GPIO_PORT GPIOB 00336 #define EVAL_I2Cx_SDA_PIN GPIO_PIN_7 /* PB.07*/ 00337 #define EVAL_I2Cx_SDA_GPIO_PORT GPIOB 00338 00339 /* Definition for I2Cx clock resources */ 00340 #define EVAL_I2Cx I2C1 00341 #define EVAL_I2Cx_CLK_ENABLE() __HAL_RCC_I2C1_CLK_ENABLE() 00342 #define EVAL_I2Cx_SDA_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00343 #define EVAL_I2Cx_SCL_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00344 00345 #define EVAL_I2Cx_FORCE_RESET() __HAL_RCC_I2C1_FORCE_RESET() 00346 #define EVAL_I2Cx_RELEASE_RESET() __HAL_RCC_I2C1_RELEASE_RESET() 00347 00348 /* Definition for I2Cx's NVIC */ 00349 #define EVAL_I2Cx_EV_IRQn I2C1_EV_IRQn 00350 #define EVAL_I2Cx_EV_IRQHandler I2C1_EV_IRQHandler 00351 #define EVAL_I2Cx_ER_IRQn I2C1_ER_IRQn 00352 #define EVAL_I2Cx_ER_IRQHandler I2C1_ER_IRQHandler 00353 00354 /* I2C clock speed configuration (in Hz) */ 00355 #ifndef BSP_I2C_SPEED 00356 #define BSP_I2C_SPEED 400000 00357 #endif /* I2C_SPEED */ 00358 00359 00360 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00361 on accurate values, they just guarantee that the application will not remain 00362 stuck if the I2C communication is corrupted. 00363 You may modify these timeout values depending on CPU frequency and application 00364 conditions (interrupts routines ...). */ 00365 #define EVAL_I2Cx_TIMEOUT_MAX 3000 00366 00367 /*##################### SPI3 ###################################*/ 00368 #define EVAL_SPIx SPI3 00369 #define EVAL_SPIx_CLK_ENABLE() __HAL_RCC_SPI3_CLK_ENABLE() 00370 00371 #define EVAL_SPIx_SCK_GPIO_PORT GPIOC /* PC.10*/ 00372 #define EVAL_SPIx_SCK_PIN GPIO_PIN_10 00373 #define EVAL_SPIx_SCK_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00374 #define EVAL_SPIx_SCK_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00375 00376 #define EVAL_SPIx_MISO_MOSI_GPIO_PORT GPIOC 00377 #define EVAL_SPIx_MISO_MOSI_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00378 #define EVAL_SPIx_MISO_MOSI_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00379 #define EVAL_SPIx_MISO_PIN GPIO_PIN_11 /* PC.11*/ 00380 #define EVAL_SPIx_MOSI_PIN GPIO_PIN_12 /* PC.12*/ 00381 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00382 on accurate values, they just guarantee that the application will not remain 00383 stuck if the SPI communication is corrupted. 00384 You may modify these timeout values depending on CPU frequency and application 00385 conditions (interrupts routines ...). */ 00386 #define EVAL_SPIx_TIMEOUT_MAX 1000 00387 00388 /** 00389 * @} 00390 */ 00391 00392 /** @addtogroup STM3210C_EVAL_COMPONENT 00393 * @{ 00394 */ 00395 00396 /*##################### LCD ###################################*/ 00397 /* Chip Select macro definition */ 00398 #define LCD_CS_LOW() HAL_GPIO_WritePin(LCD_NCS_GPIO_PORT, LCD_NCS_PIN, GPIO_PIN_RESET) 00399 #define LCD_CS_HIGH() HAL_GPIO_WritePin(LCD_NCS_GPIO_PORT, LCD_NCS_PIN, GPIO_PIN_SET) 00400 00401 /** 00402 * @brief LCD Control Interface pins 00403 */ 00404 #define LCD_NCS_PIN GPIO_PIN_2 /* PB.02*/ 00405 #define LCD_NCS_GPIO_PORT GPIOB 00406 #define LCD_NCS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00407 #define LCD_NCS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00408 00409 /*##################### SD ###################################*/ 00410 /* Chip Select macro definition */ 00411 #define SD_CS_LOW() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_RESET) 00412 #define SD_CS_HIGH() HAL_GPIO_WritePin(SD_CS_GPIO_PORT, SD_CS_PIN, GPIO_PIN_SET) 00413 00414 /** 00415 * @brief SD Control Interface pins 00416 */ 00417 #define SD_CS_PIN GPIO_PIN_4 /* PA.04*/ 00418 #define SD_CS_GPIO_PORT GPIOA 00419 #define SD_CS_GPIO_CLK_ENABLE() __HAL_RCC_GPIOA_CLK_ENABLE() 00420 #define SD_CS_GPIO_CLK_DISABLE() __HAL_RCC_GPIOA_CLK_DISABLE() 00421 00422 /** 00423 * @brief SD Detect Interface pins 00424 */ 00425 #define SD_DETECT_PIN GPIO_PIN_0 00426 #define SD_DETECT_GPIO_PORT GPIOE 00427 #define SD_DETECT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOE_CLK_ENABLE() 00428 #define SD_DETECT_GPIO_CLK_DISABLE() __HAL_RCC_GPIOE_CLK_DISABLE() 00429 #define SD_DETECT_EXTI_IRQn EXTI0_IRQn 00430 00431 /*##################### AUDIO ##########################*/ 00432 /** 00433 * @brief AUDIO I2C Interface pins 00434 */ 00435 #define AUDIO_I2C_ADDRESS 0x94 00436 00437 /** 00438 * @} 00439 */ 00440 00441 /** 00442 * @} 00443 */ 00444 00445 00446 00447 /** @addtogroup STM3210C_EVAL_Exported_Functions 00448 * @{ 00449 */ 00450 uint32_t BSP_GetVersion(void); 00451 void BSP_LED_Init(Led_TypeDef Led); 00452 void BSP_LED_On(Led_TypeDef Led); 00453 void BSP_LED_Off(Led_TypeDef Led); 00454 void BSP_LED_Toggle(Led_TypeDef Led); 00455 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode); 00456 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00457 #ifdef HAL_UART_MODULE_ENABLED 00458 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart); 00459 #endif /* HAL_UART_MODULE_ENABLED */ 00460 #ifdef HAL_I2C_MODULE_ENABLED 00461 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode); 00462 JOYState_TypeDef BSP_JOY_GetState(void); 00463 #endif /* HAL_I2C_MODULE_ENABLED */ 00464 00465 /** 00466 * @} 00467 */ 00468 00469 00470 #ifdef __cplusplus 00471 } 00472 #endif 00473 00474 #endif /* __STM3210C_EVAL_H */ 00475 00476 /** 00477 * @} 00478 */ 00479 00480 /** 00481 * @} 00482 */ 00483 00484 /** 00485 * @} 00486 */ 00487 00488 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 11 2014 15:38:28 for _BSP_User_Manual by
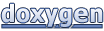