_BSP_User_Manual
|
stm3210c_eval.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm3210c_eval.c 00004 * @author MCD Application Team 00005 * @version $VERSION$ 00006 * @date $DATE$ 00007 * @brief This file provides a set of firmware functions to manage Leds, 00008 * push-button and COM ports for STM3210C_EVAL 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm3210c_eval.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @defgroup STM3210C_EVAL STM3210C-EVAL 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM3210C_EVAL_COMMON STM3210C-EVAL Common 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM3210C_EVAL_Private_TypesDefinitions Private Types Definitions 00055 * @{ 00056 */ 00057 00058 typedef struct 00059 { 00060 __IO uint16_t LCD_REG_R; /* Read Register */ 00061 __IO uint16_t LCD_RAM_R; /* Read RAM */ 00062 __IO uint16_t LCD_REG_W; /* Write Register */ 00063 __IO uint16_t LCD_RAM_W; /* Write RAM */ 00064 } TFT_LCD_TypeDef; 00065 00066 /** 00067 * @} 00068 */ 00069 00070 /** @defgroup STM3210C_EVAL_Private_Defines Private Defines 00071 * @{ 00072 */ 00073 00074 /* LINK LCD */ 00075 #define START_BYTE 0x70 00076 #define SET_INDEX 0x00 00077 #define READ_STATUS 0x01 00078 #define LCD_WRITE_REG 0x02 00079 #define LCD_READ_REG 0x03 00080 00081 /* LINK SD Card */ 00082 #define SD_DUMMY_BYTE 0xFF 00083 #define SD_NO_RESPONSE_EXPECTED 0x80 00084 00085 /** 00086 * @brief STM3210C EVAL BSP Driver version number V2.0.0 00087 */ 00088 #define __STM3210C_EVAL_BSP_VERSION_MAIN (0x02) /*!< [31:24] main version */ 00089 #define __STM3210C_EVAL_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00090 #define __STM3210C_EVAL_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00091 #define __STM3210C_EVAL_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00092 #define __STM3210C_EVAL_BSP_VERSION ((__STM3210C_EVAL_BSP_VERSION_MAIN << 24)\ 00093 |(__STM3210C_EVAL_BSP_VERSION_SUB1 << 16)\ 00094 |(__STM3210C_EVAL_BSP_VERSION_SUB2 << 8 )\ 00095 |(__STM3210C_EVAL_BSP_VERSION_RC)) 00096 00097 00098 /* Note: LCD /CS is CE4 - Bank 4 of NOR/SRAM Bank 1~4 */ 00099 #define TFT_LCD_BASE ((uint32_t)(0x60000000 | 0x0C000000)) 00100 #define TFT_LCD ((TFT_LCD_TypeDef *) TFT_LCD_BASE) 00101 00102 /** 00103 * @} 00104 */ 00105 00106 00107 /** @defgroup STM3210C_EVAL_Private_Variables Private Variables 00108 * @{ 00109 */ 00110 /** 00111 * @brief LED variables 00112 */ 00113 GPIO_TypeDef* LED_PORT[LEDn] = {LED1_GPIO_PORT, 00114 LED2_GPIO_PORT, 00115 LED3_GPIO_PORT, 00116 LED4_GPIO_PORT}; 00117 00118 const uint16_t LED_PIN[LEDn] = {LED1_PIN, 00119 LED2_PIN, 00120 LED3_PIN, 00121 LED4_PIN}; 00122 00123 /** 00124 * @brief BUTTON variables 00125 */ 00126 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {WAKEUP_BUTTON_GPIO_PORT, 00127 TAMPER_BUTTON_GPIO_PORT, 00128 KEY_BUTTON_GPIO_PORT}; 00129 00130 const uint16_t BUTTON_PIN[BUTTONn] = {WAKEUP_BUTTON_PIN, 00131 TAMPER_BUTTON_PIN, 00132 KEY_BUTTON_PIN}; 00133 00134 const uint16_t BUTTON_IRQn[BUTTONn] = {WAKEUP_BUTTON_EXTI_IRQn, 00135 TAMPER_BUTTON_EXTI_IRQn, 00136 KEY_BUTTON_EXTI_IRQn}; 00137 00138 00139 /** 00140 * @brief COM variables 00141 */ 00142 USART_TypeDef* COM_USART[COMn] = {EVAL_COM1}; 00143 00144 GPIO_TypeDef* COM_TX_PORT[COMn] = {EVAL_COM1_TX_GPIO_PORT}; 00145 00146 GPIO_TypeDef* COM_RX_PORT[COMn] = {EVAL_COM1_RX_GPIO_PORT}; 00147 00148 const uint16_t COM_TX_PIN[COMn] = {EVAL_COM1_TX_PIN}; 00149 00150 const uint16_t COM_RX_PIN[COMn] = {EVAL_COM1_RX_PIN}; 00151 00152 /** 00153 * @brief BUS variables 00154 */ 00155 #ifdef HAL_SPI_MODULE_ENABLED 00156 uint32_t SpixTimeout = EVAL_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00157 static SPI_HandleTypeDef heval_Spi; 00158 #endif /* HAL_SPI_MODULE_ENABLED */ 00159 00160 #ifdef HAL_I2C_MODULE_ENABLED 00161 uint32_t I2cxTimeout = EVAL_I2Cx_TIMEOUT_MAX; /*<! Value of Timeout when I2C communication fails */ 00162 I2C_HandleTypeDef heval_I2c; 00163 #endif /* HAL_I2C_MODULE_ENABLED */ 00164 00165 /** 00166 * @} 00167 */ 00168 00169 /* I2Cx bus function */ 00170 #ifdef HAL_I2C_MODULE_ENABLED 00171 /* Link function for I2C EEPROM peripheral */ 00172 static void I2Cx_Init(void); 00173 static void I2Cx_ITConfig(void); 00174 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length); 00175 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00176 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value); 00177 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00178 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg); 00179 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00180 static void I2Cx_Error(uint8_t Addr); 00181 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c); 00182 00183 /* Link function for IO Expander over I2C */ 00184 void IOE_Init(void); 00185 void IOE_ITConfig(void); 00186 void IOE_Delay(uint32_t Delay); 00187 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00188 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg); 00189 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00190 00191 /* Link function for EEPROM peripheral over I2C */ 00192 void EEPROM_I2C_IO_Init(void); 00193 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00194 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00195 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00196 00197 /* Link functions for Temperature Sensor peripheral */ 00198 void TSENSOR_IO_Init(void); 00199 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length); 00200 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length); 00201 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00202 00203 /* Link function for Audio peripheral */ 00204 void AUDIO_IO_Init(void); 00205 void AUDIO_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00206 uint8_t AUDIO_IO_Read(uint8_t Addr, uint8_t Reg); 00207 00208 /* Link function for Accelero peripheral */ 00209 void ACCELERO_IO_Init(void); 00210 void ACCELERO_IO_ITConfig(void); 00211 void ACCELERO_IO_Write(uint8_t* pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite); 00212 void ACCELERO_IO_Read(uint8_t* pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead); 00213 00214 #endif /* HAL_I2C_MODULE_ENABLED */ 00215 00216 /* SPIx bus function */ 00217 #ifdef HAL_SPI_MODULE_ENABLED 00218 static void SPIx_Init(void); 00219 static void SPIx_Write(uint8_t Value); 00220 static uint32_t SPIx_Read(void); 00221 static void SPIx_Error (void); 00222 static void SPIx_MspInit(SPI_HandleTypeDef *hspi); 00223 00224 /* Link function for LCD peripheral over SPI */ 00225 void LCD_IO_Init(void); 00226 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00227 void LCD_IO_WriteReg(uint8_t Reg); 00228 uint16_t LCD_IO_ReadData(uint16_t RegValue); 00229 void LCD_Delay (uint32_t delay); 00230 00231 /* Link functions for SD Card peripheral over SPI */ 00232 void SD_IO_Init(void); 00233 HAL_StatusTypeDef SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response); 00234 HAL_StatusTypeDef SD_IO_WaitResponse(uint8_t Response); 00235 void SD_IO_WriteDummy(void); 00236 void SD_IO_WriteByte(uint8_t Data); 00237 uint8_t SD_IO_ReadByte(void); 00238 00239 #endif /* HAL_SPI_MODULE_ENABLED */ 00240 00241 00242 /** @defgroup STM3210C_EVAL_Exported_Functions Exported Functions 00243 * @{ 00244 */ 00245 00246 /** 00247 * @brief This method returns the STM3210C EVAL BSP Driver revision 00248 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00249 */ 00250 uint32_t BSP_GetVersion(void) 00251 { 00252 return __STM3210C_EVAL_BSP_VERSION; 00253 } 00254 00255 /** 00256 * @brief Configures LED GPIO. 00257 * @param Led: Specifies the Led to be configured. 00258 * This parameter can be one of following parameters: 00259 * @arg LED1 00260 * @arg LED2 00261 * @arg LED3 00262 * @arg LED4 00263 * @retval None 00264 */ 00265 void BSP_LED_Init(Led_TypeDef Led) 00266 { 00267 GPIO_InitTypeDef gpioinitstruct = {0}; 00268 00269 /* Enable the GPIO_LED clock */ 00270 LEDx_GPIO_CLK_ENABLE(Led); 00271 00272 /* Configure the GPIO_LED pin */ 00273 gpioinitstruct.Pin = LED_PIN[Led]; 00274 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00275 gpioinitstruct.Pull = GPIO_NOPULL; 00276 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00277 00278 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00279 00280 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00281 } 00282 00283 /** 00284 * @brief Turns selected LED On. 00285 * @param Led: Specifies the Led to be set on. 00286 * This parameter can be one of following parameters: 00287 * @arg LED1 00288 * @arg LED2 00289 * @arg LED3 00290 * @arg LED4 00291 * @retval None 00292 */ 00293 void BSP_LED_On(Led_TypeDef Led) 00294 { 00295 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00296 } 00297 00298 /** 00299 * @brief Turns selected LED Off. 00300 * @param Led: Specifies the Led to be set off. 00301 * This parameter can be one of following parameters: 00302 * @arg LED1 00303 * @arg LED2 00304 * @arg LED3 00305 * @arg LED4 00306 * @retval None 00307 */ 00308 void BSP_LED_Off(Led_TypeDef Led) 00309 { 00310 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00311 } 00312 00313 /** 00314 * @brief Toggles the selected LED. 00315 * @param Led: Specifies the Led to be toggled. 00316 * This parameter can be one of following parameters: 00317 * @arg LED1 00318 * @arg LED2 00319 * @arg LED3 00320 * @arg LED4 00321 * @retval None 00322 */ 00323 void BSP_LED_Toggle(Led_TypeDef Led) 00324 { 00325 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00326 } 00327 00328 /** 00329 * @brief Configures push button GPIO and EXTI Line. 00330 * @param Button: Button to be configured. 00331 * This parameter can be one of the following values: 00332 * @arg BUTTON_WAKEUP: Wakeup Push Button 00333 * @arg BUTTON_TAMPER: Tamper Push Button 00334 * @arg BUTTON_KEY: Key Push Button 00335 * @param Button_Mode: Button mode requested. 00336 * This parameter can be one of the following values: 00337 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00338 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00339 * with interrupt generation capability 00340 * @retval None 00341 */ 00342 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef Button_Mode) 00343 { 00344 GPIO_InitTypeDef gpioinitstruct = {0}; 00345 00346 /* Enable the corresponding Push Button clock */ 00347 BUTTONx_GPIO_CLK_ENABLE(Button); 00348 00349 /* Configure Push Button pin as input */ 00350 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00351 gpioinitstruct.Pull = GPIO_NOPULL; 00352 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00353 00354 if (Button_Mode == BUTTON_MODE_GPIO) 00355 { 00356 /* Configure Button pin as input */ 00357 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00358 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00359 } 00360 else if (Button_Mode == BUTTON_MODE_EXTI) 00361 { 00362 if(Button != BUTTON_WAKEUP) 00363 { 00364 /* Configure Joystick Button pin as input with External interrupt, falling edge */ 00365 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00366 } 00367 else 00368 { 00369 /* Configure Key Push Button pin as input with External interrupt, rising edge */ 00370 gpioinitstruct.Mode = GPIO_MODE_IT_RISING; 00371 } 00372 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00373 00374 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00375 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00376 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00377 } 00378 } 00379 00380 /** 00381 * @brief Returns the selected button state. 00382 * @param Button: Button to be checked. 00383 * This parameter can be one of the following values: 00384 * @arg BUTTON_TAMPER: Key/Tamper Push Button 00385 * @retval Button state 00386 */ 00387 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00388 { 00389 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00390 } 00391 00392 #ifdef HAL_I2C_MODULE_ENABLED 00393 /** 00394 * @brief Configures joystick GPIO and EXTI modes. 00395 * @param Joy_Mode: Button mode. 00396 * This parameter can be one of the following values: 00397 * @arg JOY_MODE_GPIO: Joystick pins will be used as simple IOs 00398 * @arg JOY_MODE_EXTI: Joystick pins will be connected to EXTI line 00399 * with interrupt generation capability 00400 * @retval IO_OK: if all initializations are OK. Other value if error. 00401 */ 00402 uint8_t BSP_JOY_Init(JOYMode_TypeDef Joy_Mode) 00403 { 00404 uint8_t ret = 0; 00405 00406 /* Initialize the IO functionalities */ 00407 ret = BSP_IO_Init(); 00408 00409 /* Configure joystick pins in IT mode */ 00410 if((ret == IO_OK) && (Joy_Mode == JOY_MODE_EXTI)) 00411 { 00412 /* Configure joystick pins in IT mode */ 00413 BSP_IO_ConfigPin(JOY_ALL_PINS, IO_MODE_IT_FALLING_EDGE); 00414 } 00415 00416 return ret; 00417 } 00418 00419 /** 00420 * @brief Returns the current joystick status. 00421 * @retval Code of the joystick key pressed 00422 * This code can be one of the following values: 00423 * @arg JOY_NONE 00424 * @arg JOY_SEL 00425 * @arg JOY_DOWN 00426 * @arg JOY_LEFT 00427 * @arg JOY_RIGHT 00428 * @arg JOY_UP 00429 */ 00430 JOYState_TypeDef BSP_JOY_GetState(void) 00431 { 00432 uint32_t tmp = 0; 00433 00434 /* Read the status joystick pins */ 00435 tmp = BSP_IO_ReadPin(JOY_ALL_PINS); 00436 00437 /* Check the pressed keys */ 00438 if((tmp & JOY_NONE_PIN) == JOY_NONE) 00439 { 00440 return(JOYState_TypeDef) JOY_NONE; 00441 } 00442 else if(!(tmp & JOY_SEL_PIN)) 00443 { 00444 return(JOYState_TypeDef) JOY_SEL; 00445 } 00446 else if(!(tmp & JOY_DOWN_PIN)) 00447 { 00448 return(JOYState_TypeDef) JOY_DOWN; 00449 } 00450 else if(!(tmp & JOY_LEFT_PIN)) 00451 { 00452 return(JOYState_TypeDef) JOY_LEFT; 00453 } 00454 else if(!(tmp & JOY_RIGHT_PIN)) 00455 { 00456 return(JOYState_TypeDef) JOY_RIGHT; 00457 } 00458 else if(!(tmp & JOY_UP_PIN)) 00459 { 00460 return(JOYState_TypeDef) JOY_UP; 00461 } 00462 else 00463 { 00464 return(JOYState_TypeDef) JOY_NONE; 00465 } 00466 } 00467 #endif /*HAL_I2C_MODULE_ENABLED*/ 00468 00469 #ifdef HAL_UART_MODULE_ENABLED 00470 /** 00471 * @brief Configures COM port. 00472 * @param COM: Specifies the COM port to be configured. 00473 * This parameter can be one of following parameters: 00474 * @arg COM1 00475 * @param huart: pointer to a UART_HandleTypeDef structure that 00476 * contains the configuration information for the specified UART peripheral. 00477 * @retval None 00478 */ 00479 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef* huart) 00480 { 00481 GPIO_InitTypeDef gpioinitstruct = {0}; 00482 00483 /* Enable GPIO clock */ 00484 COMx_TX_GPIO_CLK_ENABLE(COM); 00485 COMx_RX_GPIO_CLK_ENABLE(COM); 00486 00487 /* Enable USART clock */ 00488 COMx_CLK_ENABLE(COM); 00489 00490 /* Remap AFIO if needed */ 00491 AFIOCOMx_CLK_ENABLE(COM); 00492 AFIOCOMx_REMAP(COM); 00493 00494 /* Configure USART Tx as alternate function push-pull */ 00495 gpioinitstruct.Pin = COM_TX_PIN[COM]; 00496 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00497 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00498 gpioinitstruct.Pull = GPIO_PULLUP; 00499 HAL_GPIO_Init(COM_TX_PORT[COM], &gpioinitstruct); 00500 00501 /* Configure USART Rx as alternate function push-pull */ 00502 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00503 gpioinitstruct.Pin = COM_RX_PIN[COM]; 00504 HAL_GPIO_Init(COM_RX_PORT[COM], &gpioinitstruct); 00505 00506 /* USART configuration */ 00507 huart->Instance = COM_USART[COM]; 00508 HAL_UART_Init(huart); 00509 } 00510 #endif /* HAL_UART_MODULE_ENABLED */ 00511 00512 /** 00513 * @} 00514 */ 00515 00516 /** @defgroup STM3210C_EVAL_BusOperations_Functions Bus Operations Functions 00517 * @{ 00518 */ 00519 00520 /******************************************************************************* 00521 BUS OPERATIONS 00522 *******************************************************************************/ 00523 00524 #ifdef HAL_I2C_MODULE_ENABLED 00525 /******************************* I2C Routines**********************************/ 00526 00527 /** 00528 * @brief Eval I2Cx MSP Initialization 00529 * @param hi2c: I2C handle 00530 * @retval None 00531 */ 00532 static void I2Cx_MspInit(I2C_HandleTypeDef *hi2c) 00533 { 00534 GPIO_InitTypeDef gpioinitstruct = {0}; 00535 00536 if (hi2c->Instance == EVAL_I2Cx) 00537 { 00538 /*## Configure the GPIOs ################################################*/ 00539 00540 /* Enable GPIO clock */ 00541 EVAL_I2Cx_SDA_GPIO_CLK_ENABLE(); 00542 EVAL_I2Cx_SCL_GPIO_CLK_ENABLE(); 00543 00544 /* Configure I2C Tx as alternate function */ 00545 gpioinitstruct.Pin = EVAL_I2Cx_SCL_PIN; 00546 gpioinitstruct.Mode = GPIO_MODE_AF_OD; 00547 gpioinitstruct.Pull = GPIO_NOPULL; 00548 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00549 HAL_GPIO_Init(EVAL_I2Cx_SCL_GPIO_PORT, &gpioinitstruct); 00550 00551 /* Configure I2C Rx as alternate function */ 00552 gpioinitstruct.Pin = EVAL_I2Cx_SDA_PIN; 00553 HAL_GPIO_Init(EVAL_I2Cx_SDA_GPIO_PORT, &gpioinitstruct); 00554 00555 /*## Configure the Eval I2Cx peripheral #######################################*/ 00556 /* Enable Eval_I2Cx clock */ 00557 EVAL_I2Cx_CLK_ENABLE(); 00558 00559 /* Add delay related to RCC workaround */ 00560 while (READ_BIT(RCC->APB1ENR, RCC_APB1ENR_I2C1EN) != RCC_APB1ENR_I2C1EN) {}; 00561 00562 /* Force the I2C Periheral Clock Reset */ 00563 EVAL_I2Cx_FORCE_RESET(); 00564 00565 /* Release the I2C Periheral Clock Reset */ 00566 EVAL_I2Cx_RELEASE_RESET(); 00567 00568 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00569 HAL_NVIC_SetPriority(EVAL_I2Cx_EV_IRQn, 5, 0); 00570 HAL_NVIC_EnableIRQ(EVAL_I2Cx_EV_IRQn); 00571 00572 /* Enable and set Eval I2Cx Interrupt to the highest priority */ 00573 HAL_NVIC_SetPriority(EVAL_I2Cx_ER_IRQn, 5, 0); 00574 HAL_NVIC_EnableIRQ(EVAL_I2Cx_ER_IRQn); 00575 } 00576 } 00577 00578 /** 00579 * @brief Eval I2Cx Bus initialization 00580 * @retval None 00581 */ 00582 static void I2Cx_Init(void) 00583 { 00584 if(HAL_I2C_GetState(&heval_I2c) == HAL_I2C_STATE_RESET) 00585 { 00586 heval_I2c.Instance = EVAL_I2Cx; 00587 heval_I2c.Init.ClockSpeed = BSP_I2C_SPEED; 00588 heval_I2c.Init.DutyCycle = I2C_DUTYCYCLE_2; 00589 heval_I2c.Init.OwnAddress1 = 0; 00590 heval_I2c.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00591 heval_I2c.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00592 heval_I2c.Init.OwnAddress2 = 0; 00593 heval_I2c.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00594 heval_I2c.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00595 00596 /* Init the I2C */ 00597 I2Cx_MspInit(&heval_I2c); 00598 HAL_I2C_Init(&heval_I2c); 00599 } 00600 } 00601 00602 /** 00603 * @brief Configures I2C Interrupt. 00604 * @retval None 00605 */ 00606 static void I2Cx_ITConfig(void) 00607 { 00608 static uint8_t I2C_IT_Enabled = 0; 00609 GPIO_InitTypeDef gpioinitstruct = {0}; 00610 00611 if(I2C_IT_Enabled == 0) 00612 { 00613 I2C_IT_Enabled = 1; 00614 00615 /* Enable the GPIO EXTI clock */ 00616 IOE_IT_GPIO_CLK_ENABLE(); 00617 00618 gpioinitstruct.Pin = IOE_IT_PIN; 00619 gpioinitstruct.Pull = GPIO_NOPULL; 00620 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00621 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00622 HAL_GPIO_Init(IOE_IT_GPIO_PORT, &gpioinitstruct); 00623 00624 /* Set priority and Enable GPIO EXTI Interrupt */ 00625 HAL_NVIC_SetPriority((IRQn_Type)(IOE_IT_EXTI_IRQn), 5, 0); 00626 HAL_NVIC_EnableIRQ((IRQn_Type)(IOE_IT_EXTI_IRQn)); 00627 } 00628 } 00629 00630 /** 00631 * @brief Reads multiple data. 00632 * @param Addr: I2C address 00633 * @param Reg: Reg address 00634 * @param MemAddress: Internal memory address 00635 * @param Buffer: Pointer to data buffer 00636 * @param Length: Length of the data 00637 * @retval Number of read data 00638 */ 00639 static HAL_StatusTypeDef I2Cx_ReadMultiple(uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00640 { 00641 HAL_StatusTypeDef status = HAL_OK; 00642 00643 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, I2cxTimeout); 00644 00645 /* Check the communication status */ 00646 if(status != HAL_OK) 00647 { 00648 /* I2C error occured */ 00649 I2Cx_Error(Addr); 00650 } 00651 return status; 00652 } 00653 00654 /** 00655 * @brief Write a value in a register of the device through BUS. 00656 * @param Addr: Device address on BUS Bus. 00657 * @param Reg: The target register address to write 00658 * @param Value: The target register value to be written 00659 * @retval None 00660 */ 00661 static void I2Cx_WriteData(uint16_t Addr, uint8_t Reg, uint8_t Value) 00662 { 00663 HAL_StatusTypeDef status = HAL_OK; 00664 00665 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, I2cxTimeout); 00666 00667 /* Check the communication status */ 00668 if(status != HAL_OK) 00669 { 00670 /* Execute user timeout callback */ 00671 I2Cx_Error(Addr); 00672 } 00673 } 00674 00675 /** 00676 * @brief Write a value in a register of the device through BUS. 00677 * @param Addr: Device address on BUS Bus. 00678 * @param Reg: The target register address to write 00679 * @param RegSize: The target register size (can be 8BIT or 16BIT) 00680 * @param pBuffer: The target register value to be written 00681 * @param Length: buffer size to be written 00682 * @retval None 00683 */ 00684 static HAL_StatusTypeDef I2Cx_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00685 { 00686 HAL_StatusTypeDef status = HAL_OK; 00687 00688 status = HAL_I2C_Mem_Write(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00689 00690 /* Check the communication status */ 00691 if(status != HAL_OK) 00692 { 00693 /* Re-Initiaize the BUS */ 00694 I2Cx_Error(Addr); 00695 } 00696 return status; 00697 } 00698 00699 /** 00700 * @brief Read a value in a register of the device through BUS. 00701 * @param Addr: Device address on BUS Bus. 00702 * @param Reg: The target register address to write 00703 * @retval Data read at register @ 00704 */ 00705 static uint8_t I2Cx_ReadData(uint16_t Addr, uint8_t Reg) 00706 { 00707 HAL_StatusTypeDef status = HAL_OK; 00708 uint8_t value = 0; 00709 00710 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &value, 1, I2cxTimeout); 00711 00712 /* Check the communication status */ 00713 if(status != HAL_OK) 00714 { 00715 /* Execute user timeout callback */ 00716 I2Cx_Error(Addr); 00717 00718 } 00719 return value; 00720 } 00721 00722 /** 00723 * @brief Reads multiple data on the BUS. 00724 * @param Addr: I2C Address 00725 * @param Reg: Reg Address 00726 * @param RegSize : The target register size (can be 8BIT or 16BIT) 00727 * @param pBuffer: pointer to read data buffer 00728 * @param Length: length of the data 00729 * @retval 0 if no problems to read multiple data 00730 */ 00731 static HAL_StatusTypeDef I2Cx_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00732 { 00733 HAL_StatusTypeDef status = HAL_OK; 00734 00735 status = HAL_I2C_Mem_Read(&heval_I2c, Addr, (uint16_t)Reg, RegSize, pBuffer, Length, I2cxTimeout); 00736 00737 /* Check the communication status */ 00738 if(status != HAL_OK) 00739 { 00740 /* Re-Initiaize the BUS */ 00741 I2Cx_Error(Addr); 00742 } 00743 return status; 00744 } 00745 00746 /** 00747 * @brief Checks if target device is ready for communication. 00748 * @note This function is used with Memory devices 00749 * @param DevAddress: Target device address 00750 * @param Trials: Number of trials 00751 * @retval HAL status 00752 */ 00753 static HAL_StatusTypeDef I2Cx_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00754 { 00755 return (HAL_I2C_IsDeviceReady(&heval_I2c, DevAddress, Trials, I2cxTimeout)); 00756 } 00757 00758 /** 00759 * @brief Manages error callback by re-initializing I2C. 00760 * @param Addr: I2C Address 00761 * @retval None 00762 */ 00763 static void I2Cx_Error(uint8_t Addr) 00764 { 00765 /* De-initialize the IOE comunication BUS */ 00766 HAL_I2C_DeInit(&heval_I2c); 00767 00768 /* Re-Initiaize the IOE comunication BUS */ 00769 I2Cx_Init(); 00770 } 00771 00772 #endif /* HAL_I2C_MODULE_ENABLED */ 00773 00774 /******************************* SPI Routines**********************************/ 00775 #ifdef HAL_SPI_MODULE_ENABLED 00776 /** 00777 * @brief Initializes SPI MSP. 00778 * @retval None 00779 */ 00780 static void SPIx_MspInit(SPI_HandleTypeDef *hspi) 00781 { 00782 GPIO_InitTypeDef gpioinitstruct = {0}; 00783 00784 /*** Configure the GPIOs ***/ 00785 /* Enable GPIO clock */ 00786 EVAL_SPIx_SCK_GPIO_CLK_ENABLE(); 00787 EVAL_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00788 __HAL_RCC_AFIO_CLK_ENABLE(); 00789 __HAL_AFIO_REMAP_SPI3_ENABLE(); 00790 00791 /* configure SPI SCK */ 00792 gpioinitstruct.Pin = EVAL_SPIx_SCK_PIN; 00793 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00794 gpioinitstruct.Pull = GPIO_NOPULL; 00795 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00796 HAL_GPIO_Init(EVAL_SPIx_SCK_GPIO_PORT, &gpioinitstruct); 00797 00798 /* configure SPI MISO and MOSI */ 00799 gpioinitstruct.Pin = (EVAL_SPIx_MISO_PIN | EVAL_SPIx_MOSI_PIN); 00800 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00801 gpioinitstruct.Pull = GPIO_NOPULL; 00802 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00803 HAL_GPIO_Init(EVAL_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00804 00805 /*** Configure the SPI peripheral ***/ 00806 /* Enable SPI clock */ 00807 EVAL_SPIx_CLK_ENABLE(); 00808 } 00809 00810 /** 00811 * @brief Initializes SPI HAL. 00812 * @retval None 00813 */ 00814 static void SPIx_Init(void) 00815 { 00816 /* DeInitializes the SPI peripheral */ 00817 heval_Spi.Instance = EVAL_SPIx; 00818 HAL_SPI_DeInit(&heval_Spi); 00819 00820 /* SPI Config */ 00821 /* SPI baudrate is set to 9 MHz (PCLK2/SPI_BaudRatePrescaler = 72/8 = 9 MHz) */ 00822 heval_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_8; 00823 heval_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00824 heval_Spi.Init.CLKPhase = SPI_PHASE_2EDGE; 00825 heval_Spi.Init.CLKPolarity = SPI_POLARITY_HIGH; 00826 heval_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00827 heval_Spi.Init.CRCPolynomial = 7; 00828 heval_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00829 heval_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00830 heval_Spi.Init.NSS = SPI_NSS_SOFT; 00831 heval_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00832 heval_Spi.Init.Mode = SPI_MODE_MASTER; 00833 00834 SPIx_MspInit(&heval_Spi); 00835 if (HAL_SPI_Init(&heval_Spi) != HAL_OK) 00836 { 00837 /* Should not occur */ 00838 while(1) {}; 00839 } 00840 } 00841 00842 /** 00843 * @brief SPI Read 4 bytes from device 00844 * @retval Read data 00845 */ 00846 static uint32_t SPIx_Read(void) 00847 { 00848 HAL_StatusTypeDef status = HAL_OK; 00849 uint32_t readvalue = 0; 00850 uint32_t writevalue = 0xFFFFFFFF; 00851 00852 status = HAL_SPI_TransmitReceive(&heval_Spi, (uint8_t*) &writevalue, (uint8_t*) &readvalue, 1, SpixTimeout); 00853 00854 /* Check the communication status */ 00855 if(status != HAL_OK) 00856 { 00857 /* Execute user timeout callback */ 00858 SPIx_Error(); 00859 } 00860 00861 return readvalue; 00862 } 00863 00864 /** 00865 * @brief SPI Write a byte to device 00866 * @param Value: value to be written 00867 * @retval None 00868 */ 00869 static void SPIx_Write(uint8_t Value) 00870 { 00871 HAL_StatusTypeDef status = HAL_OK; 00872 00873 status = HAL_SPI_Transmit(&heval_Spi, (uint8_t*) &Value, 1, SpixTimeout); 00874 00875 /* Check the communication status */ 00876 if(status != HAL_OK) 00877 { 00878 /* Execute user timeout callback */ 00879 SPIx_Error(); 00880 } 00881 } 00882 00883 /** 00884 * @brief SPI error treatment function 00885 * @retval None 00886 */ 00887 static void SPIx_Error (void) 00888 { 00889 /* De-initialize the SPI communication BUS */ 00890 HAL_SPI_DeInit(&heval_Spi); 00891 00892 /* Re- Initiaize the SPI communication BUS */ 00893 SPIx_Init(); 00894 } 00895 #endif /* HAL_SPI_MODULE_ENABLED */ 00896 00897 /** 00898 * @} 00899 */ 00900 00901 /** @defgroup STM3210C_EVAL_LinkOperations_Functions Link Operations Functions 00902 * @{ 00903 */ 00904 00905 /******************************************************************************* 00906 LINK OPERATIONS 00907 *******************************************************************************/ 00908 00909 #ifdef HAL_I2C_MODULE_ENABLED 00910 /***************************** LINK IOE ***************************************/ 00911 00912 /** 00913 * @brief Initializes IOE low level. 00914 * @retval None 00915 */ 00916 void IOE_Init(void) 00917 { 00918 I2Cx_Init(); 00919 } 00920 00921 /** 00922 * @brief Configures IOE low level Interrupt. 00923 * @retval None 00924 */ 00925 void IOE_ITConfig(void) 00926 { 00927 I2Cx_ITConfig(); 00928 } 00929 00930 /** 00931 * @brief IOE writes single data. 00932 * @param Addr: I2C address 00933 * @param Reg: Reg address 00934 * @param Value: Data to be written 00935 * @retval None 00936 */ 00937 void IOE_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00938 { 00939 I2Cx_WriteData(Addr, Reg, Value); 00940 } 00941 00942 /** 00943 * @brief IOE reads single data. 00944 * @param Addr: I2C address 00945 * @param Reg: Reg address 00946 * @retval Read data 00947 */ 00948 uint8_t IOE_Read(uint8_t Addr, uint8_t Reg) 00949 { 00950 return I2Cx_ReadData(Addr, Reg); 00951 } 00952 00953 /** 00954 * @brief IOE reads multiple data. 00955 * @param Addr: I2C address 00956 * @param Reg: Reg address 00957 * @param Buffer: Pointer to data buffer 00958 * @param Length: Length of the data 00959 * @retval Number of read data 00960 */ 00961 uint16_t IOE_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00962 { 00963 return I2Cx_ReadMultiple(Addr, Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00964 } 00965 00966 /** 00967 * @brief IOE delay. 00968 * @param Delay: Delay in ms 00969 * @retval None 00970 */ 00971 void IOE_Delay(uint32_t Delay) 00972 { 00973 HAL_Delay(Delay); 00974 } 00975 00976 #endif /* HAL_I2C_MODULE_ENABLED */ 00977 00978 #ifdef HAL_SPI_MODULE_ENABLED 00979 /********************************* LINK LCD ***********************************/ 00980 00981 /** 00982 * @brief Configures the LCD_SPI interface. 00983 * @retval None 00984 */ 00985 void LCD_IO_Init(void) 00986 { 00987 GPIO_InitTypeDef gpioinitstruct; 00988 00989 /* Configure the LCD Control pins ------------------------------------------*/ 00990 LCD_NCS_GPIO_CLK_ENABLE(); 00991 00992 /* Configure NCS in Output Push-Pull mode */ 00993 gpioinitstruct.Pin = LCD_NCS_PIN; 00994 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00995 gpioinitstruct.Pull = GPIO_NOPULL; 00996 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 00997 HAL_GPIO_Init(LCD_NCS_GPIO_PORT, &gpioinitstruct); 00998 00999 /* Set or Reset the control line */ 01000 LCD_CS_LOW(); 01001 LCD_CS_HIGH(); 01002 01003 SPIx_Init(); 01004 } 01005 01006 /** 01007 * @brief Write register value. 01008 * @param pData Pointer on the register value 01009 * @param Size Size of byte to transmit to the register 01010 * @retval None 01011 */ 01012 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 01013 { 01014 uint32_t counter = 0; 01015 01016 /* Reset LCD control line(/CS) and Send data */ 01017 LCD_CS_LOW(); 01018 01019 /* Send Start Byte */ 01020 SPIx_Write(START_BYTE | LCD_WRITE_REG); 01021 01022 for (counter = Size; counter != 0; counter--) 01023 { 01024 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01025 { 01026 } 01027 /* Need to invert bytes for LCD*/ 01028 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *(pData+1); 01029 01030 while(((heval_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 01031 { 01032 } 01033 *((__IO uint8_t*)&heval_Spi.Instance->DR) = *pData; 01034 counter--; 01035 pData += 2; 01036 } 01037 01038 /* Wait until the bus is ready before releasing Chip select */ 01039 while(((heval_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 01040 { 01041 } 01042 01043 /* Reset LCD control line(/CS) and Send data */ 01044 LCD_CS_HIGH(); 01045 } 01046 01047 /** 01048 * @brief register address. 01049 * @param Reg 01050 * @retval None 01051 */ 01052 void LCD_IO_WriteReg(uint8_t Reg) 01053 { 01054 /* Reset LCD control line(/CS) and Send command */ 01055 LCD_CS_LOW(); 01056 01057 /* Send Start Byte */ 01058 SPIx_Write(START_BYTE | SET_INDEX); 01059 01060 /* Write 16-bit Reg Index (High Byte is 0) */ 01061 SPIx_Write(0x00); 01062 SPIx_Write(Reg); 01063 01064 /* Deselect : Chip Select high */ 01065 LCD_CS_HIGH(); 01066 } 01067 01068 /** 01069 * @brief Read register value. 01070 * @param Reg 01071 * @retval None 01072 */ 01073 uint16_t LCD_IO_ReadData(uint16_t Reg) 01074 { 01075 uint32_t readvalue = 0; 01076 01077 /* Send Reg value to Read */ 01078 LCD_IO_WriteReg(Reg); 01079 01080 /* Reset LCD control line(/CS) and Send command */ 01081 LCD_CS_LOW(); 01082 01083 /* Send Start Byte */ 01084 SPIx_Write(START_BYTE | LCD_READ_REG); 01085 /* Read Upper Byte */ 01086 SPIx_Write(0xFF); 01087 readvalue = SPIx_Read(); 01088 readvalue = readvalue << 8; 01089 readvalue |= SPIx_Read(); 01090 01091 HAL_Delay(10); 01092 01093 /* Deselect : Chip Select high */ 01094 LCD_CS_HIGH(); 01095 return readvalue; 01096 } 01097 01098 /** 01099 * @brief Wait for loop in ms. 01100 * @param Delay in ms. 01101 * @retval None 01102 */ 01103 void LCD_Delay (uint32_t Delay) 01104 { 01105 HAL_Delay(Delay); 01106 } 01107 01108 /******************************** LINK SD Card ********************************/ 01109 01110 /** 01111 * @brief Initializes the SD Card and put it into StandBy State (Ready for 01112 * data transfer). 01113 * @retval None 01114 */ 01115 void SD_IO_Init(void) 01116 { 01117 GPIO_InitTypeDef gpioinitstruct; 01118 uint8_t counter; 01119 01120 /* SD_CS_GPIO and SD_DETECT_GPIO Periph clock enable */ 01121 SD_CS_GPIO_CLK_ENABLE(); 01122 SD_DETECT_GPIO_CLK_ENABLE(); 01123 01124 /* Configure SD_CS_PIN pin: SD Card CS pin */ 01125 gpioinitstruct.Pin = SD_CS_PIN; 01126 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 01127 gpioinitstruct.Pull = GPIO_PULLUP; 01128 gpioinitstruct.Speed = GPIO_SPEED_HIGH; 01129 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 01130 01131 /* Configure SD_DETECT_PIN pin: SD Card detect pin */ 01132 gpioinitstruct.Pin = SD_DETECT_PIN; 01133 gpioinitstruct.Mode = GPIO_MODE_IT_RISING_FALLING; 01134 gpioinitstruct.Pull = GPIO_PULLUP; 01135 HAL_GPIO_Init(SD_DETECT_GPIO_PORT, &gpioinitstruct); 01136 01137 /* Enable and set SD EXTI Interrupt to the lowest priority */ 01138 HAL_NVIC_SetPriority(SD_DETECT_EXTI_IRQn, 0x0F, 0); 01139 HAL_NVIC_EnableIRQ(SD_DETECT_EXTI_IRQn); 01140 01141 /*------------Put SD in SPI mode--------------*/ 01142 /* SD SPI Config */ 01143 SPIx_Init(); 01144 01145 /* SD chip select high */ 01146 SD_CS_HIGH(); 01147 01148 /* Send dummy byte 0xFF, 10 times with CS high */ 01149 /* Rise CS and MOSI for 80 clocks cycles */ 01150 for (counter = 0; counter <= 9; counter++) 01151 { 01152 /* Send dummy byte 0xFF */ 01153 SD_IO_WriteByte(SD_DUMMY_BYTE); 01154 } 01155 } 01156 01157 /** 01158 * @brief Write a byte on the SD. 01159 * @param Data: byte to send. 01160 * @retval None 01161 */ 01162 void SD_IO_WriteByte(uint8_t Data) 01163 { 01164 /* Send the byte */ 01165 SPIx_Write(Data); 01166 } 01167 01168 /** 01169 * @brief Read a byte from the SD. 01170 * @retval The received byte. 01171 */ 01172 uint8_t SD_IO_ReadByte(void) 01173 { 01174 uint8_t data = 0; 01175 01176 /* Get the received data */ 01177 data = SPIx_Read(); 01178 01179 /* Return the shifted data */ 01180 return data; 01181 } 01182 01183 /** 01184 * @brief Send 5 bytes command to the SD card and get response 01185 * @param Cmd: The user expected command to send to SD card. 01186 * @param Arg: The command argument. 01187 * @param Crc: The CRC. 01188 * @param Response: Expected response from the SD card 01189 * @retval HAL_StatusTypeDef HAL Status 01190 */ 01191 HAL_StatusTypeDef SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Response) 01192 { 01193 uint32_t counter = 0x00; 01194 uint8_t frame[6]; 01195 01196 /* Prepare Frame to send */ 01197 frame[0] = (Cmd | 0x40); /* Construct byte 1 */ 01198 frame[1] = (uint8_t)(Arg >> 24); /* Construct byte 2 */ 01199 frame[2] = (uint8_t)(Arg >> 16); /* Construct byte 3 */ 01200 frame[3] = (uint8_t)(Arg >> 8); /* Construct byte 4 */ 01201 frame[4] = (uint8_t)(Arg); /* Construct byte 5 */ 01202 frame[5] = (Crc); /* Construct CRC: byte 6 */ 01203 01204 /* SD chip select low */ 01205 SD_CS_LOW(); 01206 01207 /* Send Frame */ 01208 for (counter = 0; counter < 6; counter++) 01209 { 01210 SD_IO_WriteByte(frame[counter]); /* Send the Cmd bytes */ 01211 } 01212 01213 if(Response != SD_NO_RESPONSE_EXPECTED) 01214 { 01215 return SD_IO_WaitResponse(Response); 01216 } 01217 01218 return HAL_OK; 01219 } 01220 01221 /** 01222 * @brief Wait response from the SD card 01223 * @param Response: Expected response from the SD card 01224 * @retval HAL_StatusTypeDef HAL Status 01225 */ 01226 HAL_StatusTypeDef SD_IO_WaitResponse(uint8_t Response) 01227 { 01228 uint32_t timeout = 0xFFFF; 01229 uint8_t resp = 0; 01230 /* Check if response is got or a timeout is happen */ 01231 resp = SD_IO_ReadByte(); 01232 while ((resp != Response) && timeout) 01233 { 01234 timeout--; 01235 resp = SD_IO_ReadByte(); 01236 } 01237 01238 if (timeout == 0) 01239 { 01240 /* After time out */ 01241 return HAL_TIMEOUT; 01242 } 01243 else 01244 { 01245 /* Right response got */ 01246 return HAL_OK; 01247 } 01248 } 01249 01250 /** 01251 * @brief Send dummy byte with CS High 01252 * @retval None 01253 */ 01254 void SD_IO_WriteDummy(void) 01255 { 01256 /* SD chip select high */ 01257 SD_CS_HIGH(); 01258 01259 /* Send Dummy byte 0xFF */ 01260 SD_IO_WriteByte(SD_DUMMY_BYTE); 01261 } 01262 01263 #endif /* HAL_SPI_MODULE_ENABLED */ 01264 01265 #ifdef HAL_I2C_MODULE_ENABLED 01266 /********************************* LINK I2C EEPROM *****************************/ 01267 /** 01268 * @brief Initializes peripherals used by the I2C EEPROM driver. 01269 * @retval None 01270 */ 01271 void EEPROM_I2C_IO_Init(void) 01272 { 01273 I2Cx_Init(); 01274 } 01275 01276 /** 01277 * @brief Write data to I2C EEPROM driver 01278 * @param DevAddress: Target device address 01279 * @param MemAddress: Internal memory address 01280 * @param pBuffer: Pointer to data buffer 01281 * @param BufferSize: Amount of data to be sent 01282 * @retval HAL status 01283 */ 01284 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01285 { 01286 return (I2Cx_WriteBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01287 } 01288 01289 /** 01290 * @brief Read data from I2C EEPROM driver 01291 * @param DevAddress: Target device address 01292 * @param MemAddress: Internal memory address 01293 * @param pBuffer: Pointer to data buffer 01294 * @param BufferSize: Amount of data to be read 01295 * @retval HAL status 01296 */ 01297 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize) 01298 { 01299 return (I2Cx_ReadBuffer(DevAddress, MemAddress, I2C_MEMADD_SIZE_16BIT, pBuffer, BufferSize)); 01300 } 01301 01302 /** 01303 * @brief Checks if target device is ready for communication. 01304 * @note This function is used with Memory devices 01305 * @param DevAddress: Target device address 01306 * @param Trials: Number of trials 01307 * @retval HAL status 01308 */ 01309 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01310 { 01311 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01312 } 01313 01314 /********************************* LINK I2C TEMPERATURE SENSOR *****************************/ 01315 /** 01316 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 01317 * @retval None 01318 */ 01319 void TSENSOR_IO_Init(void) 01320 { 01321 I2Cx_Init(); 01322 } 01323 01324 /** 01325 * @brief Writes one byte to the TSENSOR. 01326 * @param DevAddress: Target device address 01327 * @param pBuffer: Pointer to data buffer 01328 * @param WriteAddr: TSENSOR's internal address to write to. 01329 * @param Length: Number of data to write 01330 * @retval None 01331 */ 01332 void TSENSOR_IO_Write(uint16_t DevAddress, uint8_t* pBuffer, uint8_t WriteAddr, uint16_t Length) 01333 { 01334 I2Cx_WriteBuffer(DevAddress, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01335 } 01336 01337 /** 01338 * @brief Reads one byte from the TSENSOR. 01339 * @param DevAddress: Target device address 01340 * @param pBuffer : pointer to the buffer that receives the data read from the TSENSOR. 01341 * @param ReadAddr : TSENSOR's internal address to read from. 01342 * @param Length: Number of data to read 01343 * @retval None 01344 */ 01345 void TSENSOR_IO_Read(uint16_t DevAddress, uint8_t* pBuffer, uint8_t ReadAddr, uint16_t Length) 01346 { 01347 I2Cx_ReadBuffer(DevAddress, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, Length); 01348 } 01349 01350 /** 01351 * @brief Checks if Temperature Sensor is ready for communication. 01352 * @param DevAddress: Target device address 01353 * @param Trials: Number of trials 01354 * @retval HAL status 01355 */ 01356 uint16_t TSENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 01357 { 01358 return (I2Cx_IsDeviceReady(DevAddress, Trials)); 01359 } 01360 01361 /***************************** LINK ACCELERO *****************************/ 01362 /** 01363 * @brief Configures ACCELEROMETER SPI interface. 01364 * @retval None 01365 */ 01366 void ACCELERO_IO_Init(void) 01367 { 01368 /* Initialize the IO functionalities */ 01369 BSP_IO_Init(); 01370 } 01371 01372 01373 /** 01374 * @brief Configures ACCELERO INT2 config. 01375 EXTI0 is already used by user button so INT1 is configured here 01376 * @retval None 01377 */ 01378 void ACCELERO_IO_ITConfig(void) 01379 { 01380 BSP_IO_ConfigPin(MEMS_ALL_PINS, IO_MODE_IT_FALLING_EDGE); 01381 } 01382 01383 /** 01384 * @brief Writes one byte to the ACCELEROMETER. 01385 * @param pBuffer : pointer to the buffer containing the data to be written to the ACCELEROMETER. 01386 * @param WriteAddr : ACCELEROMETER's internal address to write to. 01387 * @param NumByteToWrite: Number of bytes to write. 01388 * @retval None 01389 */ 01390 void ACCELERO_IO_Write(uint8_t* pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite) 01391 { 01392 I2Cx_WriteBuffer(L1S302DL_I2C_ADDRESS, WriteAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, NumByteToWrite); 01393 } 01394 01395 /** 01396 * @brief Reads a block of data from the ACCELEROMETER. 01397 * @param pBuffer : pointer to the buffer that receives the data read from the ACCELEROMETER. 01398 * @param ReadAddr : ACCELEROMETER's internal address to read from. 01399 * @param NumByteToRead : number of bytes to read from the ACCELEROMETER. 01400 * @retval None 01401 */ 01402 void ACCELERO_IO_Read(uint8_t* pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead) 01403 { 01404 I2Cx_ReadBuffer(L1S302DL_I2C_ADDRESS, ReadAddr, I2C_MEMADD_SIZE_8BIT, pBuffer, NumByteToRead); 01405 } 01406 01407 /********************************* LINK AUDIO ***********************************/ 01408 01409 /** 01410 * @brief Initializes Audio low level. 01411 * @retval None 01412 */ 01413 void AUDIO_IO_Init(void) 01414 { 01415 /* Initialize the IO functionalities */ 01416 BSP_IO_Init(); 01417 01418 BSP_IO_ConfigPin(AUDIO_RESET_PIN, IO_MODE_OUTPUT); 01419 01420 /* Power Down the codec */ 01421 BSP_IO_WritePin(AUDIO_RESET_PIN, GPIO_PIN_RESET); 01422 01423 /* wait for a delay to insure registers erasing */ 01424 HAL_Delay(5); 01425 01426 /* Power on the codec */ 01427 BSP_IO_WritePin(AUDIO_RESET_PIN, GPIO_PIN_SET); 01428 01429 /* wait for a delay to insure registers erasing */ 01430 HAL_Delay(5); 01431 01432 } 01433 01434 /** 01435 * @brief Writes a single data. 01436 * @param Addr: I2C address 01437 * @param Reg: Reg address 01438 * @param Value: Data to be written 01439 * @retval None 01440 */ 01441 void AUDIO_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) 01442 { 01443 I2Cx_WriteData(Addr, Reg, Value); 01444 } 01445 01446 /** 01447 * @brief Reads a single data. 01448 * @param Addr: I2C address 01449 * @param Reg: Reg address 01450 * @retval Data to be read 01451 */ 01452 uint8_t AUDIO_IO_Read (uint8_t Addr, uint8_t Reg) 01453 { 01454 return I2Cx_ReadData(Addr, Reg); 01455 } 01456 01457 #endif /* HAL_I2C_MODULE_ENABLED */ 01458 01459 /** 01460 * @} 01461 */ 01462 01463 /** 01464 * @} 01465 */ 01466 01467 /** 01468 * @} 01469 */ 01470 01471 /** 01472 * @} 01473 */ 01474 01475 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Dec 11 2014 15:38:28 for _BSP_User_Manual by
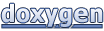