STM32091C_EVAL BSP User Manual
|
stm32091c_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32091c_eval_lcd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the driver for Liquid Crystal Display modules 00006 * mounted on STM32091C-EVAL evaluation board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 @verbatim 00036 ============================================================================== 00037 ##### How to use this driver ##### 00038 ============================================================================== 00039 [..] 00040 (#) This driver is used to drive indirectly an LCD TFT. 00041 (#) This driver supports the AM-240320LDTNQW00H (SPFD5408D) and 00042 AM240320LGTNQW00H (HX8347D) LCD mounted on MB895 daughter board 00043 (#) The SPFD5408D and HX8347D components driver MUST be included with this driver. 00044 00045 (#) Initialization steps: 00046 (++) Initialize the LCD using the LCD_Init() function. 00047 00048 (#) Display on LCD 00049 (++) Clear the hole LCD using yhe LCD_Clear() function or only one specified 00050 string line using the LCD_ClearStringLine() function. 00051 (++) Display a character on the specified line and column using the LCD_DisplayChar() 00052 function or a complete string line using the LCD_DisplayStringAtLine() function. 00053 (++) Display a string line on the specified position (x,y in pixel) and align mode 00054 using the LCD_DisplayStringAtLine() function. 00055 (++) Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00056 on LCD using a set of functions. 00057 @endverbatim 00058 */ 00059 00060 00061 /* Includes ------------------------------------------------------------------*/ 00062 #include "stm32091c_eval_lcd.h" 00063 #include "../../../Utilities/Fonts/fonts.h" 00064 #include "../../../Utilities/Fonts/font24.c" 00065 #include "../../../Utilities/Fonts/font20.c" 00066 #include "../../../Utilities/Fonts/font16.c" 00067 #include "../../../Utilities/Fonts/font12.c" 00068 #include "../../../Utilities/Fonts/font8.c" 00069 00070 /** @addtogroup BSP 00071 * @{ 00072 */ 00073 00074 /** @addtogroup STM32091C_EVAL 00075 * @{ 00076 */ 00077 00078 /** @addtogroup STM32091C_EVAL_LCD 00079 * @{ 00080 */ 00081 00082 /** @addtogroup STM32091C_EVAL_LCD_Private_Defines 00083 * @{ 00084 */ 00085 #define POLY_X(Z) ((int32_t)((pPoints + (Z))->X)) 00086 #define POLY_Y(Z) ((int32_t)((pPoints + (Z))->Y)) 00087 00088 #define MAX_HEIGHT_FONT 17 00089 #define MAX_WIDTH_FONT 24 00090 #define OFFSET_BITMAP 54 00091 /** 00092 * @} 00093 */ 00094 00095 /** @addtogroup STM32091C_EVAL_LCD_Private_Macros 00096 * @{ 00097 */ 00098 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00099 00100 /** 00101 * @} 00102 */ 00103 00104 /** @addtogroup STM32091C_EVAL_LCD_Private_Variables STM32091C_EVAL_LCD_Private_Variables 00105 * @{ 00106 */ 00107 LCD_DrawPropTypeDef DrawProp; 00108 00109 static LCD_DrvTypeDef *lcd_drv; 00110 00111 /* Max size of bitmap will based on a font24 (17x24) */ 00112 static uint8_t bitmap[MAX_HEIGHT_FONT*MAX_WIDTH_FONT*2+OFFSET_BITMAP] = {0}; 00113 00114 /** 00115 * @} 00116 */ 00117 00118 /** @addtogroup STM32091C_EVAL_LCD_Private_Functions 00119 * @{ 00120 */ 00121 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar); 00122 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00123 /** 00124 * @} 00125 */ 00126 00127 /** @addtogroup STM32091C_EVAL_LCD_Exported_Functions 00128 * @{ 00129 */ 00130 00131 /** 00132 * @brief Initializes the LCD. 00133 * @retval LCD state 00134 */ 00135 uint8_t BSP_LCD_Init(void) 00136 { 00137 uint8_t ret = LCD_ERROR; 00138 00139 /* Default value for draw propriety */ 00140 DrawProp.BackColor = 0xFFFF; 00141 DrawProp.pFont = &Font24; 00142 DrawProp.TextColor = 0x0000; 00143 00144 if(spfd5408_drv.ReadID() == SPFD5408_ID) 00145 { 00146 lcd_drv = &spfd5408_drv; 00147 ret = LCD_OK; 00148 } 00149 else 00150 { 00151 /*HX8347D_ID connected*/ 00152 lcd_drv = &hx8347d_drv; 00153 ret = LCD_OK; 00154 } 00155 00156 if(ret != LCD_ERROR) 00157 { 00158 /* LCD Init */ 00159 lcd_drv->Init(); 00160 00161 /* Initialize the font */ 00162 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00163 } 00164 00165 return ret; 00166 } 00167 00168 /** 00169 * @brief Gets the LCD X size. 00170 * @retval Used LCD X size 00171 */ 00172 uint32_t BSP_LCD_GetXSize(void) 00173 { 00174 return(lcd_drv->GetLcdPixelWidth()); 00175 } 00176 00177 /** 00178 * @brief Gets the LCD Y size. 00179 * @retval Used LCD Y size 00180 */ 00181 uint32_t BSP_LCD_GetYSize(void) 00182 { 00183 return(lcd_drv->GetLcdPixelHeight()); 00184 } 00185 00186 /** 00187 * @brief Gets the LCD text color. 00188 * @retval Used text color. 00189 */ 00190 uint16_t BSP_LCD_GetTextColor(void) 00191 { 00192 return DrawProp.TextColor; 00193 } 00194 00195 /** 00196 * @brief Gets the LCD background color. 00197 * @retval Used background color 00198 */ 00199 uint16_t BSP_LCD_GetBackColor(void) 00200 { 00201 return DrawProp.BackColor; 00202 } 00203 00204 /** 00205 * @brief Sets the LCD text color. 00206 * @param Color Text color code RGB(5-6-5) 00207 * @retval None 00208 */ 00209 void BSP_LCD_SetTextColor(uint16_t Color) 00210 { 00211 DrawProp.TextColor = Color; 00212 } 00213 00214 /** 00215 * @brief Sets the LCD background color. 00216 * @param Color Background color code RGB(5-6-5) 00217 * @retval None 00218 */ 00219 void BSP_LCD_SetBackColor(uint16_t Color) 00220 { 00221 DrawProp.BackColor = Color; 00222 } 00223 00224 /** 00225 * @brief Sets the LCD text font. 00226 * @param pFonts Font to be used 00227 * @retval None 00228 */ 00229 void BSP_LCD_SetFont(sFONT *pFonts) 00230 { 00231 DrawProp.pFont = pFonts; 00232 } 00233 00234 /** 00235 * @brief Gets the LCD text font. 00236 * @retval Used font 00237 */ 00238 sFONT *BSP_LCD_GetFont(void) 00239 { 00240 return DrawProp.pFont; 00241 } 00242 00243 /** 00244 * @brief Clears the hole LCD. 00245 * @param Color Color of the background 00246 * @retval None 00247 */ 00248 void BSP_LCD_Clear(uint16_t Color) 00249 { 00250 uint32_t counter = 0; 00251 00252 uint32_t color_backup = DrawProp.TextColor; 00253 DrawProp.TextColor = Color; 00254 00255 for(counter = 0; counter < BSP_LCD_GetYSize(); counter++) 00256 { 00257 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00258 } 00259 00260 DrawProp.TextColor = color_backup; 00261 BSP_LCD_SetTextColor(DrawProp.TextColor); 00262 } 00263 00264 /** 00265 * @brief Clears the selected line. 00266 * @param Line Line to be cleared 00267 * This parameter can be one of the following values: 00268 * @arg 0..9: if the Current fonts is Font16x24 00269 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00270 * @arg 0..29: if the Current fonts is Font8x8 00271 * @retval None 00272 */ 00273 void BSP_LCD_ClearStringLine(uint16_t Line) 00274 { 00275 uint32_t colorbackup = DrawProp.TextColor; 00276 DrawProp.TextColor = DrawProp.BackColor;; 00277 00278 /* Draw a rectangle with background color */ 00279 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00280 00281 DrawProp.TextColor = colorbackup; 00282 BSP_LCD_SetTextColor(DrawProp.TextColor); 00283 } 00284 00285 /** 00286 * @brief Displays one character. 00287 * @param Xpos Start column address 00288 * @param Ypos Line where to display the character shape. 00289 * @param Ascii Character ascii code 00290 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00291 * @retval None 00292 */ 00293 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00294 { 00295 LCD_DrawChar(Ypos, Xpos, &DrawProp.pFont->table[(Ascii-' ') *\ 00296 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00297 } 00298 00299 /** 00300 * @brief Displays characters on the LCD. 00301 * @param Xpos X position (in pixel) 00302 * @param Ypos Y position (in pixel) 00303 * @param pText Pointer to string to display on LCD 00304 * @param Mode Display mode 00305 * This parameter can be one of the following values: 00306 * @arg CENTER_MODE 00307 * @arg RIGHT_MODE 00308 * @arg LEFT_MODE 00309 * @retval None 00310 */ 00311 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *pText, Line_ModeTypdef Mode) 00312 { 00313 uint16_t refcolumn = 1, counter = 0; 00314 uint32_t size = 0, ysize = 0; 00315 uint8_t *ptr = pText; 00316 00317 /* Get the text size */ 00318 while (*ptr++) size ++ ; 00319 00320 /* Characters number per line */ 00321 ysize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00322 00323 switch (Mode) 00324 { 00325 case CENTER_MODE: 00326 { 00327 refcolumn = Xpos + ((ysize - size)* DrawProp.pFont->Width) / 2; 00328 break; 00329 } 00330 case LEFT_MODE: 00331 { 00332 refcolumn = Xpos; 00333 break; 00334 } 00335 case RIGHT_MODE: 00336 { 00337 refcolumn = Xpos + ((ysize - size)*DrawProp.pFont->Width); 00338 break; 00339 } 00340 default: 00341 { 00342 refcolumn = Xpos; 00343 break; 00344 } 00345 } 00346 00347 /* Send the string character by character on lCD */ 00348 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (counter*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00349 { 00350 /* Display one character on LCD */ 00351 BSP_LCD_DisplayChar(refcolumn, Ypos, *pText); 00352 /* Decrement the column position by 16 */ 00353 refcolumn += DrawProp.pFont->Width; 00354 /* Point on the next character */ 00355 pText++; 00356 counter++; 00357 } 00358 } 00359 00360 /** 00361 * @brief Displays a character on the LCD. 00362 * @param Line Line where to display the character shape 00363 * This parameter can be one of the following values: 00364 * @arg 0..9: if the Current fonts is Font16x24 00365 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00366 * @arg 0..29: if the Current fonts is Font8x8 00367 * @param pText Pointer to string to display on LCD 00368 * @retval None 00369 */ 00370 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *pText) 00371 { 00372 BSP_LCD_DisplayStringAt(0, LINE(Line),pText, LEFT_MODE); 00373 } 00374 00375 /** 00376 * @brief Reads an LCD pixel. 00377 * @param Xpos X position 00378 * @param Ypos Y position 00379 * @retval RGB pixel color 00380 */ 00381 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00382 { 00383 uint16_t ret = 0; 00384 00385 if(lcd_drv->ReadPixel != NULL) 00386 { 00387 ret = lcd_drv->ReadPixel(Xpos, Ypos); 00388 } 00389 00390 return ret; 00391 } 00392 00393 /** 00394 * @brief Draws an horizontal line. 00395 * @param Xpos X position 00396 * @param Ypos Y position 00397 * @param Length Line length 00398 * @retval None 00399 */ 00400 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00401 { 00402 uint32_t index = 0; 00403 00404 if(lcd_drv->DrawHLine != NULL) 00405 { 00406 lcd_drv->DrawHLine(DrawProp.TextColor, Ypos, Xpos, Length); 00407 } 00408 else 00409 { 00410 for(index = 0; index < Length; index++) 00411 { 00412 BSP_LCD_DrawPixel((Ypos + index), Xpos, DrawProp.TextColor); 00413 } 00414 } 00415 } 00416 00417 /** 00418 * @brief Draws a vertical line. 00419 * @param Xpos X position 00420 * @param Ypos Y position 00421 * @param Length Line length 00422 * @retval None 00423 */ 00424 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00425 { 00426 uint32_t index = 0; 00427 00428 if(lcd_drv->DrawVLine != NULL) 00429 { 00430 LCD_SetDisplayWindow(Ypos, Xpos, 1, Length); 00431 lcd_drv->DrawVLine(DrawProp.TextColor, Ypos, Xpos, Length); 00432 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00433 } 00434 else 00435 { 00436 for(index = 0; index < Length; index++) 00437 { 00438 BSP_LCD_DrawPixel(Ypos, Xpos + index, DrawProp.TextColor); 00439 } 00440 } 00441 } 00442 00443 /** 00444 * @brief Draws an uni-line (between two points). 00445 * @param X1 Point 1 X position 00446 * @param Y1 Point 1 Y position 00447 * @param X2 Point 2 X position 00448 * @param Y2 Point 2 Y position 00449 * @retval None 00450 */ 00451 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2) 00452 { 00453 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00454 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00455 curpixel = 0; 00456 00457 deltax = ABS(Y2 - Y1); /* The difference between the x's */ 00458 deltay = ABS(X2 - X1); /* The difference between the y's */ 00459 x = Y1; /* Start x off at the first pixel */ 00460 y = X1; /* Start y off at the first pixel */ 00461 00462 if (Y2 >= Y1) /* The x-values are increasing */ 00463 { 00464 xinc1 = 1; 00465 xinc2 = 1; 00466 } 00467 else /* The x-values are decreasing */ 00468 { 00469 xinc1 = -1; 00470 xinc2 = -1; 00471 } 00472 00473 if (X2 >= X1) /* The y-values are increasing */ 00474 { 00475 yinc1 = 1; 00476 yinc2 = 1; 00477 } 00478 else /* The y-values are decreasing */ 00479 { 00480 yinc1 = -1; 00481 yinc2 = -1; 00482 } 00483 00484 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00485 { 00486 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00487 yinc2 = 0; /* Don't change the y for every iteration */ 00488 den = deltax; 00489 num = deltax / 2; 00490 numadd = deltay; 00491 numpixels = deltax; /* There are more x-values than y-values */ 00492 } 00493 else /* There is at least one y-value for every x-value */ 00494 { 00495 xinc2 = 0; /* Don't change the x for every iteration */ 00496 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00497 den = deltay; 00498 num = deltay / 2; 00499 numadd = deltax; 00500 numpixels = deltay; /* There are more y-values than x-values */ 00501 } 00502 00503 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00504 { 00505 BSP_LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00506 num += numadd; /* Increase the numerator by the top of the fraction */ 00507 if (num >= den) /* Check if numerator >= denominator */ 00508 { 00509 num -= den; /* Calculate the new numerator value */ 00510 x += xinc1; /* Change the x as appropriate */ 00511 y += yinc1; /* Change the y as appropriate */ 00512 } 00513 x += xinc2; /* Change the x as appropriate */ 00514 y += yinc2; /* Change the y as appropriate */ 00515 } 00516 } 00517 00518 /** 00519 * @brief Draws a rectangle. 00520 * @param Xpos X position 00521 * @param Ypos Y position 00522 * @param Width Rectangle width 00523 * @param Height Rectangle height 00524 * @retval None 00525 */ 00526 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00527 { 00528 /* Draw horizontal lines */ 00529 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00530 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00531 00532 /* Draw vertical lines */ 00533 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00534 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00535 } 00536 00537 /** 00538 * @brief Draws a circle. 00539 * @param Xpos X position 00540 * @param Ypos Y position 00541 * @param Radius Circle radius 00542 * @retval None 00543 */ 00544 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00545 { 00546 int32_t decision; /* Decision Variable */ 00547 uint32_t curx; /* Current X Value */ 00548 uint32_t cury; /* Current Y Value */ 00549 00550 decision = 3 - (Radius << 1); 00551 curx = 0; 00552 cury = Radius; 00553 00554 while (curx <= cury) 00555 { 00556 BSP_LCD_DrawPixel((Ypos + curx), (Xpos - cury), DrawProp.TextColor); 00557 00558 BSP_LCD_DrawPixel((Ypos - curx), (Xpos - cury), DrawProp.TextColor); 00559 00560 BSP_LCD_DrawPixel((Ypos + cury), (Xpos - curx), DrawProp.TextColor); 00561 00562 BSP_LCD_DrawPixel((Ypos - cury), (Xpos - curx), DrawProp.TextColor); 00563 00564 BSP_LCD_DrawPixel((Ypos + curx), (Xpos + cury), DrawProp.TextColor); 00565 00566 BSP_LCD_DrawPixel((Ypos - curx), (Xpos + cury), DrawProp.TextColor); 00567 00568 BSP_LCD_DrawPixel((Ypos + cury), (Xpos + curx), DrawProp.TextColor); 00569 00570 BSP_LCD_DrawPixel((Ypos - cury), (Xpos + curx), DrawProp.TextColor); 00571 00572 /* Initialize the font */ 00573 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00574 00575 if (decision < 0) 00576 { 00577 decision += (curx << 2) + 6; 00578 } 00579 else 00580 { 00581 decision += ((curx - cury) << 2) + 10; 00582 cury--; 00583 } 00584 curx++; 00585 } 00586 } 00587 00588 /** 00589 * @brief Draws an poly-line (between many points). 00590 * @param pPoints Pointer to the points array 00591 * @param PointCount Number of points 00592 * @retval None 00593 */ 00594 void BSP_LCD_DrawPolygon(pPoint pPoints, uint16_t PointCount) 00595 { 00596 int16_t x = 0, y = 0; 00597 00598 if(PointCount < 2) 00599 { 00600 return; 00601 } 00602 00603 BSP_LCD_DrawLine(pPoints->X, pPoints->Y, (pPoints+PointCount-1)->X, (pPoints+PointCount-1)->Y); 00604 00605 while(--PointCount) 00606 { 00607 x = pPoints->X; 00608 y = pPoints->Y; 00609 pPoints++; 00610 BSP_LCD_DrawLine(x, y, pPoints->X, pPoints->Y); 00611 } 00612 00613 } 00614 00615 /** 00616 * @brief Draws an ellipse on LCD. 00617 * @param Xpos X position 00618 * @param Ypos Y position 00619 * @param XRadius Ellipse X radius 00620 * @param YRadius Ellipse Y radius 00621 * @retval None 00622 */ 00623 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00624 { 00625 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00626 float k = 0, rad1 = 0, rad2 = 0; 00627 00628 rad1 = YRadius; 00629 rad2 = XRadius; 00630 00631 k = (float)(rad2/rad1); 00632 00633 do { 00634 BSP_LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00635 BSP_LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00636 BSP_LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00637 BSP_LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00638 00639 e2 = err; 00640 if (e2 <= x) { 00641 err += ++x*2+1; 00642 if (-y == x && e2 <= y) e2 = 0; 00643 } 00644 if (e2 > y) err += ++y*2+1; 00645 } 00646 while (y <= 0); 00647 } 00648 00649 /** 00650 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 00651 * @param Xpos Bmp X position in the LCD 00652 * @param Ypos Bmp Y position in the LCD 00653 * @param pBmp Pointer to Bmp picture address in the internal Flash 00654 * @retval None 00655 */ 00656 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pBmp) 00657 { 00658 uint32_t height = 0, width = 0; 00659 00660 /* Read bitmap width */ 00661 width = *(uint16_t *) (pBmp + 18); 00662 width |= (*(uint16_t *) (pBmp + 20)) << 16; 00663 00664 /* Read bitmap height */ 00665 height = *(uint16_t *) (pBmp + 22); 00666 height |= (*(uint16_t *) (pBmp + 24)) << 16; 00667 00668 /* Remap Ypos, hx8347d works with inverted X in case of bitmap */ 00669 /* X = 0, cursor is on Bottom corner */ 00670 if(lcd_drv == &hx8347d_drv) 00671 { 00672 Ypos = BSP_LCD_GetYSize() - Ypos - height; 00673 } 00674 00675 LCD_SetDisplayWindow(Ypos, Xpos, width, height); 00676 00677 if(lcd_drv->DrawBitmap != NULL) 00678 { 00679 lcd_drv->DrawBitmap(Ypos, Xpos, pBmp); 00680 } 00681 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00682 } 00683 00684 /** 00685 * @brief Draws a full rectangle. 00686 * @param Xpos X position 00687 * @param Ypos Y position 00688 * @param Width Rectangle width 00689 * @param Height Rectangle height 00690 * @retval None 00691 */ 00692 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00693 { 00694 BSP_LCD_SetTextColor(DrawProp.TextColor); 00695 do 00696 { 00697 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00698 } 00699 while(Height--); 00700 } 00701 00702 /** 00703 * @brief Draws a full circle. 00704 * @param Xpos X position 00705 * @param Ypos Y position 00706 * @param Radius Circle radius 00707 * @retval None 00708 */ 00709 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00710 { 00711 int32_t decision; /* Decision Variable */ 00712 uint32_t curx; /* Current X Value */ 00713 uint32_t cury; /* Current Y Value */ 00714 00715 decision = 3 - (Radius << 1); 00716 00717 curx = 0; 00718 cury = Radius; 00719 00720 BSP_LCD_SetTextColor(DrawProp.TextColor); 00721 00722 while (curx <= cury) 00723 { 00724 if(cury > 0) 00725 { 00726 BSP_LCD_DrawVLine(Xpos + curx, Ypos - cury, 2*cury); 00727 BSP_LCD_DrawVLine(Xpos - curx, Ypos - cury, 2*cury); 00728 } 00729 00730 if(curx > 0) 00731 { 00732 BSP_LCD_DrawVLine(Xpos - cury, Ypos - curx, 2*curx); 00733 BSP_LCD_DrawVLine(Xpos + cury, Ypos - curx, 2*curx); 00734 } 00735 if (decision < 0) 00736 { 00737 decision += (curx << 2) + 6; 00738 } 00739 else 00740 { 00741 decision += ((curx - cury) << 2) + 10; 00742 cury--; 00743 } 00744 curx++; 00745 } 00746 00747 BSP_LCD_SetTextColor(DrawProp.TextColor); 00748 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00749 } 00750 00751 /** 00752 * @brief Draws a full ellipse. 00753 * @param Xpos X position 00754 * @param Ypos Y position 00755 * @param XRadius Ellipse X radius 00756 * @param YRadius Ellipse Y radius 00757 * @retval None 00758 */ 00759 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00760 { 00761 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00762 float k = 0, rad1 = 0, rad2 = 0; 00763 00764 rad1 = YRadius; 00765 rad2 = XRadius; 00766 00767 k = (float)(rad2/rad1); 00768 00769 do 00770 { 00771 BSP_LCD_DrawVLine((Xpos+y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00772 BSP_LCD_DrawVLine((Xpos-y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00773 00774 e2 = err; 00775 if (e2 <= x) 00776 { 00777 err += ++x*2+1; 00778 if (-y == x && e2 <= y) e2 = 0; 00779 } 00780 if (e2 > y) err += ++y*2+1; 00781 } 00782 while (y <= 0); 00783 } 00784 00785 /** 00786 * @brief Enables the display. 00787 * @retval None 00788 */ 00789 void BSP_LCD_DisplayOn(void) 00790 { 00791 lcd_drv->DisplayOn(); 00792 } 00793 00794 /** 00795 * @brief Disables the display. 00796 * @retval None 00797 */ 00798 void BSP_LCD_DisplayOff(void) 00799 { 00800 lcd_drv->DisplayOff(); 00801 } 00802 00803 /** 00804 * @brief Draws a pixel on LCD. 00805 * @param Xpos X position 00806 * @param Ypos Y position 00807 * @param RGBCode Pixel color in RGB mode (5-6-5) 00808 * @retval None 00809 */ 00810 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode) 00811 { 00812 if(lcd_drv->WritePixel != NULL) 00813 { 00814 lcd_drv->WritePixel(Xpos, Ypos, RGBCode); 00815 } 00816 } 00817 /** 00818 * @} 00819 */ 00820 00821 /****************************************************************************** 00822 Static Function 00823 *******************************************************************************/ 00824 /** @addtogroup STM32091C_EVAL_LCD_Private_Functions 00825 * @{ 00826 */ 00827 00828 /** 00829 * @brief Draws a character on LCD. 00830 * @param Xpos Line where to display the character shape 00831 * @param Ypos Start column address 00832 * @param pChar Pointer to the character data 00833 * @retval None 00834 */ 00835 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar) 00836 { 00837 uint32_t counterh = 0, counterw = 0, index = 0; 00838 uint16_t height = 0, width = 0; 00839 uint8_t offset = 0; 00840 uint8_t *pchar = NULL; 00841 uint32_t line = 0; 00842 00843 height = DrawProp.pFont->Height; 00844 width = DrawProp.pFont->Width; 00845 00846 /* Fill bitmap header*/ 00847 *(uint16_t *) (bitmap + 2) = (uint16_t)(height*width*2+OFFSET_BITMAP); 00848 *(uint16_t *) (bitmap + 4) = (uint16_t)((height*width*2+OFFSET_BITMAP)>>16); 00849 *(uint16_t *) (bitmap + 10) = OFFSET_BITMAP; 00850 *(uint16_t *) (bitmap + 18) = (uint16_t)(width); 00851 *(uint16_t *) (bitmap + 20) = (uint16_t)((width)>>16); 00852 *(uint16_t *) (bitmap + 22) = (uint16_t)(height); 00853 *(uint16_t *) (bitmap + 24) = (uint16_t)((height)>>16); 00854 00855 offset = 8 *((width + 7)/8) - width ; 00856 00857 for(counterh = 0; counterh < height; counterh++) 00858 { 00859 pchar = ((uint8_t *)pChar + (width + 7)/8 * counterh); 00860 00861 if(((width + 7)/8) == 3) 00862 { 00863 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 00864 } 00865 00866 if(((width + 7)/8) == 2) 00867 { 00868 line = (pchar[0]<< 8) | pchar[1]; 00869 } 00870 00871 if(((width + 7)/8) == 1) 00872 { 00873 line = pchar[0]; 00874 } 00875 00876 for (counterw = 0; counterw < width; counterw++) 00877 { 00878 /* Image in the bitmap is written from the bottom to the top */ 00879 /* Need to invert image in the bitmap */ 00880 index = (((height-counterh-1)*width)+(counterw))*2+OFFSET_BITMAP; 00881 if(line & (1 << (width- counterw + offset- 1))) 00882 { 00883 bitmap[index] = (uint8_t)DrawProp.TextColor; 00884 bitmap[index+1] = (uint8_t)(DrawProp.TextColor >> 8); 00885 } 00886 else 00887 { 00888 bitmap[index] = (uint8_t)DrawProp.BackColor; 00889 bitmap[index+1] = (uint8_t)(DrawProp.BackColor >> 8); 00890 } 00891 } 00892 } 00893 00894 BSP_LCD_DrawBitmap(Ypos, Xpos, bitmap); 00895 } 00896 00897 /** 00898 * @brief Sets display window. 00899 * @param Xpos LCD X position 00900 * @param Ypos LCD Y position 00901 * @param Width LCD window width 00902 * @param Height LCD window height 00903 * @retval None 00904 */ 00905 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00906 { 00907 if(lcd_drv->SetDisplayWindow != NULL) 00908 { 00909 lcd_drv->SetDisplayWindow(Xpos, Ypos, Width, Height); 00910 } 00911 } 00912 /** 00913 * @} 00914 */ 00915 00916 /** 00917 * @} 00918 */ 00919 00920 /** 00921 * @} 00922 */ 00923 00924 /** 00925 * @} 00926 */ 00927 00928 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jul 5 2017 09:21:50 for STM32091C_EVAL BSP User Manual by
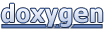