STM32072B_EVAL BSP User Manual
|
This file provides a set of functions needed to manage the SPI SD Card memory mounted on STM32072B-EVAL board. It implements a high level communication layer for read and write from/to this memory. The needed STM32F0xx hardware resources (SPI and GPIO) are defined in stm32072b_eval.h file, and the initialization is performed in SD_IO_Init() function declared in stm32072b_eval.c file. You can easily tailor this driver to any other development board, by just adapting the defines for hardware resources and SD_IO_Init() function. More...
#include "stm32072b_eval_sd.h"
#include "stm32f0xx_hal.h"
#include "stdlib.h"
#include "string.h"
#include "stdio.h"
Go to the source code of this file.
Data Structures | |
struct | SD_CmdAnswer_typedef |
Defines | |
#define | SD_DUMMY_BYTE 0xFF |
#define | SD_MAX_FRAME_LENGTH 17 /* Lenght = 16 + 1 */ |
#define | SD_CMD_LENGTH 6 |
#define | SD_MAX_TRY 100 /* Number of try */ |
#define | SD_CSD_STRUCT_V1 0x2 /* CSD struct version V1 */ |
#define | SD_CSD_STRUCT_V2 0x1 /* CSD struct version V2 */ |
#define | SD_TOKEN_START_DATA_SINGLE_BLOCK_READ 0xFE /* Data token start byte, Start Single Block Read */ |
Start Data tokens: Tokens (necessary because at nop/idle (and CS active) only 0xff is on the data/command line) | |
#define | SD_TOKEN_START_DATA_MULTIPLE_BLOCK_READ 0xFE /* Data token start byte, Start Multiple Block Read */ |
#define | SD_TOKEN_START_DATA_SINGLE_BLOCK_WRITE 0xFE /* Data token start byte, Start Single Block Write */ |
#define | SD_TOKEN_START_DATA_MULTIPLE_BLOCK_WRITE 0xFD /* Data token start byte, Start Multiple Block Write */ |
#define | SD_TOKEN_STOP_DATA_MULTIPLE_BLOCK_WRITE 0xFD /* Data toke stop byte, Stop Multiple Block Write */ |
#define | SD_CMD_GO_IDLE_STATE 0 /* CMD0 = 0x40 */ |
Commands: CMDxx = CMD-number | 0x40. | |
#define | SD_CMD_SEND_OP_COND 1 /* CMD1 = 0x41 */ |
#define | SD_CMD_SEND_IF_COND 8 /* CMD8 = 0x48 */ |
#define | SD_CMD_SEND_CSD 9 /* CMD9 = 0x49 */ |
#define | SD_CMD_SEND_CID 10 /* CMD10 = 0x4A */ |
#define | SD_CMD_STOP_TRANSMISSION 12 /* CMD12 = 0x4C */ |
#define | SD_CMD_SEND_STATUS 13 /* CMD13 = 0x4D */ |
#define | SD_CMD_SET_BLOCKLEN 16 /* CMD16 = 0x50 */ |
#define | SD_CMD_READ_SINGLE_BLOCK 17 /* CMD17 = 0x51 */ |
#define | SD_CMD_READ_MULT_BLOCK 18 /* CMD18 = 0x52 */ |
#define | SD_CMD_SET_BLOCK_COUNT 23 /* CMD23 = 0x57 */ |
#define | SD_CMD_WRITE_SINGLE_BLOCK 24 /* CMD24 = 0x58 */ |
#define | SD_CMD_WRITE_MULT_BLOCK 25 /* CMD25 = 0x59 */ |
#define | SD_CMD_PROG_CSD 27 /* CMD27 = 0x5B */ |
#define | SD_CMD_SET_WRITE_PROT 28 /* CMD28 = 0x5C */ |
#define | SD_CMD_CLR_WRITE_PROT 29 /* CMD29 = 0x5D */ |
#define | SD_CMD_SEND_WRITE_PROT 30 /* CMD30 = 0x5E */ |
#define | SD_CMD_SD_ERASE_GRP_START 32 /* CMD32 = 0x60 */ |
#define | SD_CMD_SD_ERASE_GRP_END 33 /* CMD33 = 0x61 */ |
#define | SD_CMD_UNTAG_SECTOR 34 /* CMD34 = 0x62 */ |
#define | SD_CMD_ERASE_GRP_START 35 /* CMD35 = 0x63 */ |
#define | SD_CMD_ERASE_GRP_END 36 /* CMD36 = 0x64 */ |
#define | SD_CMD_UNTAG_ERASE_GROUP 37 /* CMD37 = 0x65 */ |
#define | SD_CMD_ERASE 38 /* CMD38 = 0x66 */ |
#define | SD_CMD_SD_APP_OP_COND 41 /* CMD41 = 0x69 */ |
#define | SD_CMD_APP_CMD 55 /* CMD55 = 0x77 */ |
#define | SD_CMD_READ_OCR 58 /* CMD55 = 0x79 */ |
Enumerations | |
enum | SD_Answer_type { SD_ANSWER_R1_EXPECTED, SD_ANSWER_R1B_EXPECTED, SD_ANSWER_R2_EXPECTED, SD_ANSWER_R3_EXPECTED, SD_ANSWER_R4R5_EXPECTED, SD_ANSWER_R7_EXPECTED } |
SD ansewer format. More... | |
enum | SD_Error { SD_R1_NO_ERROR = (0x00), SD_R1_IN_IDLE_STATE = (0x01), SD_R1_ERASE_RESET = (0x02), SD_R1_ILLEGAL_COMMAND = (0x04), SD_R1_COM_CRC_ERROR = (0x08), SD_R1_ERASE_SEQUENCE_ERROR = (0x10), SD_R1_ADDRESS_ERROR = (0x20), SD_R1_PARAMETER_ERROR = (0x40), SD_R2_NO_ERROR = 0x00, SD_R2_CARD_LOCKED = 0x01, SD_R2_LOCKUNLOCK_ERROR = 0x02, SD_R2_ERROR = 0x04, SD_R2_CC_ERROR = 0x08, SD_R2_CARD_ECC_FAILED = 0x10, SD_R2_WP_VIOLATION = 0x20, SD_R2_ERASE_PARAM = 0x40, SD_R2_OUTOFRANGE = 0x80, SD_DATA_OK = (0x05), SD_DATA_CRC_ERROR = (0x0B), SD_DATA_WRITE_ERROR = (0x0D), SD_DATA_OTHER_ERROR = (0xFF) } |
SD reponses and error flags. More... | |
Functions | |
static uint8_t | SD_GetCIDRegister (SD_CID *Cid) |
Reads the SD card CID register. | |
static uint8_t | SD_GetCSDRegister (SD_CSD *Csd) |
Reads the SD card SCD register. | |
static uint8_t | SD_GetDataResponse (void) |
Gets the SD card data response and check the busy flag. | |
static uint8_t | SD_GoIdleState (void) |
Put the SD in Idle state. | |
static SD_CmdAnswer_typedef | SD_SendCmd (uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t Answer) |
Send 5 bytes command to the SD card and get response. | |
static uint8_t | SD_WaitData (uint8_t data) |
Waits a data from the SD card. | |
static uint8_t | SD_ReadData (void) |
Waits a data until a value different from SD_DUMMY_BITE. | |
uint8_t | BSP_SD_Init (void) |
Initializes the SD/SD communication. | |
uint8_t | BSP_SD_IsDetected (void) |
Detects if SD card is correctly plugged in the memory slot or not. | |
uint8_t | BSP_SD_GetCardInfo (SD_CardInfo *pCardInfo) |
Returns information about specific card. | |
uint8_t | BSP_SD_ReadBlocks (uint32_t *pData, uint32_t ReadAddr, uint16_t BlockSize, uint32_t NumberOfBlocks) |
Reads block(s) from a specified address in the SD card, in polling mode. | |
uint8_t | BSP_SD_WriteBlocks (uint32_t *pData, uint32_t WriteAddr, uint16_t BlockSize, uint32_t NumberOfBlocks) |
Writes block(s) to a specified address in the SD card, in polling mode. | |
uint8_t | BSP_SD_Erase (uint32_t StartAddr, uint32_t EndAddr) |
Erases the specified memory area of the given SD card. | |
uint8_t | BSP_SD_GetStatus (void) |
Returns the SD status. | |
Variables | |
__IO uint8_t | SdStatus = SD_NOT_PRESENT |
uint16_t | flag_SDHC = 0 |
Detailed Description
This file provides a set of functions needed to manage the SPI SD Card memory mounted on STM32072B-EVAL board. It implements a high level communication layer for read and write from/to this memory. The needed STM32F0xx hardware resources (SPI and GPIO) are defined in stm32072b_eval.h file, and the initialization is performed in SD_IO_Init() function declared in stm32072b_eval.c file. You can easily tailor this driver to any other development board, by just adapting the defines for hardware resources and SD_IO_Init() function.
- Attention:
© COPYRIGHT(c) 2016 STMicroelectronics
Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: 1. Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. 3. Neither the name of STMicroelectronics nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
============================================================================== ##### How to use this driver ##### ============================================================================== [..] (#) This driver is used to drive the micro SD external card mounted on STM32072B-EVAL evaluation board. (#) This driver does not need a specific component driver for the micro SD device to be included with. (#) Initialization steps: (++) Initialize the micro SD card using the SD_Init() function. (++) To check the SD card presence you can use the function BSP_SD_IsDetected() which returns the detection status (++) The function BSP_SD_GetCardInfo() is used to get the micro SD card information which is stored in the structure "SD_CardInfo". (#) Micro SD card operations (++) The micro SD card can be accessed with read/write block(s) operations once it is reay for access. The access cand be performed in polling mode by calling the functions SD_ReadBlocks()/SD_WriteBlocks() (++) The SD erase block(s) is performed using the function BSP_SD_Erase() with specifying the number of blocks to erase. (++) The SD runtime status is returned when calling the function BSP_SD_GetStatus().
Definition in file stm32072b_eval_sd.c.
Generated on Wed Jul 5 2017 08:56:10 for STM32072B_EVAL BSP User Manual by
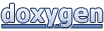