STM32072B_EVAL BSP User Manual
|
stm32072b_eval_sd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32072b_eval_sd.h 00004 * @author MCD Application Team 00005 * @brief This file contains the common defines and functions prototypes for 00006 * the stm32072b_eval_sd.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32072B_EVAL_SD_H 00039 #define __STM32072B_EVAL_SD_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32072b_eval.h" 00047 00048 /** @addtogroup BSP 00049 * @{ 00050 */ 00051 00052 /** @addtogroup STM32072B_EVAL 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32072B_EVAL_SD STM32072B_EVAL SD 00057 * @{ 00058 */ 00059 00060 /** @defgroup STM32072B_EVAL_SD_Exported_Types Exported Types 00061 * @{ 00062 */ 00063 00064 /** 00065 * @brief SD status structure definition 00066 */ 00067 enum { 00068 BSP_SD_OK = 0x00, 00069 MSD_OK = 0x00, 00070 BSP_SD_ERROR = 0x01, 00071 MSD_ERROR = 0x01, 00072 BSP_SD_TIMEOUT 00073 }; 00074 00075 typedef struct 00076 { 00077 uint8_t Reserved1:2; /* Reserved */ 00078 uint16_t DeviceSize:12; /* Device Size */ 00079 uint8_t MaxRdCurrentVDDMin:3; /* Max. read current @ VDD min */ 00080 uint8_t MaxRdCurrentVDDMax:3; /* Max. read current @ VDD max */ 00081 uint8_t MaxWrCurrentVDDMin:3; /* Max. write current @ VDD min */ 00082 uint8_t MaxWrCurrentVDDMax:3; /* Max. write current @ VDD max */ 00083 uint8_t DeviceSizeMul:3; /* Device size multiplier */ 00084 } struct_v1; 00085 00086 00087 typedef struct 00088 { 00089 uint8_t Reserved1:6; /* Reserved */ 00090 uint32_t DeviceSize:22; /* Device Size */ 00091 uint8_t Reserved2:1; /* Reserved */ 00092 } struct_v2; 00093 00094 /** 00095 * @brief Card Specific Data: CSD Register 00096 */ 00097 typedef struct 00098 { 00099 /* Header part */ 00100 uint8_t CSDStruct:2; /* CSD structure */ 00101 uint8_t Reserved1:6; /* Reserved */ 00102 uint8_t TAAC:8; /* Data read access-time 1 */ 00103 uint8_t NSAC:8; /* Data read access-time 2 in CLK cycles */ 00104 uint8_t MaxBusClkFrec:8; /* Max. bus clock frequency */ 00105 uint16_t CardComdClasses:12; /* Card command classes */ 00106 uint8_t RdBlockLen:4; /* Max. read data block length */ 00107 uint8_t PartBlockRead:1; /* Partial blocks for read allowed */ 00108 uint8_t WrBlockMisalign:1; /* Write block misalignment */ 00109 uint8_t RdBlockMisalign:1; /* Read block misalignment */ 00110 uint8_t DSRImpl:1; /* DSR implemented */ 00111 00112 /* v1 or v2 struct */ 00113 union csd_version { 00114 struct_v1 v1; 00115 struct_v2 v2; 00116 } version; 00117 00118 uint8_t EraseSingleBlockEnable:1; /* Erase single block enable */ 00119 uint8_t EraseSectorSize:7; /* Erase group size multiplier */ 00120 uint8_t WrProtectGrSize:7; /* Write protect group size */ 00121 uint8_t WrProtectGrEnable:1; /* Write protect group enable */ 00122 uint8_t Reserved2:2; /* Reserved */ 00123 uint8_t WrSpeedFact:3; /* Write speed factor */ 00124 uint8_t MaxWrBlockLen:4; /* Max. write data block length */ 00125 uint8_t WriteBlockPartial:1; /* Partial blocks for write allowed */ 00126 uint8_t Reserved3:5; /* Reserved */ 00127 uint8_t FileFormatGrouop:1; /* File format group */ 00128 uint8_t CopyFlag:1; /* Copy flag (OTP) */ 00129 uint8_t PermWrProtect:1; /* Permanent write protection */ 00130 uint8_t TempWrProtect:1; /* Temporary write protection */ 00131 uint8_t FileFormat:2; /* File Format */ 00132 uint8_t Reserved4:2; /* Reserved */ 00133 uint8_t crc:7; /* Reserved */ 00134 uint8_t Reserved5:1; /* always 1*/ 00135 00136 } SD_CSD; 00137 00138 /** 00139 * @brief Card Identification Data: CID Register 00140 */ 00141 typedef struct 00142 { 00143 __IO uint8_t ManufacturerID; /* ManufacturerID */ 00144 __IO uint16_t OEM_AppliID; /* OEM/Application ID */ 00145 __IO uint32_t ProdName1; /* Product Name part1 */ 00146 __IO uint8_t ProdName2; /* Product Name part2*/ 00147 __IO uint8_t ProdRev; /* Product Revision */ 00148 __IO uint32_t ProdSN; /* Product Serial Number */ 00149 __IO uint8_t Reserved1; /* Reserved1 */ 00150 __IO uint16_t ManufactDate; /* Manufacturing Date */ 00151 __IO uint8_t CID_CRC; /* CID CRC */ 00152 __IO uint8_t Reserved2; /* always 1 */ 00153 } SD_CID; 00154 00155 /** 00156 * @brief SD Card information 00157 */ 00158 typedef struct 00159 { 00160 SD_CSD Csd; 00161 SD_CID Cid; 00162 uint32_t CardCapacity; /* Card Capacity */ 00163 uint32_t CardBlockSize; /* Card Block Size */ 00164 } SD_CardInfo; 00165 00166 /** 00167 * @} 00168 */ 00169 00170 /** @defgroup STM32072B_EVAL_SPI_SD_Exported_Constants Exported Constants 00171 * @{ 00172 */ 00173 00174 /** 00175 * @brief Block Size 00176 */ 00177 #define SD_BLOCK_SIZE 0x200 00178 00179 /** 00180 * @brief SD detection on its memory slot 00181 */ 00182 #define SD_PRESENT ((uint8_t)0x01) 00183 #define SD_NOT_PRESENT ((uint8_t)0x00) 00184 00185 /** 00186 * @} 00187 */ 00188 00189 /** @defgroup STM32091C_EVAL_SD_Exported_Macro Exported Macro 00190 * @{ 00191 */ 00192 00193 /** 00194 * @} 00195 */ 00196 00197 /** @defgroup STM32072B_EVAL_SD_Exported_Functions Exported Functions 00198 * @{ 00199 */ 00200 uint8_t BSP_SD_Init(void); 00201 uint8_t BSP_SD_IsDetected(void); 00202 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint32_t ReadAddr, uint16_t BlockSize, uint32_t NumberOfBlocks); 00203 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint32_t WriteAddr, uint16_t BlockSize, uint32_t NumberOfBlocks); 00204 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr); 00205 uint8_t BSP_SD_GetStatus(void); 00206 uint8_t BSP_SD_GetCardInfo(SD_CardInfo *pCardInfo); 00207 00208 /* Link functions for SD Card peripheral */ 00209 void SD_IO_Init(void); 00210 void SD_IO_CSState(uint8_t state); 00211 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00212 uint8_t SD_IO_WriteByte(uint8_t Data); 00213 00214 /** 00215 * @} 00216 */ 00217 00218 /** @defgroup STM32072B_EVAL_SD_LINK_Operations_Functions LINK Operations Functions 00219 * @{ 00220 */ 00221 00222 /** 00223 * @} 00224 */ 00225 00226 /** 00227 * @} 00228 */ 00229 00230 /** 00231 * @} 00232 */ 00233 00234 /** 00235 * @} 00236 */ 00237 00238 #ifdef __cplusplus 00239 } 00240 #endif 00241 00242 #endif /* __STM32072B_EVAL_SD_H */ 00243 00244 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jul 5 2017 08:56:10 for STM32072B_EVAL BSP User Manual by
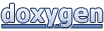