RLL Computer Vision Code
1.0
江苏科技大学HLL战队机器视觉代码
|
tool.cpp
浏览该文件的文档.
9 createTrackbar("trackBar", windowName, &g_trackBarLocation, static_cast<int>(count), onTrackBarCallback, &file);
Definition: tool.h:84
Definition: tool.h:83
Definition: tool.h:82
Definition: tool.h:85
static void addKeyboardControl(VideoCapture &srcFile, const int &delay=1)
添加键盘按键控制
Definition: tool.cpp:23
static void setTrackBarFollow(const string &windowName, const VideoCapture &file)
添加滑动控制条跟随视频进度功能
Definition: tool.cpp:17
制作者
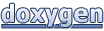