RLL Computer Vision Code
1.0
江苏科技大学HLL战队机器视觉代码
|
mainwindow.cpp
浏览该文件的文档.
void on_horizontalScrollBar_redMin_valueChanged(int value)
Definition: mainwindow.cpp:43
void on_minHeightGapRat_valueChanged(double arg1)
Definition: mainwindow.cpp:125
void on_minHeightWidthRat_valueChanged(double arg1)
Definition: mainwindow.cpp:100
Definition: mainwindow.h:10
void on_horizontalScrollBar_blueMax_valueChanged(int value)
Definition: mainwindow.cpp:78
int getThreshod(int channel, int minOrMax) const
获取指定通道最大值或者最小值
Definition: image_preprocessor.cpp:70
void on_minArea_valueChanged(double arg1)
Definition: mainwindow.cpp:90
void on_maxHeightGapRat_valueChanged(double arg1)
Definition: mainwindow.cpp:120
void on_maxHeightRat_valueChanged(double arg1)
Definition: mainwindow.cpp:110
void on_horizontalScrollBar_greenMin_valueChanged(int value)
Definition: mainwindow.cpp:50
void on_maxAngleDiff_valueChanged(double arg1)
Definition: mainwindow.cpp:115
void on_horizontalScrollBar_blueMin_valueChanged(int value)
Definition: mainwindow.cpp:57
void setThreshod(int channel, int minOrMax, int value)
设置预处理图像阈值
Definition: image_preprocessor.cpp:65
void on_horizontalScrollBar_redMax_valueChanged(int value)
Definition: mainwindow.cpp:64
void on_maxHeightWidthRat_valueChanged(double arg1)
Definition: mainwindow.cpp:95
struct HCVC::ArmourDetector::Params params
float minHeightWidthRat
Definition: armour_detector.h:81
void on_maxWidthRat_valueChanged(double arg1)
Definition: mainwindow.cpp:105
void on_angleRange_valueChanged(double arg1)
Definition: mainwindow.cpp:85
float maxHeightWidthRat
Definition: armour_detector.h:80
void on_horizontalScrollBar_greenMax_valueChanged(int value)
Definition: mainwindow.cpp:71
Definition: mainwindow.h:14
制作者
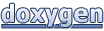