![]() |
OpenNI 2.0
|
#include <OpenNI.h>
Classes | |
class | FrameAllocator |
class | NewFrameListener |
Public Member Functions | |
VideoStream () | |
VideoStream (OniStreamHandle handle) | |
~VideoStream () | |
Status | addNewFrameListener (NewFrameListener *pListener) |
Status | create (const Device &device, SensorType sensorType) |
void | destroy () |
CameraSettings * | getCameraSettings () |
bool | getCropping (int *pOriginX, int *pOriginY, int *pWidth, int *pHeight) const |
float | getHorizontalFieldOfView () const |
int | getMaxPixelValue () const |
int | getMinPixelValue () const |
bool | getMirroringEnabled () const |
Status | getProperty (int propertyId, void *data, int *dataSize) const |
template<class T > | |
Status | getProperty (int propertyId, T *value) const |
const SensorInfo & | getSensorInfo () const |
float | getVerticalFieldOfView () const |
VideoMode | getVideoMode () const |
Status | invoke (int commandId, void *data, int dataSize) |
template<class T > | |
Status | invoke (int commandId, T &value) |
bool | isCommandSupported (int commandId) const |
bool | isCroppingSupported () const |
bool | isPropertySupported (int propertyId) const |
bool | isValid () const |
Status | readFrame (VideoFrameRef *pFrame) |
void | removeNewFrameListener (NewFrameListener *pListener) |
Status | resetCropping () |
Status | setCropping (int originX, int originY, int width, int height) |
Status | setFrameBuffersAllocator (FrameAllocator *pAllocator) |
Status | setMirroringEnabled (bool isEnabled) |
Status | setProperty (int propertyId, const void *data, int dataSize) |
template<class T > | |
Status | setProperty (int propertyId, const T &value) |
Status | setVideoMode (const VideoMode &videoMode) |
Status | start () |
void | stop () |
Friends | |
class | Device |
Detailed Description
The VideoStream object encapsulates a single video stream from a device. Once created, it is used to start data flow from the device, and to read individual frames of data. This is the central class used to obtain data in OpenNI. It provides the ability to manually read data in a polling loop, as well as providing events and a Listener class that can be used to implement event-driven data acquisition.
Aside from the video data frames themselves, the class offers a number of functions used for obtaining information about a VideoStream. Field of view, available video modes, and minimum and maximum valid pixel values can all be obtained.
In addition to obtaining data, the VideoStream object is used to set all configuration properties that apply to a specific stream (rather than to an entire device). In particular, it is used to control cropping, mirroring, and video modes.
A pointer to a valid, initialized device that provides the desired stream type is required to create a stream.
Several video streams can be created to stream data from the same sensor. This is useful if several components of an application need to read frames separately.
While some device might allow different streams from the same sensor to have different configurations, most devices will have a single configuration for the sensor, shared by all streams.
Constructor & Destructor Documentation
openni::VideoStream::VideoStream | ( | ) | [inline] |
Default constructor. Creates a new, non-valid VideoStream object. The object created will be invalid until its create() function is called with a valid Device.
openni::VideoStream::VideoStream | ( | OniStreamHandle | handle | ) | [inline, explicit] |
Handle constructor. Creates a VideoStream object based on the given initialized handle. This object will not destroy the underlying handle when destroy() or destructor is called
openni::VideoStream::~VideoStream | ( | ) | [inline] |
Destructor. The destructor calls the destroy() function, but it is considered a best practice for applications to call destroy() manually on any VideoStream that they run create() on.
Member Function Documentation
Status openni::VideoStream::addNewFrameListener | ( | NewFrameListener * | pListener | ) | [inline] |
Adds a new Listener to receive this VideoStream onNewFrame event. See VideoStream::NewFrameListener for more information on implementing an event driven frame reading architecture. An instance of a listener can be added to only one source.
- Parameters:
-
[in] pListener Pointer to a VideoStream::NewFrameListener object (or a derivative) that will respond to this event.
- Returns:
- Status code indicating success or failure of the operation.
Status openni::VideoStream::create | ( | const Device & | device, |
SensorType | sensorType | ||
) | [inline] |
Creates a stream of frames from a specific sensor type of a specific device. You must supply a reference to a Device that supplies the sensor type requested. You can use Device::hasSensor() to check whether a given sensor is available on your target device before calling create().
- Parameters:
-
[in] device A reference to the Device you want to create the stream on. [in] sensorType The type of sensor the stream should produce data from.
- Returns:
- Status code indicating success or failure for this operation.
void openni::VideoStream::destroy | ( | ) | [inline] |
Destroy this stream. This function is currently called automatically by the destructor, but it is considered a best practice for applications to manually call this function on any VideoStream that they call create() for.
CameraSettings* openni::VideoStream::getCameraSettings | ( | ) | [inline] |
Gets an object through which several camera settings can be configured.
- Returns:
- NULL if the stream doesn't support camera settings.
bool openni::VideoStream::getCropping | ( | int * | pOriginX, |
int * | pOriginY, | ||
int * | pWidth, | ||
int * | pHeight | ||
) | const [inline] |
Obtains the current cropping settings for this stream.
- Parameters:
-
[out] pOriginX X coordinate of the upper left corner of the cropping window [out] pOriginY Y coordinate of the upper left corner of the cropping window [out] pWidth Horizontal width of the cropping window, in pixels [out] pHeight Vertical width of the cropping window, in pixels returns true if cropping is currently enabled, false if it is not.
float openni::VideoStream::getHorizontalFieldOfView | ( | ) | const [inline] |
Gets the horizontal field of view of frames received from this stream.
- Returns:
- Horizontal field of view, in radians.
int openni::VideoStream::getMaxPixelValue | ( | ) | const [inline] |
Provides the maximum possible value for pixels obtained by this stream. This is most useful for getting the maximum possible value of depth streams.
- Returns:
- Maximum possible pixel value.
int openni::VideoStream::getMinPixelValue | ( | ) | const [inline] |
Provides the smallest possible value for pixels obtains by this VideoStream. This is most useful for getting the minimum possible value that will be reported by a depth stream.
- Returns:
- Minimum possible pixel value that can come from this stream.
bool openni::VideoStream::getMirroringEnabled | ( | ) | const [inline] |
Check whether mirroring is currently turned on for this stream.
- Returns:
- true if mirroring is currently enabled, false otherwise.
Status openni::VideoStream::getProperty | ( | int | propertyId, |
void * | data, | ||
int * | dataSize | ||
) | const [inline] |
General function for obtaining the value of stream specific properties. There are convenience functions available for all commonly used properties, so it is not expected that applications will make direct use of the getProperty function very often.
- Parameters:
-
[in] propertyId The numerical ID of the property to be queried. [out] data Place to store the value of the property. [in,out] dataSize IN: Size of the buffer passed in the data
argument. OUT: the actual written size.
- Returns:
- Status code indicating success or failure of this operation.
Status openni::VideoStream::getProperty | ( | int | propertyId, |
T * | value | ||
) | const [inline] |
Function for getting the value from a property using an arbitrary output type. There are convenience functions available for all commonly used properties, so it is not expected that applications will make direct use of this function very often.
- Template Parameters:
-
[in] T Data type of the value to be read.
- Parameters:
-
[in] propertyId The numerical ID of the property to be read. [in,out] value Pointer to a place to store the value read from the property.
- Returns:
- Status code indicating success or failure of this operation.
const SensorInfo& openni::VideoStream::getSensorInfo | ( | ) | const [inline] |
Provides the SensorInfo object associated with the sensor that is producing this VideoStream. Note that this function will return NULL if the stream has not yet been initialized with the create() function.
SensorInfo is useful primarily as a means of learning which video modes are valid for this VideoStream.
- Returns:
- Reference to the SensorInfo object associated with the sensor providing this stream.
float openni::VideoStream::getVerticalFieldOfView | ( | ) | const [inline] |
Gets the vertical field of view of frames received from this stream.
- Returns:
- Vertical field of view, in radians.
VideoMode openni::VideoStream::getVideoMode | ( | ) | const [inline] |
Get the current video mode information for this video stream. This includes its resolution, fps and stream format.
- Returns:
- Current video mode information for this video stream.
Status openni::VideoStream::invoke | ( | int | commandId, |
void * | data, | ||
int | dataSize | ||
) | [inline] |
Invokes a command that takes an arbitrary data type as its input. It is not expected that application code will need this function frequently, as all commonly used properties have higher level functions provided.
- Parameters:
-
[in] commandId Numerical code of the property to be invoked. [in] data Data to be passed to the property. [in] dataSize size of the buffer passed in data
.
- Returns:
- Status code indicating success or failure of this operation.
Status openni::VideoStream::invoke | ( | int | commandId, |
T & | value | ||
) | [inline] |
Invokes a command that takes an arbitrary data type as its input. It is not expected that application code will need this function frequently, as all commonly used properties have higher level functions provided.
- Template Parameters:
-
[in] T Type of data to be passed to the property.
- Parameters:
-
[in] commandId Numerical code of the property to be invoked. [in] value Data to be passed to the property.
- Returns:
- Status code indicating success or failure of this operation.
bool openni::VideoStream::isCommandSupported | ( | int | commandId | ) | const [inline] |
Checks if a specific command is supported by the video stream.
- Parameters:
-
[in] commandId Command to be checked.
- Returns:
- true if the command is supported, false otherwise.
bool openni::VideoStream::isCroppingSupported | ( | ) | const [inline] |
Checks whether this stream supports cropping.
- Returns:
- true if the stream supports cropping, false if it does not.
bool openni::VideoStream::isPropertySupported | ( | int | propertyId | ) | const [inline] |
Checks if a specific property is supported by the video stream.
- Parameters:
-
[in] propertyId Property to be checked.
- Returns:
- true if the property is supported, false otherwise.
bool openni::VideoStream::isValid | ( | ) | const [inline] |
Checks to see if this object has been properly initialized and currently points to a valid stream.
- Returns:
- true if this object has been previously initialized, false otherwise.
Status openni::VideoStream::readFrame | ( | VideoFrameRef * | pFrame | ) | [inline] |
Read the next frame from this video stream, delivered as a VideoFrameRef. This is the primary method for manually obtaining frames of video data. If no new frame is available, the call will block until one is available. To avoid blocking, use VideoStream::Listener to implement an event driven architecture. Another alternative is to use OpenNI::waitForAnyStream() to wait for new frames from several streams.
- Parameters:
-
[out] pFrame Pointer to a VideoFrameRef object to hold the reference to the new frame.
- Returns:
- Status code to indicated success or failure of this function.
void openni::VideoStream::removeNewFrameListener | ( | NewFrameListener * | pListener | ) | [inline] |
Removes a Listener from this video stream list. The listener removed will no longer receive new frame events from this stream.
- Parameters:
-
[in] pListener Pointer to the listener object to be removed.
Status openni::VideoStream::resetCropping | ( | ) | [inline] |
Disables cropping.
- Returns:
- Status code indicating success or failure of this operation.
Status openni::VideoStream::setCropping | ( | int | originX, |
int | originY, | ||
int | width, | ||
int | height | ||
) | [inline] |
Changes the cropping settings for this stream. You can use the isCroppingSupported() function to make sure cropping is supported before calling this function.
- Parameters:
-
[in] originX New X coordinate of the upper left corner of the cropping window. [in] originY New Y coordinate of the upper left corner of the cropping window. [in] width New horizontal width for the cropping window, in pixels. [in] height New vertical height for the cropping window, in pixels.
- Returns:
- Status code indicating success or failure of this operation.
Status openni::VideoStream::setFrameBuffersAllocator | ( | FrameAllocator * | pAllocator | ) | [inline] |
Sets the frame buffers allocator for this video stream.
- Parameters:
-
[in] pAllocator Pointer to the frame buffers allocator object. Pass NULL to return to default frame allocator.
- Returns:
- ONI_STATUS_OUT_OF_FLOW The frame buffers allocator cannot be set while stream is streaming.
Status openni::VideoStream::setMirroringEnabled | ( | bool | isEnabled | ) | [inline] |
Enable or disable mirroring for this stream.
- Parameters:
-
[in] isEnabled true to enable mirroring, false to disable it.
- Returns:
- Status code indicating the success or failure of this operation.
Status openni::VideoStream::setProperty | ( | int | propertyId, |
const void * | data, | ||
int | dataSize | ||
) | [inline] |
General function for setting the value of stream specific properties. There are convenience functions available for all commonly used properties, so it is not expected that applications will make direct use of the setProperty function very often.
- Parameters:
-
[in] propertyId The numerical ID of the property to be set. [in] data Place to store the data to be written to the property. [in] dataSize Size of the data to be written to the property.
- Returns:
- Status code indicating success or failure of this operation.
Status openni::VideoStream::setProperty | ( | int | propertyId, |
const T & | value | ||
) | [inline] |
Function for setting a value of a stream property using an arbitrary input type. There are convenience functions available for all commonly used properties, so it is not expected that applications will make direct use of this function very often.
- Template Parameters:
-
[in] T Data type of the value to be passed to the property.
- Parameters:
-
[in] propertyId The numerical ID of the property to be set. [in] value Data to be sent to the property.
- Returns:
- Status code indicating success or failure of this operation.
Changes the current video mode of this stream. Recommended practice is to use Device::getSensorInfo(), and then SensorInfo::getSupportedVideoModes() to obtain a list of valid video mode settings for this stream. Then, pass a valid VideoMode to setVideoMode to ensure correct operation.
- Parameters:
-
[in] videoMode Desired new video mode for this stream. returns Status code indicating success or failure of this operation.
Status openni::VideoStream::start | ( | ) | [inline] |
Starts data generation from this video stream.
void openni::VideoStream::stop | ( | ) | [inline] |
Stops data generation from this video stream.
Friends And Related Function Documentation
friend class Device [friend] |
The documentation for this class was generated from the following file:
Generated on Tue Nov 12 2013 16:10:45 for OpenNI 2.0 by
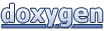