![]() |
OpenNI 2.0
|
#include <OpenNI.h>
Static Public Member Functions | |
static Status | convertDepthToColor (const VideoStream &depthStream, const VideoStream &colorStream, int depthX, int depthY, DepthPixel depthZ, int *pColorX, int *pColorY) |
static Status | convertDepthToWorld (const VideoStream &depthStream, int depthX, int depthY, DepthPixel depthZ, float *pWorldX, float *pWorldY, float *pWorldZ) |
static Status | convertDepthToWorld (const VideoStream &depthStream, float depthX, float depthY, float depthZ, float *pWorldX, float *pWorldY, float *pWorldZ) |
static Status | convertWorldToDepth (const VideoStream &depthStream, float worldX, float worldY, float worldZ, int *pDepthX, int *pDepthY, DepthPixel *pDepthZ) |
static Status | convertWorldToDepth (const VideoStream &depthStream, float worldX, float worldY, float worldZ, float *pDepthX, float *pDepthY, float *pDepthZ) |
Detailed Description
The CoordinateConverter class converts points between the different coordinate systems.
Depth and World coordinate systems
OpenNI applications commonly use two different coordinate systems to represent depth. These two systems are referred to as Depth and World representation.
Depth coordinates are the native data representation. In this system, the frame is a map (two dimensional array), and each pixel is assigned a depth value. This depth value represents the distance between the camera plane and whatever object is in the given pixel. The X and Y coordinates are simply the location in the map, where the origin is the top-left corner of the field of view.
World coordinates superimpose a more familiar 3D Cartesian coordinate system on the world, with the camera lens at the origin. In this system, every point is specified by 3 points -- x, y and z. The x axis of this system is along a line that passes through the infrared projector and CMOS imager of the camera. The y axis is parallel to the front face of the camera, and perpendicular to the x axis (it will also be perpendicular to the ground if the camera is upright and level). The z axis runs into the scene, perpendicular to both the x and y axis. From the perspective of the camera, an object moving from left to right is moving along the increasing x axis. An object moving up is moving along the increasing y axis, and an object moving away from the camera is moving along the increasing z axis.
Mathematically, the Depth coordinate system is the projection of the scene on the CMOS. If the sensor's angular field of view and resolution are known, then an angular size can be calculated for each pixel. This is how the conversion algorithms work. The dependence of this calculation on FoV and resolution is the reason that a VideoStream pointer must be provided to these functions. The VideoStream pointer is used to determine parameters for the specific points to be converted.
Since Depth coordinates are a projective, the apparent size of objects in depth coordinates (measured in pixels) will increase as an object moves closer to the sensor. The size of objects in the World coordinate system is independent of distance from the sensor.
Note that converting from Depth to World coordinates is relatively expensive computationally. It is generally not practical to convert the entire raw depth map to World coordinates. A better approach is to have your computer vision algorithm work in Depth coordinates for as long as possible, and only converting a few specific points to World coordinates right before output.
Note that when converting from Depth to World or vice versa, the Z value remains the same.
Member Function Documentation
static Status openni::CoordinateConverter::convertDepthToColor | ( | const VideoStream & | depthStream, |
const VideoStream & | colorStream, | ||
int | depthX, | ||
int | depthY, | ||
DepthPixel | depthZ, | ||
int * | pColorX, | ||
int * | pColorY | ||
) | [inline, static] |
For a given depth point, provides the coordinates of the corresponding color value. Useful for superimposing the depth and color images. This operation is the same as turning on registration, but is performed on a single pixel rather than the whole image.
- Parameters:
-
[in] depthStream Reference to a openni::VideoStream that produced the depth value [in] colorStream Reference to a openni::VideoStream that we want to find the appropriate color pixel in [in] depthX X value of the depth point, given in Depth coordinates and measured in pixels [in] depthY Y value of the depth point, given in Depth coordinates and measured in pixels [in] depthZ Z(depth) value of the depth point, given in the PixelFormat of depthStream [out] pColorX The X coordinate of the color pixel that overlaps the given depth pixel, measured in pixels [out] pColorY The Y coordinate of the color pixel that overlaps the given depth pixel, measured in pixels
static Status openni::CoordinateConverter::convertDepthToWorld | ( | const VideoStream & | depthStream, |
int | depthX, | ||
int | depthY, | ||
DepthPixel | depthZ, | ||
float * | pWorldX, | ||
float * | pWorldY, | ||
float * | pWorldZ | ||
) | [inline, static] |
Converts a single point from the Depth coordinate system to the World coordinate system.
- Parameters:
-
[in] depthStream Reference to an openi::VideoStream that will be used to determine the format of the Depth coordinates [in] depthX The X coordinate of the point to be converted, measured in pixels with 0 at the far left of the image [in] depthY The Y coordinate of the point to be converted, measured in pixels with 0 at the top of the image [in] depthZ the Z(depth) coordinate of the point to be converted, measured in the PixelFormat of depthStream [out] pWorldX Pointer to a place to store the X coordinate of the output value, measured in millimeters in World coordinates [out] pWorldY Pointer to a place to store the Y coordinate of the output value, measured in millimeters in World coordinates [out] pWorldZ Pointer to a place to store the Z coordinate of the output value, measured in millimeters in World coordinates
static Status openni::CoordinateConverter::convertDepthToWorld | ( | const VideoStream & | depthStream, |
float | depthX, | ||
float | depthY, | ||
float | depthZ, | ||
float * | pWorldX, | ||
float * | pWorldY, | ||
float * | pWorldZ | ||
) | [inline, static] |
Converts a single point from a floating point representation of the Depth coordinate system to the World coordinate system.
- Parameters:
-
[in] depthStream Reference to an openi::VideoStream that will be used to determine the format of the Depth coordinates [in] depthX The X coordinate of the point to be converted, measured in pixels with 0.0 at the far left of the image [in] depthY The Y coordinate of the point to be converted, measured in pixels with 0.0 at the top of the image [in] depthZ Z(depth) coordinate of the point to be converted, measured in the PixelFormat of depthStream [out] pWorldX Pointer to a place to store the X coordinate of the output value, measured in millimeters in World coordinates [out] pWorldY Pointer to a place to store the Y coordinate of the output value, measured in millimeters in World coordinates [out] pWorldZ Pointer to a place to store the Z coordinate of the output value, measured in millimeters in World coordinates
static Status openni::CoordinateConverter::convertWorldToDepth | ( | const VideoStream & | depthStream, |
float | worldX, | ||
float | worldY, | ||
float | worldZ, | ||
int * | pDepthX, | ||
int * | pDepthY, | ||
DepthPixel * | pDepthZ | ||
) | [inline, static] |
Converts a single point from the World coordinate system to the Depth coordinate system.
- Parameters:
-
[in] depthStream Reference to an openni::VideoStream that will be used to determine the format of the Depth coordinates [in] worldX The X coordinate of the point to be converted, measured in millimeters in World coordinates [in] worldY The Y coordinate of the point to be converted, measured in millimeters in World coordinates [in] worldZ The Z coordinate of the point to be converted, measured in millimeters in World coordinates [out] pDepthX Pointer to a place to store the X coordinate of the output value, measured in pixels with 0 at far left of image [out] pDepthY Pointer to a place to store the Y coordinate of the output value, measured in pixels with 0 at top of image [out] pDepthZ Pointer to a place to store the Z(depth) coordinate of the output value, measured in the PixelFormat of depthStream
static Status openni::CoordinateConverter::convertWorldToDepth | ( | const VideoStream & | depthStream, |
float | worldX, | ||
float | worldY, | ||
float | worldZ, | ||
float * | pDepthX, | ||
float * | pDepthY, | ||
float * | pDepthZ | ||
) | [inline, static] |
Converts a single point from the World coordinate system to a floating point representation of the Depth coordinate system
- Parameters:
-
[in] depthStream Reference to an openni::VideoStream that will be used to determine the format of the Depth coordinates [in] worldX The X coordinate of the point to be converted, measured in millimeters in World coordinates [in] worldY The Y coordinate of the point to be converted, measured in millimeters in World coordinates [in] worldZ The Z coordinate of the point to be converted, measured in millimeters in World coordinates [out] pDepthX Pointer to a place to store the X coordinate of the output value, measured in pixels with 0.0 at far left of the image [out] pDepthY Pointer to a place to store the Y coordinate of the output value, measured in pixels with 0.0 at the top of the image [out] pDepthZ Pointer to a place to store the Z(depth) coordinate of the output value, measured in millimeters with 0.0 at the camera lens
The documentation for this class was generated from the following file:
Generated on Tue Nov 12 2013 16:10:45 for OpenNI 2.0 by
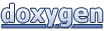