![]() |
OpenNI 2.0
|
#include <OpenNI.h>
Public Member Functions | |
Device () | |
Device (OniDeviceHandle handle) | |
~Device () | |
void | close () |
bool | getDepthColorSyncEnabled () |
const DeviceInfo & | getDeviceInfo () const |
ImageRegistrationMode | getImageRegistrationMode () const |
PlaybackControl * | getPlaybackControl () |
Status | getProperty (int propertyId, void *data, int *dataSize) const |
template<class T > | |
Status | getProperty (int propertyId, T *value) const |
const SensorInfo * | getSensorInfo (SensorType sensorType) |
bool | hasSensor (SensorType sensorType) |
Status | invoke (int commandId, void *data, int dataSize) |
template<class T > | |
Status | invoke (int propertyId, T &value) |
bool | isCommandSupported (int commandId) const |
bool | isFile () const |
bool | isImageRegistrationModeSupported (ImageRegistrationMode mode) const |
bool | isPropertySupported (int propertyId) const |
bool | isValid () const |
Status | open (const char *uri) |
Status | setDepthColorSyncEnabled (bool isEnabled) |
Status | setImageRegistrationMode (ImageRegistrationMode mode) |
Status | setProperty (int propertyId, const void *data, int dataSize) |
template<class T > | |
Status | setProperty (int propertyId, const T &value) |
Detailed Description
The Device object abstracts a specific device; either a single hardware device, or a file device holding a recording from a hardware device. It offers the ability to connect to the device, and obtain information about its configuration and the data streams it can offer.
It provides the means to query and change all configuration parameters that apply to the device as a whole. This includes enabling depth/color image registration and frame synchronization.
Devices are used when creating and initializing VideoStreams -- you will need a valid pointer to a Device in order to use the VideoStream.create() function. This, along with configuration, is the primary use of this class for application developers.
Before devices can be created, OpenNI::initialize() must have been run to make the device drivers on the system available to the API.
Constructor & Destructor Documentation
openni::Device::Device | ( | ) | [inline] |
openni::Device::Device | ( | OniDeviceHandle | handle | ) | [inline, explicit] |
openni::Device::~Device | ( | ) | [inline] |
Member Function Documentation
void openni::Device::close | ( | ) | [inline] |
Closes the device. This properly closes any files or shuts down hardware, as appropriate. This function is currently called by the destructor if not called manually by application code, but it is considered a best practice to manually close any device that was opened.
bool openni::Device::getDepthColorSyncEnabled | ( | ) | [inline] |
const DeviceInfo& openni::Device::getDeviceInfo | ( | ) | const [inline] |
Provides information about this device in the form of a DeviceInfo object. This object can be used to access the URI of the device, as well as various USB descriptor strings that might be useful to an application.
Note that valid device info will not be available if this device has not yet been opened. If you are trying to obtain a URI to open a device, use OpenNI::enumerateDevices() instead.
- Returns:
- DeviceInfo object for this Device
ImageRegistrationMode openni::Device::getImageRegistrationMode | ( | ) | const [inline] |
Gets the current image registration mode of this device. Image registration is used to properly superimpose two images from cameras located at different points in space. Please see the OpenNi 2.0 Programmer's Guide for more information about registration.
- Returns:
- Current image registration mode. See ImageRegistrationMode for possible return values.
PlaybackControl* openni::Device::getPlaybackControl | ( | ) | [inline] |
Gets an object through which playback of a file device can be controlled.
- Returns:
- NULL if this device is not a file device.
Status openni::Device::getProperty | ( | int | propertyId, |
void * | data, | ||
int * | dataSize | ||
) | const [inline] |
Get the value of a general property of the device. There are convenience functions for all the commonly used properties, such as image registration and frame synchronization. It is expected for this reason that this function will rarely be directly used by applications.
- Parameters:
-
[in] propertyId Numerical ID of the property you would like to check. [out] data Place to store the value of the property. [in,out] dataSize IN: Size of the buffer passed in the data
argument. OUT: the actual written size.
- Returns:
- Status code indicating results of this operation.
Status openni::Device::getProperty | ( | int | propertyId, |
T * | value | ||
) | const [inline] |
Checks a property that provides an arbitrary data type as its output. It is not expected that application code will need this function frequently, as all commonly used properties have higher level functions provided.
- Template Parameters:
-
[in] T Data type of the value to be read.
- Parameters:
-
[in] propertyId The numerical ID of the property to be read. [in,out] value Pointer to a place to store the value read from the property.
- Returns:
- Status code indicating success or failure of this operation.
const SensorInfo* openni::Device::getSensorInfo | ( | SensorType | sensorType | ) | [inline] |
Get the SensorInfo for a specific sensor type on this device. The SensorInfo is useful primarily for determining which video modes are supported by the sensor.
- Parameters:
-
[in] sensorType of sensor to get information about.
- Returns:
- SensorInfo object corresponding to the sensor type specified, or NULL if such a sensor is not available from this device.
bool openni::Device::hasSensor | ( | SensorType | sensorType | ) | [inline] |
This function checks to see if one of the specific sensor types defined in SensorType is available on this device. This allows an application to, for example, query for the presence of a depth sensor, or color sensor.
- Parameters:
-
[in] sensorType of sensor to query for
- Returns:
- true if the Device supports the sensor queried, false otherwise.
Status openni::Device::invoke | ( | int | commandId, |
void * | data, | ||
int | dataSize | ||
) | [inline] |
Invokes a command that takes an arbitrary data type as its input. It is not expected that application code will need this function frequently, as all commonly used properties have higher level functions provided.
- Parameters:
-
[in] commandId Numerical code of the property to be invoked. [in] data Data to be passed to the property. [in] dataSize size of the buffer passed in data
.
- Returns:
- Status code indicating success or failure of this operation.
Status openni::Device::invoke | ( | int | propertyId, |
T & | value | ||
) | [inline] |
Invokes a command that takes an arbitrary data type as its input. It is not expected that application code will need this function frequently, as all commonly used properties have higher level functions provided.
- Template Parameters:
-
[in] T Type of data to be passed to the property.
- Parameters:
-
[in] propertyId Numerical code of the property to be invoked. [in] value Data to be passed to the property.
- Returns:
- Status code indicating success or failure of this operation.
bool openni::Device::isCommandSupported | ( | int | commandId | ) | const [inline] |
Checks if a specific command is supported by the device.
- Parameters:
-
[in] commandId Command to be checked.
- Returns:
- true if the command is supported, false otherwise.
bool openni::Device::isFile | ( | ) | const [inline] |
Checks whether this device is a file device (i.e. a recording).
- Returns:
- true if this is a file device, false otherwise.
bool openni::Device::isImageRegistrationModeSupported | ( | ImageRegistrationMode | mode | ) | const [inline] |
Checks to see if this device can support registration of color video and depth video. Image registration is used to properly superimpose two images from cameras located at different points in space. Please see the OpenNi 2.0 Programmer's Guide for more information about registration.
- Returns:
- true if image registration is supported by this device, false otherwise.
bool openni::Device::isPropertySupported | ( | int | propertyId | ) | const [inline] |
Checks if a specific property is supported by the device.
- Parameters:
-
[in] propertyId Property to be checked.
- Returns:
- true if the property is supported, false otherwise.
bool openni::Device::isValid | ( | ) | const [inline] |
Status openni::Device::open | ( | const char * | uri | ) | [inline] |
Opens a device. This can either open a device chosen arbitrarily from all devices on the system, or open a specific device selected by passing this function the device URI.
To open any device, simply pass the constantANY_DEVICE to this function. If multiple devices are connected to the system, then one of them will be opened. This procedure is most useful when it is known that exactly one device is (or can be) connected to the system. In that case, requesting a list of all devices and iterating through it would be a waste of effort.
If multiple devices are (or may be) connected to a system, then a URI will be required to select a specific device to open. There are two ways to obtain a URI: from a DeviceConnected event, or by calling OpenNI::enumerateDevices().
In the case of a DeviceConnected event, the OpenNI::Listener will be provided with a DeviceInfo object as an argument to its onDeviceConnected() function. The DeviceInfo.getUri() function can then be used to obtain the URI.
If the application is not using event handlers, then it can also call the static function OpenNI::enumerateDevices(). This will return an array of DeviceInfo objects, one for each device currently available to the system. The application can then iterate through this list and select the desired device. The URI is again obtained via the DeviceInfo::getUri() function.
Standard codes of type Status are returned indicating whether opening was successful.
- Parameters:
-
[in] uri String containing the URI of the device to be opened, or ANY_DEVICE.
- Returns:
- Status code with the outcome of the open operation.
- Remarks:
- For opening a recording file, pass the file path as a uri.
Status openni::Device::setDepthColorSyncEnabled | ( | bool | isEnabled | ) | [inline] |
Used to turn the depth/color frame synchronization feature on and off. When frame synchronization is enabled, the device will deliver depth and image frames that are separated in time by some maximum value. When disabled, the phase difference between depth and image frame generation cannot be guaranteed.
- Parameters:
-
[in] isEnabled Set to TRUE to enable synchronization, FALSE to disable it
- Returns:
- Status code indicating success or failure of this operation
Status openni::Device::setImageRegistrationMode | ( | ImageRegistrationMode | mode | ) | [inline] |
Sets the image registration on this device. Image registration is used to properly superimpose two images from cameras located at different points in space. Please see the OpenNi 2.0 Programmer's Guide for more information about registration.
See ImageRegistrationMode for a list of valid settings to pass to this function.
It is a good practice to first check if the mode is supported by calling isImageRegistrationModeSupported().
- Parameters:
-
[in] mode Desired new value for the image registration mode.
- Returns:
- Status code for the operation.
Status openni::Device::setProperty | ( | int | propertyId, |
const void * | data, | ||
int | dataSize | ||
) | [inline] |
Sets the value of a general property of the device. There are convenience functions for all the commonly used properties, such as image registration and frame synchronization. It is expected for this reason that this function will rarely be directly used by applications.
- Parameters:
-
[in] propertyId The numerical ID of the property to be set. [in] data Place to store the data to be written to the property. [in] dataSize Size of the data to be written to the property.
- Returns:
- Status code indicating results of this operation.
Status openni::Device::setProperty | ( | int | propertyId, |
const T & | value | ||
) | [inline] |
Sets a property that takes an arbitrary data type as its input. It is not expected that application code will need this function frequently, as all commonly used properties have higher level functions provided.
- Template Parameters:
-
T Type of data to be passed to the property.
- Parameters:
-
[in] propertyId The numerical ID of the property to be set. [in] value Place to store the data to be written to the property.
- Returns:
- Status code indicating success or failure of this operation.
The documentation for this class was generated from the following file:
Generated on Tue Nov 12 2013 16:10:45 for OpenNI 2.0 by
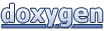