![]() |
CC3200 Peripheral Driver Library User's Guide
1.2.0
|
Functions | |
void | SPIEnable (unsigned long ulBase) |
void | SPIDisable (unsigned long ulBase) |
void | SPIDmaEnable (unsigned long ulBase, unsigned long ulFlags) |
void | SPIDmaDisable (unsigned long ulBase, unsigned long ulFlags) |
void | SPIReset (unsigned long ulBase) |
void | SPIConfigSetExpClk (unsigned long ulBase, unsigned long ulSPIClk, unsigned long ulBitRate, unsigned long ulMode, unsigned long ulSubMode, unsigned long ulConfig) |
long | SPIDataGetNonBlocking (unsigned long ulBase, unsigned long *pulData) |
void | SPIDataGet (unsigned long ulBase, unsigned long *pulData) |
long | SPIDataPutNonBlocking (unsigned long ulBase, unsigned long ulData) |
void | SPIDataPut (unsigned long ulBase, unsigned long ulData) |
void | SPIFIFOEnable (unsigned long ulBase, unsigned long ulFlags) |
void | SPIFIFODisable (unsigned long ulBase, unsigned long ulFlags) |
void | SPIFIFOLevelSet (unsigned long ulBase, unsigned long ulTxLevel, unsigned long ulRxLevel) |
void | SPIFIFOLevelGet (unsigned long ulBase, unsigned long *pulTxLevel, unsigned long *pulRxLevel) |
void | SPIWordCountSet (unsigned long ulBase, unsigned long ulWordCount) |
void | SPIIntRegister (unsigned long ulBase, void(*pfnHandler)(void)) |
void | SPIIntUnregister (unsigned long ulBase) |
void | SPIIntEnable (unsigned long ulBase, unsigned long ulIntFlags) |
void | SPIIntDisable (unsigned long ulBase, unsigned long ulIntFlags) |
unsigned long | SPIIntStatus (unsigned long ulBase, tBoolean bMasked) |
void | SPIIntClear (unsigned long ulBase, unsigned long ulIntFlags) |
void | SPICSEnable (unsigned long ulBase) |
void | SPICSDisable (unsigned long ulBase) |
long | SPITransfer (unsigned long ulBase, unsigned char *ucDout, unsigned char *ucDin, unsigned long ulCount, unsigned long ulFlags) |
Detailed Description
Function Documentation
void SPIConfigSetExpClk | ( | unsigned long | ulBase, |
unsigned long | ulSPIClk, | ||
unsigned long | ulBitRate, | ||
unsigned long | ulMode, | ||
unsigned long | ulSubMode, | ||
unsigned long | ulConfig | ||
) |
Sets the configuration of a SPI module
- Parameters
-
ulBase is the base address of the SPI module ulSPIClk is the rate of clock supplied to the SPI module. ulBitRate is the desired bit rate.(master mode) ulMode is the mode of operation. ulSubMode is one of the valid sub-modes. ulConfig is logical OR of configuration paramaters.
This function configures SPI port for operation in specified sub-mode and required bit rated as specified by ulMode and ulBitRate parameters respectively.
The SPI module can operate in either master or slave mode. The parameter ulMode can be one of the following -SPI_MODE_MASTER -SPI_MODE_SLAVE
The SPI module supports 4 sub modes based on SPI clock polarity and phase.
Polarity Phase Sub-Mode 0 0 0 0 1 1 1 0 2 1 1 3
Required sub mode can be select by setting ulSubMode parameter to one of the following
- SPI_SUB_MODE_0
- SPI_SUB_MODE_1
- SPI_SUB_MODE_2
- SPI_SUB_MODE_3
The parameter ulConfig is logical OR of five values: the word length, active level for chip select, software or hardware controled chip select, 3 or 4 pin mode and turbo mode. mode.
SPI support 8, 16 and 32 bit word lengths defined by:-
- SPI_WL_8
- SPI_WL_16
- SPI_WL_32
Active state of Chip[ Selece can be defined by:-
- SPI_CS_ACTIVELOW
- SPI_CS_ACTIVEHIGH
SPI chip select can be configured to be controlled either by hardware or software:-
- SPI_SW_CS
- SPI_HW_CS
The module can work in 3 or 4 pin mode defined by:-
- SPI_3PIN_MODE
- SPI_4PIN_MODE
Turbo mode can be set on or turned off using:-
- SPI_TURBO_MODE_ON
- SPI_TURBO_MODE_OFF
- Returns
- None.
void SPICSDisable | ( | unsigned long | ulBase | ) |
Disables the chip select in software controlled mode
- Parameters
-
ulBase is the base address of the SPI module.
This function disables the Chip select in software controlled mode. The active state of CS will depend on the configuration done via sa SPIConfigSetExpClk().
- Returns
- None.
void SPICSEnable | ( | unsigned long | ulBase | ) |
Enables the chip select in software controlled mode
- Parameters
-
ulBase is the base address of the SPI module.
This function enables the Chip select in software controlled mode. The active state of CS will depend on the configuration done via
- See also
- SPIConfigExpClkSet().
- Returns
- None.
void SPIDataGet | ( | unsigned long | ulBase, |
unsigned long * | pulData | ||
) |
Waits for the word to be received on the specified port.
- Parameters
-
ulBase is the base address of the SPI module. pulData is pointer to receive data variable.
This function gets a SPI word from the receive FIFO for the specified port. If there is no word available, this function waits until a word is received before returning.
- Returns
- Returns the word read from the specified port, cast as an unsigned long.
long SPIDataGetNonBlocking | ( | unsigned long | ulBase, |
unsigned long * | pulData | ||
) |
Receives a word from the specified port.
- Parameters
-
ulBase is the base address of the SPI module. pulData is pointer to receive data variable.
This function gets a SPI word from the receive FIFO for the specified port.
- Returns
- Returns the number of elements read from the receive FIFO.
void SPIDataPut | ( | unsigned long | ulBase, |
unsigned long | ulData | ||
) |
Waits until the word is transmitted on the specified port.
- Parameters
-
ulBase is the base address of the SPI module ulData is data to be transmitted.
This function transmits a SPI word on the transmit FIFO for the specified port. This function waits until the space is available on transmit FIFO
- Returns
- None
long SPIDataPutNonBlocking | ( | unsigned long | ulBase, |
unsigned long | ulData | ||
) |
Transmits a word on the specified port.
- Parameters
-
ulBase is the base address of the SPI module ulData is data to be transmitted.
This function transmits a SPI word on the transmit FIFO for the specified port.
- Returns
- Returns the number of elements written to the transmit FIFO.
void SPIDisable | ( | unsigned long | ulBase | ) |
Disables the transmitting and receiving.
- Parameters
-
ulBase is the base address of the SPI module
This function disables the SPI channel for transmitting and receiving.
- Returns
- None
void SPIDmaDisable | ( | unsigned long | ulBase, |
unsigned long | ulFlags | ||
) |
Disables the SPI DMA operation for transmitting and/or receving.
- Parameters
-
ulBase is the base address of the SPI module ulFlags selectes the DMA signal for transmit and/or receive.
This function disables transmit and/or receive DMA request based on the ulFlags parameter.
The parameter ulFlags is the logical OR of one or more of the following :
- SPI_RX_DMA
- SPI_TX_DMA
- Returns
- None.
void SPIDmaEnable | ( | unsigned long | ulBase, |
unsigned long | ulFlags | ||
) |
Enables the SPI DMA operation for transmitting and/or receving.
- Parameters
-
ulBase is the base address of the SPI module ulFlags selectes the DMA signal for transmit and/or receive.
This function enables transmit and/or receive DMA request based on the ulFlags parameter.
The parameter ulFlags is the logical OR of one or more of the following :
- SPI_RX_DMA
- SPI_TX_DMA
- Returns
- None.
void SPIEnable | ( | unsigned long | ulBase | ) |
Enables transmitting and receiving.
- Parameters
-
ulBase is the base address of the SPI module
This function enables the SPI channel for transmitting and receiving.
- Returns
- None
void SPIFIFODisable | ( | unsigned long | ulBase, |
unsigned long | ulFlags | ||
) |
Disables the transmit and/or receive FIFOs.
- Parameters
-
ulBase is the base address of the SPI module ulFlags selects the FIFO(s) to be enabled
This function disables transmit and/or receive FIFOs. as specified by ulFlags. The parameter ulFlags shoulde be logical OR of one or more of the following:
- SPI_TX_FIFO
- SPI_RX_FIFO
- Returns
- None.
void SPIFIFOEnable | ( | unsigned long | ulBase, |
unsigned long | ulFlags | ||
) |
Enables the transmit and/or receive FIFOs.
- Parameters
-
ulBase is the base address of the SPI module ulFlags selects the FIFO(s) to be enabled
This function enables the transmit and/or receive FIFOs as specified by ulFlags. The parameter ulFlags shoulde be logical OR of one or more of the following:
- SPI_TX_FIFO
- SPI_RX_FIFO
- Returns
- None.
void SPIFIFOLevelGet | ( | unsigned long | ulBase, |
unsigned long * | pulTxLevel, | ||
unsigned long * | pulRxLevel | ||
) |
Gets the FIFO level at which DMA requests or interrupts are generated.
- Parameters
-
ulBase is the base address of the SPI module pulTxLevel is a pointer to storage for the transmit FIFO level pulRxLevel is a pointer to storage for the receive FIFO level
This function gets the FIFO level at which DMA requests or interrupts are generated.
- Returns
- None.
void SPIFIFOLevelSet | ( | unsigned long | ulBase, |
unsigned long | ulTxLevel, | ||
unsigned long | ulRxLevel | ||
) |
Sets the FIFO level at which DMA requests or interrupts are generated.
- Parameters
-
ulBase is the base address of the SPI module ulTxLevel is the Almost Empty Level for transmit FIFO. ulRxLevel is the Almost Full Level for the receive FIFO.
This function Sets the FIFO level at which DMA requests or interrupts are generated.
- Returns
- None.
void SPIIntClear | ( | unsigned long | ulBase, |
unsigned long | ulIntFlags | ||
) |
Clears SPI interrupt sources.
- Parameters
-
ulBase is the base address of the SPI module ulIntFlags is a bit mask of the interrupt sources to be cleared.
The specified SPI interrupt sources are cleared, so that they no longer assert. This function must be called in the interrupt handler to keep the interrupt from being recognized again immediately upon exit.
The ulIntFlags parameter has the same definition as the ulIntFlags parameter to SPIIntEnable().
- Returns
- None.
void SPIIntDisable | ( | unsigned long | ulBase, |
unsigned long | ulIntFlags | ||
) |
Disables individual SPI interrupt sources.
- Parameters
-
ulBase is the base address of the SPI module ulIntFlags is the bit mask of the interrupt sources to be disabled.
This function disables the indicated SPI interrupt sources. Only the sources that are enabled can be reflected to the processor interrupt; disabled sources have no effect on the processor.
The ulIntFlags parameter has the same definition as the ulIntFlags parameter to SPIIntEnable().
- Returns
- None.
void SPIIntEnable | ( | unsigned long | ulBase, |
unsigned long | ulIntFlags | ||
) |
Enables individual SPI interrupt sources.
- Parameters
-
ulBase is the base address of the SPI module ulIntFlags is the bit mask of the interrupt sources to be enabled.
This function enables the indicated SPI interrupt sources. Only the sources that are enabled can be reflected to the processor interrupt; disabled sources have no effect on the processor.
The ulIntFlags parameter is the logical OR of any of the following:
- SPI_INT_DMATX
- SPI_INT_DMARX
- SPI_INT_EOW
- SPI_INT_RX_OVRFLOW
- SPI_INT_RX_FULL
- SPI_INT_TX_UDRFLOW
- SPI_INT_TX_EMPTY
- Returns
- None.
void SPIIntRegister | ( | unsigned long | ulBase, |
void(*)(void) | pfnHandler | ||
) |
Registers an interrupt handler for a SPI interrupt.
- Parameters
-
ulBase is the base address of the SPI module pfnHandler is a pointer to the function to be called when the SPI interrupt occurs.
This function does the actual registering of the interrupt handler. This function enables the global interrupt in the interrupt controller; specific SPI interrupts must be enabled via SPIIntEnable(). It is the interrupt handler's responsibility to clear the interrupt source.
- See also
- IntRegister() for important information about registering interrupt handlers.
- Returns
- None.
unsigned long SPIIntStatus | ( | unsigned long | ulBase, |
tBoolean | bMasked | ||
) |
Gets the current interrupt status.
- Parameters
-
ulBase is the base address of the SPI module bMasked is false if the raw interrupt status is required and true if the masked interrupt status is required.
This function returns the interrupt status for the specified SPI. The status of interrupts that are allowed to reflect to the processor can be returned.
- Returns
- Returns the current interrupt status, enumerated as a bit field of values described in SPIIntEnable().
void SPIIntUnregister | ( | unsigned long | ulBase | ) |
Unregisters an interrupt handler for a SPI interrupt.
- Parameters
-
ulBase is the base address of the SPI module
This function does the actual unregistering of the interrupt handler. It clears the handler to be called when a SPI interrupt occurs. This function also masks off the interrupt in the interrupt controller so that the interrupt handler no longer is called.
- See also
- IntRegister() for important information about registering interrupt handlers.
- Returns
- None.
void SPIReset | ( | unsigned long | ulBase | ) |
Performs a software reset of the specified SPI module
- Parameters
-
ulBase is the base address of the SPI module
This function performs a software reset of the specified SPI module
- Returns
- None.
long SPITransfer | ( | unsigned long | ulBase, |
unsigned char * | ucDout, | ||
unsigned char * | ucDin, | ||
unsigned long | ulCount, | ||
unsigned long | ulFlags | ||
) |
Send/Receive data buffer over SPI channel
- Parameters
-
ulBase is the base address of SPI module ucDout is the pointer to Tx data buffer or 0. ucDin is pointer to Rx data buffer or 0 ulCount is the size of data in bytes. ulFlags controlls chip select toggling.
This function transfers ulCount bytes of data over SPI channel. Since the API sends a SPI word at a time ulCount should be a multiple of word length set using SPIConfigSetExpClk().
If the ucDout parameter is set to 0, the function will send 0xFF over the SPI MOSI line.
If the ucDin parameter is set to 0, the function will ignore data on SPI MISO line.
The parameter ulFlags is logical OR of one or more of the following
- SPI_CS_ENABLE if CS needs to be enabled at start of transfer.
- SPI_CS_DISABLE if CS need to be disabled at the end of transfer.
This function will not return until data has been transmitted
- Returns
- Returns 0 on success, -1 otherwise.
void SPIWordCountSet | ( | unsigned long | ulBase, |
unsigned long | ulWordCount | ||
) |
Sets the word count.
- Parameters
-
ulBase is the base address of the SPI module ulWordCount is number of SPI words to be transmitted.
This function sets the word count, which is the number of SPI word to be transferred on channel when using the FIFO buffer.
- Returns
- None.
Generated on Thu Feb 18 2016 13:22:02 for CC3200 Peripheral Driver Library User's Guide by
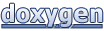