![]() |
CC3200 Peripheral Driver Library User's Guide
1.2.0
|
Functions | |
void | SHAMD5DMAEnable (uint32_t ui32Base) |
void | SHAMD5DMADisable (uint32_t ui32Base) |
uint32_t | SHAMD5IntStatus (uint32_t ui32Base, bool bMasked) |
void | SHAMD5IntEnable (uint32_t ui32Base, uint32_t ui32IntFlags) |
void | SHAMD5IntDisable (uint32_t ui32Base, uint32_t ui32IntFlags) |
void | SHAMD5IntClear (uint32_t ui32Base, uint32_t ui32IntFlags) |
void | SHAMD5IntRegister (uint32_t ui32Base, void(*pfnHandler)(void)) |
void | SHAMD5IntUnregister (uint32_t ui32Base) |
void | SHAMD5DataLengthSet (uint32_t ui32Base, uint32_t ui32Length) |
void | SHAMD5ConfigSet (uint32_t ui32Base, uint32_t ui32Mode) |
bool | SHAMD5DataWriteNonBlocking (uint32_t ui32Base, uint8_t *pui8Src) |
void | SHAMD5DataWrite (uint32_t ui32Base, uint8_t *pui8Src) |
void | SHAMD5ResultRead (uint32_t ui32Base, uint8_t *pui8Dest) |
bool | SHAMD5DataProcess (uint32_t ui32Base, uint8_t *pui8DataSrc, uint32_t ui32DataLength, uint8_t *pui8HashResult) |
bool | SHAMD5HMACProcess (uint32_t ui32Base, uint8_t *pui8DataSrc, uint32_t ui32DataLength, uint8_t *pui8HashResult) |
void | SHAMD5HMACPPKeyGenerate (uint32_t ui32Base, uint8_t *pui8Key, uint8_t *pui8PPKey) |
void | SHAMD5HMACKeySet (uint32_t ui32Base, uint8_t *pui8Src) |
void | SHAMD5HMACPPKeySet (uint32_t ui32Base, uint8_t *pui8Src) |
Detailed Description
Function Documentation
void SHAMD5ConfigSet | ( | uint32_t | ui32Base, |
uint32_t | ui32Mode | ||
) |
Writes the mode in the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. ui32Mode is the mode of the SHA/MD5 module.
This function writes the mode register configuring the SHA/MD5 module.
The ui32Mode paramerter is a bit-wise OR of values:
- SHAMD5_ALGO_MD5 - Regular hash with MD5
- SHAMD5_ALGO_SHA1 - Regular hash with SHA-1
- SHAMD5_ALGO_SHA224 - Regular hash with SHA-224
- SHAMD5_ALGO_SHA256 - Regular hash with SHA-256
- SHAMD5_ALGO_HMAC_MD5 - HMAC with MD5
- SHAMD5_ALGO_HMAC_SHA1 - HMAC with SHA-1
- SHAMD5_ALGO_HMAC_SHA224 - HMAC with SHA-224
- SHAMD5_ALGO_HMAC_SHA256 - HMAC with SHA-256
- Returns
- None
void SHAMD5DataLengthSet | ( | uint32_t | ui32Base, |
uint32_t | ui32Length | ||
) |
Write the hash length to the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. ui32Length is the hash length in bytes.
This function writes the length of the hash data of the current operation to the SHA/MD5 module. The value must be a multiple of 64 if the close hash is not set in the mode register.
- Note
- When this register is written, hash processing is triggered.
- Returns
- None.
bool SHAMD5DataProcess | ( | uint32_t | ui32Base, |
uint8_t * | pui8DataSrc, | ||
uint32_t | ui32DataLength, | ||
uint8_t * | pui8HashResult | ||
) |
Compute a hash using the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. pui8DataSrc is a pointer to an array of data that contains the data that will be hashed. ui32DataLength specifies the length of the data to be hashed in bytes. pui8HashResult is a pointer to an array that holds the result of the hashing operation.
This function computes the hash of an array of data using the SHA/MD5 module.
The length of the hash result is dependent on the algorithm that is in use. The following table shows the correct array size for each algorithm:
| Algorithm | Number of Words in Result |
| MD5 | 4 Words (128 bits) | | SHA-1 | 5 Words (160 bits) | | SHA-224 | 7 Words (224 bits) |
| SHA-256 | 8 Words (256 bits) |
- Returns
- None
void SHAMD5DataWrite | ( | uint32_t | ui32Base, |
uint8_t * | pui8Src | ||
) |
Perform a blocking write of 64 bytes of data to the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. pui8Src is the pointer to the 64-byte array of data that will be written.
This function does not return until the module is ready to accept data and the data has been written.
- Returns
- None.
bool SHAMD5DataWriteNonBlocking | ( | uint32_t | ui32Base, |
uint8_t * | pui8Src | ||
) |
Perform a non-blocking write of 16 words of data to the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. pui8Src is the pointer to the 16-word array of data that will be written.
This function writes 16 words of data into the data register.
- Returns
- This function returns true if the write completed successfully. It returns false if the module was not ready.
void SHAMD5DMADisable | ( | uint32_t | ui32Base | ) |
Disables the uDMA requests in the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module.
This function configures the DMA options of the SHA/MD5 module.
- Returns
- None
void SHAMD5DMAEnable | ( | uint32_t | ui32Base | ) |
Enables the uDMA requests in the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module.
This function configures the DMA options of the SHA/MD5 module.
- Returns
- None
void SHAMD5HMACKeySet | ( | uint32_t | ui32Base, |
uint8_t * | pui8Src | ||
) |
Writes an HMAC key to the digest registers in the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. pui8Src is the pointer to the 16-word array of the HMAC key.
This function is used to write HMAC key to the digest registers for key preprocessing. The size of pui8Src must be 512 bytes. If the key is less than 512 bytes, then it must be padded with zeros.
- Note
- It is recommended to use the SHAMD5GetIntStatus function to check whether the context is ready before writing the key.
- Returns
- None
void SHAMD5HMACPPKeyGenerate | ( | uint32_t | ui32Base, |
uint8_t * | pui8Key, | ||
uint8_t * | pui8PPKey | ||
) |
Process an HMAC key using the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. pui8Key is a pointer to an array that contains the key to be processed. pui8PPKey is the pointer to the array that contains the pre-processed key.
This function processes an HMAC key using the SHA/MD5. The resultant pre-processed key can then be used with later HMAC operations to speed processing time.
The pui8Key array must be 512 bits long. If the key is less than 512 bits, it must be padded with zeros. The pui8PPKey array must each be 512 bits long.
- Returns
- None
void SHAMD5HMACPPKeySet | ( | uint32_t | ui32Base, |
uint8_t * | pui8Src | ||
) |
Writes a pre-processed HMAC key to the digest registers in the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. pui8Src is the pointer to the 16-word array of the HMAC key.
This function is used to write HMAC key to the digest registers for key preprocessing. The size of pui8Src must be 512 bytes. If the key is less than 512 bytes, then it must be padded with zeros.
- Note
- It is recommended to use the SHAMD5GetIntStatus function to check whether the context is ready before writing the key.
- Returns
- None
bool SHAMD5HMACProcess | ( | uint32_t | ui32Base, |
uint8_t * | pui8DataSrc, | ||
uint32_t | ui32DataLength, | ||
uint8_t * | pui8HashResult | ||
) |
Compute a HMAC with key pre-processing using the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. pui8DataSrc is a pointer to an array of data that contains the data that is to be hashed. ui32DataLength specifies the length of the data to be hashed in bytes. pui8HashResult is a pointer to an array that holds the result of the hashing operation.
This function computes a HMAC with the given data using the SHA/MD5 module with a preprocessed key.
The length of the hash result is dependent on the algorithm that is selected with the ui32Algo argument. The following table shows the correct array size for each algorithm:
| Algorithm | Number of Words in Result |
| MD5 | 4 Words (128 bits) | | SHA-1 | 5 Words (160 bits) | | SHA-224 | 7 Words (224 bits) |
| SHA-256 | 8 Words (256 bits) |
- Returns
- None
void SHAMD5IntClear | ( | uint32_t | ui32Base, |
uint32_t | ui32IntFlags | ||
) |
Clears interrupt sources in the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. ui32IntFlags contains desired interrupts to disable.
ui32IntFlags must be a logical OR of one or more of the following values:
- SHAMD5_INT_CONTEXT_READY - Context input registers are ready.
- SHAMD5_INT_PARTHASH_READY - Context output registers are ready after a context switch.
- SHAMD5_INT_INPUT_READY - Data FIFO is ready to receive data.
- SHAMD5_INT_OUTPUT_READY - Context output registers are ready.
- Returns
- None.
void SHAMD5IntDisable | ( | uint32_t | ui32Base, |
uint32_t | ui32IntFlags | ||
) |
Disable interrupt sources in the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. ui32IntFlags contains desired interrupts to disable.
ui32IntFlags must be a logical OR of one or more of the following values:
- SHAMD5_INT_CONTEXT_READY - Context input registers are ready.
- SHAMD5_INT_PARTHASH_READY - Context output registers are ready after a context switch.
- SHAMD5_INT_INPUT_READY - Data FIFO is ready to receive data.
- SHAMD5_INT_OUTPUT_READY - Context output registers are ready.
- Returns
- None.
void SHAMD5IntEnable | ( | uint32_t | ui32Base, |
uint32_t | ui32IntFlags | ||
) |
Enable interrupt sources in the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. ui32IntFlags contains desired interrupts to enable.
This function enables interrupt sources in the SHA/MD5 module. ui32IntFlags must be a logical OR of one or more of the following values:
- SHAMD5_INT_CONTEXT_READY - Context input registers are ready.
- SHAMD5_INT_PARTHASH_READY - Context output registers are ready after a context switch.
- SHAMD5_INT_INPUT_READY - Data FIFO is ready to receive data.
- SHAMD5_INT_OUTPUT_READY - Context output registers are ready.
- Returns
- None.
void SHAMD5IntRegister | ( | uint32_t | ui32Base, |
void(*)(void) | pfnHandler | ||
) |
Registers an interrupt handler for the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. pfnHandler is a pointer to the function to be called when the enabled SHA/MD5 interrupts occur.
This function registers the interrupt handler in the interrupt vector table, and enables SHA/MD5 interrupts on the interrupt controller; specific SHA/MD5 interrupt sources must be enabled using SHAMD5IntEnable(). The interrupt handler being registered must clear the source of the interrupt using SHAMD5IntClear().
If the application is using a static interrupt vector table stored in flash, then it is not necessary to register the interrupt handler this way. Instead, IntEnable() should be used to enable SHA/MD5 interrupts on the interrupt controller.
- See also
- IntRegister() for important information about registering interrupt handlers.
- Returns
- None.
uint32_t SHAMD5IntStatus | ( | uint32_t | ui32Base, |
bool | bMasked | ||
) |
Get the interrupt status of the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. bMasked is false if the raw interrupt status is required and true if the masked interrupt status is required.
This function returns the current value of the IRQSTATUS register. The value will be a logical OR of the following:
- SHAMD5_INT_CONTEXT_READY - Context input registers are ready.
- SHAMD5_INT_PARTHASH_READY - Context output registers are ready after a context switch.
- SHAMD5_INT_INPUT_READY - Data FIFO is ready to receive data.
- SHAMD5_INT_OUTPUT_READY - Context output registers are ready.
- Returns
- Interrupt status
void SHAMD5IntUnregister | ( | uint32_t | ui32Base | ) |
Unregisters an interrupt handler for the SHA/MD5 module.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module.
This function unregisters the previously registered interrupt handler and disables the interrupt in the interrupt controller.
- See also
- IntRegister() for important information about registering interrupt handlers.
- Returns
- None.
void SHAMD5ResultRead | ( | uint32_t | ui32Base, |
uint8_t * | pui8Dest | ||
) |
Reads the result of a hashing operation.
- Parameters
-
ui32Base is the base address of the SHA/MD5 module. pui8Dest is the pointer to the byte array of data that will be written.
This function does not return until the module is ready to accept data and
the data has been written.
| Algorithm | Number of Words in Result |
| MD5 | 16 Bytes (128 bits) | | SHA-1 | 20 Bytes (160 bits) | | SHA-224 | 28 Bytes (224 bits) |
| SHA-256 | 32 Bytes (256 bits) |
- Returns
- None.
Generated on Thu Feb 18 2016 13:22:02 for CC3200 Peripheral Driver Library User's Guide by
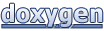