![]() |
CC3200 Peripheral Driver Library User's Guide
1.2.0
|
Functions | |
void | GPIODirModeSet (unsigned long ulPort, unsigned char ucPins, unsigned long ulPinIO) |
unsigned long | GPIODirModeGet (unsigned long ulPort, unsigned char ucPin) |
void | GPIOIntTypeSet (unsigned long ulPort, unsigned char ucPins, unsigned long ulIntType) |
unsigned long | GPIOIntTypeGet (unsigned long ulPort, unsigned char ucPin) |
void | GPIOIntEnable (unsigned long ulPort, unsigned long ulIntFlags) |
void | GPIOIntDisable (unsigned long ulPort, unsigned long ulIntFlags) |
long | GPIOIntStatus (unsigned long ulPort, tBoolean bMasked) |
void | GPIOIntClear (unsigned long ulPort, unsigned long ulIntFlags) |
void | GPIOIntRegister (unsigned long ulPort, void(*pfnIntHandler)(void)) |
void | GPIOIntUnregister (unsigned long ulPort) |
long | GPIOPinRead (unsigned long ulPort, unsigned char ucPins) |
void | GPIOPinWrite (unsigned long ulPort, unsigned char ucPins, unsigned char ucVal) |
void | GPIODMATriggerEnable (unsigned long ulPort) |
void | GPIODMATriggerDisable (unsigned long ulPort) |
Detailed Description
Function Documentation
unsigned long GPIODirModeGet | ( | unsigned long | ulPort, |
unsigned char | ucPin | ||
) |
Gets the direction and mode of a pin.
- Parameters
-
ulPort is the base address of the GPIO port. ucPin is the pin number.
This function gets the direction and control mode for a specified pin on the selected GPIO port. The pin can be configured as either an input or output under software control, or it can be under hardware control. The type of control and direction are returned as an enumerated data type.
- Returns
- Returns one of the enumerated data types described for GPIODirModeSet().
void GPIODirModeSet | ( | unsigned long | ulPort, |
unsigned char | ucPins, | ||
unsigned long | ulPinIO | ||
) |
Sets the direction and mode of the specified pin(s).
- Parameters
-
ulPort is the base address of the GPIO port ucPins is the bit-packed representation of the pin(s). ulPinIO is the pin direction and/or mode.
This function will set the specified pin(s) on the selected GPIO port as either an input or output under software control, or it will set the pin to be under hardware control.
The parameter ulPinIO is an enumerated data type that can be one of the following values:
- GPIO_DIR_MODE_IN
- GPIO_DIR_MODE_OUT
where GPIO_DIR_MODE_IN specifies that the pin will be programmed as a software controlled input, GPIO_DIR_MODE_OUT specifies that the pin will be programmed as a software controlled output.
The pin(s) are specified using a bit-packed byte, where each bit that is set identifies the pin to be accessed, and where bit 0 of the byte represents GPIO port pin 0, bit 1 represents GPIO port pin 1, and so on.
- Note
- GPIOPadConfigSet() must also be used to configure the corresponding pad(s) in order for them to propagate the signal to/from the GPIO.
- Returns
- None.
void GPIODMATriggerDisable | ( | unsigned long | ulPort | ) |
Disables a GPIO port as a trigger to start a DMA transaction.
- Parameters
-
ulPort is the base address of the GPIO port.
This function disables a GPIO port to be used as a trigger to start a uDMA transaction. This function can be used to disable this feature if it was enabled via a call to GPIODMATriggerEnable().
- Returns
- None.
void GPIODMATriggerEnable | ( | unsigned long | ulPort | ) |
Enables a GPIO port as a trigger to start a DMA transaction.
- Parameters
-
ulPort is the base address of the GPIO port.
This function enables a GPIO port to be used as a trigger to start a uDMA transaction. The GPIO pin will still generate interrupts if the interrupt is enabled for the selected pin.
- Returns
- None.
void GPIOIntClear | ( | unsigned long | ulPort, |
unsigned long | ulIntFlags | ||
) |
Clears the interrupt for the specified pin(s).
- Parameters
-
ulPort is the base address of the GPIO port. ulIntFlags is a bit mask of the interrupt sources to be cleared.
Clears the interrupt for the specified pin(s).
The ulIntFlags parameter has the same definition as the ulIntFlags parameter to GPIOIntEnable().
- Returns
- None.
void GPIOIntDisable | ( | unsigned long | ulPort, |
unsigned long | ulIntFlags | ||
) |
Disables the specified GPIO interrupts.
- Parameters
-
ulPort is the base address of the GPIO port. ulIntFlags is the bit mask of the interrupt sources to disable.
This function disables the indicated GPIO interrupt sources. Only the sources that are enabled can be reflected to the processor interrupt; disabled sources have no effect on the processor.
The ulIntFlags parameter is the logical OR of any of the following:
- GPIO_INT_DMA - interrupt due to GPIO triggered DMA Done
- GPIO_INT_PIN_0 - interrupt due to activity on Pin 0.
- GPIO_INT_PIN_1 - interrupt due to activity on Pin 1.
- GPIO_INT_PIN_2 - interrupt due to activity on Pin 2.
- GPIO_INT_PIN_3 - interrupt due to activity on Pin 3.
- GPIO_INT_PIN_4 - interrupt due to activity on Pin 4.
- GPIO_INT_PIN_5 - interrupt due to activity on Pin 5.
- GPIO_INT_PIN_6 - interrupt due to activity on Pin 6.
- GPIO_INT_PIN_7 - interrupt due to activity on Pin 7.
- Returns
- None.
void GPIOIntEnable | ( | unsigned long | ulPort, |
unsigned long | ulIntFlags | ||
) |
Enables the specified GPIO interrupts.
- Parameters
-
ulPort is the base address of the GPIO port. ulIntFlags is the bit mask of the interrupt sources to enable.
This function enables the indicated GPIO interrupt sources. Only the sources that are enabled can be reflected to the processor interrupt; disabled sources have no effect on the processor.
The ulIntFlags parameter is the logical OR of any of the following:
- GPIO_INT_DMA - interrupt due to GPIO triggered DMA Done
- GPIO_INT_PIN_0 - interrupt due to activity on Pin 0.
- GPIO_INT_PIN_1 - interrupt due to activity on Pin 1.
- GPIO_INT_PIN_2 - interrupt due to activity on Pin 2.
- GPIO_INT_PIN_3 - interrupt due to activity on Pin 3.
- GPIO_INT_PIN_4 - interrupt due to activity on Pin 4.
- GPIO_INT_PIN_5 - interrupt due to activity on Pin 5.
- GPIO_INT_PIN_6 - interrupt due to activity on Pin 6.
- GPIO_INT_PIN_7 - interrupt due to activity on Pin 7.
- Returns
- None.
void GPIOIntRegister | ( | unsigned long | ulPort, |
void(*)(void) | pfnIntHandler | ||
) |
Registers an interrupt handler for a GPIO port.
- Parameters
-
ulPort is the base address of the GPIO port. pfnIntHandler is a pointer to the GPIO port interrupt handling function.
This function will ensure that the interrupt handler specified by pfnIntHandler is called when an interrupt is detected from the selected GPIO port. This function will also enable the corresponding GPIO interrupt in the interrupt controller; individual pin interrupts and interrupt sources must be enabled with GPIOIntEnable().
- See also
- IntRegister() for important information about registering interrupt handlers.
- Returns
- None.
long GPIOIntStatus | ( | unsigned long | ulPort, |
tBoolean | bMasked | ||
) |
Gets interrupt status for the specified GPIO port.
- Parameters
-
ulPort is the base address of the GPIO port. bMasked specifies whether masked or raw interrupt status is returned.
If bMasked is set as true, then the masked interrupt status is returned; otherwise, the raw interrupt status will be returned.
- Returns
- Returns the current interrupt status, enumerated as a bit field of values described in GPIOIntEnable().
unsigned long GPIOIntTypeGet | ( | unsigned long | ulPort, |
unsigned char | ucPin | ||
) |
Gets the interrupt type for a pin.
- Parameters
-
ulPort is the base address of the GPIO port. ucPin is the pin number.
This function gets the interrupt type for a specified pin on the selected GPIO port. The pin can be configured as a falling edge, rising edge, or both edge detected interrupt, or it can be configured as a low level or high level detected interrupt. The type of interrupt detection mechanism is returned as an enumerated data type.
- Returns
- Returns one of the enumerated data types described for GPIOIntTypeSet().
void GPIOIntTypeSet | ( | unsigned long | ulPort, |
unsigned char | ucPins, | ||
unsigned long | ulIntType | ||
) |
Sets the interrupt type for the specified pin(s).
- Parameters
-
ulPort is the base address of the GPIO port. ucPins is the bit-packed representation of the pin(s). ulIntType specifies the type of interrupt trigger mechanism.
This function sets up the various interrupt trigger mechanisms for the specified pin(s) on the selected GPIO port.
The parameter ulIntType is an enumerated data type that can be one of the following values:
- GPIO_FALLING_EDGE
- GPIO_RISING_EDGE
- GPIO_BOTH_EDGES
- GPIO_LOW_LEVEL
- GPIO_HIGH_LEVEL
The pin(s) are specified using a bit-packed byte, where each bit that is set identifies the pin to be accessed, and where bit 0 of the byte represents GPIO port pin 0, bit 1 represents GPIO port pin 1, and so on.
- Note
- In order to avoid any spurious interrupts, the user must ensure that the GPIO inputs remain stable for the duration of this function.
- Returns
- None.
void GPIOIntUnregister | ( | unsigned long | ulPort | ) |
Removes an interrupt handler for a GPIO port.
- Parameters
-
ulPort is the base address of the GPIO port.
This function will unregister the interrupt handler for the specified GPIO port. This function will also disable the corresponding GPIO port interrupt in the interrupt controller; individual GPIO interrupts and interrupt sources must be disabled with GPIOIntDisable().
- See also
- IntRegister() for important information about registering interrupt handlers.
- Returns
- None.
long GPIOPinRead | ( | unsigned long | ulPort, |
unsigned char | ucPins | ||
) |
Reads the values present of the specified pin(s).
- Parameters
-
ulPort is the base address of the GPIO port. ucPins is the bit-packed representation of the pin(s).
The values at the specified pin(s) are read, as specified by ucPins. Values are returned for both input and output pin(s), and the value for pin(s) that are not specified by ucPins are set to 0.
The pin(s) are specified using a bit-packed byte, where each bit that is set identifies the pin to be accessed, and where bit 0 of the byte represents GPIO port pin 0, bit 1 represents GPIO port pin 1, and so on.
- Returns
- Returns a bit-packed byte providing the state of the specified pin, where bit 0 of the byte represents GPIO port pin 0, bit 1 represents GPIO port pin 1, and so on. Any bit that is not specified by ucPins is returned as a 0. Bits 31:8 should be ignored.
void GPIOPinWrite | ( | unsigned long | ulPort, |
unsigned char | ucPins, | ||
unsigned char | ucVal | ||
) |
Writes a value to the specified pin(s).
- Parameters
-
ulPort is the base address of the GPIO port. ucPins is the bit-packed representation of the pin(s). ucVal is the value to write to the pin(s).
Writes the corresponding bit values to the output pin(s) specified by ucPins. Writing to a pin configured as an input pin has no effect.
The pin(s) are specified using a bit-packed byte, where each bit that is set identifies the pin to be accessed, and where bit 0 of the byte represents GPIO port pin 0, bit 1 represents GPIO port pin 1, and so on.
- Returns
- None.
Generated on Thu Feb 18 2016 13:22:02 for CC3200 Peripheral Driver Library User's Guide by
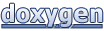