![]() |
BlueNRG-MS pack for STM32CubeMX
V4.4.0
The BlueNRG-MS pack is an additional software for STM32CubeMX.
|
#include "bluenrg_types.h"
#include "bluenrg_def.h"
#include "bluenrg_aci.h"
#include "bluenrg_utils.h"
#include "hci.h"
#include "hci_le.h"
#include "string.h"
Macros | |
#define | SUPPORTED_BOOTLOADER_VERSION_MIN 3 |
#define | SUPPORTED_BOOTLOADER_VERSION_MAX 5 |
#define | BASE_ADDRESS 0x10010000 |
#define | FW_OFFSET (2*1024) |
#define | FW_OFFSET_MS 0 |
#define | FULL_STACK_SIZE (66*1024) |
#define | BOOTLOADER_SIZE (2*1024) |
#define | SECTOR_SIZE (2*1024) |
#define | CRC_POLY 0x04C11DB7 |
#define | BOOTLOADER_CRC_NOT_PATCHED 0x878FB3FC |
#define | IFR_SIZE 192 |
#define | IFR_BASE_ADDRESS 0x10020000 |
#define | IFR_CONFIG_DATA_OFFSET (SECTOR_SIZE-IFR_SIZE) |
#define | IFR_WRITE_OFFSET_BEGIN 0 |
#define | BLUE_FLAG_OFFSET 0x8C0 |
#define | MAX_ERASE_RETRIES 2 |
#define | MAX_WRITE_RETRIES 2 |
#define | MIN_WRITE_BLOCK_SIZE 4 |
#define | MAX_WRITE_BLOCK_SIZE 64 |
#define | READ_BLOCK_SIZE 64 |
#define | RETRY_COMMAND(func, num_ret, error) |
#define | MIN(a, b) (((a)<(b))?(a):(b)) |
Functions | |
int | program_device (const uint8_t *fw_image, uint32_t fw_size) |
Flash a new firmware using internal bootloader. More... | |
int | read_IFR (uint8_t *data) |
void | parse_IFR_data_config (const uint8_t data[64], IFR_config2_TypeDef *IFR_config) |
Parse IFR raw data. More... | |
int | IFR_validate (IFR_config2_TypeDef *IFR_config) |
Check for the correctness of parsed data. More... | |
void | change_IFR_data_config (IFR_config2_TypeDef *IFR_config, uint8_t data[64]) |
Modify IFR data. (Last 64-bytes block). More... | |
int | program_IFR (const IFR_config_TypeDef *ifr_image) |
Program raw data to IFR (3 64-bytes blocks). More... | |
uint8_t | verify_IFR (const IFR_config_TypeDef *ifr_data) |
Verify raw data from IFR (3 64-bytes blocks). More... | |
uint8_t | getBlueNRGVersion (uint8_t *hwVersion, uint16_t *fwVersion) |
Get BlueNRG hardware and firmware version. More... | |
uint8_t | getBlueNRGUpdaterVersion (uint8_t *version) |
Get BlueNRG updater version. More... | |
uint8_t | isHWBootloader_Patched (void) |
Verifies if the bootloader is patched or not. This function shall be used to fix a bug on the HW bootloader related to the 32 MHz external crystal oscillator. More... | |
Macro Definition Documentation
◆ BASE_ADDRESS
#define BASE_ADDRESS 0x10010000 |
◆ BLUE_FLAG_OFFSET
#define BLUE_FLAG_OFFSET 0x8C0 |
◆ BOOTLOADER_CRC_NOT_PATCHED
#define BOOTLOADER_CRC_NOT_PATCHED 0x878FB3FC |
◆ BOOTLOADER_SIZE
#define BOOTLOADER_SIZE (2*1024) |
◆ CRC_POLY
#define CRC_POLY 0x04C11DB7 |
◆ FULL_STACK_SIZE
#define FULL_STACK_SIZE (66*1024) |
◆ FW_OFFSET
#define FW_OFFSET (2*1024) |
◆ FW_OFFSET_MS
#define FW_OFFSET_MS 0 |
◆ IFR_BASE_ADDRESS
#define IFR_BASE_ADDRESS 0x10020000 |
◆ IFR_CONFIG_DATA_OFFSET
#define IFR_CONFIG_DATA_OFFSET (SECTOR_SIZE-IFR_SIZE) |
◆ IFR_SIZE
#define IFR_SIZE 192 |
◆ IFR_WRITE_OFFSET_BEGIN
#define IFR_WRITE_OFFSET_BEGIN 0 |
◆ MAX_ERASE_RETRIES
#define MAX_ERASE_RETRIES 2 |
◆ MAX_WRITE_BLOCK_SIZE
#define MAX_WRITE_BLOCK_SIZE 64 |
◆ MAX_WRITE_RETRIES
#define MAX_WRITE_RETRIES 2 |
◆ MIN
#define MIN | ( | a, | |
b | |||
) | (((a)<(b))?(a):(b)) |
◆ MIN_WRITE_BLOCK_SIZE
#define MIN_WRITE_BLOCK_SIZE 4 |
◆ READ_BLOCK_SIZE
#define READ_BLOCK_SIZE 64 |
◆ RETRY_COMMAND
#define RETRY_COMMAND | ( | func, | |
num_ret, | |||
error | |||
) |
Value:
{ \
uint8_t num_retries; \
num_retries = 0; \
error = 0; \
while (num_retries++ < num_ret) { \
if (func == BLE_STATUS_SUCCESS) \
break; \
if (num_retries == num_ret) \
error = BLE_UTIL_ACI_ERROR; \
} \
}
◆ SECTOR_SIZE
#define SECTOR_SIZE (2*1024) |
◆ SUPPORTED_BOOTLOADER_VERSION_MAX
#define SUPPORTED_BOOTLOADER_VERSION_MAX 5 |
◆ SUPPORTED_BOOTLOADER_VERSION_MIN
#define SUPPORTED_BOOTLOADER_VERSION_MIN 3 |
Function Documentation
◆ change_IFR_data_config()
void change_IFR_data_config | ( | IFR_config2_TypeDef * | IFR_config, |
uint8_t | data[64] | ||
) |
Modify IFR data. (Last 64-bytes block).
- Parameters
-
IFR_config Structure that contains the new parameters inside the IFR configuration data.
- Note
- It is highly recommended to parse the IFR configuration from a working IFR block (this should be done with parse_IFR_data_config()). Then it is possible to write the new parameters inside the IFR_config structure.
- Parameters
-
data Pointer to the buffer that contains the original data. It will be modified according to the new data in the IFR_config structure. Then this data must be written in the last 64-byte block in the IFR. Its size must be 64 bytes.
- Return values
-
None
◆ getBlueNRGUpdaterVersion()
uint8_t getBlueNRGUpdaterVersion | ( | uint8_t * | version | ) |
Get BlueNRG updater version.
- Parameters
-
version This parameter returns the updater version. If the updadter version is 0x03 the chip has the updater old, needs to update the bootloader.
- Return values
-
Status of the call
◆ getBlueNRGVersion()
uint8_t getBlueNRGVersion | ( | uint8_t * | hwVersion, |
uint16_t * | fwVersion | ||
) |
Get BlueNRG hardware and firmware version.
- Parameters
-
hwVersion This parameter returns the Hardware Version (i.e. CUT 3.0 = 0x30, CUT 3.1 = 0x31). fwVersion This parameter returns the Firmware Version in the format 0xJJMN where JJ = Major Version number, M = Minor Version number and N = Patch Version number.
- Return values
-
Status of the call
◆ IFR_validate()
int IFR_validate | ( | IFR_config2_TypeDef * | IFR_config | ) |
Check for the correctness of parsed data.
- Parameters
-
IFR_config Data structure filled with parsed data.
- Return values
-
int It returns 0 if successful, or PARSE_ERROR in case data is not correct.
◆ isHWBootloader_Patched()
uint8_t isHWBootloader_Patched | ( | void | ) |
Verifies if the bootloader is patched or not. This function shall be used to fix a bug on the HW bootloader related to the 32 MHz external crystal oscillator.
- Return values
-
TRUE if the HW bootloader is already patched, FALSE otherwise
◆ parse_IFR_data_config()
void parse_IFR_data_config | ( | const uint8_t | data[64], |
IFR_config2_TypeDef * | IFR_config | ||
) |
Parse IFR raw data.
- Parameters
-
data Pointer to the raw data: last 64 bytes read from IFR sector. IFR_config Data structure that will be filled with parsed data.
- Return values
-
None
◆ program_device()
int program_device | ( | const uint8_t * | fw_image, |
uint32_t | fw_size | ||
) |
Flash a new firmware using internal bootloader.
- Parameters
-
fw_image Pointer to the firmware image (raw binary data, little-endian). fw_size Size of the firmware image. The firmware image size shall be multiple of 4 bytes.
- Return values
-
int It returns 0 if successful, or a number not equal to 0 in case of error (ACI_ERROR, UNSUPPORTED_VERSION, WRONG_IMAGE_SIZE, CRC_ERROR)
◆ program_IFR()
int program_IFR | ( | const IFR_config_TypeDef * | ifr_image | ) |
Program raw data to IFR (3 64-bytes blocks).
- Parameters
-
ifr_image Pointer to the buffer that will contain the data to program. Its size must be 192 bytes.
- Return values
-
int It returns 0 if successful
◆ read_IFR()
int read_IFR | ( | uint8_t * | data | ) |
◆ verify_IFR()
uint8_t verify_IFR | ( | const IFR_config_TypeDef * | ifr_data | ) |
Verify raw data from IFR (3 64-bytes blocks).
- Parameters
-
ifr_data Pointer to the buffer that will contain the data to verify. Its size must be 192 bytes.
- Return values
-
int It returns 0 if successful, or a number not equal to 0 in case of error (ACI_ERROR, BLE_UTIL_WRONG_VERIFY)
Generated on Mon Apr 15 2019 18:10:39 for BlueNRG-MS pack for STM32CubeMX by
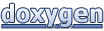