![]() |
BlueNRG-MS pack for STM32CubeMX
V4.4.0
The BlueNRG-MS pack is an additional software for STM32CubeMX.
|
hci_le.h
Go to the documentation of this file.
114 int hci_le_set_advertising_parameters(uint16_t min_interval, uint16_t max_interval, uint8_t advtype,
115 uint8_t own_bdaddr_type, uint8_t direct_bdaddr_type, const tBDAddr direct_bdaddr, uint8_t chan_map,
154 int hci_le_create_connection(uint16_t interval, uint16_t window, uint8_t initiator_filter, uint8_t peer_bdaddr_type,
155 const tBDAddr peer_bdaddr, uint8_t own_bdaddr_type, uint16_t min_interval, uint16_t max_interval,
156 uint16_t latency, uint16_t supervision_timeout, uint16_t min_ce_length, uint16_t max_ce_length);
176 int hci_le_read_local_version(uint8_t *hci_version, uint16_t *hci_revision, uint8_t *lmp_pal_version,
int hci_le_add_device_to_white_list(uint8_t bdaddr_type, tBDAddr bdaddr)
Definition: hci_le.c:569
int hci_read_transmit_power_level(uint16_t *conn_handle, uint8_t type, int8_t *tx_level)
Definition: hci_le.c:617
int hci_le_read_local_supported_features(uint8_t *features)
Definition: hci_le.c:682
int hci_le_set_scan_parameters(uint8_t type, uint16_t interval, uint16_t window, uint8_t own_bdaddr_type, uint8_t filter)
Definition: hci_le.c:210
int hci_le_read_local_version(uint8_t *hci_version, uint16_t *hci_revision, uint8_t *lmp_pal_version, uint16_t *manufacturer_name, uint16_t *lmp_pal_subversion)
Definition: hci_le.c:69
int hci_le_set_advertising_data(uint8_t length, const uint8_t data[])
Definition: hci_le.c:163
int hci_le_set_scan_resp_data(uint8_t length, const uint8_t data[])
Definition: hci_le.c:290
int hci_le_read_channel_map(uint16_t conn_handle, uint8_t ch_map[5])
Definition: hci_le.c:708
int hci_acl_data(const uint8_t *data, uint16_t len)
int hci_le_set_advertising_parameters(uint16_t min_interval, uint16_t max_interval, uint8_t advtype, uint8_t own_bdaddr_type, uint8_t direct_bdaddr_type, const tBDAddr direct_bdaddr, uint8_t chan_map, uint8_t filter)
Definition: hci_le.c:130
int hci_le_set_scan_enable(uint8_t enable, uint8_t filter_dup)
Definition: hci_le.c:239
int hci_le_read_supported_states(uint8_t states[8])
Definition: hci_le.c:739
int hci_le_transmitter_test(uint8_t frequency, uint8_t length, uint8_t payload)
Definition: hci_le.c:788
int hci_le_create_connection(uint16_t interval, uint16_t window, uint8_t initiator_filter, uint8_t peer_bdaddr_type, const tBDAddr peer_bdaddr, uint8_t own_bdaddr_type, uint16_t min_interval, uint16_t max_interval, uint16_t latency, uint16_t supervision_timeout, uint16_t min_ce_length, uint16_t max_ce_length)
Definition: hci_le.c:390
int hci_le_remove_device_from_white_list(uint8_t bdaddr_type, tBDAddr bdaddr)
Definition: hci_le.c:593
int hci_le_read_buffer_size(uint16_t *pkt_len, uint8_t *max_pkt)
Definition: hci_le.c:102
int hci_le_encrypt(uint8_t key[16], uint8_t plaintextData[16], uint8_t encryptedData[16])
Definition: hci_le.c:444
int hci_le_read_advertising_channel_tx_power(int8_t *tx_power_level)
Definition: hci_le.c:314
Generated on Mon Apr 15 2019 18:10:39 for BlueNRG-MS pack for STM32CubeMX by
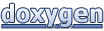