B-L475E-IOT01 BSP User Manual
|
stm32l475e_iot01.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l475e_iot01.h 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 17-March-2017 00007 * @brief STM32L475E IOT01 board support package 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© Copyright (c) 2017 STMicroelectronics International N.V. 00012 * All rights reserved.</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted, provided that the following conditions are met: 00016 * 00017 * 1. Redistribution of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of other 00023 * contributors to this software may be used to endorse or promote products 00024 * derived from this software without specific written permission. 00025 * 4. This software, including modifications and/or derivative works of this 00026 * software, must execute solely and exclusively on microcontroller or 00027 * microprocessor devices manufactured by or for STMicroelectronics. 00028 * 5. Redistribution and use of this software other than as permitted under 00029 * this license is void and will automatically terminate your rights under 00030 * this license. 00031 * 00032 * THIS SOFTWARE IS PROVIDED BY STMICROELECTRONICS AND CONTRIBUTORS "AS IS" 00033 * AND ANY EXPRESS, IMPLIED OR STATUTORY WARRANTIES, INCLUDING, BUT NOT 00034 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY, FITNESS FOR A 00035 * PARTICULAR PURPOSE AND NON-INFRINGEMENT OF THIRD PARTY INTELLECTUAL PROPERTY 00036 * RIGHTS ARE DISCLAIMED TO THE FULLEST EXTENT PERMITTED BY LAW. IN NO EVENT 00037 * SHALL STMICROELECTRONICS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00038 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00039 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, 00040 * OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF 00041 * LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00042 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, 00043 * EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00044 * 00045 ****************************************************************************** 00046 */ 00047 00048 /* Define to prevent recursive inclusion -------------------------------------*/ 00049 #ifndef __STM32L475E_IOT01_H 00050 #define __STM32L475E_IOT01_H 00051 00052 #ifdef __cplusplus 00053 extern "C" { 00054 #endif 00055 00056 /* Includes ------------------------------------------------------------------*/ 00057 #include "stm32l4xx_hal.h" 00058 00059 /** @addtogroup BSP 00060 * @{ 00061 */ 00062 00063 /** @addtogroup STM32L475E_IOT01 00064 * @{ 00065 */ 00066 00067 /** @addtogroup STM32L475E_IOT01_LOW_LEVEL 00068 * @{ 00069 */ 00070 00071 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Exported_Types LOW LEVEL Exported Types 00072 * @{ 00073 */ 00074 typedef enum 00075 { 00076 LED2 = 0, 00077 LED_GREEN = LED2, 00078 }Led_TypeDef; 00079 00080 00081 typedef enum 00082 { 00083 BUTTON_USER = 0 00084 }Button_TypeDef; 00085 00086 typedef enum 00087 { 00088 BUTTON_MODE_GPIO = 0, 00089 BUTTON_MODE_EXTI = 1 00090 }ButtonMode_TypeDef; 00091 00092 typedef enum 00093 { 00094 COM1 = 0, 00095 COM2 = 0, 00096 }COM_TypeDef; 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Exported_Constants LOW LEVEL Exported Constants 00102 * @{ 00103 */ 00104 00105 /** 00106 * @brief Define for STM32L475E_IOT01 board 00107 */ 00108 #if !defined (USE_STM32L475E_IOT01) 00109 #define USE_STM32L475E_IOT01 00110 #endif 00111 00112 #define LEDn ((uint8_t)1) 00113 00114 #define LED2_PIN GPIO_PIN_14 00115 #define LED2_GPIO_PORT GPIOB 00116 #define LED2_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00117 #define LED2_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00118 00119 00120 00121 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do{if((__INDEX__) == 0) LED2_GPIO_CLK_ENABLE();}while(0) 00122 00123 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) do{if((__INDEX__) == 0) LED2_GPIO_CLK_DISABLE();}while(0) 00124 00125 /* Only one User/Wakeup button */ 00126 #define BUTTONn ((uint8_t)1) 00127 00128 /** 00129 * @brief Wakeup push-button 00130 */ 00131 #define USER_BUTTON_PIN GPIO_PIN_13 00132 #define USER_BUTTON_GPIO_PORT GPIOC 00133 #define USER_BUTTON_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00134 #define USER_BUTTON_GPIO_CLK_DISABLE() __HAL_RCC_GPIOC_CLK_DISABLE() 00135 #define USER_BUTTON_EXTI_IRQn EXTI15_10_IRQn 00136 00137 00138 00139 #define COMn ((uint8_t)1) 00140 00141 /** 00142 * @brief Definition for COM port1, connected to USART1 00143 */ 00144 #define DISCOVERY_COM1 USART1 00145 #define DISCOVERY_COM1_CLK_ENABLE() __HAL_RCC_USART1_CLK_ENABLE() 00146 #define DISCOVERY_COM1_CLK_DISABLE() __HAL_RCC_USART1_CLK_DISABLE() 00147 00148 #define DISCOVERY_COM1_TX_PIN GPIO_PIN_6 00149 #define DISCOVERY_COM1_TX_GPIO_PORT GPIOB 00150 #define DISCOVERY_COM1_TX_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00151 #define DISCOVERY_COM1_TX_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00152 #define DISCOVERY_COM1_TX_AF GPIO_AF7_USART1 00153 00154 #define DISCOVERY_COM1_RX_PIN GPIO_PIN_7 00155 #define DISCOVERY_COM1_RX_GPIO_PORT GPIOB 00156 #define DISCOVERY_COM1_RX_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00157 #define DISCOVERY_COM1_RX_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00158 #define DISCOVERY_COM1_RX_AF GPIO_AF7_USART1 00159 00160 #define DISCOVERY_COM1_IRQn USART1_IRQn 00161 00162 00163 #define DISCOVERY_COMx_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == COM1) {DISCOVERY_COM1_CLK_ENABLE();}} while(0) 00164 #define DISCOVERY_COMx_CLK_DISABLE(__INDEX__) do { if((__INDEX__) == COM1) {DISCOVERY_COM1_CLK_DISABLE();}} while(0) 00165 00166 #define DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == COM1) {DISCOVERY_COM1_TX_GPIO_CLK_ENABLE();}} while(0) 00167 #define DISCOVERY_COMx_TX_GPIO_CLK_DISABLE(__INDEX__) do { if((__INDEX__) == COM1) {DISCOVERY_COM1_TX_GPIO_CLK_DISABLE();}} while(0) 00168 00169 #define DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(__INDEX__) do { if((__INDEX__) == COM1) {DISCOVERY_COM1_RX_GPIO_CLK_ENABLE();}} while(0) 00170 #define DISCOVERY_COMx_RX_GPIO_CLK_DISABLE(__INDEX__) do { if((__INDEX__) == COM1) {DISCOVERY_COM1_RX_GPIO_CLK_DISABLE();}} while(0) 00171 00172 00173 /* User can use this section to tailor I2Cx instance used and associated resources */ 00174 /* Definition for I2Cx resources */ 00175 #define DISCOVERY_I2Cx I2C2 00176 #define DISCOVERY_I2Cx_CLK_ENABLE() __HAL_RCC_I2C2_CLK_ENABLE() 00177 #define DISCOVERY_I2Cx_CLK_DISABLE() __HAL_RCC_I2C2_CLK_DISABLE() 00178 #define DISCOVERY_DMAx_CLK_ENABLE() __HAL_RCC_DMA1_CLK_ENABLE() 00179 #define DISCOVERY_I2Cx_SCL_SDA_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00180 #define DISCOVERY_I2Cx_SCL_SDA_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00181 00182 #define DISCOVERY_I2Cx_FORCE_RESET() __HAL_RCC_I2C2_FORCE_RESET() 00183 #define DISCOVERY_I2Cx_RELEASE_RESET() __HAL_RCC_I2C2_RELEASE_RESET() 00184 00185 /* Definition for I2Cx Pins */ 00186 #define DISCOVERY_I2Cx_SCL_PIN GPIO_PIN_10 00187 #define DISCOVERY_I2Cx_SDA_PIN GPIO_PIN_11 00188 #define DISCOVERY_I2Cx_SCL_SDA_GPIO_PORT GPIOB 00189 #define DISCOVERY_I2Cx_SCL_SDA_AF GPIO_AF4_I2C2 00190 00191 /* I2C interrupt requests */ 00192 #define DISCOVERY_I2Cx_EV_IRQn I2C2_EV_IRQn 00193 #define DISCOVERY_I2Cx_ER_IRQn I2C2_ER_IRQn 00194 00195 /* I2C clock speed configuration (in Hz) 00196 WARNING: 00197 Make sure that this define is not already declared in other files. 00198 It can be used in parallel by other modules. */ 00199 #ifndef DISCOVERY_I2C_SPEED 00200 #define DISCOVERY_I2C_SPEED 100000 00201 #endif /* DISCOVERY_I2C_SPEED */ 00202 00203 #ifndef DISCOVERY_I2Cx_TIMING 00204 #define DISCOVERY_I2Cx_TIMING ((uint32_t)0x00702681) 00205 #endif /* DISCOVERY_I2Cx_TIMING */ 00206 00207 00208 /* I2C Sensors address */ 00209 /* LPS22HB (Pressure) I2C Address */ 00210 #define LPS22HB_I2C_ADDRESS (uint8_t)0xBA 00211 /* HTS221 (Humidity) I2C Address */ 00212 #define HTS221_I2C_ADDRESS (uint8_t)0xBE 00213 00214 #ifdef USE_LPS22HB_TEMP 00215 /* LPS22HB Sensor hardware I2C address */ 00216 #define TSENSOR_I2C_ADDRESS LPS22HB_I2C_ADDRESS 00217 #else /* USE_HTS221_TEMP */ 00218 /* HTS221 Sensor hardware I2C address */ 00219 #define TSENSOR_I2C_ADDRESS HTS221_I2C_ADDRESS 00220 #endif 00221 /** 00222 * @} 00223 */ 00224 00225 /* Exported types ------------------------------------------------------------*/ 00226 /* Exported constants --------------------------------------------------------*/ 00227 /* Exported macros -----------------------------------------------------------*/ 00228 /* Private macros ------------------------------------------------------------*/ 00229 /* Exported functions --------------------------------------------------------*/ 00230 00231 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Exported_Functions LOW LEVEL Exported Functions 00232 * @{ 00233 */ 00234 uint32_t BSP_GetVersion(void); 00235 void BSP_LED_Init(Led_TypeDef Led); 00236 void BSP_LED_DeInit(Led_TypeDef Led); 00237 void BSP_LED_On(Led_TypeDef Led); 00238 void BSP_LED_Off(Led_TypeDef Led); 00239 void BSP_LED_Toggle(Led_TypeDef Led); 00240 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode); 00241 void BSP_PB_DeInit(Button_TypeDef Button); 00242 uint32_t BSP_PB_GetState(Button_TypeDef Button); 00243 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *husart); 00244 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart); 00245 /** 00246 * @} 00247 */ 00248 00249 /** 00250 * @} 00251 */ 00252 00253 /** 00254 * @} 00255 */ 00256 00257 /** 00258 * @} 00259 */ 00260 #ifdef __cplusplus 00261 } 00262 #endif 00263 00264 #endif /* __STM32L475E_IOT01_H */ 00265 00266 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Mar 16 2017 10:38:32 for B-L475E-IOT01 BSP User Manual by
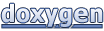