B-L475E-IOT01 BSP User Manual
|
stm32l475e_iot01.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l475e_iot01.c 00004 * @author MCD Application Team 00005 * @version V1.0.0 00006 * @date 17-March-2017 00007 * @brief STM32L475E-IOT01 board support package 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© Copyright (c) 2017 STMicroelectronics International N.V. 00012 * All rights reserved.</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without 00015 * modification, are permitted, provided that the following conditions are met: 00016 * 00017 * 1. Redistribution of source code must retain the above copyright notice, 00018 * this list of conditions and the following disclaimer. 00019 * 2. Redistributions in binary form must reproduce the above copyright notice, 00020 * this list of conditions and the following disclaimer in the documentation 00021 * and/or other materials provided with the distribution. 00022 * 3. Neither the name of STMicroelectronics nor the names of other 00023 * contributors to this software may be used to endorse or promote products 00024 * derived from this software without specific written permission. 00025 * 4. This software, including modifications and/or derivative works of this 00026 * software, must execute solely and exclusively on microcontroller or 00027 * microprocessor devices manufactured by or for STMicroelectronics. 00028 * 5. Redistribution and use of this software other than as permitted under 00029 * this license is void and will automatically terminate your rights under 00030 * this license. 00031 * 00032 * THIS SOFTWARE IS PROVIDED BY STMICROELECTRONICS AND CONTRIBUTORS "AS IS" 00033 * AND ANY EXPRESS, IMPLIED OR STATUTORY WARRANTIES, INCLUDING, BUT NOT 00034 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY, FITNESS FOR A 00035 * PARTICULAR PURPOSE AND NON-INFRINGEMENT OF THIRD PARTY INTELLECTUAL PROPERTY 00036 * RIGHTS ARE DISCLAIMED TO THE FULLEST EXTENT PERMITTED BY LAW. IN NO EVENT 00037 * SHALL STMICROELECTRONICS OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, 00038 * INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00039 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, 00040 * OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF 00041 * LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING 00042 * NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, 00043 * EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00044 * 00045 ****************************************************************************** 00046 */ 00047 00048 /* Includes ------------------------------------------------------------------*/ 00049 #include "stm32l475e_iot01.h" 00050 /** @defgroup BSP BSP 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32L475E_IOT01 STM32L475E_IOT01 00055 * @{ 00056 */ 00057 00058 /** @defgroup STM32L475E_IOT01_LOW_LEVEL LOW LEVEL 00059 * @{ 00060 */ 00061 00062 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Private_Defines LOW LEVEL Private Def 00063 * @{ 00064 */ 00065 /** 00066 * @brief STM32L475E IOT01 BSP Driver version number V1.0.0 00067 */ 00068 #define __STM32L475E_IOT01_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00069 #define __STM32L475E_IOT01_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00070 #define __STM32L475E_IOT01_BSP_VERSION_SUB2 (0x00) /*!< [15:8] sub2 version */ 00071 #define __STM32L475E_IOT01_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00072 #define __STM32L475E_IOT01_BSP_VERSION ((__STM32L475E_IOT01_BSP_VERSION_MAIN << 24)\ 00073 |(__STM32L475E_IOT01_BSP_VERSION_SUB1 << 16)\ 00074 |(__STM32L475E_IOT01_BSP_VERSION_SUB2 << 8 )\ 00075 |(__STM32L475E_IOT01_BSP_VERSION_RC)) 00076 /** 00077 * @} 00078 */ 00079 00080 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Private_Variables LOW LEVEL Variables 00081 * @{ 00082 */ 00083 00084 const uint32_t GPIO_PIN[LEDn] = {LED2_PIN}; 00085 00086 00087 GPIO_TypeDef* GPIO_PORT[LEDn] = {LED2_GPIO_PORT}; 00088 00089 00090 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00091 00092 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00093 00094 const uint16_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn}; 00095 00096 USART_TypeDef* COM_USART[COMn] = {DISCOVERY_COM1}; 00097 00098 GPIO_TypeDef* COM_TX_PORT[COMn] = {DISCOVERY_COM1_TX_GPIO_PORT}; 00099 00100 GPIO_TypeDef* COM_RX_PORT[COMn] = {DISCOVERY_COM1_RX_GPIO_PORT}; 00101 00102 const uint16_t COM_TX_PIN[COMn] = {DISCOVERY_COM1_TX_PIN}; 00103 00104 const uint16_t COM_RX_PIN[COMn] = {DISCOVERY_COM1_RX_PIN}; 00105 00106 const uint16_t COM_TX_AF[COMn] = {DISCOVERY_COM1_TX_AF}; 00107 00108 const uint16_t COM_RX_AF[COMn] = {DISCOVERY_COM1_RX_AF}; 00109 00110 I2C_HandleTypeDef hI2cHandler; 00111 UART_HandleTypeDef hDiscoUart; 00112 /** 00113 * @} 00114 */ 00115 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Private_FunctionPrototypes LOW LEVEL Private Function Prototypes 00116 * @{ 00117 */ 00118 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler); 00119 static void I2Cx_MspDeInit(I2C_HandleTypeDef *i2c_handler); 00120 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler); 00121 static void I2Cx_DeInit(I2C_HandleTypeDef *i2c_handler); 00122 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00123 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddSize, uint8_t *Buffer, uint16_t Length); 00124 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials); 00125 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr); 00126 00127 /* Sensors IO functions */ 00128 void SENSOR_IO_Init(void); 00129 void SENSOR_IO_DeInit(void); 00130 void SENSOR_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00131 uint8_t SENSOR_IO_Read(uint8_t Addr, uint8_t Reg); 00132 uint16_t SENSOR_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00133 void SENSOR_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length); 00134 HAL_StatusTypeDef SENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00135 void SENSOR_IO_Delay(uint32_t Delay); 00136 00137 /** 00138 * @} 00139 */ 00140 00141 /** @defgroup STM32L475E_IOT01_LOW_LEVEL_Private_Functions LOW LEVEL Private Functions 00142 * @{ 00143 */ 00144 00145 /** 00146 * @brief This method returns the STM32L475E IOT01 BSP Driver revision 00147 * @retval version: 0xXYZR (8bits for each decimal, R for RC) 00148 */ 00149 uint32_t BSP_GetVersion(void) 00150 { 00151 return __STM32L475E_IOT01_BSP_VERSION; 00152 } 00153 00154 /** 00155 * @brief Configures LEDs. 00156 * @param Led: LED to be configured. 00157 * This parameter can be one of the following values: 00158 * @arg LED2 00159 */ 00160 void BSP_LED_Init(Led_TypeDef Led) 00161 { 00162 GPIO_InitTypeDef gpio_init_structure; 00163 00164 LEDx_GPIO_CLK_ENABLE(Led); 00165 /* Configure the GPIO_LED pin */ 00166 gpio_init_structure.Pin = GPIO_PIN[Led]; 00167 gpio_init_structure.Mode = GPIO_MODE_OUTPUT_PP; 00168 gpio_init_structure.Pull = GPIO_NOPULL; 00169 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00170 00171 HAL_GPIO_Init(GPIO_PORT[Led], &gpio_init_structure); 00172 } 00173 00174 /** 00175 * @brief DeInit LEDs. 00176 * @param Led: LED to be configured. 00177 * This parameter can be one of the following values: 00178 * @arg LED2 00179 */ 00180 void BSP_LED_DeInit(Led_TypeDef Led) 00181 { 00182 GPIO_InitTypeDef gpio_init_structure; 00183 00184 /* DeInit the GPIO_LED pin */ 00185 gpio_init_structure.Pin = GPIO_PIN[Led]; 00186 00187 /* Turn off LED */ 00188 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00189 HAL_GPIO_DeInit(GPIO_PORT[Led], gpio_init_structure.Pin); 00190 } 00191 00192 /** 00193 * @brief Turns selected LED On. 00194 * @param Led: LED to be set on 00195 * This parameter can be one of the following values: 00196 * @arg LED2 00197 */ 00198 void BSP_LED_On(Led_TypeDef Led) 00199 { 00200 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_SET); 00201 } 00202 00203 /** 00204 * @brief Turns selected LED Off. 00205 * @param Led: LED to be set off 00206 * This parameter can be one of the following values: 00207 * @arg LED2 00208 */ 00209 void BSP_LED_Off(Led_TypeDef Led) 00210 { 00211 HAL_GPIO_WritePin(GPIO_PORT[Led], GPIO_PIN[Led], GPIO_PIN_RESET); 00212 } 00213 00214 /** 00215 * @brief Toggles the selected LED. 00216 * @param Led: LED to be toggled 00217 * This parameter can be one of the following values: 00218 * @arg LED2 00219 */ 00220 void BSP_LED_Toggle(Led_TypeDef Led) 00221 { 00222 HAL_GPIO_TogglePin(GPIO_PORT[Led], GPIO_PIN[Led]); 00223 } 00224 00225 /** 00226 * @brief Configures button GPIO and EXTI Line. 00227 * @param Button: Button to be configured 00228 * This parameter can be one of the following values: 00229 * @arg BUTTON_WAKEUP: Wakeup Push Button 00230 * @param ButtonMode: Button mode 00231 * This parameter can be one of the following values: 00232 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00233 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line 00234 * with interrupt generation capability 00235 */ 00236 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00237 { 00238 GPIO_InitTypeDef gpio_init_structure; 00239 00240 /* Enable the BUTTON clock */ 00241 USER_BUTTON_GPIO_CLK_ENABLE(); 00242 00243 if(ButtonMode == BUTTON_MODE_GPIO) 00244 { 00245 /* Configure Button pin as input */ 00246 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00247 gpio_init_structure.Mode = GPIO_MODE_INPUT; 00248 gpio_init_structure.Pull = GPIO_PULLUP; 00249 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00250 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00251 } 00252 00253 if(ButtonMode == BUTTON_MODE_EXTI) 00254 { 00255 /* Configure Button pin as input with External interrupt */ 00256 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00257 gpio_init_structure.Pull = GPIO_PULLUP; 00258 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00259 00260 gpio_init_structure.Mode = GPIO_MODE_IT_RISING; 00261 00262 HAL_GPIO_Init(BUTTON_PORT[Button], &gpio_init_structure); 00263 00264 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00265 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0x00); 00266 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00267 } 00268 } 00269 00270 /** 00271 * @brief Push Button DeInit. 00272 * @param Button: Button to be configured 00273 * This parameter can be one of the following values: 00274 * @arg BUTTON_WAKEUP: Wakeup Push Button 00275 * @note PB DeInit does not disable the GPIO clock 00276 */ 00277 void BSP_PB_DeInit(Button_TypeDef Button) 00278 { 00279 GPIO_InitTypeDef gpio_init_structure; 00280 00281 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00282 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00283 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00284 } 00285 00286 00287 /** 00288 * @brief Returns the selected button state. 00289 * @param Button: Button to be checked 00290 * This parameter can be one of the following values: 00291 * @arg BUTTON_WAKEUP: Wakeup Push Button 00292 * @retval The Button GPIO pin value (GPIO_PIN_RESET = button pressed) 00293 */ 00294 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00295 { 00296 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00297 } 00298 00299 /** 00300 * @brief Configures COM port. 00301 * @param COM: COM port to be configured. 00302 * This parameter can be one of the following values: 00303 * @arg COM1 00304 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00305 * configuration information for the specified USART peripheral. 00306 */ 00307 void BSP_COM_Init(COM_TypeDef COM, UART_HandleTypeDef *huart) 00308 { 00309 GPIO_InitTypeDef gpio_init_structure; 00310 00311 /* Enable GPIO clock */ 00312 DISCOVERY_COMx_TX_GPIO_CLK_ENABLE(COM); 00313 DISCOVERY_COMx_RX_GPIO_CLK_ENABLE(COM); 00314 00315 /* Enable USART clock */ 00316 DISCOVERY_COMx_CLK_ENABLE(COM); 00317 00318 /* Configure USART Tx as alternate function */ 00319 gpio_init_structure.Pin = COM_TX_PIN[COM]; 00320 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00321 gpio_init_structure.Speed = GPIO_SPEED_FREQ_HIGH; 00322 gpio_init_structure.Pull = GPIO_NOPULL; 00323 gpio_init_structure.Alternate = COM_TX_AF[COM]; 00324 HAL_GPIO_Init(COM_TX_PORT[COM], &gpio_init_structure); 00325 00326 /* Configure USART Rx as alternate function */ 00327 gpio_init_structure.Pin = COM_RX_PIN[COM]; 00328 gpio_init_structure.Mode = GPIO_MODE_AF_PP; 00329 gpio_init_structure.Alternate = COM_RX_AF[COM]; 00330 HAL_GPIO_Init(COM_RX_PORT[COM], &gpio_init_structure); 00331 00332 /* USART configuration */ 00333 huart->Instance = COM_USART[COM]; 00334 HAL_UART_Init(huart); 00335 } 00336 00337 /** 00338 * @brief DeInit COM port. 00339 * @param COM: COM port to be configured. 00340 * This parameter can be one of the following values: 00341 * @arg COM1 00342 * @param huart: Pointer to a UART_HandleTypeDef structure that contains the 00343 * configuration information for the specified USART peripheral. 00344 */ 00345 void BSP_COM_DeInit(COM_TypeDef COM, UART_HandleTypeDef *huart) 00346 { 00347 /* USART configuration */ 00348 huart->Instance = COM_USART[COM]; 00349 HAL_UART_DeInit(huart); 00350 00351 /* Enable USART clock */ 00352 DISCOVERY_COMx_CLK_DISABLE(COM); 00353 00354 /* DeInit GPIO pins can be done in the application 00355 (by surcharging this __weak function) */ 00356 00357 /* GPIO pins clock, FMC clock and DMA clock can be shut down in the application 00358 by surcharging this __weak function */ 00359 } 00360 00361 00362 /******************************************************************************* 00363 BUS OPERATIONS 00364 *******************************************************************************/ 00365 00366 /******************************* I2C Routines *********************************/ 00367 /** 00368 * @brief Initializes I2C MSP. 00369 * @param i2c_handler : I2C handler 00370 * @retval None 00371 */ 00372 static void I2Cx_MspInit(I2C_HandleTypeDef *i2c_handler) 00373 { 00374 GPIO_InitTypeDef gpio_init_structure; 00375 00376 /*** Configure the GPIOs ***/ 00377 /* Enable GPIO clock */ 00378 DISCOVERY_I2Cx_SCL_SDA_GPIO_CLK_ENABLE(); 00379 00380 /* Configure I2C Tx, Rx as alternate function */ 00381 gpio_init_structure.Pin = DISCOVERY_I2Cx_SCL_PIN | DISCOVERY_I2Cx_SDA_PIN; 00382 gpio_init_structure.Mode = GPIO_MODE_AF_OD; 00383 gpio_init_structure.Pull = GPIO_PULLUP; 00384 gpio_init_structure.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00385 gpio_init_structure.Alternate = DISCOVERY_I2Cx_SCL_SDA_AF; 00386 HAL_GPIO_Init(DISCOVERY_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00387 00388 HAL_GPIO_Init(DISCOVERY_I2Cx_SCL_SDA_GPIO_PORT, &gpio_init_structure); 00389 00390 /*** Configure the I2C peripheral ***/ 00391 /* Enable I2C clock */ 00392 DISCOVERY_I2Cx_CLK_ENABLE(); 00393 00394 /* Force the I2C peripheral clock reset */ 00395 DISCOVERY_I2Cx_FORCE_RESET(); 00396 00397 /* Release the I2C peripheral clock reset */ 00398 DISCOVERY_I2Cx_RELEASE_RESET(); 00399 00400 /* Enable and set I2Cx Interrupt to a lower priority */ 00401 HAL_NVIC_SetPriority(DISCOVERY_I2Cx_EV_IRQn, 0x0F, 0); 00402 HAL_NVIC_EnableIRQ(DISCOVERY_I2Cx_EV_IRQn); 00403 00404 /* Enable and set I2Cx Interrupt to a lower priority */ 00405 HAL_NVIC_SetPriority(DISCOVERY_I2Cx_ER_IRQn, 0x0F, 0); 00406 HAL_NVIC_EnableIRQ(DISCOVERY_I2Cx_ER_IRQn); 00407 } 00408 00409 /** 00410 * @brief DeInitializes I2C MSP. 00411 * @param i2c_handler : I2C handler 00412 * @retval None 00413 */ 00414 static void I2Cx_MspDeInit(I2C_HandleTypeDef *i2c_handler) 00415 { 00416 GPIO_InitTypeDef gpio_init_structure; 00417 00418 /* Configure I2C Tx, Rx as alternate function */ 00419 gpio_init_structure.Pin = DISCOVERY_I2Cx_SCL_PIN | DISCOVERY_I2Cx_SDA_PIN; 00420 HAL_GPIO_DeInit(DISCOVERY_I2Cx_SCL_SDA_GPIO_PORT, gpio_init_structure.Pin); 00421 /* Disable GPIO clock */ 00422 DISCOVERY_I2Cx_SCL_SDA_GPIO_CLK_DISABLE(); 00423 00424 /* Disable I2C clock */ 00425 DISCOVERY_I2Cx_CLK_DISABLE(); 00426 } 00427 00428 /** 00429 * @brief Initializes I2C HAL. 00430 * @param i2c_handler : I2C handler 00431 * @retval None 00432 */ 00433 static void I2Cx_Init(I2C_HandleTypeDef *i2c_handler) 00434 { 00435 /* I2C configuration */ 00436 i2c_handler->Instance = DISCOVERY_I2Cx; 00437 i2c_handler->Init.Timing = DISCOVERY_I2Cx_TIMING; 00438 i2c_handler->Init.OwnAddress1 = 0; 00439 i2c_handler->Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00440 i2c_handler->Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00441 i2c_handler->Init.OwnAddress2 = 0; 00442 i2c_handler->Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00443 i2c_handler->Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00444 00445 /* Init the I2C */ 00446 I2Cx_MspInit(i2c_handler); 00447 HAL_I2C_Init(i2c_handler); 00448 00449 /**Configure Analogue filter */ 00450 HAL_I2CEx_ConfigAnalogFilter(i2c_handler, I2C_ANALOGFILTER_ENABLE); 00451 } 00452 00453 /** 00454 * @brief DeInitializes I2C HAL. 00455 * @param i2c_handler : I2C handler 00456 * @retval None 00457 */ 00458 static void I2Cx_DeInit(I2C_HandleTypeDef *i2c_handler) 00459 { /* DeInit the I2C */ 00460 I2Cx_MspDeInit(i2c_handler); 00461 HAL_I2C_DeInit(i2c_handler); 00462 } 00463 00464 /** 00465 * @brief Reads multiple data. 00466 * @param i2c_handler : I2C handler 00467 * @param Addr: I2C address 00468 * @param Reg: Reg address 00469 * @param MemAddress: memory address 00470 * @param Buffer: Pointer to data buffer 00471 * @param Length: Length of the data 00472 * @retval HAL status 00473 */ 00474 static HAL_StatusTypeDef I2Cx_ReadMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00475 { 00476 HAL_StatusTypeDef status = HAL_OK; 00477 00478 status = HAL_I2C_Mem_Read(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00479 00480 /* Check the communication status */ 00481 if(status != HAL_OK) 00482 { 00483 /* I2C error occured */ 00484 I2Cx_Error(i2c_handler, Addr); 00485 } 00486 return status; 00487 } 00488 00489 00490 /** 00491 * @brief Writes a value in a register of the device through BUS in using DMA mode. 00492 * @param i2c_handler : I2C handler 00493 * @param Addr: Device address on BUS Bus. 00494 * @param Reg: The target register address to write 00495 * @param MemAddress: memory address 00496 * @param Buffer: The target register value to be written 00497 * @param Length: buffer size to be written 00498 * @retval HAL status 00499 */ 00500 static HAL_StatusTypeDef I2Cx_WriteMultiple(I2C_HandleTypeDef *i2c_handler, uint8_t Addr, uint16_t Reg, uint16_t MemAddress, uint8_t *Buffer, uint16_t Length) 00501 { 00502 HAL_StatusTypeDef status = HAL_OK; 00503 00504 status = HAL_I2C_Mem_Write(i2c_handler, Addr, (uint16_t)Reg, MemAddress, Buffer, Length, 1000); 00505 00506 /* Check the communication status */ 00507 if(status != HAL_OK) 00508 { 00509 /* Re-Initiaize the I2C Bus */ 00510 I2Cx_Error(i2c_handler, Addr); 00511 } 00512 return status; 00513 } 00514 00515 /** 00516 * @brief Checks if target device is ready for communication. 00517 * @note This function is used with Memory devices 00518 * @param i2c_handler : I2C handler 00519 * @param DevAddress: Target device address 00520 * @param Trials: Number of trials 00521 * @retval HAL status 00522 */ 00523 static HAL_StatusTypeDef I2Cx_IsDeviceReady(I2C_HandleTypeDef *i2c_handler, uint16_t DevAddress, uint32_t Trials) 00524 { 00525 return (HAL_I2C_IsDeviceReady(i2c_handler, DevAddress, Trials, 1000)); 00526 } 00527 00528 /** 00529 * @brief Manages error callback by re-initializing I2C. 00530 * @param i2c_handler : I2C handler 00531 * @param Addr: I2C Address 00532 * @retval None 00533 */ 00534 static void I2Cx_Error(I2C_HandleTypeDef *i2c_handler, uint8_t Addr) 00535 { 00536 /* De-initialize the I2C communication bus */ 00537 HAL_I2C_DeInit(i2c_handler); 00538 00539 /* Re-Initialize the I2C communication bus */ 00540 I2Cx_Init(i2c_handler); 00541 } 00542 00543 /** 00544 * @} 00545 */ 00546 00547 /******************************************************************************* 00548 LINK OPERATIONS 00549 *******************************************************************************/ 00550 /******************************** LINK Sensors ********************************/ 00551 00552 /** 00553 * @brief Initializes Sensors low level. 00554 * @retval None 00555 */ 00556 void SENSOR_IO_Init(void) 00557 { 00558 I2Cx_Init(&hI2cHandler); 00559 } 00560 00561 /** 00562 * @brief DeInitializes Sensors low level. 00563 * @retval None 00564 */ 00565 void SENSOR_IO_DeInit(void) 00566 { 00567 I2Cx_DeInit(&hI2cHandler); 00568 } 00569 00570 /** 00571 * @brief Writes a single data. 00572 * @param Addr: I2C address 00573 * @param Reg: Reg address 00574 * @param Value: Data to be written 00575 * @retval None 00576 */ 00577 void SENSOR_IO_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00578 { 00579 I2Cx_WriteMultiple(&hI2cHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT,(uint8_t*)&Value, 1); 00580 } 00581 00582 /** 00583 * @brief Reads a single data. 00584 * @param Addr: I2C address 00585 * @param Reg: Reg address 00586 * @retval Data to be read 00587 */ 00588 uint8_t SENSOR_IO_Read(uint8_t Addr, uint8_t Reg) 00589 { 00590 uint8_t read_value = 0; 00591 00592 I2Cx_ReadMultiple(&hI2cHandler, Addr, Reg, I2C_MEMADD_SIZE_8BIT, (uint8_t*)&read_value, 1); 00593 00594 return read_value; 00595 } 00596 00597 /** 00598 * @brief Reads multiple data with I2C communication 00599 * channel from TouchScreen. 00600 * @param Addr: I2C address 00601 * @param Reg: Register address 00602 * @param Buffer: Pointer to data buffer 00603 * @param Length: Length of the data 00604 * @retval HAL status 00605 */ 00606 uint16_t SENSOR_IO_ReadMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00607 { 00608 return I2Cx_ReadMultiple(&hI2cHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00609 } 00610 00611 /** 00612 * @brief Writes multiple data with I2C communication 00613 * channel from MCU to TouchScreen. 00614 * @param Addr: I2C address 00615 * @param Reg: Register address 00616 * @param Buffer: Pointer to data buffer 00617 * @param Length: Length of the data 00618 * @retval None 00619 */ 00620 void SENSOR_IO_WriteMultiple(uint8_t Addr, uint8_t Reg, uint8_t *Buffer, uint16_t Length) 00621 { 00622 I2Cx_WriteMultiple(&hI2cHandler, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, Buffer, Length); 00623 } 00624 00625 /** 00626 * @brief Checks if target device is ready for communication. 00627 * @note This function is used with Memory devices 00628 * @param DevAddress: Target device address 00629 * @param Trials: Number of trials 00630 * @retval HAL status 00631 */ 00632 HAL_StatusTypeDef SENSOR_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00633 { 00634 return (I2Cx_IsDeviceReady(&hI2cHandler, DevAddress, Trials)); 00635 } 00636 00637 /** 00638 * @brief Delay function used in TouchScreen low level driver. 00639 * @param Delay: Delay in ms 00640 * @retval None 00641 */ 00642 void SENSOR_IO_Delay(uint32_t Delay) 00643 { 00644 HAL_Delay(Delay); 00645 } 00646 00647 /** 00648 * @} 00649 */ 00650 00651 /** 00652 * @} 00653 */ 00654 00655 /** 00656 * @} 00657 */ 00658 00659 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Mar 16 2017 10:38:32 for B-L475E-IOT01 BSP User Manual by
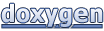