![]() |
Overview | ![]() |
Example
Delphi Code Sample: |
---|
uses graphics32, clipper; ... var sub, clp, sol: TPolygons; begin //set up the subject and clip polygons ... setlength(sub, 3); sub[0] := GetEllipsePoints(IntRect(100,100,300,300)); sub[1] := GetEllipsePoints(IntRect(125,130,275,180)); sub[2] := GetEllipsePoints(IntRect(125,220,275,270)); setlength(clp, 1); clp[0] := GetEllipsePoints(IntRect(140,70,220,320)); //display the subject and clip polygons ... DrawPolygons(img.Bitmap, sub, 0x8033FFFF); DrawPolygons(img.Bitmap, clp, 0x80FFFF33); //get the intersection of the subject and clip polygons ... with TClipper.Create do try AddPolygons(sub, ptSubject); AddPolygons(clp, ptClip); Execute(ctIntersection, sol, pftEvenOdd, pftEvenOdd); finally free; end; //finally draw the intersection polygons ... DrawPolygons(img.Bitmap, sol, 0x40808080); |
C++ Code Sample: |
---|
#include "clipper.hpp" ... //from clipper.hpp ... //typedef long long long64; //struct IntPoint {long64 X; long64 Y;}; //typedef std::vector<IntPoint> Polygon; //typedef std::vector<Polygon> Polygons; using namespace ClipperLib; //set up the subject and clip polygons ... Polygons sub(3); sub[0] = GetEllipsePoints(IntRect(100,100,300,300)); sub[1] = GetEllipsePoints(IntRect(125,130,275,180)); sub[2] = GetEllipsePoints(IntRect(125,220,275,270)); Polygons clp(1); clp[0] = GetEllipsePoints(IntRect(140,70,220,320)); //display the subject and clip polygons ... DrawPolygons(img->Bitmap, sub, 0x8033FFFF); DrawPolygons(img->Bitmap, clp, 0x80FFFF33); //get the intersection of the subject and clip polygons ... Clipper clpr; clpr.AddPolygons(sub, ptSubject); clpr.AddPolygons(clp, ptClip); Polygons sol; clpr.Execute(ctIntersection, sol, pftEvenOdd, pftEvenOdd); //finally draw the intersection polygons ... DrawPolygons(img->Bitmap, sol, 0x40808080); |
C# Code Sample: |
---|
... using ClipperLib; ... using Polygon = List<IntPoint>; using Polygons = List<List<IntPoint>>; ... Polygons subjs = new Polygons(3); Polygons clips = new Polygons(1); Polygons solution = new Polygons(); subjs.Add(GetEllipsePoints(new IntRect(100,100,300,300))); subjs.Add(GetEllipsePoints(new IntRect(125,130,275,180))); subjs.Add(GetEllipsePoints(new IntRect(125,220,275,270))); clips.Add(GetEllipsePoints(new IntRect(140,70,220,320))); DrawPolygons(subjs, 0x8033FFFF); DrawPolygons(clips, 0x80FFFF33); Clipper c = new Clipper(); c.AddPolygons(subjs, PolyType.ptSubject); c.AddPolygons(clips, PolyType.ptClip); c.Execute(ClipType.ctIntersection, solution); DrawPolygons(solution, 0x40808080); |
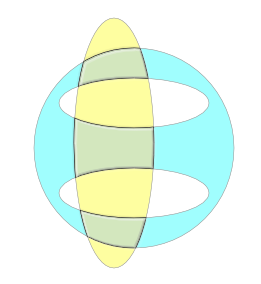
Copyright ©2010-2012 Angus Johnson - Clipper version 5.1.0 - Help file built on 17-February-2013