sl_mqtt_client
|
D:/Project/SimpleLink/mqtt/doxygen/client/sl_mqtt_client.h
Go to the documentation of this file.
00001 /******************************************************************************* 00002 Copyright (c) (2014) Texas Instruments Incorporated 00003 All rights reserved not granted herein. 00004 00005 Limited License. 00006 00007 Texas Instruments Incorporated grants a world-wide, royalty-free, non-exclusive 00008 license under copyrights and patents it now or hereafter owns or controls to make, 00009 have made, use, import, offer to sell and sell ("Utilize") this software subject 00010 to the terms herein. With respect to the foregoing patent license, such license 00011 is granted solely to the extent that any such patent is necessary to Utilize the 00012 software alone. The patent license shall not apply to any combinations which 00013 include this software, other than combinations with devices manufactured by or 00014 for TI (�TI Devices�). No hardware patent is licensed hereunder. 00015 00016 Redistributions must preserve existing copyright notices and reproduce this license 00017 (including the above copyright notice and the disclaimer and (if applicable) source 00018 code license limitations below) in the documentation and/or other materials provided 00019 with the distribution 00020 00021 Redistribution and use in binary form, without modification, are permitted provided 00022 that the following conditions are met: 00023 * No reverse engineering, decompilation, or disassembly of this software is 00024 permitted with respect to any software provided in binary form. 00025 * any redistribution and use are licensed by TI for use only with TI Devices. 00026 * Nothing shall obligate TI to provide you with source code for the software 00027 licensed and provided to you in object code. 00028 00029 If software source code is provided to you, modification and redistribution of the 00030 source code are permitted provided that the following conditions are met; 00031 * any redistribution and use of the source code, including any resulting derivative 00032 works, are licensed by TI for use only with TI Devices. 00033 * any redistribution and use of any object code compiled from the source code and 00034 any resulting derivative works, are licensed by TI for use only with TI Devices. 00035 00036 Neither the name of Texas Instruments Incorporated nor the names of its suppliers 00037 may be used to endorse or promote products derived from this software without 00038 specific prior written permission. 00039 00040 DISCLAIMER. 00041 00042 THIS SOFTWARE IS PROVIDED BY TI AND TI�S LICENSORS "AS IS" AND ANY EXPRESS OR IMPLIED 00043 WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY 00044 AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL TI AND TI�S 00045 LICENSORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00046 CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE 00047 GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) 00048 HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00049 OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF 00050 THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00051 00052 ******************************************************************************/ 00053 00054 #include <stdio.h> 00055 #include <string.h> 00056 #include <stdbool.h> 00057 #include "simplelink.h" 00058 00059 #ifndef __SL_MQTT_H__ 00060 #define __SL_MQTT_H__ 00061 00062 #ifdef __cplusplus 00063 extern "C" 00064 { 00065 #endif 00066 00134 #define SL_MQTT_CL_EVT_PUBACK 0x04 00135 #define SL_MQTT_CL_EVT_PUBCOMP 0x07 00136 #define SL_MQTT_CL_EVT_SUBACK 0x09 00137 #define SL_MQTT_CL_EVT_UNSUBACK 0x0B /* End Client events */ 00139 00140 00141 /* Define server structure which holds , server address and port number. 00142 These values are set by the sl_MqttSet API and retrieved by sl_MqttGet API*/ 00143 00154 typedef struct { 00155 00171 void (*sl_ExtLib_MqttRecv)(void *app_hndl, const char *topstr, _i32 toplen, 00172 const void *payload, _i32 pay_len, 00173 bool dup, unsigned char qos, 00174 bool retain); 00175 00192 void (*sl_ExtLib_MqttEvent)(void *app_hndl, _i32 evt, const void *buf, 00193 _u32 len); 00194 00201 void (*sl_ExtLib_MqttDisconn)(void *app_hndl); 00202 00203 00204 } SlMqttClientCbs_t; 00205 00206 typedef struct { 00207 00208 const char *will_topic; 00209 const char *will_msg; 00210 char will_qos; 00211 bool retain; 00213 } SlMqttWill_t; 00214 00215 00217 typedef struct { 00218 00219 #define SL_MQTT_NETCONN_IP6 0x04 00220 #define SL_MQTT_NETCONN_URL 0x08 00221 #define SL_MQTT_NETCONN_SEC 0x10 00223 _u32 netconn_flags; 00224 const char *server_addr; 00225 _u16 port_number; 00226 char method; 00227 _u32 cipher; 00228 _u32 n_files; 00229 char * const *secure_files; /* SL needs 4 files*/ 00230 00231 } SlMqttServer_t; 00232 00233 00235 typedef struct 00236 { 00239 _u16 loopback_port; 00241 _u32 rx_tsk_priority; 00242 _u32 resp_time; 00243 bool aux_debug_en; 00244 _i32 (*dbg_print)(const char *pcFormat, ...); 00246 } SlMqttClientLibCfg_t; 00247 00248 00250 typedef struct 00251 { 00252 SlMqttServer_t server_info; 00253 bool mqtt_mode31; 00254 bool blocking_send; 00256 } SlMqttClientCtxCfg_t; 00257 00264 _i32 sl_ExtLib_MqttClientInit(const SlMqttClientLibCfg_t *cfg); 00265 00270 _i32 sl_ExtLib_MqttClientExit(); 00271 00279 void *sl_ExtLib_MqttClientCtxCreate(const SlMqttClientCtxCfg_t *ctx_cfg, 00280 const SlMqttClientCbs_t *msg_cbs, 00281 void *app_hndl); 00282 00288 _i32 sl_ExtLib_MqttClientCtxDelete(void *cli_ctx); 00289 00293 #define SL_MQTT_PARAM_CLIENT_ID 0x01 00294 #define SL_MQTT_PARAM_USER_NAME 0x02 00295 #define SL_MQTT_PARAM_PASS_WORD 0x03 00296 #define SL_MQTT_PARAM_TOPIC_QOS1 0x04 00297 #define SL_MQTT_PARAM_WILL_PARAM 0x05 00314 _i32 sl_ExtLib_MqttClientSet(void *cli_ctx, _i32 param, const void *value, _u32 len); 00315 00316 /*\brief None defined at the moment 00317 */ 00318 _i32 sl_ExtLib_MqttClientGet(void *cli_ctx, _i32 param, void *value, _u32 len); 00319 00320 00335 _i32 sl_ExtLib_MqttClientConnect(void *cli_ctx, bool clean, _u16 keep_alive_time); 00336 00345 _i32 sl_ExtLib_MqttClientDisconnect(void *cli_ctx); 00346 00347 00368 _i32 sl_ExtLib_MqttClientSub(void *cli_ctx, char* const *topics, 00369 _u8 *qos, _i32 count); 00370 00371 00389 _i32 sl_ExtLib_MqttClientUnsub(void *cli_ctx, char* const *topics, _i32 count); 00390 00391 00410 _i32 sl_ExtLib_MqttClientSend(void *cli_ctx, const char *topic, 00411 const void *data, _i32 len, 00412 char qos, bool retain); 00413 00416 static inline _i32 sl_ExtLib_MqttClientPub(void *cli_ctx, const char *topic, 00417 const void *data, _i32 len, 00418 char qos, bool retain) 00419 { 00420 return sl_ExtLib_MqttClientSend(cli_ctx, topic, data, len, 00421 qos, retain); 00422 } 00423 /* End Client API */ 00425 00426 #ifdef __cplusplus 00427 } 00428 #endif 00429 00430 00431 00432 #endif // __SL_MQTT_H__
Generated on Thu Jan 15 2015 18:26:27 for sl_mqtt_client by
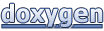