sl_mqtt_client
|
Modules | |
SL MQTT Client Events | |
SL MQTT Oper Paramters | |
Classes | |
struct | SlMqttClientCbs_t |
struct | SlMqttWill_t |
struct | SlMqttServer_t |
struct | SlMqttClientLibCfg_t |
struct | SlMqttClientCtxCfg_t |
Functions | |
_i32 | sl_ExtLib_MqttClientInit (const SlMqttClientLibCfg_t *cfg) |
_i32 | sl_ExtLib_MqttClientExit () |
void * | sl_ExtLib_MqttClientCtxCreate (const SlMqttClientCtxCfg_t *ctx_cfg, const SlMqttClientCbs_t *msg_cbs, void *app_hndl) |
_i32 | sl_ExtLib_MqttClientCtxDelete (void *cli_ctx) |
_i32 | sl_ExtLib_MqttClientSet (void *cli_ctx, _i32 param, const void *value, _u32 len) |
_i32 | sl_ExtLib_MqttClientGet (void *cli_ctx, _i32 param, void *value, _u32 len) |
_i32 | sl_ExtLib_MqttClientConnect (void *cli_ctx, bool clean, _u16 keep_alive_time) |
_i32 | sl_ExtLib_MqttClientDisconnect (void *cli_ctx) |
_i32 | sl_ExtLib_MqttClientSub (void *cli_ctx, char *const *topics, _u8 *qos, _i32 count) |
_i32 | sl_ExtLib_MqttClientUnsub (void *cli_ctx, char *const *topics, _i32 count) |
_i32 | sl_ExtLib_MqttClientSend (void *cli_ctx, const char *topic, const void *data, _i32 len, char qos, bool retain) |
Function Documentation
_i32 sl_ExtLib_MqttClientConnect | ( | void * | cli_ctx, |
bool | clean, | ||
_u16 | keep_alive_time | ||
) |
CONNECT to the server. This routine establishes a connection with the server for MQTT transactions. The caller should specify a time-period with-in which the implementation should send a message to the server to keep-alive the connection.
- Parameters:
-
[in] cli_ctx refers to the handle to the client context [in] clean assert to make a clean start and purge the previous session [in] keep_alive_time the maximum time within which client should send a message to server. The unit of the interval is in seconds.
- Returns:
- on success, variable header of CONNACK message in network byte order. Lowest Byte[Byte0] contains CONNACK Return Code. Byte1 Contains Session Present Bit. on failure returns(-1)
void* sl_ExtLib_MqttClientCtxCreate | ( | const SlMqttClientCtxCfg_t * | ctx_cfg, |
const SlMqttClientCbs_t * | msg_cbs, | ||
void * | app_hndl | ||
) |
Create a new client context to connect to a server. A context has to be created prior to invoking the client services.
- Parameters:
-
[in] ctx_cfg refers to client context configuration parameters [in] msg_cbs refers to callbacks into application [in] app refers to the application callback to be returned on callback
_i32 sl_ExtLib_MqttClientCtxDelete | ( | void * | cli_ctx | ) |
Deletes the specified client context.
\param[in] cli_ctx refers to client context to be deleted \return Success (0) or Failure (< 0)
_i32 sl_ExtLib_MqttClientDisconnect | ( | void * | cli_ctx | ) |
DISCONNECT from the server. The caller must use this service to close the connection with the server.
- Parameters:
-
[in] cli_ctx refers to the handle to the client context
- Returns:
- Success (0) or Failure (< 0)
_i32 sl_ExtLib_MqttClientExit | ( | ) |
Exit the SL MQTT Implementation.
\return Success (0) or Failure (-1)
_i32 sl_ExtLib_MqttClientGet | ( | void * | cli_ctx, |
_i32 | param, | ||
void * | value, | ||
_u32 | len | ||
) |
_i32 sl_ExtLib_MqttClientInit | ( | const SlMqttClientLibCfg_t * | cfg | ) |
Initialize the SL MQTT Implementation. A caller must initialize the MQTT implementation prior to using its services.
- Parameters:
-
[in] cfg refers to client lib configuration parameters
- Returns:
- Success (0) or Failure (-1)
_i32 sl_ExtLib_MqttClientSend | ( | void * | cli_ctx, |
const char * | topic, | ||
const void * | data, | ||
_i32 | len, | ||
char | qos, | ||
bool | retain | ||
) |
PUBLISH a named message to the server. In addition to the PUBLISH specific parameters, the caller can indicate whether the routine should block until the time, the message has been acknowleged by the server. This is applicable only for non-QoS0 messages.
In case, the app has chosen not to await for the ACK from the server, the SL MQTT implementation will notify the app about the subscription through the callback routine.
- Parameters:
-
[in] cli_ctx refers to the handle to the client context [in] topic topic of the data to be published. It is NULL terminated. [in] data binary data to be published [in] len length of the data [in] qos QoS for the publish message [in] retain assert if server should retain the message [in] flags Command flag. Refer to sl_mqtt_cl_cmdflags
- Returns:
- Success(transaction Message ID) or Failure(< 0)
_i32 sl_ExtLib_MqttClientSet | ( | void * | cli_ctx, |
_i32 | param, | ||
const void * | value, | ||
_u32 | len | ||
) |
Set parameters in SL MQTT implementation. The caller must configure these paramters prior to invoking any MQTT transaction.
- Note:
- The implementation does not copy the contents referred. Therefore, the caller must ensure that contents are persistent in the memory.
- Parameters:
-
[in] cli_ctx refers to the handle to the client context [in] param identifies parameter to set. Refer to SL MQTT Oper Paramters [in] value refers to the place-holder of value to be set [in] len length of the value of the parameter
- Returns:
- Success (0) or Failure (-1)
_i32 sl_ExtLib_MqttClientSub | ( | void * | cli_ctx, |
char *const * | topics, | ||
_u8 * | qos, | ||
_i32 | count | ||
) |
SUBSCRIBE a set of topics. To receive data about a set of topics from the server, the app through this routine must subscribe to those topic names with the server. The caller can indicate whether the routine should block until a time, the message has been acknowledged by the server.
In case, the app has chosen not to await for the ACK from the server, the SL MQTT implementation will notify the app about the subscription through the callback routine.
- Parameters:
-
[in] cli_ctx refers to the handle to the client context [in] topics set of topic names to subscribe. It is an array of pointers to NUL terminated strings. [in,out] qos array of qos values for each topic in the same order of the topic array. If configured to await for SUB-ACK from server, the array will contain qos responses for topics from the server. [in] count number of such topics
- Returns:
- Success(transaction Message ID) or Failure(< 0)
_i32 sl_ExtLib_MqttClientUnsub | ( | void * | cli_ctx, |
char *const * | topics, | ||
_i32 | count | ||
) |
UNSUBSCRIBE a set of topics. The app should use this service to stop receiving data for the named topics from the server. The caller can indicate whether the routine should block until a time, the message has been acknowleged by the server.
In case, the app has chosen not to await for the ACK from the server, the SL MQTT implementation will notify the app about the subscription through the callback routine.
- Parameters:
-
[in] cli_ctx refers to the handle to the client context [in] topics set of topics to be unsubscribed. It is an array of pointers to NUL terminated strings. [in] count number of topics to be unsubscribed
- Returns:
- Success(transaction Message ID) or Failure(< 0)
Generated on Thu Jan 15 2015 18:26:27 for sl_mqtt_client by
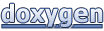