STM32L152D_EVAL BSP User Manual
|
stm32l152d_eval_sd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152d_eval_sd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the uSD card driver. 00006 ****************************************************************************** 00007 * @attention 00008 * 00009 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00010 * 00011 * Redistribution and use in source and binary forms, with or without modification, 00012 * are permitted provided that the following conditions are met: 00013 * 1. Redistributions of source code must retain the above copyright notice, 00014 * this list of conditions and the following disclaimer. 00015 * 2. Redistributions in binary form must reproduce the above copyright notice, 00016 * this list of conditions and the following disclaimer in the documentation 00017 * and/or other materials provided with the distribution. 00018 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00019 * may be used to endorse or promote products derived from this software 00020 * without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00023 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00024 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00025 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00026 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00027 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00028 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00029 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00030 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00031 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00032 * 00033 ****************************************************************************** 00034 */ 00035 00036 /* Define to prevent recursive inclusion -------------------------------------*/ 00037 #ifndef __STM32L152D_EVAL_SD_H 00038 #define __STM32L152D_EVAL_SD_H 00039 00040 #ifdef __cplusplus 00041 extern "C" { 00042 #endif 00043 00044 /* Includes ------------------------------------------------------------------*/ 00045 #include "stm32l1xx_hal.h" 00046 00047 /** @addtogroup BSP 00048 * @{ 00049 */ 00050 00051 /** @addtogroup STM32L152D_EVAL 00052 * @{ 00053 */ 00054 00055 /** @addtogroup STM32L152D_EVAL_SD 00056 * @{ 00057 */ 00058 00059 /* Exported types ------------------------------------------------------------*/ 00060 00061 /** @defgroup STM32L152D_EVAL_SD_Exported_Types Exported Types 00062 * @{ 00063 */ 00064 00065 /** 00066 * @brief SD Card information structure 00067 */ 00068 #define SD_CardInfo HAL_SD_CardInfoTypedef 00069 00070 /** 00071 * @brief SD status structure definition 00072 */ 00073 #define MSD_OK 0x00 00074 #define MSD_ERROR 0x01 00075 00076 /** 00077 * @} 00078 */ 00079 00080 00081 /* Exported constants --------------------------------------------------------*/ 00082 00083 /** @defgroup STM324x9I_EVAL_SD_Exported_Constants Exported Constants 00084 * @{ 00085 */ 00086 #define SD_DETECT_PIN GPIO_PIN_7 00087 #define SD_DETECT_GPIO_PORT GPIOC 00088 #define __SD_DETECT_GPIO_CLK_ENABLE() __HAL_RCC_GPIOC_CLK_ENABLE() 00089 #define SD_DETECT_IRQn EXTI9_5_IRQn 00090 00091 #define SD_DATATIMEOUT ((uint32_t)100000000) 00092 00093 #define SD_PRESENT ((uint8_t)0x01) 00094 #define SD_NOT_PRESENT ((uint8_t)0x00) 00095 00096 /* DMA definitions for SD DMA transfer */ 00097 #define __DMAx_TxRx_CLK_ENABLE __HAL_RCC_DMA2_CLK_ENABLE 00098 #define SD_DMAx_Tx_STREAM DMA2_Channel4 00099 #define SD_DMAx_Rx_STREAM DMA2_Channel4 00100 #define SD_DMAx_Tx_IRQn DMA2_Channel4_IRQn 00101 #define SD_DMAx_Rx_IRQn DMA2_Channel4_IRQn 00102 #define SD_DMAx_Tx_IRQHandler DMA2_Channel4_IRQHandler 00103 #define SD_DMAx_Rx_IRQHandler DMA2_Channel4_IRQHandler 00104 #define SD_DetectIRQHandler() HAL_GPIO_EXTI_IRQHandler(GPIO_PIN_7) 00105 00106 /** 00107 * @} 00108 */ 00109 00110 00111 /* Exported functions --------------------------------------------------------*/ 00112 00113 /** @addtogroup STM32L152D_EVAL_SD_Exported_Functions 00114 * @{ 00115 */ 00116 uint8_t BSP_SD_Init(void); 00117 uint8_t BSP_SD_ITConfig(void); 00118 void BSP_SD_DetectIT(void); 00119 void BSP_SD_DetectCallback(void); 00120 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks); 00121 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks); 00122 uint8_t BSP_SD_ReadBlocks_DMA(uint32_t *pData, uint64_t ReadAddr, uint32_t BlockSize, uint32_t NumOfBlocks); 00123 uint8_t BSP_SD_WriteBlocks_DMA(uint32_t *pData, uint64_t WriteAddr, uint32_t BlockSize, uint32_t NumOfBlocks); 00124 uint8_t BSP_SD_Erase(uint64_t StartAddr, uint64_t EndAddr); 00125 void BSP_SD_IRQHandler(void); 00126 void BSP_SD_DMA_Tx_IRQHandler(void); 00127 void BSP_SD_DMA_Rx_IRQHandler(void); 00128 HAL_SD_TransferStateTypedef BSP_SD_GetStatus(void); 00129 void BSP_SD_GetCardInfo(HAL_SD_CardInfoTypedef *CardInfo); 00130 uint8_t BSP_SD_IsDetected(void); 00131 00132 #ifdef __cplusplus 00133 } 00134 #endif 00135 00136 #endif /* __STM32L152D_EVAL_SD_H */ 00137 00138 /** 00139 * @} 00140 */ 00141 00142 /** 00143 * @} 00144 */ 00145 00146 /** 00147 * @} 00148 */ 00149 00150 /** 00151 * @} 00152 */ 00153 00154 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Aug 24 2017 17:57:47 for STM32L152D_EVAL BSP User Manual by
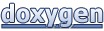