STM32L152D_EVAL BSP User Manual
|
stm32l152d_eval_nor.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152d_eval_nor.c 00004 * @author MCD Application Team 00005 * @brief This file includes a standard driver for the M29W128GL and 00006 * M29W256GL NOR memories mounted on STM32L152D-EVAL board. 00007 @verbatim 00008 ============================================================================== 00009 ##### How to use this driver ##### 00010 ============================================================================== 00011 [..] 00012 (#) This driver is used to drive the M29W128GL and M29W256GL NOR flash external 00013 memory mounted on STM32L152D-EVAL evaluation board. 00014 00015 (#) This driver does not need a specific component driver for the NOR device 00016 to be included with. 00017 00018 (#) Initialization steps: 00019 (++) Initialize the NOR external memory using the BSP_NOR_Init() function. This 00020 function includes the MSP layer hardware resources initialization and the 00021 FSMC controller configuration to interface with the external NOR memory. 00022 00023 (#) NOR flash operations 00024 (++) NOR external memory can be accessed with read/write operations once it is 00025 initialized. 00026 Read/write operation can be performed with AHB access using the functions 00027 BSP_NOR_ReadData()/BSP_NOR_WriteData(). The BSP_NOR_WriteData() performs write operation 00028 of an amount of data by unit (halfword). You can also perform a program data 00029 operation of an amount of data using the function BSP_NOR_ProgramData(). 00030 (++) The function BSP_NOR_Read_ID() returns the chip IDs stored in the structure 00031 "NOR_IDTypeDef". (see the NOR IDs in the memory data sheet) 00032 (++) Perform erase block operation using the function BSP_NOR_Erase_Block() and by 00033 specifying the block address. You can perform an erase operation of the whole 00034 chip by calling the function BSP_NOR_Erase_Chip(). 00035 (++) After other operations, the function BSP_NOR_ReturnToReadMode() allows the NOR 00036 flash to return to read mode to perform read operations on it. 00037 @endverbatim 00038 ****************************************************************************** 00039 * @attention 00040 * 00041 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00042 * 00043 * Redistribution and use in source and binary forms, with or without modification, 00044 * are permitted provided that the following conditions are met: 00045 * 1. Redistributions of source code must retain the above copyright notice, 00046 * this list of conditions and the following disclaimer. 00047 * 2. Redistributions in binary form must reproduce the above copyright notice, 00048 * this list of conditions and the following disclaimer in the documentation 00049 * and/or other materials provided with the distribution. 00050 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00051 * may be used to endorse or promote products derived from this software 00052 * without specific prior written permission. 00053 * 00054 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00055 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00056 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00057 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00058 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00059 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00060 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00061 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00062 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00063 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00064 * 00065 ****************************************************************************** 00066 */ 00067 00068 /* Includes ------------------------------------------------------------------*/ 00069 #include "stm32l152d_eval_nor.h" 00070 00071 /** @addtogroup BSP 00072 * @{ 00073 */ 00074 00075 /** @addtogroup STM32L152D_EVAL 00076 * @{ 00077 */ 00078 00079 /** @defgroup STM32L152D_EVAL_NOR STM32L152D-EVAL NOR 00080 * @{ 00081 */ 00082 00083 00084 /* Private variables ---------------------------------------------------------*/ 00085 00086 /** @defgroup STM32L152D_EVAL_NOR_Private_Variables Private Variables 00087 * @{ 00088 */ 00089 static NOR_HandleTypeDef norHandle; 00090 static FSMC_NORSRAM_TimingTypeDef Timing; 00091 00092 /** 00093 * @} 00094 */ 00095 00096 00097 00098 /* Private functions ---------------------------------------------------------*/ 00099 00100 /** @defgroup STM32L152D_EVAL_NOR_Private_Functions Private Functions 00101 * @{ 00102 */ 00103 00104 static void NOR_MspInit(void); 00105 00106 /** 00107 * @} 00108 */ 00109 00110 /** @defgroup STM32L152D_EVAL_NOR_Exported_Functions Exported Functions 00111 * @{ 00112 */ 00113 00114 /** 00115 * @brief Initializes the NOR device. 00116 * @retval NOR memory status 00117 */ 00118 uint8_t BSP_NOR_Init(void) 00119 { 00120 norHandle.Instance = FSMC_NORSRAM_DEVICE; 00121 norHandle.Extended = FSMC_NORSRAM_EXTENDED_DEVICE; 00122 00123 /* NOR device configuration */ 00124 Timing.AddressSetupTime = 4; 00125 Timing.AddressHoldTime = 3; 00126 Timing.DataSetupTime = 7; 00127 Timing.BusTurnAroundDuration = 1; 00128 Timing.CLKDivision = 2; 00129 Timing.DataLatency = 2; 00130 Timing.AccessMode = FSMC_ACCESS_MODE_B; 00131 00132 norHandle.Init.NSBank = FSMC_NORSRAM_BANK2; 00133 norHandle.Init.DataAddressMux = FSMC_DATA_ADDRESS_MUX_DISABLE; 00134 norHandle.Init.MemoryType = FSMC_MEMORY_TYPE_NOR; 00135 norHandle.Init.MemoryDataWidth = NOR_MEMORY_WIDTH; 00136 norHandle.Init.BurstAccessMode = NOR_BURSTACCESS; 00137 norHandle.Init.WaitSignalPolarity = FSMC_WAIT_SIGNAL_POLARITY_LOW; 00138 norHandle.Init.WrapMode = FSMC_WRAP_MODE_DISABLE; 00139 norHandle.Init.WaitSignalActive = FSMC_WAIT_TIMING_BEFORE_WS; 00140 norHandle.Init.WriteOperation = FSMC_WRITE_OPERATION_ENABLE; 00141 norHandle.Init.WaitSignal = FSMC_WAIT_SIGNAL_DISABLE; 00142 norHandle.Init.ExtendedMode = FSMC_EXTENDED_MODE_DISABLE; 00143 norHandle.Init.AsynchronousWait = FSMC_ASYNCHRONOUS_WAIT_DISABLE; 00144 norHandle.Init.WriteBurst = NOR_WRITEBURST; 00145 00146 /* NOR controller initialization */ 00147 NOR_MspInit(); 00148 00149 if(HAL_NOR_Init(&norHandle, &Timing, &Timing) != HAL_OK) 00150 { 00151 return NOR_STATUS_ERROR; 00152 } 00153 else 00154 { 00155 return NOR_STATUS_OK; 00156 } 00157 } 00158 00159 /** 00160 * @brief Reads an amount of data from the NOR device. 00161 * @param uwStartAddress: Read start address 00162 * @param pData: Pointer to data to be read 00163 * @param uwDataSize: Size of data to read 00164 * @retval NOR memory status 00165 */ 00166 uint8_t BSP_NOR_ReadData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00167 { 00168 if(HAL_NOR_ReadBuffer(&norHandle, NOR_DEVICE_ADDR + uwStartAddress, pData, uwDataSize) != HAL_OK) 00169 { 00170 return NOR_STATUS_ERROR; 00171 } 00172 else 00173 { 00174 return NOR_STATUS_OK; 00175 } 00176 } 00177 00178 /** 00179 * @brief Returns the NOR memory to read mode. 00180 * @retval None 00181 */ 00182 void BSP_NOR_ReturnToReadMode(void) 00183 { 00184 HAL_NOR_ReturnToReadMode(&norHandle); 00185 } 00186 00187 /** 00188 * @brief Writes an amount of data to the NOR device. 00189 * @param uwStartAddress: Write start address 00190 * @param pData: Pointer to data to be written 00191 * @param uwDataSize: Size of data to write 00192 * @retval NOR memory status 00193 */ 00194 uint8_t BSP_NOR_WriteData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00195 { 00196 uint32_t index = uwDataSize; 00197 00198 while(index > 0) 00199 { 00200 /* Write data to NOR */ 00201 HAL_NOR_Program(&norHandle, (uint32_t *)(NOR_DEVICE_ADDR + uwStartAddress), pData); 00202 00203 /* Read NOR device status */ 00204 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, PROGRAM_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00205 { 00206 return NOR_STATUS_ERROR; 00207 } 00208 00209 /* Update the counters */ 00210 index--; 00211 uwStartAddress += 2; 00212 pData++; 00213 } 00214 00215 return NOR_STATUS_OK; 00216 } 00217 00218 /** 00219 * @brief Programs an amount of data to the NOR device. 00220 * @param uwStartAddress: Write start address 00221 * @param pData: Pointer to data to be written 00222 * @param uwDataSize: Size of data to write 00223 * @retval NOR memory status 00224 */ 00225 uint8_t BSP_NOR_ProgramData(uint32_t uwStartAddress, uint16_t* pData, uint32_t uwDataSize) 00226 { 00227 /* Send NOR program buffer operation */ 00228 HAL_NOR_ProgramBuffer(&norHandle, uwStartAddress, pData, uwDataSize); 00229 00230 /* Return the NOR memory status */ 00231 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, PROGRAM_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00232 { 00233 return NOR_STATUS_ERROR; 00234 } 00235 else 00236 { 00237 return NOR_STATUS_OK; 00238 } 00239 } 00240 00241 /** 00242 * @brief Erases the specified block of the NOR device. 00243 * @param BlockAddress: Block address to erase 00244 * @retval NOR memory status 00245 */ 00246 uint8_t BSP_NOR_Erase_Block(uint32_t BlockAddress) 00247 { 00248 /* Send NOR erase block operation */ 00249 HAL_NOR_Erase_Block(&norHandle, BlockAddress, NOR_DEVICE_ADDR); 00250 00251 /* Return the NOR memory status */ 00252 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, BLOCKERASE_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00253 { 00254 return NOR_STATUS_ERROR; 00255 } 00256 else 00257 { 00258 return NOR_STATUS_OK; 00259 } 00260 } 00261 00262 /** 00263 * @brief Erases the entire NOR chip. 00264 * @retval NOR memory status 00265 */ 00266 uint8_t BSP_NOR_Erase_Chip(void) 00267 { 00268 /* Send NOR Erase chip operation */ 00269 HAL_NOR_Erase_Chip(&norHandle, NOR_DEVICE_ADDR); 00270 00271 /* Return the NOR memory status */ 00272 if(HAL_NOR_GetStatus(&norHandle, NOR_DEVICE_ADDR, CHIPERASE_TIMEOUT) != HAL_NOR_STATUS_SUCCESS) 00273 { 00274 return NOR_STATUS_ERROR; 00275 } 00276 else 00277 { 00278 return NOR_STATUS_OK; 00279 } 00280 } 00281 00282 /** 00283 * @brief Reads NOR flash IDs. 00284 * @param pNOR_ID : Pointer to NOR ID structure 00285 * @retval NOR memory status 00286 */ 00287 uint8_t BSP_NOR_Read_ID(NOR_IDTypeDef *pNOR_ID) 00288 { 00289 if(HAL_NOR_Read_ID(&norHandle, pNOR_ID) != HAL_OK) 00290 { 00291 return NOR_STATUS_ERROR; 00292 } 00293 else 00294 { 00295 return NOR_STATUS_OK; 00296 } 00297 } 00298 00299 /** 00300 * @} 00301 */ 00302 00303 /** @addtogroup STM32L152D_EVAL_NOR_Private_Functions 00304 * @{ 00305 */ 00306 00307 /** 00308 * @brief Initializes the NOR MSP. 00309 * @retval None 00310 */ 00311 static void NOR_MspInit(void) 00312 { 00313 GPIO_InitTypeDef gpioinitstruct = {0}; 00314 00315 /* Enable FMC clock */ 00316 __HAL_RCC_FSMC_CLK_ENABLE(); 00317 00318 /* Enable GPIOs clock */ 00319 __HAL_RCC_GPIOD_CLK_ENABLE(); 00320 __HAL_RCC_GPIOE_CLK_ENABLE(); 00321 __HAL_RCC_GPIOF_CLK_ENABLE(); 00322 __HAL_RCC_GPIOG_CLK_ENABLE(); 00323 00324 /* Common GPIO configuration */ 00325 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00326 gpioinitstruct.Pull = GPIO_PULLUP; 00327 gpioinitstruct.Speed = GPIO_SPEED_FREQ_VERY_HIGH; 00328 gpioinitstruct.Alternate = GPIO_AF12_FSMC; 00329 00330 /* GPIOD configuration */ 00331 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_4 |GPIO_PIN_5 | GPIO_PIN_6 | \ 00332 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | \ 00333 GPIO_PIN_13 |GPIO_PIN_14 | GPIO_PIN_15; 00334 00335 HAL_GPIO_Init(GPIOD, &gpioinitstruct); 00336 00337 /* GPIOE configuration */ 00338 gpioinitstruct.Pin = GPIO_PIN_3 | GPIO_PIN_4 | GPIO_PIN_5 | GPIO_PIN_6 |GPIO_PIN_7 | \ 00339 GPIO_PIN_8 | GPIO_PIN_9 | GPIO_PIN_10 | GPIO_PIN_11 | GPIO_PIN_12 | \ 00340 GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00341 HAL_GPIO_Init(GPIOE, &gpioinitstruct); 00342 00343 /* GPIOF configuration */ 00344 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | \ 00345 GPIO_PIN_5 | GPIO_PIN_12 | GPIO_PIN_13 | GPIO_PIN_14 | GPIO_PIN_15; 00346 HAL_GPIO_Init(GPIOF, &gpioinitstruct); 00347 00348 /* GPIOG configuration */ 00349 gpioinitstruct.Pin = GPIO_PIN_0 | GPIO_PIN_1 | GPIO_PIN_2 | GPIO_PIN_3 | GPIO_PIN_4 | \ 00350 GPIO_PIN_5 | GPIO_PIN_9; 00351 HAL_GPIO_Init(GPIOG, &gpioinitstruct); 00352 } 00353 00354 /** 00355 * @brief NOR BSP Wait for Ready/Busy signal. 00356 * @param hnor: Pointer to NOR handle 00357 * @param Timeout: Timeout duration 00358 * @retval None 00359 */ 00360 void HAL_NOR_MspWait(NOR_HandleTypeDef *hnor, uint32_t Timeout) 00361 { 00362 uint32_t timeout = Timeout; 00363 00364 /* Polling on Ready/Busy signal */ 00365 while((HAL_GPIO_ReadPin(NOR_READY_BUSY_GPIO, NOR_READY_BUSY_PIN) != NOR_BUSY_STATE) && (timeout > 0)) 00366 { 00367 timeout--; 00368 } 00369 00370 timeout = Timeout; 00371 00372 /* Polling on Ready/Busy signal */ 00373 while((HAL_GPIO_ReadPin(NOR_READY_BUSY_GPIO, NOR_READY_BUSY_PIN) != NOR_READY_STATE) && (timeout > 0)) 00374 { 00375 timeout--; 00376 } 00377 } 00378 00379 /** 00380 * @} 00381 */ 00382 00383 /** 00384 * @} 00385 */ 00386 00387 /** 00388 * @} 00389 */ 00390 00391 /** 00392 * @} 00393 */ 00394 00395 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00396
Generated on Thu Aug 24 2017 17:57:47 for STM32L152D_EVAL BSP User Manual by
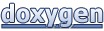