STM32L152D_EVAL BSP User Manual
|
stm32l152d_eval_eeprom.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152d_eval_eeprom.h 00004 * @author MCD Application Team 00005 * @brief This file contains all the functions prototypes for 00006 * the stm32l152d_eval_eeprom.c firmware driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32L152D_EVAL_EEPROM_H 00039 #define __STM32L152D_EVAL_EEPROM_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32l152d_eval.h" 00047 00048 /** @addtogroup BSP 00049 * @{ 00050 */ 00051 00052 /** @addtogroup STM32L152D_EVAL 00053 * @{ 00054 */ 00055 00056 /** @addtogroup STM32L152D_EVAL_EEPROM 00057 * @{ 00058 */ 00059 00060 /** @defgroup STM32L152D_EVAL_EEPROM_Exported_Types Exported Types 00061 * @{ 00062 */ 00063 typedef struct 00064 { 00065 uint32_t (*Init)(void); 00066 uint32_t (*ReadBuffer)(uint8_t* , uint16_t , uint32_t* ); 00067 uint32_t (*WritePage)(uint8_t* , uint16_t , uint32_t* ); 00068 }EEPROM_DrvTypeDef; 00069 /** 00070 * @} 00071 */ 00072 00073 /** @defgroup STM32L152D_EVAL_EEPROM_Exported_Constants Exported Constants 00074 * @{ 00075 */ 00076 /* EEPROMs hardware address and page size */ 00077 #define EEPROM_ADDRESS_M24LR64_A01 0xA0 /* RF EEPROM ANT7-M24LR-A01 used */ 00078 #define EEPROM_ADDRESS_M24LR64_A02 0xA6 /* RF EEPROM ANT7-M24LR-A02 used */ 00079 00080 #define EEPROM_PAGESIZE_M24LR64 4 /* RF EEPROM ANT7-M24LR-A used */ 00081 #define EEPROM_PAGESIZE_M95040 16 /* EEPROM M95040-R used */ 00082 00083 /* EEPROM BSP return values */ 00084 #define EEPROM_OK 0 00085 #define EEPROM_FAIL 1 00086 #define EEPROM_TIMEOUT 2 00087 00088 /* EEPROM BSP devices definition list supported */ 00089 #define BSP_EEPROM_M24LR64 1 /* RF I2C EEPROM M24LR64 */ 00090 #define BSP_EEPROM_M95040 3 /* SPI EEPROM M95040-R */ 00091 00092 /* Maximum number of trials for EEPROM_I2C_WaitEepromStandbyState() function */ 00093 #define EEPROM_MAX_TRIALS 300 00094 /** 00095 * @} 00096 */ 00097 00098 00099 /** @addtogroup STM32L152D_EVAL_EEPROM_Exported_Functions 00100 * @{ 00101 */ 00102 uint32_t BSP_EEPROM_Init(void); 00103 void BSP_EEPROM_SelectDevice(uint8_t DeviceID); 00104 uint32_t BSP_EEPROM_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead); 00105 uint32_t BSP_EEPROM_WriteBuffer(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t NumByteToWrite); 00106 00107 /* USER Callbacks: This function is declared as __weak in EEPROM driver and 00108 should be implemented into user application. 00109 BSP_EEPROM_TIMEOUT_UserCallback() function is called whenever a timeout condition 00110 occure during communication (waiting on an event that doesn't occur, bus 00111 errors, busy devices ...). */ 00112 void BSP_EEPROM_TIMEOUT_UserCallback(void); 00113 00114 00115 /* Link functions for I2C EEPROM peripheral */ 00116 void EEPROM_I2C_IO_Init(void); 00117 HAL_StatusTypeDef EEPROM_I2C_IO_WriteData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00118 HAL_StatusTypeDef EEPROM_I2C_IO_ReadData(uint16_t DevAddress, uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00119 HAL_StatusTypeDef EEPROM_I2C_IO_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00120 00121 /* Link functions for EEPROM peripheral over SPI */ 00122 void EEPROM_SPI_IO_Init(void); 00123 void EEPROM_SPI_IO_WriteByte(uint8_t Data); 00124 uint8_t EEPROM_SPI_IO_ReadByte(void); 00125 HAL_StatusTypeDef EEPROM_SPI_IO_WriteData(uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00126 HAL_StatusTypeDef EEPROM_SPI_IO_ReadData(uint16_t MemAddress, uint8_t* pBuffer, uint32_t BufferSize); 00127 HAL_StatusTypeDef EEPROM_SPI_IO_WaitEepromStandbyState(void); 00128 void EEPROM_SPI_IO_WriteDummy(void); 00129 00130 #ifdef __cplusplus 00131 } 00132 #endif 00133 00134 #endif /* __STM32L152D_EVAL_EEPROM_H */ 00135 00136 /** 00137 * @} 00138 */ 00139 00140 /** 00141 * @} 00142 */ 00143 00144 /** 00145 * @} 00146 */ 00147 00148 /** 00149 * @} 00150 */ 00151 00152 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Thu Aug 24 2017 17:57:47 for STM32L152D_EVAL BSP User Manual by
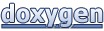