STM32L152D_EVAL BSP User Manual
|
stm32l152d_eval_eeprom.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l152d_eval_eeprom.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage a M24LR64 00006 * I2C EEPROM memory, or a M95040-R SPI EEPROM memory. 00007 * 00008 * =================================================================== 00009 * Notes: 00010 * - This driver is intended for STM32L1xx families devices only. 00011 * - The I2C EEPROM memory (M24LR64) is available on separate daughter 00012 * board ANT7-M24LR-A, which is provided with the STM32L152D 00013 * EVAL board. 00014 * 00015 * - The SPI EEPROM memory (M95040-R) is available directly 00016 * on STM32L152D EVAL board. 00017 * =================================================================== 00018 * 00019 * It implements a high level communication layer for read and write 00020 * from/to this memory. The needed STM32L10x hardware resources (I2C, 00021 * SPI and GPIO) are defined in stm32l152d_eval.h file, 00022 * and the initialization is performed depending of EEPROMs 00023 * in EEPROM_I2C_IO_Init() or EEPROM_SPI_IO_Init() functions 00024 * declared in stm32l152d_eval.c file. 00025 * You can easily tailor this driver to any other development board, 00026 * by just adapting the defines for hardware resources and 00027 * EEPROM_I2C_IO_Init() or EEPROM_SPI_IO_Init() functions. 00028 * 00029 * @note In this driver, basic read and write functions 00030 * (BSP_EEPROM_ReadBuffer() and BSP_EEPROM_WriteBuffer()) 00031 * use Polling mode to perform the data transfer to/from EEPROM memories. 00032 * +-----------------------------------------------------------------+ 00033 * | Pin assignment for M24LR64 EEPROM | 00034 * +---------------------------------------+-----------+-------------+ 00035 * | STM32L1xx I2C Pins | EEPROM | Pin | 00036 * +---------------------------------------+-----------+-------------+ 00037 * | EEPROM_I2C_SDA_PIN/ SDA | SDA | 1 | 00038 * | EEPROM_I2C_SCL_PIN/ SCL | SCL | 2 | 00039 * | . | VDD | 3 (1.8V) | 00040 * | . | GND | 4 (0 V) | 00041 * +---------------------------------------+-----------+-------------+ 00042 * 00043 * +-----------------------------------------------------------------+ 00044 * | Pin assignment for M95040-R EEPROM | 00045 * +---------------------------------------+-----------+-------------+ 00046 * | STM32L1xx SPI Pins | EEPROM | Pin | 00047 * +---------------------------------------+-----------+-------------+ 00048 * | EEPROM_CS_PIN | ChipSelect| 1 (/S) | 00049 * | EEPROM_SPI_MISO_PIN/ MISO | DataOut | 2 (Q) | 00050 * | EEPROM_SPI_WP_PIN | WR Protect| 3 (/W) | 00051 * | . | GND | 4 (0 V) | 00052 * | EEPROM_SPI_MOSI_PIN/ MOSI | DataIn | 5 (D) | 00053 * | EEPROM_SPI_SCK_PIN/ SCLK | Clock | 6 (C) | 00054 * | . | Hold | 7 (/HOLD)| 00055 * | . | VCC | 8 (1.8 V)| 00056 * +---------------------------------------+-----------+-------------+ 00057 ****************************************************************************** 00058 * @attention 00059 * 00060 * <h2><center>© COPYRIGHT(c) 2017 STMicroelectronics</center></h2> 00061 * 00062 * Redistribution and use in source and binary forms, with or without modification, 00063 * are permitted provided that the following conditions are met: 00064 * 1. Redistributions of source code must retain the above copyright notice, 00065 * this list of conditions and the following disclaimer. 00066 * 2. Redistributions in binary form must reproduce the above copyright notice, 00067 * this list of conditions and the following disclaimer in the documentation 00068 * and/or other materials provided with the distribution. 00069 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00070 * may be used to endorse or promote products derived from this software 00071 * without specific prior written permission. 00072 * 00073 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00074 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00075 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00076 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00077 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00078 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00079 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00080 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00081 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00082 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00083 * 00084 ****************************************************************************** 00085 */ 00086 00087 /* Includes ------------------------------------------------------------------*/ 00088 #include "stm32l152d_eval_eeprom.h" 00089 00090 /** @addtogroup BSP 00091 * @{ 00092 */ 00093 00094 00095 /** @addtogroup STM32L152D_EVAL 00096 * @{ 00097 */ 00098 00099 /** @addtogroup STM32L152D_EVAL_EEPROM STM32L152D-EVAL EEPROM 00100 * @brief This file includes the I2C and SPI EEPROM driver 00101 * of STM32L152D-EVAL board. 00102 * @{ 00103 */ 00104 00105 00106 /** @defgroup STM32L152D_EVAL_EEPROM_Private_Variables Private Variables 00107 * @{ 00108 */ 00109 __IO uint16_t EEPROMAddress = 0; 00110 __IO uint16_t EEPROMPageSize = 0; 00111 __IO uint16_t EEPROMDataRead = 0; 00112 __IO uint8_t EEPROMDataWrite = 0; 00113 00114 static EEPROM_DrvTypeDef *EEPROM_SelectedDevice = 0; 00115 /** 00116 * @} 00117 */ 00118 00119 00120 /** @defgroup STM32L152D_EVAL_EEPROM_Private_Functions Private Functions 00121 * @{ 00122 */ 00123 static uint32_t EEPROM_I2C_Init(void); 00124 static uint32_t EEPROM_I2C_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead); 00125 static uint32_t EEPROM_I2C_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite); 00126 static uint32_t EEPROM_I2C_WaitEepromStandbyState(void); 00127 00128 static uint32_t EEPROM_SPI_Init(void); 00129 static uint32_t EEPROM_SPI_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead); 00130 static uint32_t EEPROM_SPI_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite); 00131 static uint32_t EEPROM_SPI_WaitEepromStandbyState(void); 00132 00133 /** 00134 * @} 00135 */ 00136 00137 /** @defgroup STM32L152D_EVAL_EEPROM_Private_Types Private Types 00138 * @{ 00139 */ 00140 /* EEPROM I2C driver typedef */ 00141 EEPROM_DrvTypeDef EEPROM_I2C_Drv = 00142 { 00143 EEPROM_I2C_Init, 00144 EEPROM_I2C_ReadBuffer, 00145 EEPROM_I2C_WritePage 00146 }; 00147 00148 /* EEPROM SPI driver typedef */ 00149 EEPROM_DrvTypeDef EEPROM_SPI_Drv = 00150 { 00151 EEPROM_SPI_Init, 00152 EEPROM_SPI_ReadBuffer, 00153 EEPROM_SPI_WritePage 00154 }; 00155 /** 00156 * @} 00157 */ 00158 00159 /** @defgroup STM32L152D_EVAL_EEPROM_Exported_Functions Exported Functions 00160 * @{ 00161 */ 00162 00163 /** 00164 * @brief Initializes peripherals used by the EEPROM device selected. 00165 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00166 * different from EEPROM_OK (0) 00167 */ 00168 uint32_t BSP_EEPROM_Init(void) 00169 { 00170 if(EEPROM_SelectedDevice->Init != 0) 00171 { 00172 return (EEPROM_SelectedDevice->Init()); 00173 } 00174 else 00175 { 00176 return EEPROM_FAIL; 00177 } 00178 } 00179 00180 /** 00181 * @brief Select the EEPROM device to communicate. 00182 * @param DeviceID: Specifies the EEPROM device to be selected. 00183 * This parameter can be one of following parameters: 00184 * @arg BSP_EEPROM_M24LR64 00185 * @arg BSP_EEPROM_M24M01 00186 * @arg BSP_EEPROM_M95M01 00187 * 00188 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00189 * different from EEPROM_OK (0) 00190 */ 00191 void BSP_EEPROM_SelectDevice(uint8_t DeviceID) 00192 { 00193 switch(DeviceID) 00194 { 00195 case BSP_EEPROM_M24LR64 : 00196 EEPROM_SelectedDevice = &EEPROM_I2C_Drv; 00197 break; 00198 00199 case BSP_EEPROM_M95040 : 00200 EEPROM_SelectedDevice = &EEPROM_SPI_Drv; 00201 break; 00202 00203 default: 00204 break; 00205 } 00206 } 00207 00208 /** 00209 * @brief Reads a block of data from the EEPROM device selected. 00210 * @param pBuffer : pointer to the buffer that receives the data read from 00211 * the EEPROM. 00212 * @param ReadAddr : EEPROM's internal address to start reading from. 00213 * @param NumByteToRead : pointer to the variable holding number of bytes to 00214 * be read from the EEPROM. 00215 * 00216 * @note The variable pointed by NumByteToRead is reset to 0 when all the 00217 * data are read from the EEPROM. Application should monitor this 00218 * variable in order know when the transfer is complete. 00219 * 00220 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00221 * different from EEPROM_OK (0) or the timeout user callback. 00222 */ 00223 uint32_t BSP_EEPROM_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead) 00224 { 00225 if(EEPROM_SelectedDevice->ReadBuffer != 0) 00226 { 00227 return (EEPROM_SelectedDevice->ReadBuffer(pBuffer, ReadAddr, NumByteToRead)); 00228 } 00229 else 00230 { 00231 return EEPROM_FAIL; 00232 } 00233 } 00234 00235 /** 00236 * @brief Writes buffer of data to the EEPROM device selected. 00237 * @param pBuffer : pointer to the buffer containing the data to be written 00238 * to the EEPROM. 00239 * @param WriteAddr : EEPROM's internal address to write to. 00240 * @param NumByteToWrite : number of bytes to write to the EEPROM. 00241 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00242 * different from EEPROM_OK (0) or the timeout user callback. 00243 */ 00244 uint32_t BSP_EEPROM_WriteBuffer(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t NumByteToWrite) 00245 { 00246 uint16_t numofpage = 0, numofsingle = 0, count = 0; 00247 uint16_t addr = 0; 00248 uint32_t dataindex = 0; 00249 uint32_t status = EEPROM_OK; 00250 00251 addr = WriteAddr % EEPROMPageSize; 00252 count = EEPROMPageSize - addr; 00253 numofpage = NumByteToWrite / EEPROMPageSize; 00254 numofsingle = NumByteToWrite % EEPROMPageSize; 00255 00256 if(EEPROM_SelectedDevice->WritePage == 0) 00257 { 00258 return EEPROM_FAIL; 00259 } 00260 00261 /*!< If WriteAddr is EEPROM_PAGESIZE aligned */ 00262 if(addr == 0) 00263 { 00264 /*!< If NumByteToWrite < EEPROM_PAGESIZE */ 00265 if(numofpage == 0) 00266 { 00267 /* Store the number of data to be written */ 00268 dataindex = numofsingle; 00269 /* Start writing data */ 00270 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00271 if (status != EEPROM_OK) 00272 { 00273 return status; 00274 } 00275 } 00276 /*!< If NumByteToWrite > EEPROM_PAGESIZE */ 00277 else 00278 { 00279 while(numofpage--) 00280 { 00281 /* Store the number of data to be written */ 00282 dataindex = EEPROMPageSize; 00283 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00284 if (status != EEPROM_OK) 00285 { 00286 return status; 00287 } 00288 00289 WriteAddr += EEPROMPageSize; 00290 pBuffer += EEPROMPageSize; 00291 } 00292 00293 if(numofsingle!=0) 00294 { 00295 /* Store the number of data to be written */ 00296 dataindex = numofsingle; 00297 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00298 if (status != EEPROM_OK) 00299 { 00300 return status; 00301 } 00302 } 00303 } 00304 } 00305 /*!< If WriteAddr is not EEPROM_PAGESIZE aligned */ 00306 else 00307 { 00308 /*!< If NumByteToWrite < EEPROM_PAGESIZE */ 00309 if(numofpage== 0) 00310 { 00311 /*!< If the number of data to be written is more than the remaining space 00312 in the current page: */ 00313 if (NumByteToWrite > count) 00314 { 00315 /* Store the number of data to be written */ 00316 dataindex = count; 00317 /*!< Write the data contained in same page */ 00318 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00319 if (status != EEPROM_OK) 00320 { 00321 return status; 00322 } 00323 00324 /* Store the number of data to be written */ 00325 dataindex = (NumByteToWrite - count); 00326 /*!< Write the remaining data in the following page */ 00327 status = EEPROM_SelectedDevice->WritePage((uint8_t*)(pBuffer + count), (WriteAddr + count), (uint32_t*)(&dataindex)); 00328 if (status != EEPROM_OK) 00329 { 00330 return status; 00331 } 00332 } 00333 else 00334 { 00335 /* Store the number of data to be written */ 00336 dataindex = numofsingle; 00337 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00338 if (status != EEPROM_OK) 00339 { 00340 return status; 00341 } 00342 } 00343 } 00344 /*!< If NumByteToWrite > EEPROM_PAGESIZE */ 00345 else 00346 { 00347 NumByteToWrite -= count; 00348 numofpage = NumByteToWrite / EEPROMPageSize; 00349 numofsingle = NumByteToWrite % EEPROMPageSize; 00350 00351 if(count != 0) 00352 { 00353 /* Store the number of data to be written */ 00354 dataindex = count; 00355 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00356 if (status != EEPROM_OK) 00357 { 00358 return status; 00359 } 00360 WriteAddr += count; 00361 pBuffer += count; 00362 } 00363 00364 while(numofpage--) 00365 { 00366 /* Store the number of data to be written */ 00367 dataindex = EEPROMPageSize; 00368 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00369 if (status != EEPROM_OK) 00370 { 00371 return status; 00372 } 00373 WriteAddr += EEPROMPageSize; 00374 pBuffer += EEPROMPageSize; 00375 } 00376 if(numofsingle != 0) 00377 { 00378 /* Store the number of data to be written */ 00379 dataindex = numofsingle; 00380 status = EEPROM_SelectedDevice->WritePage(pBuffer, WriteAddr, (uint32_t*)(&dataindex)); 00381 if (status != EEPROM_OK) 00382 { 00383 return status; 00384 } 00385 } 00386 } 00387 } 00388 00389 /* If all operations OK, return EEPROM_OK (0) */ 00390 return EEPROM_OK; 00391 } 00392 00393 /** 00394 * @brief Basic management of the timeout situation. 00395 * @retval None. 00396 */ 00397 __weak void BSP_EEPROM_TIMEOUT_UserCallback(void) 00398 { 00399 } 00400 /** 00401 * @} 00402 */ 00403 00404 /** @addtogroup STM32L152D_EVAL_EEPROM_Private_Functions 00405 * @{ 00406 */ 00407 00408 /** 00409 * @brief Initializes peripherals used by the I2C EEPROM driver. 00410 * @note There are 2 different versions of M24LR64 (A01 & A02). 00411 * Then try to connect on 1st one (EEPROM_I2C_ADDRESS_A01) 00412 * and if problem, check the 2nd one (EEPROM_I2C_ADDRESS_A02) 00413 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00414 * different from EEPROM_OK (0) 00415 */ 00416 static uint32_t EEPROM_I2C_Init(void) 00417 { 00418 EEPROM_I2C_IO_Init(); 00419 00420 /*Select the EEPROM address for M24LR64 A02 and check if OK*/ 00421 EEPROMAddress = EEPROM_ADDRESS_M24LR64_A01; 00422 EEPROMPageSize = EEPROM_PAGESIZE_M24LR64; 00423 if (EEPROM_I2C_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00424 { 00425 /*Select the EEPROM address for M24LR64 A01 and check if OK*/ 00426 EEPROMAddress = EEPROM_ADDRESS_M24LR64_A02; 00427 EEPROMPageSize = EEPROM_PAGESIZE_M24LR64; 00428 if (EEPROM_I2C_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00429 { 00430 return EEPROM_FAIL; 00431 } 00432 } 00433 00434 return EEPROM_OK; 00435 } 00436 00437 /** 00438 * @brief Reads a block of data from the I2C EEPROM. 00439 * @param pBuffer : pointer to the buffer that receives the data read from 00440 * the EEPROM. 00441 * @param ReadAddr : EEPROM's internal address to start reading from. 00442 * @param NumByteToRead : pointer to the variable holding number of bytes to 00443 * be read from the EEPROM. 00444 * 00445 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00446 * different from EEPROM_OK (0) or the timeout user callback. 00447 */ 00448 static uint32_t EEPROM_I2C_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead) 00449 { 00450 uint32_t buffersize = *NumByteToRead; 00451 00452 if (EEPROM_I2C_IO_ReadData(EEPROMAddress, ReadAddr, pBuffer, buffersize) != HAL_OK) 00453 { 00454 return EEPROM_FAIL; 00455 } 00456 00457 /* If all operations OK, return EEPROM_OK (0) */ 00458 return EEPROM_OK; 00459 } 00460 00461 /** 00462 * @brief Writes more than one byte to the EEPROM with a single WRITE cycle. 00463 * 00464 * @note The number of bytes (combined to write start address) must not 00465 * cross the EEPROM page boundary. This function can only write into 00466 * the boundaries of an EEPROM page. 00467 * This function doesn't check on boundaries condition (in this driver 00468 * the function BSP_EEPROM_WriteBuffer() which calls EEPROM_WritePage() is 00469 * responsible of checking on Page boundaries). 00470 * 00471 * @param pBuffer : pointer to the buffer containing the data to be written to 00472 * the EEPROM. 00473 * @param WriteAddr : EEPROM's internal address to write to. 00474 * @param NumByteToWrite : pointer to the variable holding number of bytes to 00475 * be written into the EEPROM. 00476 * 00477 * @note The variable pointed by NumByteToWrite is reset to 0 when all the 00478 * data are written to the EEPROM. Application should monitor this 00479 * variable in order know when the transfer is complete. 00480 * 00481 * 00482 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00483 * different from EEPROM_OK (0) or the timeout user callback. 00484 */ 00485 static uint32_t EEPROM_I2C_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite) 00486 { 00487 uint32_t buffersize = *NumByteToWrite; 00488 00489 if (EEPROM_I2C_IO_WriteData(EEPROMAddress, WriteAddr, pBuffer, buffersize) != HAL_OK) 00490 { 00491 return EEPROM_FAIL; 00492 } 00493 00494 /* Wait for EEPROM Standby state */ 00495 if (EEPROM_I2C_WaitEepromStandbyState() != EEPROM_OK) 00496 { 00497 return EEPROM_FAIL; 00498 } 00499 00500 return EEPROM_OK; 00501 } 00502 00503 /** 00504 * @brief Wait for EEPROM I2C Standby state. 00505 * 00506 * @note This function allows to wait and check that EEPROM has finished the 00507 * last operation. It is mostly used after Write operation: after receiving 00508 * the buffer to be written, the EEPROM may need additional time to actually 00509 * perform the write operation. During this time, it doesn't answer to 00510 * I2C packets addressed to it. Once the write operation is complete 00511 * the EEPROM responds to its address. 00512 * 00513 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00514 * different from EEPROM_OK (0) or the timeout user callback. 00515 */ 00516 static uint32_t EEPROM_I2C_WaitEepromStandbyState(void) 00517 { 00518 /* Check if the maximum allowed number of trials has bee reached */ 00519 if (EEPROM_I2C_IO_IsDeviceReady(EEPROMAddress, EEPROM_MAX_TRIALS) != HAL_OK) 00520 { 00521 /* If the maximum number of trials has been reached, exit the function */ 00522 BSP_EEPROM_TIMEOUT_UserCallback(); 00523 return EEPROM_TIMEOUT; 00524 } 00525 return EEPROM_OK; 00526 } 00527 00528 /** 00529 * @brief Initializes peripherals used by the SPI EEPROM driver. 00530 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00531 * different from EEPROM_OK (0) 00532 */ 00533 static uint32_t EEPROM_SPI_Init(void) 00534 { 00535 EEPROM_SPI_IO_Init(); 00536 00537 /* Check if EEPROM SPI is ready to use */ 00538 EEPROMPageSize = EEPROM_PAGESIZE_M95040; 00539 if(EEPROM_SPI_WaitEepromStandbyState() != HAL_OK) 00540 { 00541 return EEPROM_FAIL; 00542 } 00543 return EEPROM_OK; 00544 } 00545 00546 /** 00547 * @brief Reads a block of data from the SPI EEPROM. 00548 * @param pBuffer : pointer to the buffer that receives the data read from 00549 * the EEPROM. 00550 * @param ReadAddr : EEPROM's internal address to start reading from. 00551 * @param NumByteToRead : pointer to the variable holding number of bytes to 00552 * be read from the EEPROM. 00553 * 00554 * @note The variable pointed by NumByteToRead is reset to 0 when all the 00555 * data are read from the EEPROM. Application should monitor this 00556 * variable in order know when the transfer is complete. 00557 * 00558 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00559 * different from EEPROM_OK (0) or the timeout user callback. 00560 */ 00561 static uint32_t EEPROM_SPI_ReadBuffer(uint8_t* pBuffer, uint16_t ReadAddr, uint32_t* NumByteToRead) 00562 { 00563 uint32_t buffersize = *NumByteToRead; 00564 EEPROM_SPI_IO_ReadData(ReadAddr, pBuffer, buffersize); 00565 00566 /* Wait for EEPROM Standby state */ 00567 if (EEPROM_SPI_WaitEepromStandbyState() != EEPROM_OK) 00568 { 00569 return EEPROM_FAIL; 00570 } 00571 00572 return EEPROM_OK; 00573 } 00574 00575 /** 00576 * @brief Writes more than one byte to the EEPROM with a single WRITE cycle. 00577 * 00578 * @note The number of bytes (combined to write start address) must not 00579 * cross the EEPROM page boundary. This function can only write into 00580 * the boundaries of an EEPROM page. 00581 * This function doesn't check on boundaries condition (in this driver 00582 * the function BSP_EEPROM_WriteBuffer() which calls EEPROM_WritePage() is 00583 * responsible of checking on Page boundaries). 00584 * 00585 * @param pBuffer : pointer to the buffer containing the data to be written to 00586 * the EEPROM. 00587 * @param WriteAddr : EEPROM's internal address to write to. 00588 * @param NumByteToWrite : pointer to the variable holding number of bytes to 00589 * be written into the EEPROM. 00590 * 00591 * @note The variable pointed by NumByteToWrite is reset to 0 when all the 00592 * data are written to the EEPROM. Application should monitor this 00593 * variable in order know when the transfer is complete. 00594 * 00595 * 00596 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00597 * different from EEPROM_OK (0) or the timeout user callback. 00598 */ 00599 static uint32_t EEPROM_SPI_WritePage(uint8_t* pBuffer, uint16_t WriteAddr, uint32_t* NumByteToWrite) 00600 { 00601 uint32_t buffersize = *NumByteToWrite; 00602 00603 if (EEPROM_SPI_IO_WriteData(WriteAddr, pBuffer, buffersize) != HAL_OK) 00604 { 00605 return EEPROM_FAIL; 00606 } 00607 00608 /* Wait for EEPROM Standby state */ 00609 if (EEPROM_SPI_WaitEepromStandbyState() != EEPROM_OK) 00610 { 00611 return EEPROM_FAIL; 00612 } 00613 00614 return EEPROM_OK; 00615 } 00616 00617 /** 00618 * @brief Wait for EEPROM SPI Standby state. 00619 * 00620 * @note This function allows to wait and check that EEPROM has finished the 00621 * last operation. It is mostly used after Write operation. 00622 * 00623 * @retval EEPROM_OK (0) if operation is correctly performed, else return value 00624 * different from EEPROM_OK (0) or the timeout user callback. 00625 */ 00626 static uint32_t EEPROM_SPI_WaitEepromStandbyState(void) 00627 { 00628 if(EEPROM_SPI_IO_WaitEepromStandbyState() != HAL_OK) 00629 { 00630 BSP_EEPROM_TIMEOUT_UserCallback(); 00631 return EEPROM_TIMEOUT; 00632 } 00633 return EEPROM_OK; 00634 } 00635 /** 00636 * @} 00637 */ 00638 00639 /** 00640 * @} 00641 */ 00642 00643 /** 00644 * @} 00645 */ 00646 00647 /** 00648 * @} 00649 */ 00650 00651 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00652
Generated on Thu Aug 24 2017 17:57:47 for STM32L152D_EVAL BSP User Manual by
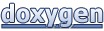