STM32L073Z_EVAL BSP User Manual
|
stm32l073z_eval_tsensor.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l073z_eval_tsensor.c 00004 * @author MCD Application Team 00005 * @brief This file provides a set of functions needed to manage the I2C LM75 00006 * temperature sensor mounted on STM32L073Z-EVAL board . 00007 * It implements a high level communication layer for read and write 00008 * from/to this sensor. The needed STM323L073 hardware resources (I2C and 00009 * GPIO) are defined in stm32l073z_eval.h file, and the initialization is 00010 * performed in TSENSOR_IO_Init() function declared in stm32l073z_eval.c 00011 * file. 00012 * You can easily tailor this driver to any other development board, 00013 * by just adapting the defines for hardware resources and 00014 * TSENSOR_IO_Init() function. 00015 * 00016 * +--------------------------------------------------------------------+ 00017 * | Pin assignment | 00018 * +----------------------------------------+--------------+------------+ 00019 * | STM32L073x I2C Pins | STLM75 | Pin | 00020 * +----------------------------------------+--------------+------------+ 00021 * | EVAL_I2C2_SDA_PIN | SDA | 1 | 00022 * | EVAL_I2C2_SCL_PIN | SCL | 2 | 00023 * | EVAL_I2C2_SMBUS_PIN | OS/INT | 3 | 00024 * | . | GND | 4 (0V) | 00025 * | . | A2 | 5 | 00026 * | . | A1 | 6 | 00027 * | . | A0 | 7 | 00028 * | . | VDD | 8 (5V) | 00029 * +----------------------------------------+--------------+------------+ 00030 ****************************************************************************** 00031 * @attention 00032 * 00033 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00034 * 00035 * Redistribution and use in source and binary forms, with or without modification, 00036 * are permitted provided that the following conditions are met: 00037 * 1. Redistributions of source code must retain the above copyright notice, 00038 * this list of conditions and the following disclaimer. 00039 * 2. Redistributions in binary form must reproduce the above copyright notice, 00040 * this list of conditions and the following disclaimer in the documentation 00041 * and/or other materials provided with the distribution. 00042 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00043 * may be used to endorse or promote products derived from this software 00044 * without specific prior written permission. 00045 * 00046 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00047 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00048 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00049 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00050 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00051 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00052 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00053 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00054 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00055 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00056 * 00057 ****************************************************************************** 00058 */ 00059 00060 /* Includes ------------------------------------------------------------------*/ 00061 #include "stm32l073z_eval_tsensor.h" 00062 00063 /** @addtogroup BSP 00064 * @{ 00065 */ 00066 00067 /** @addtogroup STM32L0_EVAL 00068 * @{ 00069 */ 00070 00071 /** @addtogroup STM32L073Z_EVAL 00072 * @{ 00073 */ 00074 00075 /** @addtogroup STM32L073Z_EVAL_TSENSOR 00076 * @brief This file includes the LM75 Temperature Sensor driver of 00077 * STM32L073Z-EVAL board. 00078 * @{ 00079 */ 00080 00081 /** @defgroup STM32L073Z_EVAL_TSENSOR_Private_Types 00082 * @{ 00083 */ 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM32L073Z_EVAL_TSENSOR_Private_Defines 00089 * @{ 00090 */ 00091 /** 00092 * @} 00093 */ 00094 00095 /** @defgroup STM32L073Z_EVAL_TSENSOR_Private_Macros 00096 * @{ 00097 */ 00098 /** 00099 * @} 00100 */ 00101 00102 /** @defgroup STM32L073Z_EVAL_TSENSOR_Private_Variables 00103 * @{ 00104 */ 00105 static TSENSOR_DrvTypeDef *tsensor_drv; 00106 __IO uint16_t TSENSORAddress = 0; 00107 /** 00108 * @} 00109 */ 00110 00111 /** @defgroup STM32L073Z_EVAL_TSENSOR_Private_Function_Prototypes 00112 * @{ 00113 */ 00114 00115 /** 00116 * @} 00117 */ 00118 00119 00120 /** @defgroup STM32L073Z_EVAL_TSENSOR_Private_Functions 00121 * @{ 00122 */ 00123 00124 /** 00125 * @brief Initializes peripherals used by the I2C Temperature Sensor driver. 00126 * @param None 00127 * @retval TSENSOR status 00128 */ 00129 uint32_t BSP_TSENSOR_Init(void) 00130 { 00131 uint8_t ret = TSENSOR_ERROR; 00132 TSENSOR_InitTypeDef STLM75_InitStructure; 00133 00134 /* Temperature Sensor Initialization */ 00135 if(Stlm75Drv.IsReady(TSENSOR_I2C_ADDRESS_A01, TSENSOR_MAX_TRIALS) == HAL_OK) 00136 { 00137 /* Initialize the temperature sensor driver structure */ 00138 TSENSORAddress = TSENSOR_I2C_ADDRESS_A01; 00139 tsensor_drv = &Stlm75Drv; 00140 00141 ret = TSENSOR_OK; 00142 } 00143 else 00144 { 00145 if(Stlm75Drv.IsReady(TSENSOR_I2C_ADDRESS_A02, TSENSOR_MAX_TRIALS) == HAL_OK) 00146 { 00147 /* Initialize the temperature sensor driver structure */ 00148 TSENSORAddress = TSENSOR_I2C_ADDRESS_A02; 00149 tsensor_drv = &Stlm75Drv; 00150 00151 ret = TSENSOR_OK; 00152 } 00153 else 00154 { 00155 ret = TSENSOR_ERROR; 00156 } 00157 } 00158 00159 if (ret == TSENSOR_OK) 00160 { 00161 /* Configure Temperature Sensor : Conversion 9 bits in continuous mode */ 00162 /* Alert outside range Limit Temperature 12� <-> 24�c */ 00163 STLM75_InitStructure.AlertMode = STLM75_INTERRUPT_MODE; 00164 STLM75_InitStructure.ConversionMode = STLM75_CONTINUOUS_MODE; 00165 STLM75_InitStructure.TemperatureLimitHigh = 24; 00166 STLM75_InitStructure.TemperatureLimitLow = 12; 00167 00168 /* TSENSOR Init */ 00169 tsensor_drv->Init(TSENSORAddress, &STLM75_InitStructure); 00170 00171 ret = TSENSOR_OK; 00172 } 00173 00174 return ret; 00175 } 00176 00177 /** 00178 * @brief Returns the Temperature Sensor status. 00179 * @param None 00180 * @retval The Temperature Sensor status. 00181 */ 00182 uint8_t BSP_TSENSOR_ReadStatus(void) 00183 { 00184 return (tsensor_drv->ReadStatus(TSENSORAddress)); 00185 } 00186 00187 /** 00188 * @brief Read Temperature register of LM75. 00189 * @param None 00190 * @retval STLM75 measured temperature value. 00191 */ 00192 uint16_t BSP_TSENSOR_ReadTemp(void) 00193 { 00194 return tsensor_drv->ReadTemp(TSENSORAddress); 00195 } 00196 00197 /** 00198 * @} 00199 */ 00200 00201 00202 /** 00203 * @} 00204 */ 00205 00206 00207 /** 00208 * @} 00209 */ 00210 00211 /** 00212 * @} 00213 */ 00214 00215 /** 00216 * @} 00217 */ 00218 00219 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:54:25 for STM32L073Z_EVAL BSP User Manual by
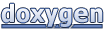