STM32L073Z_EVAL BSP User Manual
|
stm32l073z_eval_sd.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l073z_eval_sd.h 00004 * @author MCD Application Team 00005 * @brief This file contains the common defines and functions prototypes for 00006 * the stm32l073z_eval_sd.c driver. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Define to prevent recursive inclusion -------------------------------------*/ 00038 #ifndef __STM32L073Z_EVAL_SD_H 00039 #define __STM32L073Z_EVAL_SD_H 00040 00041 #ifdef __cplusplus 00042 extern "C" { 00043 #endif 00044 00045 /* Includes ------------------------------------------------------------------*/ 00046 #include "stm32l073z_eval.h" 00047 #include "stm32l073z_eval_io.h" 00048 00049 /** @addtogroup BSP 00050 * @{ 00051 */ 00052 00053 /** @addtogroup STM32L073Z_EVAL 00054 * @{ 00055 */ 00056 00057 /** @addtogroup STM32L073Z_EVAL_SD 00058 * @{ 00059 */ 00060 00061 /** @defgroup STM32L073Z_EVAL_SD_Exported_Types 00062 * @{ 00063 */ 00064 00065 /** 00066 * @brief SD status structure definition 00067 */ 00068 enum { 00069 BSP_SD_OK = 0x00, 00070 MSD_OK = 0x00, 00071 BSP_SD_ERROR = 0x01, 00072 MSD_ERROR = 0x01, 00073 BSP_SD_TIMEOUT 00074 }; 00075 00076 typedef struct 00077 { 00078 uint8_t Reserved1:2; /* Reserved */ 00079 uint16_t DeviceSize:12; /* Device Size */ 00080 uint8_t MaxRdCurrentVDDMin:3; /* Max. read current @ VDD min */ 00081 uint8_t MaxRdCurrentVDDMax:3; /* Max. read current @ VDD max */ 00082 uint8_t MaxWrCurrentVDDMin:3; /* Max. write current @ VDD min */ 00083 uint8_t MaxWrCurrentVDDMax:3; /* Max. write current @ VDD max */ 00084 uint8_t DeviceSizeMul:3; /* Device size multiplier */ 00085 } struct_v1; 00086 00087 00088 typedef struct 00089 { 00090 uint8_t Reserved1:6; /* Reserved */ 00091 uint32_t DeviceSize:22; /* Device Size */ 00092 uint8_t Reserved2:1; /* Reserved */ 00093 } struct_v2; 00094 00095 /** 00096 * @brief Card Specific Data: CSD Register 00097 */ 00098 typedef struct 00099 { 00100 /* Header part */ 00101 uint8_t CSDStruct:2; /* CSD structure */ 00102 // uint8_t SysSpecVersion; /* System specification version */ 00103 uint8_t Reserved1:6; /* Reserved */ 00104 uint8_t TAAC:8; /* Data read access-time 1 */ 00105 uint8_t NSAC:8; /* Data read access-time 2 in CLK cycles */ 00106 uint8_t MaxBusClkFrec:8; /* Max. bus clock frequency */ 00107 uint16_t CardComdClasses:12; /* Card command classes */ 00108 uint8_t RdBlockLen:4; /* Max. read data block length */ 00109 uint8_t PartBlockRead:1; /* Partial blocks for read allowed */ 00110 uint8_t WrBlockMisalign:1; /* Write block misalignment */ 00111 uint8_t RdBlockMisalign:1; /* Read block misalignment */ 00112 uint8_t DSRImpl:1; /* DSR implemented */ 00113 00114 /* v1 or v2 struct */ 00115 union csd_version { 00116 struct_v1 v1; 00117 struct_v2 v2; 00118 } version; 00119 00120 uint8_t EraseSingleBlockEnable:1; /* Erase single block enable */ 00121 uint8_t EraseSectorSize:7; /* Erase group size multiplier */ 00122 uint8_t WrProtectGrSize:7; /* Write protect group size */ 00123 uint8_t WrProtectGrEnable:1; /* Write protect group enable */ 00124 uint8_t Reserved2:2; /* Reserved */ 00125 uint8_t WrSpeedFact:3; /* Write speed factor */ 00126 uint8_t MaxWrBlockLen:4; /* Max. write data block length */ 00127 uint8_t WriteBlockPartial:1; /* Partial blocks for write allowed */ 00128 uint8_t Reserved3:5; /* Reserved */ 00129 uint8_t FileFormatGrouop:1; /* File format group */ 00130 uint8_t CopyFlag:1; /* Copy flag (OTP) */ 00131 uint8_t PermWrProtect:1; /* Permanent write protection */ 00132 uint8_t TempWrProtect:1; /* Temporary write protection */ 00133 uint8_t FileFormat:2; /* File Format */ 00134 uint8_t Reserved4:2; /* Reserved */ 00135 uint8_t crc:7; /* Reserved */ 00136 uint8_t Reserved5:1; /* always 1*/ 00137 00138 } SD_CSD; 00139 00140 /** 00141 * @brief Card Identification Data: CID Register 00142 */ 00143 typedef struct 00144 { 00145 __IO uint8_t ManufacturerID; /* ManufacturerID */ 00146 __IO uint16_t OEM_AppliID; /* OEM/Application ID */ 00147 __IO uint32_t ProdName1; /* Product Name part1 */ 00148 __IO uint8_t ProdName2; /* Product Name part2*/ 00149 __IO uint8_t ProdRev; /* Product Revision */ 00150 __IO uint32_t ProdSN; /* Product Serial Number */ 00151 __IO uint8_t Reserved1; /* Reserved1 */ 00152 __IO uint16_t ManufactDate; /* Manufacturing Date */ 00153 __IO uint8_t CID_CRC; /* CID CRC */ 00154 __IO uint8_t Reserved2; /* always 1 */ 00155 } SD_CID; 00156 00157 /** 00158 * @brief SD Card information 00159 */ 00160 typedef struct 00161 { 00162 SD_CSD Csd; 00163 SD_CID Cid; 00164 uint32_t CardCapacity; /* Card Capacity */ 00165 uint32_t CardBlockSize; /* Card Block Size */ 00166 } SD_CardInfo; 00167 00168 /** 00169 * @} 00170 */ 00171 00172 /** @defgroup STM32L073Z_EVAL_SPI_SD_Exported_Constants 00173 * @{ 00174 */ 00175 00176 /** 00177 * @brief Block Size 00178 */ 00179 #define SD_BLOCK_SIZE 0x200 00180 00181 /** 00182 * @brief SD detection on its memory slot 00183 */ 00184 #define SD_PRESENT ((uint8_t)0x01) 00185 #define SD_NOT_PRESENT ((uint8_t)0x00) 00186 00187 /** 00188 * @} 00189 */ 00190 00191 /** @defgroup STM32L073Z_EVAL_SD_Exported_Macro 00192 * @{ 00193 */ 00194 00195 /** 00196 * @} 00197 */ 00198 00199 /** @defgroup STM32L073Z_EVAL_SD_Exported_Functions 00200 * @{ 00201 */ 00202 uint8_t BSP_SD_Init(void); 00203 uint8_t BSP_SD_IsDetected(void); 00204 uint8_t BSP_SD_ReadBlocks(uint32_t *pData, uint32_t ReadAddr, uint16_t BlockSize, uint32_t NumberOfBlocks); 00205 uint8_t BSP_SD_WriteBlocks(uint32_t *pData, uint32_t WriteAddr, uint16_t BlockSize, uint32_t NumberOfBlocks); 00206 uint8_t BSP_SD_Erase(uint32_t StartAddr, uint32_t EndAddr); 00207 uint8_t BSP_SD_GetStatus(void); 00208 uint8_t BSP_SD_GetCardInfo(SD_CardInfo *pCardInfo); 00209 00210 /* Link functions for SD Card peripheral*/ 00211 void SD_IO_Init(void); 00212 void SD_IO_CSState(uint8_t state); 00213 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00214 uint8_t SD_IO_WriteByte(uint8_t Data); 00215 //uint8_t SD_IO_ReadByte(void); 00216 //uint8_t SD_IO_WriteCmd(uint8_t Cmd, uint32_t Arg, uint8_t Crc, uint8_t answer); 00217 //uint8_t SD_IO_WaitResponse(uint8_t Response); 00218 //void SD_IO_WriteDummy(void); 00219 00220 /** 00221 * @} 00222 */ 00223 00224 /** 00225 * @} 00226 */ 00227 00228 /** 00229 * @} 00230 */ 00231 00232 /** 00233 * @} 00234 */ 00235 00236 #ifdef __cplusplus 00237 } 00238 #endif 00239 00240 #endif /* __STM32L073Z_EVAL_SD_H */ 00241 00242 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:54:25 for STM32L073Z_EVAL BSP User Manual by
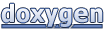