STM32L073Z_EVAL BSP User Manual
|
stm32l073z_eval_lcd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l073z_eval_lcd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the driver for Liquid Crystal Display modules 00006 * mounted on STM32L073Z-EVAL evaluation board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* File Info : ----------------------------------------------------------------- 00038 User NOTES 00039 1. How To use this driver: 00040 -------------------------- 00041 - This driver is used to drive indirectly an LCD TFT. 00042 - This driver supports the AM-240320LDTNQW00H (SPFD5408D) and 00043 AM240320LGTNQW00H (HX8347D) LCD mounted on MB895 daughter board 00044 - The HX8347D components driver MUST be included with this driver. 00045 00046 2. Driver description: 00047 --------------------- 00048 + Initialization steps: 00049 o Initialize the LCD using the LCD_Init() function. 00050 00051 + Display on LCD 00052 o Clear the hole LCD using yhe LCD_Clear() function or only one specified 00053 string line using the LCD_ClearStringLine() function. 00054 o Display a character on the specified line and column using the LCD_DisplayChar() 00055 function or a complete string line using the LCD_DisplayStringAtLine() function. 00056 o Display a string line on the specified position (x,y in pixel) and align mode 00057 using the LCD_DisplayStringAtLine() function. 00058 o Draw and fill a basic shapes (dot, line, rectangle, circle, ellipse, .. bitmap) 00059 on LCD using a set of functions. 00060 00061 ------------------------------------------------------------------------------*/ 00062 00063 /* Includes ------------------------------------------------------------------*/ 00064 #include "stm32l073z_eval_lcd.h" 00065 #include "../../../Utilities/Fonts/fonts.h" 00066 #include "../../../Utilities/Fonts/font24.c" 00067 #include "../../../Utilities/Fonts/font20.c" 00068 #include "../../../Utilities/Fonts/font16.c" 00069 #include "../../../Utilities/Fonts/font12.c" 00070 #include "../../../Utilities/Fonts/font8.c" 00071 00072 /** @addtogroup BSP 00073 * @{ 00074 */ 00075 00076 /** @addtogroup STM32L073Z_EVAL 00077 * @{ 00078 */ 00079 00080 /** @addtogroup STM32L073Z_EVAL_LCD 00081 * @{ 00082 */ 00083 00084 /** @defgroup STM32L073Z_EVAL_LCD_Private_TypesDefinitions STM32L073Z_EVAL_LCD_Private_TypesDefinitions 00085 * @{ 00086 */ 00087 00088 /** 00089 * @} 00090 */ 00091 00092 /** @defgroup STM32L073Z_EVAL_LCD_Private_Defines STM32L073Z_EVAL_LCD_Private_Defines 00093 * @{ 00094 */ 00095 #define POLY_X(Z) ((int32_t)((pPoints + (Z))->X)) 00096 #define POLY_Y(Z) ((int32_t)((pPoints + (Z))->Y)) 00097 00098 #define MAX_HEIGHT_FONT 17 00099 #define MAX_WIDTH_FONT 24 00100 #define OFFSET_BITMAP 54 00101 /** 00102 * @} 00103 */ 00104 00105 /** @defgroup STM32L073Z_EVAL_LCD_Private_Macros STM32L073Z_EVAL_LCD_Private_Macros 00106 * @{ 00107 */ 00108 #define ABS(X) ((X) > 0 ? (X) : -(X)) 00109 00110 /** 00111 * @} 00112 */ 00113 00114 /** @defgroup STM32L073Z_EVAL_LCD_Private_Variables STM32L073Z_EVAL_LCD_Private_Variables 00115 * @{ 00116 */ 00117 LCD_DrawPropTypeDef DrawProp; 00118 00119 static LCD_DrvTypeDef *lcd_drv; 00120 00121 /* Max size of bitmap will based on a font24 (17x24) */ 00122 static uint8_t bitmap[MAX_HEIGHT_FONT*MAX_WIDTH_FONT*2+OFFSET_BITMAP] = {0}; 00123 00124 /** 00125 * @} 00126 */ 00127 00128 /** @defgroup STM32L073Z_EVAL_LCD_Private_FunctionPrototypes STM32L073Z_EVAL_LCD_Private_FunctionPrototypes 00129 * @{ 00130 */ 00131 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode); 00132 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar); 00133 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height); 00134 /** 00135 * @} 00136 */ 00137 00138 00139 /** @defgroup STM32L073Z_EVAL_LCD_Private_Functions STM32L073Z_EVAL_LCD_Private_Functions 00140 * @{ 00141 */ 00142 00143 /** 00144 * @brief Initializes the LCD. 00145 * @param None 00146 * @retval LCD state 00147 */ 00148 uint8_t BSP_LCD_Init(void) 00149 { 00150 uint8_t ret = LCD_ERROR; 00151 00152 /* Default value for draw propriety */ 00153 DrawProp.BackColor = 0xFFFF; 00154 DrawProp.pFont = &Font24; 00155 DrawProp.TextColor = 0x0000; 00156 00157 //if(hx8347d_drv.ReadID() == HX8347D_ID) 00158 // ReadID is not correctly managed by this LCD... 00159 //{ 00160 /*HX8347D_ID connected*/ 00161 lcd_drv = &hx8347d_drv; 00162 ret = LCD_OK; 00163 //} 00164 00165 //if(ret != LCD_ERROR) 00166 //{ 00167 /* LCD Init */ 00168 lcd_drv->Init(); 00169 00170 /* Initialize the font */ 00171 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00172 //} 00173 00174 return ret; 00175 } 00176 00177 /** 00178 * @brief Gets the LCD X size. 00179 * @param None 00180 * @retval Used LCD X size 00181 */ 00182 uint32_t BSP_LCD_GetXSize(void) 00183 { 00184 return(lcd_drv->GetLcdPixelWidth()); 00185 } 00186 00187 /** 00188 * @brief Gets the LCD Y size. 00189 * @param None 00190 * @retval Used LCD Y size 00191 */ 00192 uint32_t BSP_LCD_GetYSize(void) 00193 { 00194 return(lcd_drv->GetLcdPixelHeight()); 00195 } 00196 00197 /** 00198 * @brief Gets the LCD text color. 00199 * @param None 00200 * @retval Used text color. 00201 */ 00202 uint16_t BSP_LCD_GetTextColor(void) 00203 { 00204 return DrawProp.TextColor; 00205 } 00206 00207 /** 00208 * @brief Gets the LCD background color. 00209 * @param None 00210 * @retval Used background color 00211 */ 00212 uint16_t BSP_LCD_GetBackColor(void) 00213 { 00214 return DrawProp.BackColor; 00215 } 00216 00217 /** 00218 * @brief Sets the LCD text color. 00219 * @param Color: Text color code RGB(5-6-5) 00220 * @retval None 00221 */ 00222 void BSP_LCD_SetTextColor(uint16_t Color) 00223 { 00224 DrawProp.TextColor = Color; 00225 } 00226 00227 /** 00228 * @brief Sets the LCD background color. 00229 * @param Color: Background color code RGB(5-6-5) 00230 * @retval None 00231 */ 00232 void BSP_LCD_SetBackColor(uint16_t Color) 00233 { 00234 DrawProp.BackColor = Color; 00235 } 00236 00237 /** 00238 * @brief Sets the LCD text font. 00239 * @param pFonts: Font to be used 00240 * @retval None 00241 */ 00242 void BSP_LCD_SetFont(sFONT *pFonts) 00243 { 00244 DrawProp.pFont = pFonts; 00245 } 00246 00247 /** 00248 * @brief Gets the LCD text font. 00249 * @param None 00250 * @retval Used font 00251 */ 00252 sFONT *BSP_LCD_GetFont(void) 00253 { 00254 return DrawProp.pFont; 00255 } 00256 00257 /** 00258 * @brief Clears the hole LCD. 00259 * @param Color: Color of the background 00260 * @retval None 00261 */ 00262 void BSP_LCD_Clear(uint16_t Color) 00263 { 00264 uint32_t counter = 0; 00265 00266 uint32_t color_backup = DrawProp.TextColor; 00267 DrawProp.TextColor = Color; 00268 00269 for(counter = 0; counter < BSP_LCD_GetYSize(); counter++) 00270 { 00271 BSP_LCD_DrawHLine(0, counter, BSP_LCD_GetXSize()); 00272 } 00273 00274 DrawProp.TextColor = color_backup; 00275 BSP_LCD_SetTextColor(DrawProp.TextColor); 00276 } 00277 00278 /** 00279 * @brief Clears the selected line. 00280 * @param Line: Line to be cleared 00281 * This parameter can be one of the following values: 00282 * @arg 0..9: if the Current fonts is Font16x24 00283 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00284 * @arg 0..29: if the Current fonts is Font8x8 00285 * @retval None 00286 */ 00287 void BSP_LCD_ClearStringLine(uint16_t Line) 00288 { 00289 uint32_t colorbackup = DrawProp.TextColor; 00290 DrawProp.TextColor = DrawProp.BackColor;; 00291 00292 /* Draw a rectangle with background color */ 00293 BSP_LCD_FillRect(0, (Line * DrawProp.pFont->Height), BSP_LCD_GetXSize(), DrawProp.pFont->Height); 00294 00295 DrawProp.TextColor = colorbackup; 00296 BSP_LCD_SetTextColor(DrawProp.TextColor); 00297 } 00298 00299 /** 00300 * @brief Displays one character. 00301 * @param Xpos: Start column address 00302 * @param Ypos: Line where to display the character shape. 00303 * @param Ascii: Character ascii code 00304 * This parameter must be a number between Min_Data = 0x20 and Max_Data = 0x7E 00305 * @retval None 00306 */ 00307 void BSP_LCD_DisplayChar(uint16_t Xpos, uint16_t Ypos, uint8_t Ascii) 00308 { 00309 LCD_DrawChar(Ypos, Xpos, &DrawProp.pFont->table[(Ascii-' ') *\ 00310 DrawProp.pFont->Height * ((DrawProp.pFont->Width + 7) / 8)]); 00311 } 00312 00313 /** 00314 * @brief Displays characters on the LCD. 00315 * @param Xpos: X position (in pixel) 00316 * @param Ypos: Y position (in pixel) 00317 * @param pText: Pointer to string to display on LCD 00318 * @param Mode: Display mode 00319 * This parameter can be one of the following values: 00320 * @arg CENTER_MODE 00321 * @arg RIGHT_MODE 00322 * @arg LEFT_MODE 00323 * @retval None 00324 */ 00325 void BSP_LCD_DisplayStringAt(uint16_t Xpos, uint16_t Ypos, uint8_t *pText, Line_ModeTypdef Mode) 00326 { 00327 uint16_t refcolumn = 1, counter = 0; 00328 uint32_t size = 0, ysize = 0; 00329 uint8_t *ptr = pText; 00330 00331 /* Get the text size */ 00332 while (*ptr++) size ++ ; 00333 00334 /* Characters number per line */ 00335 ysize = (BSP_LCD_GetXSize()/DrawProp.pFont->Width); 00336 00337 switch (Mode) 00338 { 00339 case CENTER_MODE: 00340 { 00341 refcolumn = Xpos + ((ysize - size)* DrawProp.pFont->Width) / 2; 00342 break; 00343 } 00344 case LEFT_MODE: 00345 { 00346 refcolumn = Xpos; 00347 break; 00348 } 00349 case RIGHT_MODE: 00350 { 00351 refcolumn = Xpos + ((ysize - size)*DrawProp.pFont->Width); 00352 break; 00353 } 00354 default: 00355 { 00356 refcolumn = Xpos; 00357 break; 00358 } 00359 } 00360 00361 /* Send the string character by character on lCD */ 00362 while ((*pText != 0) & (((BSP_LCD_GetXSize() - (counter*DrawProp.pFont->Width)) & 0xFFFF) >= DrawProp.pFont->Width)) 00363 { 00364 /* Display one character on LCD */ 00365 BSP_LCD_DisplayChar(refcolumn, Ypos, *pText); 00366 /* Decrement the column position by 16 */ 00367 refcolumn += DrawProp.pFont->Width; 00368 /* Point on the next character */ 00369 pText++; 00370 counter++; 00371 } 00372 } 00373 00374 /** 00375 * @brief Displays a character on the LCD. 00376 * @param Line: Line where to display the character shape 00377 * This parameter can be one of the following values: 00378 * @arg 0..9: if the Current fonts is Font16x24 00379 * @arg 0..19: if the Current fonts is Font12x12 or Font8x12 00380 * @arg 0..29: if the Current fonts is Font8x8 00381 * @param ptr: Pointer to string to display on LCD 00382 * @retval None 00383 */ 00384 void BSP_LCD_DisplayStringAtLine(uint16_t Line, uint8_t *pText) 00385 { 00386 BSP_LCD_DisplayStringAt(0, LINE(Line),pText, LEFT_MODE); 00387 } 00388 00389 /** 00390 * @brief Reads an LCD pixel. 00391 * @param Xpos: X position 00392 * @param Ypos: Y position 00393 * @retval RGB pixel color 00394 */ 00395 uint16_t BSP_LCD_ReadPixel(uint16_t Xpos, uint16_t Ypos) 00396 { 00397 uint16_t ret = 0; 00398 00399 if(lcd_drv->ReadPixel != NULL) 00400 { 00401 ret = lcd_drv->ReadPixel(Xpos, Ypos); 00402 } 00403 00404 return ret; 00405 } 00406 00407 /** 00408 * @brief Draws an pixel. 00409 * @param Xpos: X position 00410 * @param Ypos: Y position 00411 * @param Length: Line length 00412 * @retval None 00413 */ 00414 void BSP_LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos) 00415 { 00416 LCD_DrawPixel(Ypos, Xpos, DrawProp.TextColor); 00417 } 00418 00419 /** 00420 * @brief Draws an horizontal line. 00421 * @param Xpos: X position 00422 * @param Ypos: Y position 00423 * @param Length: Line length 00424 * @retval None 00425 */ 00426 void BSP_LCD_DrawHLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00427 { 00428 uint32_t index = 0; 00429 00430 if(lcd_drv->DrawHLine != NULL) 00431 { 00432 lcd_drv->DrawHLine(DrawProp.TextColor, Ypos, Xpos, Length); 00433 } 00434 else 00435 { 00436 for(index = 0; index < Length; index++) 00437 { 00438 LCD_DrawPixel((Ypos + index), Xpos, DrawProp.TextColor); 00439 } 00440 } 00441 } 00442 00443 /** 00444 * @brief Draws a vertical line. 00445 * @param Xpos: X position 00446 * @param Ypos: Y position 00447 * @param Length: Line length 00448 * @retval None 00449 */ 00450 void BSP_LCD_DrawVLine(uint16_t Xpos, uint16_t Ypos, uint16_t Length) 00451 { 00452 uint32_t index = 0; 00453 00454 if(lcd_drv->DrawVLine != NULL) 00455 { 00456 LCD_SetDisplayWindow(Ypos, Xpos, 1, Length); 00457 lcd_drv->DrawVLine(DrawProp.TextColor, Ypos, Xpos, Length); 00458 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00459 } 00460 else 00461 { 00462 for(index = 0; index < Length; index++) 00463 { 00464 LCD_DrawPixel(Ypos, Xpos + index, DrawProp.TextColor); 00465 } 00466 } 00467 } 00468 00469 /** 00470 * @brief Draws an uni-line (between two points). 00471 * @param X1: Point 1 X position 00472 * @param Y1: Point 1 Y position 00473 * @param X2: Point 2 X position 00474 * @param Y2: Point 2 Y position 00475 * @retval None 00476 */ 00477 void BSP_LCD_DrawLine(uint16_t X1, uint16_t Y1, uint16_t X2, uint16_t Y2) 00478 { 00479 int16_t deltax = 0, deltay = 0, x = 0, y = 0, xinc1 = 0, xinc2 = 0, 00480 yinc1 = 0, yinc2 = 0, den = 0, num = 0, numadd = 0, numpixels = 0, 00481 curpixel = 0; 00482 00483 deltax = ABS(Y2 - Y1); /* The difference between the x's */ 00484 deltay = ABS(X2 - X1); /* The difference between the y's */ 00485 x = Y1; /* Start x off at the first pixel */ 00486 y = X1; /* Start y off at the first pixel */ 00487 00488 if (Y2 >= Y1) /* The x-values are increasing */ 00489 { 00490 xinc1 = 1; 00491 xinc2 = 1; 00492 } 00493 else /* The x-values are decreasing */ 00494 { 00495 xinc1 = -1; 00496 xinc2 = -1; 00497 } 00498 00499 if (X2 >= X1) /* The y-values are increasing */ 00500 { 00501 yinc1 = 1; 00502 yinc2 = 1; 00503 } 00504 else /* The y-values are decreasing */ 00505 { 00506 yinc1 = -1; 00507 yinc2 = -1; 00508 } 00509 00510 if (deltax >= deltay) /* There is at least one x-value for every y-value */ 00511 { 00512 xinc1 = 0; /* Don't change the x when numerator >= denominator */ 00513 yinc2 = 0; /* Don't change the y for every iteration */ 00514 den = deltax; 00515 num = deltax / 2; 00516 numadd = deltay; 00517 numpixels = deltax; /* There are more x-values than y-values */ 00518 } 00519 else /* There is at least one y-value for every x-value */ 00520 { 00521 xinc2 = 0; /* Don't change the x for every iteration */ 00522 yinc1 = 0; /* Don't change the y when numerator >= denominator */ 00523 den = deltay; 00524 num = deltay / 2; 00525 numadd = deltax; 00526 numpixels = deltay; /* There are more y-values than x-values */ 00527 } 00528 00529 for (curpixel = 0; curpixel <= numpixels; curpixel++) 00530 { 00531 LCD_DrawPixel(x, y, DrawProp.TextColor); /* Draw the current pixel */ 00532 num += numadd; /* Increase the numerator by the top of the fraction */ 00533 if (num >= den) /* Check if numerator >= denominator */ 00534 { 00535 num -= den; /* Calculate the new numerator value */ 00536 x += xinc1; /* Change the x as appropriate */ 00537 y += yinc1; /* Change the y as appropriate */ 00538 } 00539 x += xinc2; /* Change the x as appropriate */ 00540 y += yinc2; /* Change the y as appropriate */ 00541 } 00542 } 00543 00544 /** 00545 * @brief Draws a rectangle. 00546 * @param Xpos: X position 00547 * @param Ypos: Y position 00548 * @param Width: Rectangle width 00549 * @param Height: Rectangle height 00550 * @retval None 00551 */ 00552 void BSP_LCD_DrawRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00553 { 00554 /* Draw horizontal lines */ 00555 BSP_LCD_DrawHLine(Xpos, Ypos, Width); 00556 BSP_LCD_DrawHLine(Xpos, (Ypos+ Height), Width); 00557 00558 /* Draw vertical lines */ 00559 BSP_LCD_DrawVLine(Xpos, Ypos, Height); 00560 BSP_LCD_DrawVLine((Xpos + Width), Ypos, Height); 00561 } 00562 00563 /** 00564 * @brief Draws a circle. 00565 * @param Xpos: X position 00566 * @param Ypos: Y position 00567 * @param Radius: Circle radius 00568 * @retval None 00569 */ 00570 void BSP_LCD_DrawCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00571 { 00572 int32_t decision; /* Decision Variable */ 00573 uint32_t curx; /* Current X Value */ 00574 uint32_t cury; /* Current Y Value */ 00575 00576 decision = 3 - (Radius << 1); 00577 curx = 0; 00578 cury = Radius; 00579 00580 while (curx <= cury) 00581 { 00582 LCD_DrawPixel((Ypos + curx), (Xpos - cury), DrawProp.TextColor); 00583 00584 LCD_DrawPixel((Ypos - curx), (Xpos - cury), DrawProp.TextColor); 00585 00586 LCD_DrawPixel((Ypos + cury), (Xpos - curx), DrawProp.TextColor); 00587 00588 LCD_DrawPixel((Ypos - cury), (Xpos - curx), DrawProp.TextColor); 00589 00590 LCD_DrawPixel((Ypos + curx), (Xpos + cury), DrawProp.TextColor); 00591 00592 LCD_DrawPixel((Ypos - curx), (Xpos + cury), DrawProp.TextColor); 00593 00594 LCD_DrawPixel((Ypos + cury), (Xpos + curx), DrawProp.TextColor); 00595 00596 LCD_DrawPixel((Ypos - cury), (Xpos + curx), DrawProp.TextColor); 00597 00598 /* Initialize the font */ 00599 BSP_LCD_SetFont(&LCD_DEFAULT_FONT); 00600 00601 if (decision < 0) 00602 { 00603 decision += (curx << 2) + 6; 00604 } 00605 else 00606 { 00607 decision += ((curx - cury) << 2) + 10; 00608 cury--; 00609 } 00610 curx++; 00611 } 00612 } 00613 00614 /** 00615 * @brief Draws an poly-line (between many points). 00616 * @param pPoints: Pointer to the points array 00617 * @param PointCount: Number of points 00618 * @retval None 00619 */ 00620 void BSP_LCD_DrawPolygon(pPoint pPoints, uint16_t PointCount) 00621 { 00622 int16_t x = 0, y = 0; 00623 00624 if(PointCount < 2) 00625 { 00626 return; 00627 } 00628 00629 BSP_LCD_DrawLine(pPoints->X, pPoints->Y, (pPoints+PointCount-1)->X, (pPoints+PointCount-1)->Y); 00630 00631 while(--PointCount) 00632 { 00633 x = pPoints->X; 00634 y = pPoints->Y; 00635 pPoints++; 00636 BSP_LCD_DrawLine(x, y, pPoints->X, pPoints->Y); 00637 } 00638 00639 } 00640 00641 /** 00642 * @brief Draws an ellipse on LCD. 00643 * @param Xpos: X position 00644 * @param Ypos: Y position 00645 * @param XRadius: Ellipse X radius 00646 * @param YRadius: Ellipse Y radius 00647 * @retval None 00648 */ 00649 void BSP_LCD_DrawEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00650 { 00651 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00652 float k = 0, rad1 = 0, rad2 = 0; 00653 00654 rad1 = YRadius; 00655 rad2 = XRadius; 00656 00657 k = (float)(rad2/rad1); 00658 00659 do { 00660 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00661 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos+y), DrawProp.TextColor); 00662 LCD_DrawPixel((Ypos+(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00663 LCD_DrawPixel((Ypos-(uint16_t)(x/k)), (Xpos-y), DrawProp.TextColor); 00664 00665 e2 = err; 00666 if (e2 <= x) { 00667 err += ++x*2+1; 00668 if (-y == x && e2 <= y) e2 = 0; 00669 } 00670 if (e2 > y) err += ++y*2+1; 00671 } 00672 while (y <= 0); 00673 } 00674 00675 /** 00676 * @brief Draws a bitmap picture loaded in the internal Flash (32 bpp). 00677 * @param Xpos: Bmp X position in the LCD 00678 * @param Ypos: Bmp Y position in the LCD 00679 * @param pBmp: Pointer to Bmp picture address in the internal Flash 00680 * @retval None 00681 */ 00682 void BSP_LCD_DrawBitmap(uint16_t Xpos, uint16_t Ypos, uint8_t *pBmp) 00683 { 00684 uint32_t height = 0, width = 0; 00685 00686 /* Read bitmap width */ 00687 width = *(uint16_t *) (pBmp + 18); 00688 width |= (*(uint16_t *) (pBmp + 20)) << 16; 00689 00690 /* Read bitmap height */ 00691 height = *(uint16_t *) (pBmp + 22); 00692 height |= (*(uint16_t *) (pBmp + 24)) << 16; 00693 00694 /* Remap Ypos, hx8347d works with inverted X in case of bitmap */ 00695 /* X = 0, cursor is on Bottom corner */ 00696 if(lcd_drv == &hx8347d_drv) 00697 { 00698 Ypos = BSP_LCD_GetYSize() - Ypos - height; 00699 } 00700 00701 LCD_SetDisplayWindow(Ypos, Xpos, width, height); 00702 00703 if(lcd_drv->DrawBitmap != NULL) 00704 { 00705 lcd_drv->DrawBitmap(Ypos, Xpos, pBmp); 00706 } 00707 LCD_SetDisplayWindow(0, 0, BSP_LCD_GetXSize(), BSP_LCD_GetYSize()); 00708 } 00709 00710 /** 00711 * @brief Draws a full rectangle. 00712 * @param Xpos: X position 00713 * @param Ypos: Y position 00714 * @param Width: Rectangle width 00715 * @param Height: Rectangle height 00716 * @retval None 00717 */ 00718 void BSP_LCD_FillRect(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00719 { 00720 BSP_LCD_SetTextColor(DrawProp.TextColor); 00721 do 00722 { 00723 BSP_LCD_DrawHLine(Xpos, Ypos++, Width); 00724 } 00725 while(Height--); 00726 } 00727 00728 /** 00729 * @brief Draws a full circle. 00730 * @param Xpos: X position 00731 * @param Ypos: Y position 00732 * @param Radius: Circle radius 00733 * @retval None 00734 */ 00735 void BSP_LCD_FillCircle(uint16_t Xpos, uint16_t Ypos, uint16_t Radius) 00736 { 00737 int32_t decision; /* Decision Variable */ 00738 uint32_t curx; /* Current X Value */ 00739 uint32_t cury; /* Current Y Value */ 00740 00741 decision = 3 - (Radius << 1); 00742 00743 curx = 0; 00744 cury = Radius; 00745 00746 BSP_LCD_SetTextColor(DrawProp.TextColor); 00747 00748 while (curx <= cury) 00749 { 00750 if(cury > 0) 00751 { 00752 BSP_LCD_DrawVLine(Xpos + curx, Ypos - cury, 2*cury); 00753 BSP_LCD_DrawVLine(Xpos - curx, Ypos - cury, 2*cury); 00754 } 00755 00756 if(curx > 0) 00757 { 00758 BSP_LCD_DrawVLine(Xpos - cury, Ypos - curx, 2*curx); 00759 BSP_LCD_DrawVLine(Xpos + cury, Ypos - curx, 2*curx); 00760 } 00761 if (decision < 0) 00762 { 00763 decision += (curx << 2) + 6; 00764 } 00765 else 00766 { 00767 decision += ((curx - cury) << 2) + 10; 00768 cury--; 00769 } 00770 curx++; 00771 } 00772 00773 BSP_LCD_SetTextColor(DrawProp.TextColor); 00774 BSP_LCD_DrawCircle(Xpos, Ypos, Radius); 00775 } 00776 00777 /** 00778 * @brief Draws a full ellipse. 00779 * @param Xpos: X position 00780 * @param Ypos: Y position 00781 * @param XRadius: Ellipse X radius 00782 * @param YRadius: Ellipse Y radius 00783 * @retval None 00784 */ 00785 void BSP_LCD_FillEllipse(int Xpos, int Ypos, int XRadius, int YRadius) 00786 { 00787 int x = 0, y = -XRadius, err = 2-2*YRadius, e2; 00788 float k = 0, rad1 = 0, rad2 = 0; 00789 00790 rad1 = YRadius; 00791 rad2 = XRadius; 00792 00793 k = (float)(rad2/rad1); 00794 00795 do 00796 { 00797 BSP_LCD_DrawVLine((Xpos+y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00798 BSP_LCD_DrawVLine((Xpos-y), (Ypos-(uint16_t)(x/k)), (2*(uint16_t)(x/k) + 1)); 00799 00800 e2 = err; 00801 if (e2 <= x) 00802 { 00803 err += ++x*2+1; 00804 if (-y == x && e2 <= y) e2 = 0; 00805 } 00806 if (e2 > y) err += ++y*2+1; 00807 } 00808 while (y <= 0); 00809 } 00810 00811 /** 00812 * @brief Enables the display. 00813 * @param None 00814 * @retval None 00815 */ 00816 void BSP_LCD_DisplayOn(void) 00817 { 00818 lcd_drv->DisplayOn(); 00819 } 00820 00821 /** 00822 * @brief Disables the display. 00823 * @param None 00824 * @retval None 00825 */ 00826 void BSP_LCD_DisplayOff(void) 00827 { 00828 lcd_drv->DisplayOff(); 00829 } 00830 00831 /****************************************************************************** 00832 Static Function 00833 *******************************************************************************/ 00834 /** 00835 * @brief Draws a pixel on LCD. 00836 * @param Xpos: X position 00837 * @param Ypos: Y position 00838 * @param RGB_Code: Pixel color in RGB mode (5-6-5) 00839 * @retval None 00840 */ 00841 static void LCD_DrawPixel(uint16_t Xpos, uint16_t Ypos, uint16_t RGBCode) 00842 { 00843 if(lcd_drv->WritePixel != NULL) 00844 { 00845 lcd_drv->WritePixel(Xpos, Ypos, RGBCode); 00846 } 00847 } 00848 00849 /** 00850 * @brief Draws a character on LCD. 00851 * @param Xpos: Line where to display the character shape 00852 * @param Ypos: Start column address 00853 * @param pChar: Pointer to the character data 00854 * @retval None 00855 */ 00856 static void LCD_DrawChar(uint16_t Xpos, uint16_t Ypos, const uint8_t *pChar) 00857 { 00858 uint32_t counterh = 0, counterw = 0, index = 0; 00859 uint16_t height = 0, width = 0; 00860 uint8_t offset = 0; 00861 uint8_t *pchar = NULL; 00862 uint32_t line = 0; 00863 00864 height = DrawProp.pFont->Height; 00865 width = DrawProp.pFont->Width; 00866 00867 /* Fill bitmap header*/ 00868 *(uint16_t *) (bitmap + 2) = (uint16_t)(height*width*2+OFFSET_BITMAP); 00869 *(uint16_t *) (bitmap + 4) = (uint16_t)((height*width*2+OFFSET_BITMAP)>>16); 00870 *(uint16_t *) (bitmap + 10) = OFFSET_BITMAP; 00871 *(uint16_t *) (bitmap + 18) = (uint16_t)(width); 00872 *(uint16_t *) (bitmap + 20) = (uint16_t)((width)>>16); 00873 *(uint16_t *) (bitmap + 22) = (uint16_t)(height); 00874 *(uint16_t *) (bitmap + 24) = (uint16_t)((height)>>16); 00875 00876 offset = 8 *((width + 7)/8) - width ; 00877 00878 for(counterh = 0; counterh < height; counterh++) 00879 { 00880 pchar = ((uint8_t *)pChar + (width + 7)/8 * counterh); 00881 00882 if(((width + 7)/8) == 3) 00883 { 00884 line = (pchar[0]<< 16) | (pchar[1]<< 8) | pchar[2]; 00885 } 00886 00887 if(((width + 7)/8) == 2) 00888 { 00889 line = (pchar[0]<< 8) | pchar[1]; 00890 } 00891 00892 if(((width + 7)/8) == 1) 00893 { 00894 line = pchar[0]; 00895 } 00896 00897 for (counterw = 0; counterw < width; counterw++) 00898 { 00899 /* Image in the bitmap is written from the bottom to the top */ 00900 /* Need to invert image in the bitmap */ 00901 index = (((height-counterh-1)*width)+(counterw))*2+OFFSET_BITMAP; 00902 if(line & (1 << (width- counterw + offset- 1))) 00903 { 00904 bitmap[index] = (uint8_t)DrawProp.TextColor; 00905 bitmap[index+1] = (uint8_t)(DrawProp.TextColor >> 8); 00906 } 00907 else 00908 { 00909 bitmap[index] = (uint8_t)DrawProp.BackColor; 00910 bitmap[index+1] = (uint8_t)(DrawProp.BackColor >> 8); 00911 } 00912 } 00913 } 00914 00915 BSP_LCD_DrawBitmap(Ypos, Xpos, bitmap); 00916 } 00917 00918 /** 00919 * @brief Sets display window. 00920 * @param LayerIndex: layer index 00921 * @param Xpos: LCD X position 00922 * @param Ypos: LCD Y position 00923 * @param Width: LCD window width 00924 * @param Height: LCD window height 00925 * @retval None 00926 */ 00927 static void LCD_SetDisplayWindow(uint16_t Xpos, uint16_t Ypos, uint16_t Width, uint16_t Height) 00928 { 00929 if(lcd_drv->SetDisplayWindow != NULL) 00930 { 00931 lcd_drv->SetDisplayWindow(Xpos, Ypos, Width, Height); 00932 } 00933 } 00934 00935 /** 00936 * @} 00937 */ 00938 00939 /** 00940 * @} 00941 */ 00942 00943 /** 00944 * @} 00945 */ 00946 00947 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Mon Aug 28 2017 14:54:25 for STM32L073Z_EVAL BSP User Manual by
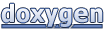