STM32L073Z_EVAL BSP User Manual
|
stm32l073z_eval_idd.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32l073z_eval_idd.c 00004 * @author MCD Application Team 00005 * @brief This file includes the Idd measurement driver for 00006 * STM32L073Z-Eval board. 00007 ****************************************************************************** 00008 * @attention 00009 * 00010 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00011 * 00012 * Redistribution and use in source and binary forms, with or without modification, 00013 * are permitted provided that the following conditions are met: 00014 * 1. Redistributions of source code must retain the above copyright notice, 00015 * this list of conditions and the following disclaimer. 00016 * 2. Redistributions in binary form must reproduce the above copyright notice, 00017 * this list of conditions and the following disclaimer in the documentation 00018 * and/or other materials provided with the distribution. 00019 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00020 * may be used to endorse or promote products derived from this software 00021 * without specific prior written permission. 00022 * 00023 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00024 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00025 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00026 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00027 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00028 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00029 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00030 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00031 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 ****************************************************************************** 00035 */ 00036 00037 /* Includes ------------------------------------------------------------------*/ 00038 #include "stm32l073z_eval_idd.h" 00039 #include "stm32l073z_eval_io.h" 00040 00041 /** @addtogroup BSP 00042 * @{ 00043 */ 00044 00045 /** @addtogroup STM32L073Z_EVAL 00046 * @{ 00047 */ 00048 00049 /** @defgroup STM32L073Z_EVAL_BOARD_IDD STM32L073Z_EVAL_BOARD Idd 00050 * @brief This file includes the Idd driver for STM32L32L073Z_EVAL board. 00051 * It allows user to measure MCU Idd current on board, especially in 00052 * different low power modes. 00053 * @{ 00054 */ 00055 00056 /** @defgroup STM32L073Z_EVAL_BOARD_IDD_Private_Defines IDD Private Defines 00057 * @{ 00058 */ 00059 00060 /** 00061 * @} 00062 */ 00063 00064 00065 /** @defgroup STM32L073Z_EVAL_BOARD_IDD_Private_Variables IDD Private Variables 00066 * @{ 00067 */ 00068 static IDD_DrvTypeDef *IddDrv = NULL; 00069 00070 /** 00071 @verbatim 00072 @endverbatim 00073 */ 00074 00075 00076 /** 00077 * @} 00078 */ 00079 00080 /** @defgroup STM32L073Z_EVAL_BOARD_IDD_Private_Functions IDD Private Functions 00081 * @{ 00082 */ 00083 00084 /** 00085 * @} 00086 */ 00087 00088 /** @defgroup STM32L073Z_EVAL_BOARD_IDD_Exported_Functions IDD Exported Functions 00089 * @{ 00090 */ 00091 00092 /** 00093 * @brief Configures IDD measurement component. 00094 * @param None 00095 * @retval IDD_OK if no problem during initialization 00096 */ 00097 uint8_t BSP_IDD_Init(void) 00098 { 00099 IDD_ConfigTypeDef iddconfig = {0}; 00100 uint8_t mfxstm32l152_id = 0; 00101 uint8_t ret = 0; 00102 00103 /* Read ID and verify if the MFX is ready */ 00104 mfxstm32l152_id = mfxstm32l152_idd_drv.ReadID(IDD_I2C_ADDRESS); 00105 00106 if((mfxstm32l152_id == MFXSTM32L152_ID_1) || (mfxstm32l152_id == MFXSTM32L152_ID_2)) 00107 { 00108 00109 /* Initialize the TS driver structure */ 00110 IddDrv = &mfxstm32l152_idd_drv; 00111 00112 /* Initialize the Idd driver */ 00113 if(IddDrv->Init != NULL) 00114 { 00115 IddDrv->Init(IDD_I2C_ADDRESS); 00116 } 00117 00118 /* Configure Idd component with default values */ 00119 iddconfig.AmpliGain = EVAL_IDD_AMPLI_GAIN; 00120 iddconfig.VddMin = EVAL_IDD_VDD_MIN; 00121 iddconfig.Shunt0Value = EVAL_IDD_SHUNT0_VALUE; 00122 iddconfig.Shunt1Value = EVAL_IDD_SHUNT1_VALUE; 00123 iddconfig.Shunt2Value = EVAL_IDD_SHUNT2_VALUE; 00124 iddconfig.Shunt3Value = EVAL_IDD_SHUNT3_VALUE; 00125 iddconfig.Shunt4Value = EVAL_IDD_SHUNT4_VALUE; 00126 iddconfig.Shunt0StabDelay = EVAL_IDD_SHUNT0_STABDELAY; 00127 iddconfig.Shunt1StabDelay = EVAL_IDD_SHUNT1_STABDELAY; 00128 iddconfig.Shunt2StabDelay = EVAL_IDD_SHUNT2_STABDELAY; 00129 iddconfig.Shunt3StabDelay = EVAL_IDD_SHUNT3_STABDELAY; 00130 iddconfig.Shunt4StabDelay = EVAL_IDD_SHUNT4_STABDELAY; 00131 iddconfig.ShuntNbOnBoard = MFXSTM32L152_IDD_SHUNT_NB_5; 00132 iddconfig.ShuntNbUsed = MFXSTM32L152_IDD_SHUNT_NB_5; 00133 iddconfig.VrefMeasurement = MFXSTM32L152_IDD_VREF_AUTO_MEASUREMENT_ENABLE; 00134 iddconfig.Calibration = MFXSTM32L152_IDD_AUTO_CALIBRATION_ENABLE; 00135 iddconfig.PreDelayUnit = MFXSTM32L152_IDD_PREDELAY_20_MS; 00136 iddconfig.PreDelayValue = 0x7F; 00137 iddconfig.MeasureNb = 100; 00138 iddconfig.DeltaDelayUnit= MFXSTM32L152_IDD_DELTADELAY_0_5_MS; 00139 iddconfig.DeltaDelayValue = 10; 00140 00141 BSP_IDD_Config(iddconfig); 00142 00143 ret = IDD_OK; 00144 } 00145 else 00146 { 00147 ret = IDD_ERROR; 00148 } 00149 00150 return ret; 00151 } 00152 00153 /** 00154 * @brief Reset Idd measurement component. 00155 * @retval None 00156 */ 00157 void BSP_IDD_Reset(void) 00158 { 00159 uint8_t mfxstm32l152_id = 0; 00160 00161 /* Read ID and verify if the MFX is ready */ 00162 mfxstm32l152_id = mfxstm32l152_idd_drv.ReadID(IDD_I2C_ADDRESS); 00163 00164 if((mfxstm32l152_id == MFXSTM32L152_ID_1) || (mfxstm32l152_id == MFXSTM32L152_ID_2)) 00165 { 00166 /* Initialize the TS driver structure */ 00167 IddDrv = &mfxstm32l152_idd_drv; 00168 if(IddDrv->Reset != NULL) 00169 { 00170 IddDrv->Reset(IDD_I2C_ADDRESS); 00171 } 00172 } 00173 } 00174 00175 /** 00176 * @brief Turn Idd measurement component in low power (standby/sleep) mode 00177 * @retval None 00178 */ 00179 void BSP_IDD_LowPower(void) 00180 { 00181 if(IddDrv->LowPower != NULL) 00182 { 00183 IddDrv->LowPower(IDD_I2C_ADDRESS); 00184 } 00185 } 00186 00187 /** 00188 * @brief Start Measurement campaign 00189 * @param None 00190 * @retval None 00191 */ 00192 void BSP_IDD_StartMeasure(void) 00193 { 00194 if(IddDrv->Start != NULL) 00195 { 00196 IddDrv->Start(IDD_I2C_ADDRESS); 00197 } 00198 } 00199 00200 /** 00201 * @brief Configure Idd component 00202 * @param IddConfig: structure of idd parameters 00203 * @retval None 00204 */ 00205 void BSP_IDD_Config(IDD_ConfigTypeDef IddConfig) 00206 { 00207 if(IddDrv->Config != NULL) 00208 { 00209 IddDrv->Config(IDD_I2C_ADDRESS, IddConfig); 00210 } 00211 } 00212 00213 /** 00214 * @brief Get Idd current value. 00215 * @param IddValue: Pointer on u32 to store Idd. Value unit is 10 nA. 00216 * @retval None 00217 */ 00218 void BSP_IDD_GetValue(uint32_t *IddValue) 00219 { 00220 if(IddDrv->GetValue != NULL) 00221 { 00222 IddDrv->GetValue(IDD_I2C_ADDRESS, IddValue); 00223 } 00224 } 00225 00226 /** 00227 * @brief Enable Idd interrupt that warn end of measurement 00228 * @param None 00229 * @retval None 00230 */ 00231 void BSP_IDD_EnableIT(void) 00232 { 00233 if(IddDrv->EnableIT != NULL) 00234 { 00235 IddDrv->EnableIT(IDD_I2C_ADDRESS); 00236 } 00237 } 00238 00239 /** 00240 * @brief Clear Idd interrupt that warn end of measurement 00241 * @param None 00242 * @retval None 00243 */ 00244 void BSP_IDD_ClearIT(void) 00245 { 00246 if(IddDrv->ClearIT != NULL) 00247 { 00248 IddDrv->ClearIT(IDD_I2C_ADDRESS); 00249 } 00250 } 00251 00252 /** 00253 * @brief Get Idd interrupt status 00254 * @param Return 00255 * @retval None 00256 */ 00257 uint8_t BSP_IDD_GetITStatus(void) 00258 { 00259 if(IddDrv->GetITStatus != NULL) 00260 { 00261 return (IddDrv->GetITStatus(IDD_I2C_ADDRESS)); 00262 } 00263 else 00264 { 00265 return IDD_ERROR; 00266 } 00267 } 00268 00269 /** 00270 * @brief Disable Idd interrupt that warn end of measurement 00271 * @param None 00272 * @retval None 00273 */ 00274 void BSP_IDD_DisableIT(void) 00275 { 00276 if(IddDrv->DisableIT != NULL) 00277 { 00278 IddDrv->DisableIT(IDD_I2C_ADDRESS); 00279 } 00280 } 00281 00282 /** 00283 * @brief Get Error Code . 00284 * @param None. 00285 * @retval Error code or error 00286 */ 00287 uint8_t BSP_IDD_ErrorGetCode(void) 00288 { 00289 00290 if(IddDrv->ErrorGetSrc != NULL) 00291 { 00292 if((IddDrv->ErrorGetSrc(IDD_I2C_ADDRESS) & MFXSTM32L152_IDD_ERROR_SRC) != RESET) 00293 { 00294 if(IddDrv->ErrorGetCode != NULL) 00295 { 00296 return IddDrv->ErrorGetCode(IDD_I2C_ADDRESS); 00297 } 00298 else 00299 { 00300 return IDD_ERROR; 00301 } 00302 } 00303 else 00304 { 00305 return IDD_ERROR; 00306 } 00307 } 00308 else 00309 { 00310 return IDD_ERROR; 00311 } 00312 00313 } 00314 00315 00316 /** 00317 * @brief Enable error interrupt that warn end of measurement 00318 * @param None 00319 * @retval None 00320 */ 00321 void BSP_IDD_ErrorEnableIT(void) 00322 { 00323 if(IddDrv->ErrorEnableIT != NULL) 00324 { 00325 IddDrv->ErrorEnableIT(IDD_I2C_ADDRESS); 00326 } 00327 } 00328 00329 /** 00330 * @brief Clear Error interrupt that warn end of measurement 00331 * @param None 00332 * @retval None 00333 */ 00334 void BSP_IDD_ErrorClearIT(void) 00335 { 00336 if(IddDrv->ErrorClearIT != NULL) 00337 { 00338 IddDrv->ErrorClearIT(IDD_I2C_ADDRESS); 00339 } 00340 } 00341 00342 /** 00343 * @brief Get Error interrupt status 00344 * @param Return 00345 * @retval None 00346 */ 00347 uint8_t BSP_IDD_ErrorGetITStatus(void) 00348 { 00349 if(IddDrv->ErrorGetITStatus != NULL) 00350 { 00351 return (IddDrv->ErrorGetITStatus(IDD_I2C_ADDRESS)); 00352 } 00353 else 00354 { 00355 return 0; 00356 } 00357 } 00358 00359 /** 00360 * @brief Disable Error interrupt 00361 * @param None 00362 * @retval None 00363 */ 00364 void BSP_IDD_ErrorDisableIT(void) 00365 { 00366 if(IddDrv->ErrorDisableIT != NULL) 00367 { 00368 IddDrv->ErrorDisableIT(IDD_I2C_ADDRESS); 00369 } 00370 } 00371 00372 /** 00373 * @brief Wakes up Idd measurement component. 00374 * @retval None 00375 */ 00376 void BSP_IDD_WakeUp(void) 00377 { 00378 /* Set Wakeup pin to high to wakeup Idd measurement compont form standby mode */ 00379 HAL_GPIO_WritePin(IDD_WAKEUP_GPIO_PORT, IDD_WAKEUP_PIN, GPIO_PIN_SET); 00380 00381 /* Wait */ 00382 HAL_Delay(1); 00383 00384 /* Set gpio pin basck to low */ 00385 HAL_GPIO_WritePin(IDD_WAKEUP_GPIO_PORT, IDD_WAKEUP_PIN, GPIO_PIN_RESET); 00386 } 00387 00388 00389 /** 00390 * @} 00391 */ 00392 00393 /** 00394 * @} 00395 */ 00396 00397 /** 00398 * @} 00399 */ 00400 00401 /** 00402 * @} 00403 */ 00404 00405 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00406
Generated on Mon Aug 28 2017 14:54:25 for STM32L073Z_EVAL BSP User Manual by
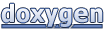