STM32F401-Discovery BSP User Manual
|
Functions | |
uint32_t | BSP_GetVersion (void) |
This method returns the STM32F401 DISCO BSP Driver revision. | |
void | BSP_LED_Init (Led_TypeDef Led) |
Configures LED GPIO. | |
void | BSP_LED_On (Led_TypeDef Led) |
Turns selected LED On. | |
void | BSP_LED_Off (Led_TypeDef Led) |
Turns selected LED Off. | |
void | BSP_LED_Toggle (Led_TypeDef Led) |
Toggles the selected LED. | |
void | BSP_PB_Init (Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) |
Configures Button GPIO and EXTI Line. | |
uint32_t | BSP_PB_GetState (Button_TypeDef Button) |
Returns the selected Button state. | |
static void | I2Cx_WriteData (uint16_t Addr, uint8_t Reg, uint8_t Value) |
Writes a value in a register of the device through BUS. | |
static uint8_t | I2Cx_ReadData (uint16_t Addr, uint8_t Reg) |
Reads a register of the device through BUS. | |
static void | I2Cx_MspInit (I2C_HandleTypeDef *hi2c) |
I2Cx MSP Init. | |
static uint8_t | SPIx_WriteRead (uint8_t Byte) |
Sends a Byte through the SPI interface and return the Byte received from the SPI bus. | |
static void | SPIx_MspInit (SPI_HandleTypeDef *hspi) |
SPI MSP Init. | |
void | GYRO_IO_Write (uint8_t *pBuffer, uint8_t WriteAddr, uint16_t NumByteToWrite) |
Writes one byte to the GYRO. | |
void | GYRO_IO_Read (uint8_t *pBuffer, uint8_t ReadAddr, uint16_t NumByteToRead) |
Reads a block of data from the GYRO. | |
void | AUDIO_IO_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
Writes a single data. | |
uint8_t | AUDIO_IO_Read (uint8_t Addr, uint8_t Reg) |
Reads a single data. | |
void | COMPASSACCELERO_IO_Write (uint16_t DeviceAddr, uint8_t RegisterAddr, uint8_t Value) |
Writes one byte to the COMPASS / ACCELERO. | |
uint8_t | COMPASSACCELERO_IO_Read (uint16_t DeviceAddr, uint8_t RegisterAddr) |
Reads a block of data from the COMPASS / ACCELERO. |
Function Documentation
uint8_t AUDIO_IO_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) |
Reads a single data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address
- Return values:
-
Data to be read
Definition at line 659 of file stm32f401_discovery.c.
References I2Cx_ReadData().
void AUDIO_IO_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
Writes a single data.
- Parameters:
-
Addr,: I2C address Reg,: Reg address Value,: Data to be written
Definition at line 648 of file stm32f401_discovery.c.
References I2Cx_WriteData().
uint32_t BSP_GetVersion | ( | void | ) |
This method returns the STM32F401 DISCO BSP Driver revision.
- Return values:
-
version,: 0xXYZR (8bits for each decimal, R for RC)
Definition at line 159 of file stm32f401_discovery.c.
References __STM32F401_DISCO_BSP_VERSION.
void BSP_LED_Init | ( | Led_TypeDef | Led | ) |
Configures LED GPIO.
- Parameters:
-
Led,: Specifies the Led to be configured. This parameter can be one of following parameters: - LED4
- LED3
- LED5
- LED6
Definition at line 173 of file stm32f401_discovery.c.
References GPIO_PIN, GPIO_PORT, and LEDx_GPIO_CLK_ENABLE.
void BSP_LED_Off | ( | Led_TypeDef | Led | ) |
Turns selected LED Off.
- Parameters:
-
Led,: Specifies the Led to be set off. This parameter can be one of following parameters: - LED4
- LED3
- LED5
- LED6
Definition at line 214 of file stm32f401_discovery.c.
void BSP_LED_On | ( | Led_TypeDef | Led | ) |
Turns selected LED On.
- Parameters:
-
Led,: Specifies the Led to be set on. This parameter can be one of following parameters: - LED4
- LED3
- LED5
- LED6
Definition at line 200 of file stm32f401_discovery.c.
void BSP_LED_Toggle | ( | Led_TypeDef | Led | ) |
Toggles the selected LED.
- Parameters:
-
Led,: Specifies the Led to be toggled. This parameter can be one of following parameters: - LED4
- LED3
- LED5
- LED6
Definition at line 228 of file stm32f401_discovery.c.
uint32_t BSP_PB_GetState | ( | Button_TypeDef | Button | ) |
Returns the selected Button state.
- Parameters:
-
Button,: Specifies the Button to be checked. This parameter should be: BUTTON_KEY
- Return values:
-
The Button GPIO pin value.
Definition at line 280 of file stm32f401_discovery.c.
References BUTTON_PIN, and BUTTON_PORT.
void BSP_PB_Init | ( | Button_TypeDef | Button, |
ButtonMode_TypeDef | ButtonMode | ||
) |
Configures Button GPIO and EXTI Line.
- Parameters:
-
Button,: Specifies the Button to be configured. This parameter should be: BUTTON_KEY ButtonMode,: Specifies Button mode. This parameter can be one of following parameters: - BUTTON_MODE_GPIO: Button will be used as simple IO
- BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt generation capability
Definition at line 243 of file stm32f401_discovery.c.
References BUTTON_IRQn, BUTTON_MODE_EXTI, BUTTON_MODE_GPIO, BUTTON_PIN, BUTTON_PORT, and BUTTONx_GPIO_CLK_ENABLE.
uint8_t COMPASSACCELERO_IO_Read | ( | uint16_t | DeviceAddr, |
uint8_t | RegisterAddr | ||
) |
Reads a block of data from the COMPASS / ACCELERO.
- Parameters:
-
DeviceAddr,: the slave address to be programmed(ACC_I2C_ADDRESS or MAG_I2C_ADDRESS). RegisterAddr,: the COMPASS / ACCELERO internal address register to read from
- Return values:
-
COMPASS / ACCELERO register value
Definition at line 726 of file stm32f401_discovery.c.
References I2Cx_ReadData().
void COMPASSACCELERO_IO_Write | ( | uint16_t | DeviceAddr, |
uint8_t | RegisterAddr, | ||
uint8_t | Value | ||
) |
Writes one byte to the COMPASS / ACCELERO.
- Parameters:
-
DeviceAddr,: the slave address to be programmed RegisterAddr,: the COMPASS / ACCELERO register to be written Value,: Data to be written
Definition at line 714 of file stm32f401_discovery.c.
References I2Cx_WriteData().
void GYRO_IO_Read | ( | uint8_t * | pBuffer, |
uint8_t | ReadAddr, | ||
uint16_t | NumByteToRead | ||
) |
Reads a block of data from the GYRO.
- Parameters:
-
pBuffer,: pointer to the buffer that receives the data read from the GYRO. ReadAddr,: GYRO's internal address to read from. NumByteToRead,: Number of bytes to read from the GYRO.
Definition at line 570 of file stm32f401_discovery.c.
References DUMMY_BYTE, GYRO_CS_HIGH, GYRO_CS_LOW, MULTIPLEBYTE_CMD, READWRITE_CMD, and SPIx_WriteRead().
void GYRO_IO_Write | ( | uint8_t * | pBuffer, |
uint8_t | WriteAddr, | ||
uint16_t | NumByteToWrite | ||
) |
Writes one byte to the GYRO.
- Parameters:
-
pBuffer,: pointer to the buffer containing the data to be written to the GYRO. WriteAddr : GYRO's internal address to write to. NumByteToWrite,: Number of bytes to write.
Definition at line 536 of file stm32f401_discovery.c.
References GYRO_CS_HIGH, GYRO_CS_LOW, MULTIPLEBYTE_CMD, and SPIx_WriteRead().
static void I2Cx_MspInit | ( | I2C_HandleTypeDef * | hi2c | ) | [static] |
I2Cx MSP Init.
- Parameters:
-
hi2c,: I2C handle
Definition at line 372 of file stm32f401_discovery.c.
References DISCOVERY_I2Cx_AF, DISCOVERY_I2Cx_CLOCK_ENABLE, DISCOVERY_I2Cx_ER_IRQn, DISCOVERY_I2Cx_EV_IRQn, DISCOVERY_I2Cx_FORCE_RESET, DISCOVERY_I2Cx_GPIO_CLK_ENABLE, DISCOVERY_I2Cx_GPIO_PORT, DISCOVERY_I2Cx_RELEASE_RESET, DISCOVERY_I2Cx_SCL_PIN, and DISCOVERY_I2Cx_SDA_PIN.
Referenced by I2Cx_Init().
static uint8_t I2Cx_ReadData | ( | uint16_t | Addr, |
uint8_t | Reg | ||
) | [static] |
Reads a register of the device through BUS.
- Parameters:
-
Addr,: Device address on BUS Bus. Reg,: The target register address to write
- Return values:
-
Data read at register address
Definition at line 340 of file stm32f401_discovery.c.
References I2cHandle, I2Cx_Error(), and I2cxTimeout.
Referenced by AUDIO_IO_Read(), and COMPASSACCELERO_IO_Read().
static void I2Cx_WriteData | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) | [static] |
Writes a value in a register of the device through BUS.
- Parameters:
-
Addr,: Device address on BUS Bus. Reg,: The target register address to write Value,: The target register value to be written
Definition at line 320 of file stm32f401_discovery.c.
References I2cHandle, I2Cx_Error(), and I2cxTimeout.
Referenced by AUDIO_IO_Write(), and COMPASSACCELERO_IO_Write().
static void SPIx_MspInit | ( | SPI_HandleTypeDef * | hspi | ) | [static] |
SPI MSP Init.
- Parameters:
-
hspi,: SPI handle
Definition at line 476 of file stm32f401_discovery.c.
References DISCOVERY_SPIx_AF, DISCOVERY_SPIx_CLOCK_ENABLE, DISCOVERY_SPIx_GPIO_CLK_ENABLE, DISCOVERY_SPIx_GPIO_PORT, DISCOVERY_SPIx_MISO_PIN, DISCOVERY_SPIx_MOSI_PIN, and DISCOVERY_SPIx_SCK_PIN.
Referenced by SPIx_Init().
static uint8_t SPIx_WriteRead | ( | uint8_t | Byte | ) | [static] |
Sends a Byte through the SPI interface and return the Byte received from the SPI bus.
- Parameters:
-
Byte,: Byte send.
- Return values:
-
The received byte value
Definition at line 446 of file stm32f401_discovery.c.
References SpiHandle, SPIx_Error(), and SpixTimeout.
Referenced by GYRO_IO_Read(), and GYRO_IO_Write().
Generated on Thu Jan 19 2017 16:53:47 for STM32F401-Discovery BSP User Manual by
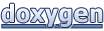