STM32F1xx_Nucleo BSP User Manual
|
Functions | |
static void | SPIx_Init (void) |
Initialize SPI HAL. | |
static void | SPIx_Write (uint8_t Value) |
SPI Write a byte to device. | |
static void | SPIx_WriteData (uint8_t *DataIn, uint16_t DataLength) |
SPI Write an amount of data to device. | |
static void | SPIx_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) |
SPI Write a byte to device. | |
static void | SPIx_Error (void) |
SPI error treatment function. | |
static void | SPIx_MspInit (void) |
Initialize SPI MSP. | |
void | SD_IO_Init (void) |
Initialize the SD Card and put it into StandBy State (Ready for data transfer). | |
void | SD_IO_CSState (uint8_t val) |
Set the SD_CS pin. | |
void | SD_IO_WriteReadData (const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) |
Write byte(s) on the SD. | |
void | SD_IO_ReadData (uint8_t *DataOut, uint16_t DataLength) |
Write an amount of data on the SD. | |
void | SD_IO_WriteData (const uint8_t *Data, uint16_t DataLength) |
Write an amount of data on the SD. | |
uint8_t | SD_IO_WriteByte (uint8_t Data) |
Write a byte on the SD. | |
uint8_t | SD_IO_ReadByte (void) |
void | LCD_IO_Init (void) |
Initialize the LCD. | |
void | LCD_IO_WriteData (uint8_t Data) |
void | LCD_IO_WriteMultipleData (uint8_t *pData, uint32_t Size) |
Write register value. | |
void | LCD_IO_WriteReg (uint8_t LCDReg) |
Write command to select the LCD register. | |
void | LCD_Delay (uint32_t Delay) |
Wait for loop in ms. | |
static HAL_StatusTypeDef | ADCx_Init (void) |
Initializes ADC HAL. | |
static void | ADCx_DeInit (void) |
Initializes ADC HAL. | |
static void | ADCx_MspInit (ADC_HandleTypeDef *hadc) |
Initialize ADC MSP. | |
static void | ADCx_MspDeInit (ADC_HandleTypeDef *hadc) |
DeInitializes ADC MSP. | |
uint8_t | BSP_JOY_Init (void) |
Configures joystick available on adafruit 1.8" TFT shield managed through ADC to detect motion. | |
void | BSP_JOY_DeInit (void) |
DeInit joystick GPIOs. | |
JOYState_TypeDef | BSP_JOY_GetState (void) |
Returns the Joystick key pressed. |
Function Documentation
static void ADCx_DeInit | ( | void | ) | [static] |
Initializes ADC HAL.
- Parameters:
-
None
- Return values:
-
None
Definition at line 804 of file stm32f1xx_nucleo.c.
References ADCx_MspDeInit(), hnucleo_Adc, and NUCLEO_ADCx.
Referenced by BSP_JOY_DeInit().
static HAL_StatusTypeDef ADCx_Init | ( | void | ) | [static] |
Initializes ADC HAL.
- Return values:
-
None
Definition at line 764 of file stm32f1xx_nucleo.c.
References ADCx_MspInit(), hnucleo_Adc, and NUCLEO_ADCx.
Referenced by BSP_JOY_Init().
static void ADCx_MspDeInit | ( | ADC_HandleTypeDef * | hadc | ) | [static] |
DeInitializes ADC MSP.
- Parameters:
-
None
- Note:
- ADC DeInit does not disable the GPIO clock
- Return values:
-
None
Definition at line 744 of file stm32f1xx_nucleo.c.
References NUCLEO_ADCx_CLK_DISABLE, NUCLEO_ADCx_GPIO_PIN, and NUCLEO_ADCx_GPIO_PORT.
Referenced by ADCx_DeInit().
static void ADCx_MspInit | ( | ADC_HandleTypeDef * | hadc | ) | [static] |
Initialize ADC MSP.
- Return values:
-
None
Definition at line 718 of file stm32f1xx_nucleo.c.
References NUCLEO_ADCx_CLK_ENABLE, NUCLEO_ADCx_GPIO_CLK_ENABLE, NUCLEO_ADCx_GPIO_PIN, and NUCLEO_ADCx_GPIO_PORT.
Referenced by ADCx_Init().
void BSP_JOY_DeInit | ( | void | ) |
DeInit joystick GPIOs.
- Note:
- JOY DeInit does not disable the Mfx, just set the Mfx pins in Off mode
- Return values:
-
None.
Definition at line 840 of file stm32f1xx_nucleo.c.
References ADCx_DeInit().
JOYState_TypeDef BSP_JOY_GetState | ( | void | ) |
Returns the Joystick key pressed.
- Note:
- To know which Joystick key is pressed we need to detect the voltage level on each key output
- None : 3.3 V / 4095
- SEL : 1.055 V / 1308
- DOWN : 0.71 V / 88
- LEFT : 3.0 V / 3720
- RIGHT : 0.595 V / 737
- UP : 1.65 V / 2046
- Return values:
-
JOYState_TypeDef,: Code of the Joystick key pressed.
Definition at line 857 of file stm32f1xx_nucleo.c.
References hnucleo_Adc, JOY_DOWN, JOY_LEFT, JOY_NONE, JOY_RIGHT, JOY_SEL, and JOY_UP.
uint8_t BSP_JOY_Init | ( | void | ) |
Configures joystick available on adafruit 1.8" TFT shield managed through ADC to detect motion.
- Return values:
-
Joystickstatus (0=> success, 1=> fail)
Definition at line 819 of file stm32f1xx_nucleo.c.
References ADCx_Init(), hnucleo_Adc, and sConfig.
void LCD_Delay | ( | uint32_t | Delay | ) |
Wait for loop in ms.
- Parameters:
-
Delay in ms.
- Return values:
-
None
Definition at line 705 of file stm32f1xx_nucleo.c.
void LCD_IO_Init | ( | void | ) |
Initialize the LCD.
- Return values:
-
None
Definition at line 603 of file stm32f1xx_nucleo.c.
References LCD_CS_GPIO_CLK_ENABLE, LCD_CS_HIGH, LCD_CS_PIN, LCD_DC_GPIO_CLK_ENABLE, LCD_DC_GPIO_PORT, LCD_DC_PIN, SD_CS_GPIO_PORT, and SPIx_Init().
void LCD_IO_WriteData | ( | uint8_t | Data | ) |
void LCD_IO_WriteMultipleData | ( | uint8_t * | pData, |
uint32_t | Size | ||
) |
Write register value.
- Parameters:
-
pData Pointer on the register value Size Size of byte to transmit to the register
- Return values:
-
None
Definition at line 654 of file stm32f1xx_nucleo.c.
References hnucleo_Spi, LCD_CS_HIGH, LCD_CS_LOW, LCD_DC_HIGH, and SPIx_Write().
void LCD_IO_WriteReg | ( | uint8_t | LCDReg | ) |
Write command to select the LCD register.
- Parameters:
-
LCDReg,: Address of the selected register.
- Return values:
-
None
Definition at line 633 of file stm32f1xx_nucleo.c.
References LCD_CS_HIGH, LCD_CS_LOW, LCD_DC_LOW, and SPIx_Write().
void SD_IO_CSState | ( | uint8_t | val | ) |
Set the SD_CS pin.
- Parameters:
-
pin value.
- Return values:
-
None
Definition at line 535 of file stm32f1xx_nucleo.c.
References SD_CS_HIGH, and SD_CS_LOW.
void SD_IO_Init | ( | void | ) |
Initialize the SD Card and put it into StandBy State (Ready for data transfer).
- Return values:
-
None
Definition at line 492 of file stm32f1xx_nucleo.c.
References LCD_CS_HIGH, LCD_CS_PIN, SD_CS_GPIO_CLK_ENABLE, SD_CS_GPIO_PORT, SD_CS_HIGH, SD_CS_PIN, SD_DUMMY_BYTE, SD_IO_WriteByte(), and SPIx_Init().
uint8_t SD_IO_ReadByte | ( | void | ) |
void SD_IO_ReadData | ( | uint8_t * | DataOut, |
uint16_t | DataLength | ||
) |
Write an amount of data on the SD.
- Parameters:
-
Data,: byte to send. DataLength,: number of bytes to write
- Return values:
-
none
Definition at line 580 of file stm32f1xx_nucleo.c.
References SD_IO_WriteReadData().
uint8_t SD_IO_WriteByte | ( | uint8_t | Data | ) |
Write a byte on the SD.
- Parameters:
-
Data,: byte to send.
- Return values:
-
Data written
Definition at line 565 of file stm32f1xx_nucleo.c.
References SPIx_WriteReadData().
Referenced by SD_IO_Init().
void SD_IO_WriteData | ( | const uint8_t * | Data, |
uint16_t | DataLength | ||
) |
Write an amount of data on the SD.
- Parameters:
-
Data,: byte to send. DataLength,: number of bytes to write
- Return values:
-
none
Definition at line 592 of file stm32f1xx_nucleo.c.
References SPIx_WriteData().
void SD_IO_WriteReadData | ( | const uint8_t * | DataIn, |
uint8_t * | DataOut, | ||
uint16_t | DataLength | ||
) |
Write byte(s) on the SD.
- Parameters:
-
DataIn,: Pointer to data buffer to write DataOut,: Pointer to data buffer for read data DataLength,: number of bytes to write
- Return values:
-
None
Definition at line 554 of file stm32f1xx_nucleo.c.
References SPIx_WriteReadData().
Referenced by SD_IO_ReadData().
static void SPIx_Error | ( | void | ) | [static] |
SPI error treatment function.
- Return values:
-
None
Definition at line 473 of file stm32f1xx_nucleo.c.
References hnucleo_Spi, and SPIx_Init().
Referenced by SPIx_Write(), SPIx_WriteData(), and SPIx_WriteReadData().
static void SPIx_Init | ( | void | ) | [static] |
Initialize SPI HAL.
- Return values:
-
None
Definition at line 379 of file stm32f1xx_nucleo.c.
References hnucleo_Spi, NUCLEO_SPIx, and SPIx_MspInit().
Referenced by LCD_IO_Init(), SD_IO_Init(), and SPIx_Error().
static void SPIx_MspInit | ( | void | ) | [static] |
Initialize SPI MSP.
- Return values:
-
None
Definition at line 347 of file stm32f1xx_nucleo.c.
References NUCLEO_SPIx_CLK_ENABLE, NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE, NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, NUCLEO_SPIx_MISO_PIN, NUCLEO_SPIx_MOSI_PIN, NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE, NUCLEO_SPIx_SCK_GPIO_PORT, and NUCLEO_SPIx_SCK_PIN.
Referenced by SPIx_Init().
static void SPIx_Write | ( | uint8_t | Value | ) | [static] |
SPI Write a byte to device.
- Parameters:
-
Value,: value to be written
- Return values:
-
None
Definition at line 454 of file stm32f1xx_nucleo.c.
References hnucleo_Spi, SPIx_Error(), and SpixTimeout.
Referenced by LCD_IO_WriteMultipleData(), and LCD_IO_WriteReg().
static void SPIx_WriteData | ( | uint8_t * | DataIn, |
uint16_t | DataLength | ||
) | [static] |
SPI Write an amount of data to device.
- Parameters:
-
Value,: value to be written DataLength,: number of bytes to write
- Return values:
-
None
Definition at line 435 of file stm32f1xx_nucleo.c.
References hnucleo_Spi, SPIx_Error(), and SpixTimeout.
Referenced by SD_IO_WriteData().
static void SPIx_WriteReadData | ( | const uint8_t * | DataIn, |
uint8_t * | DataOut, | ||
uint16_t | DataLength | ||
) | [static] |
SPI Write a byte to device.
- Parameters:
-
Value,: value to be written
- Return values:
-
None
Definition at line 415 of file stm32f1xx_nucleo.c.
References hnucleo_Spi, SPIx_Error(), and SpixTimeout.
Referenced by SD_IO_WriteByte(), and SD_IO_WriteReadData().
Generated on Fri Mar 3 2017 19:17:51 for STM32F1xx_Nucleo BSP User Manual by
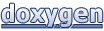