STM32F1xx_Nucleo BSP User Manual
|
stm32f1xx_nucleo.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f1xx_nucleo.c 00004 * @author MCD Application Team 00005 * @version V1.0.3 00006 * @date 29-April-2016 00007 * @brief This file provides set of firmware functions to manage: 00008 * - LEDs and push-button available on STM32F1XX-Nucleo Kit 00009 * from STMicroelectronics 00010 * - LCD, joystick and microSD available on Adafruit 1.8" TFT LCD 00011 * shield (reference ID 802) 00012 ****************************************************************************** 00013 * @attention 00014 * 00015 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00016 * 00017 * Redistribution and use in source and binary forms, with or without modification, 00018 * are permitted provided that the following conditions are met: 00019 * 1. Redistributions of source code must retain the above copyright notice, 00020 * this list of conditions and the following disclaimer. 00021 * 2. Redistributions in binary form must reproduce the above copyright notice, 00022 * this list of conditions and the following disclaimer in the documentation 00023 * and/or other materials provided with the distribution. 00024 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00025 * may be used to endorse or promote products derived from this software 00026 * without specific prior written permission. 00027 * 00028 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00029 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00030 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00031 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00032 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00033 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00034 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00035 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00036 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00037 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00038 * 00039 ****************************************************************************** 00040 */ 00041 00042 /* Includes ------------------------------------------------------------------*/ 00043 #include "stm32f1xx_nucleo.h" 00044 00045 /** @addtogroup BSP 00046 * @{ 00047 */ 00048 00049 /** @defgroup STM32F1XX_NUCLEO STM32F103RB-Nucleo 00050 * @brief This file provides set of firmware functions to manage Leds and push-button 00051 * available on STM32F1XX-Nucleo Kit from STMicroelectronics. 00052 * It provides also LCD, joystick and uSD functions to communicate with 00053 * Adafruit 1.8" TFT LCD shield (reference ID 802) 00054 * @{ 00055 */ 00056 00057 00058 /** @defgroup STM32F1XX_NUCLEO_Private_Defines Private Defines 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief STM32F103RB NUCLEO BSP Driver version 00064 */ 00065 #define __STM32F1XX_NUCLEO_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00066 #define __STM32F1XX_NUCLEO_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00067 #define __STM32F1XX_NUCLEO_BSP_VERSION_SUB2 (0x03) /*!< [15:8] sub2 version */ 00068 #define __STM32F1XX_NUCLEO_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00069 #define __STM32F1XX_NUCLEO_BSP_VERSION ((__STM32F1XX_NUCLEO_BSP_VERSION_MAIN << 24)\ 00070 |(__STM32F1XX_NUCLEO_BSP_VERSION_SUB1 << 16)\ 00071 |(__STM32F1XX_NUCLEO_BSP_VERSION_SUB2 << 8 )\ 00072 |(__STM32F1XX_NUCLEO_BSP_VERSION_RC)) 00073 00074 /** 00075 * @brief LINK SD Card 00076 */ 00077 #define SD_DUMMY_BYTE 0xFF 00078 #define SD_NO_RESPONSE_EXPECTED 0x80 00079 00080 /** 00081 * @} 00082 */ 00083 00084 00085 /** @defgroup STM32F1XX_NUCLEO_Private_Variables Private Variables 00086 * @{ 00087 */ 00088 GPIO_TypeDef* LED_PORT[LEDn] = {LED2_GPIO_PORT}; 00089 00090 const uint16_t LED_PIN[LEDn] = {LED2_PIN}; 00091 00092 GPIO_TypeDef* BUTTON_PORT[BUTTONn] = {USER_BUTTON_GPIO_PORT}; 00093 const uint16_t BUTTON_PIN[BUTTONn] = {USER_BUTTON_PIN}; 00094 const uint8_t BUTTON_IRQn[BUTTONn] = {USER_BUTTON_EXTI_IRQn }; 00095 00096 /** 00097 * @brief BUS variables 00098 */ 00099 00100 #ifdef HAL_SPI_MODULE_ENABLED 00101 uint32_t SpixTimeout = NUCLEO_SPIx_TIMEOUT_MAX; /*<! Value of Timeout when SPI communication fails */ 00102 static SPI_HandleTypeDef hnucleo_Spi; 00103 #endif /* HAL_SPI_MODULE_ENABLED */ 00104 00105 #ifdef HAL_ADC_MODULE_ENABLED 00106 static ADC_HandleTypeDef hnucleo_Adc; 00107 /* ADC channel configuration structure declaration */ 00108 static ADC_ChannelConfTypeDef sConfig; 00109 #endif /* HAL_ADC_MODULE_ENABLED */ 00110 00111 /** 00112 * @} 00113 */ 00114 00115 /** @defgroup STM32F1XX_NUCLEO_Private_Functions Private Functions 00116 * @{ 00117 */ 00118 #ifdef HAL_SPI_MODULE_ENABLED 00119 static void SPIx_Init(void); 00120 static void SPIx_Write(uint8_t Value); 00121 static void SPIx_WriteData(uint8_t *DataIn, uint16_t DataLength); 00122 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLegnth); 00123 static void SPIx_Error (void); 00124 static void SPIx_MspInit(void); 00125 00126 /* SD IO functions */ 00127 void SD_IO_Init(void); 00128 void SD_IO_CSState(uint8_t state); 00129 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength); 00130 void SD_IO_ReadData(uint8_t *DataOut, uint16_t DataLength); 00131 void SD_IO_WriteData(const uint8_t *Data, uint16_t DataLength); 00132 uint8_t SD_IO_WriteByte(uint8_t Data); 00133 uint8_t SD_IO_ReadByte(void); 00134 00135 /* LCD IO functions */ 00136 void LCD_IO_Init(void); 00137 void LCD_IO_WriteData(uint8_t Data); 00138 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size); 00139 void LCD_IO_WriteReg(uint8_t LCDReg); 00140 void LCD_Delay(uint32_t delay); 00141 #endif /* HAL_SPI_MODULE_ENABLED */ 00142 00143 #ifdef HAL_ADC_MODULE_ENABLED 00144 static HAL_StatusTypeDef ADCx_Init(void); 00145 static void ADCx_DeInit(void); 00146 static void ADCx_MspInit(ADC_HandleTypeDef *hadc); 00147 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc); 00148 #endif /* HAL_ADC_MODULE_ENABLED */ 00149 /** 00150 * @} 00151 */ 00152 00153 /** @defgroup STM32F1XX_NUCLEO_Exported_Functions Exported Functions 00154 * @{ 00155 */ 00156 00157 /** 00158 * @brief This method returns the STM32F1XX NUCLEO BSP Driver revision 00159 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00160 */ 00161 uint32_t BSP_GetVersion(void) 00162 { 00163 return __STM32F1XX_NUCLEO_BSP_VERSION; 00164 } 00165 00166 /** @defgroup STM32F1XX_NUCLEO_LED_Functions LED Functions 00167 * @{ 00168 */ 00169 00170 /** 00171 * @brief Configures LED GPIO. 00172 * @param Led: Led to be configured. 00173 * This parameter can be one of the following values: 00174 * @arg LED2 00175 * @retval None 00176 */ 00177 void BSP_LED_Init(Led_TypeDef Led) 00178 { 00179 GPIO_InitTypeDef gpioinitstruct; 00180 00181 /* Enable the GPIO_LED Clock */ 00182 LEDx_GPIO_CLK_ENABLE(Led); 00183 00184 /* Configure the GPIO_LED pin */ 00185 gpioinitstruct.Pin = LED_PIN[Led]; 00186 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00187 gpioinitstruct.Pull = GPIO_NOPULL; 00188 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00189 00190 HAL_GPIO_Init(LED_PORT[Led], &gpioinitstruct); 00191 00192 /* Reset PIN to switch off the LED */ 00193 HAL_GPIO_WritePin(LED_PORT[Led],LED_PIN[Led], GPIO_PIN_RESET); 00194 } 00195 00196 /** 00197 * @brief DeInit LEDs. 00198 * @param Led: LED to be de-init. 00199 * This parameter can be one of the following values: 00200 * @arg LED2 00201 * @note Led DeInit does not disable the GPIO clock nor disable the Mfx 00202 * @retval None 00203 */ 00204 void BSP_LED_DeInit(Led_TypeDef Led) 00205 { 00206 GPIO_InitTypeDef gpio_init_structure; 00207 00208 /* Turn off LED */ 00209 HAL_GPIO_WritePin(LED_PORT[Led],LED_PIN[Led], GPIO_PIN_RESET); 00210 /* DeInit the GPIO_LED pin */ 00211 gpio_init_structure.Pin = LED_PIN[Led]; 00212 HAL_GPIO_DeInit(LED_PORT[Led], gpio_init_structure.Pin); 00213 } 00214 00215 /** 00216 * @brief Turns selected LED On. 00217 * @param Led: Specifies the Led to be set on. 00218 * This parameter can be one of following parameters: 00219 * @arg LED2 00220 * @retval None 00221 */ 00222 void BSP_LED_On(Led_TypeDef Led) 00223 { 00224 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00225 } 00226 00227 /** 00228 * @brief Turns selected LED Off. 00229 * @param Led: Specifies the Led to be set off. 00230 * This parameter can be one of following parameters: 00231 * @arg LED2 00232 * @retval None 00233 */ 00234 void BSP_LED_Off(Led_TypeDef Led) 00235 { 00236 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00237 } 00238 00239 /** 00240 * @brief Toggles the selected LED. 00241 * @param Led: Specifies the Led to be toggled. 00242 * This parameter can be one of following parameters: 00243 * @arg LED2 00244 * @retval None 00245 */ 00246 void BSP_LED_Toggle(Led_TypeDef Led) 00247 { 00248 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00249 } 00250 00251 /** 00252 * @} 00253 */ 00254 00255 /** @defgroup STM32F1XX_NUCLEO_BUTTON_Functions BUTTON Functions 00256 * @{ 00257 */ 00258 00259 /** 00260 * @brief Configures Button GPIO and EXTI Line. 00261 * @param Button: Specifies the Button to be configured. 00262 * This parameter should be: BUTTON_USER 00263 * @param ButtonMode: Specifies Button mode. 00264 * This parameter can be one of following parameters: 00265 * @arg BUTTON_MODE_GPIO: Button will be used as simple IO 00266 * @arg BUTTON_MODE_EXTI: Button will be connected to EXTI line with interrupt 00267 * generation capability 00268 * @retval None 00269 */ 00270 void BSP_PB_Init(Button_TypeDef Button, ButtonMode_TypeDef ButtonMode) 00271 { 00272 GPIO_InitTypeDef gpioinitstruct; 00273 00274 /* Enable the BUTTON Clock */ 00275 BUTTONx_GPIO_CLK_ENABLE(Button); 00276 00277 gpioinitstruct.Pin = BUTTON_PIN[Button]; 00278 gpioinitstruct.Pull = GPIO_NOPULL; 00279 gpioinitstruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 00280 00281 if (ButtonMode == BUTTON_MODE_GPIO) 00282 { 00283 /* Configure Button pin as input */ 00284 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00285 00286 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00287 } 00288 00289 if (ButtonMode == BUTTON_MODE_EXTI) 00290 { 00291 /* Configure Button pin as input with External interrupt */ 00292 gpioinitstruct.Mode = GPIO_MODE_IT_FALLING; 00293 HAL_GPIO_Init(BUTTON_PORT[Button], &gpioinitstruct); 00294 00295 /* Enable and set Button EXTI Interrupt to the lowest priority */ 00296 HAL_NVIC_SetPriority((IRQn_Type)(BUTTON_IRQn[Button]), 0x0F, 0); 00297 HAL_NVIC_EnableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00298 } 00299 } 00300 00301 /** 00302 * @brief Push Button DeInit. 00303 * @param Button: Button to be configured 00304 * This parameter should be: BUTTON_USER 00305 * @note PB DeInit does not disable the GPIO clock 00306 * @retval None 00307 */ 00308 void BSP_PB_DeInit(Button_TypeDef Button) 00309 { 00310 GPIO_InitTypeDef gpio_init_structure; 00311 00312 gpio_init_structure.Pin = BUTTON_PIN[Button]; 00313 HAL_NVIC_DisableIRQ((IRQn_Type)(BUTTON_IRQn[Button])); 00314 HAL_GPIO_DeInit(BUTTON_PORT[Button], gpio_init_structure.Pin); 00315 } 00316 00317 /** 00318 * @brief Returns the selected Button state. 00319 * @param Button: Specifies the Button to be checked. 00320 * This parameter should be: BUTTON_USER 00321 * @retval Button state. 00322 */ 00323 uint32_t BSP_PB_GetState(Button_TypeDef Button) 00324 { 00325 return HAL_GPIO_ReadPin(BUTTON_PORT[Button], BUTTON_PIN[Button]); 00326 } 00327 /** 00328 * @} 00329 */ 00330 00331 /** 00332 * @} 00333 */ 00334 00335 /** @addtogroup STM32F1XX_NUCLEO_Private_Functions 00336 * @{ 00337 */ 00338 00339 #ifdef HAL_SPI_MODULE_ENABLED 00340 /****************************************************************************** 00341 BUS OPERATIONS 00342 *******************************************************************************/ 00343 /** 00344 * @brief Initialize SPI MSP. 00345 * @retval None 00346 */ 00347 static void SPIx_MspInit(void) 00348 { 00349 GPIO_InitTypeDef gpioinitstruct = {0}; 00350 00351 /*** Configure the GPIOs ***/ 00352 /* Enable GPIO clock */ 00353 NUCLEO_SPIx_SCK_GPIO_CLK_ENABLE(); 00354 NUCLEO_SPIx_MISO_MOSI_GPIO_CLK_ENABLE(); 00355 00356 /* Configure SPI SCK */ 00357 gpioinitstruct.Pin = NUCLEO_SPIx_SCK_PIN; 00358 gpioinitstruct.Mode = GPIO_MODE_AF_PP; 00359 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00360 HAL_GPIO_Init(NUCLEO_SPIx_SCK_GPIO_PORT, &gpioinitstruct); 00361 00362 /* Configure SPI MISO and MOSI */ 00363 gpioinitstruct.Pin = NUCLEO_SPIx_MOSI_PIN; 00364 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00365 00366 gpioinitstruct.Pin = NUCLEO_SPIx_MISO_PIN; 00367 gpioinitstruct.Mode = GPIO_MODE_INPUT; 00368 HAL_GPIO_Init(NUCLEO_SPIx_MISO_MOSI_GPIO_PORT, &gpioinitstruct); 00369 00370 /*** Configure the SPI peripheral ***/ 00371 /* Enable SPI clock */ 00372 NUCLEO_SPIx_CLK_ENABLE(); 00373 } 00374 00375 /** 00376 * @brief Initialize SPI HAL. 00377 * @retval None 00378 */ 00379 static void SPIx_Init(void) 00380 { 00381 if(HAL_SPI_GetState(&hnucleo_Spi) == HAL_SPI_STATE_RESET) 00382 { 00383 /* SPI Config */ 00384 hnucleo_Spi.Instance = NUCLEO_SPIx; 00385 /* SPI baudrate is set to 8 MHz maximum (PCLK2/SPI_BaudRatePrescaler = 64/8 = 8 MHz) 00386 to verify these constraints: 00387 - ST7735 LCD SPI interface max baudrate is 15MHz for write and 6.66MHz for read 00388 Since the provided driver doesn't use read capability from LCD, only constraint 00389 on write baudrate is considered. 00390 - SD card SPI interface max baudrate is 25MHz for write/read 00391 - PCLK2 max frequency is 32 MHz 00392 */ 00393 hnucleo_Spi.Init.BaudRatePrescaler = SPI_BAUDRATEPRESCALER_8; 00394 hnucleo_Spi.Init.Direction = SPI_DIRECTION_2LINES; 00395 hnucleo_Spi.Init.CLKPhase = SPI_PHASE_1EDGE; 00396 hnucleo_Spi.Init.CLKPolarity = SPI_POLARITY_LOW; 00397 hnucleo_Spi.Init.CRCCalculation = SPI_CRCCALCULATION_DISABLE; 00398 hnucleo_Spi.Init.CRCPolynomial = 7; 00399 hnucleo_Spi.Init.DataSize = SPI_DATASIZE_8BIT; 00400 hnucleo_Spi.Init.FirstBit = SPI_FIRSTBIT_MSB; 00401 hnucleo_Spi.Init.NSS = SPI_NSS_SOFT; 00402 hnucleo_Spi.Init.TIMode = SPI_TIMODE_DISABLE; 00403 hnucleo_Spi.Init.Mode = SPI_MODE_MASTER; 00404 00405 SPIx_MspInit(); 00406 HAL_SPI_Init(&hnucleo_Spi); 00407 } 00408 } 00409 00410 /** 00411 * @brief SPI Write a byte to device 00412 * @param Value: value to be written 00413 * @retval None 00414 */ 00415 static void SPIx_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00416 { 00417 HAL_StatusTypeDef status = HAL_OK; 00418 00419 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) DataIn, DataOut, DataLength, SpixTimeout); 00420 00421 /* Check the communication status */ 00422 if(status != HAL_OK) 00423 { 00424 /* Execute user timeout callback */ 00425 SPIx_Error(); 00426 } 00427 } 00428 00429 /** 00430 * @brief SPI Write an amount of data to device 00431 * @param Value: value to be written 00432 * @param DataLength: number of bytes to write 00433 * @retval None 00434 */ 00435 static void SPIx_WriteData(uint8_t *DataIn, uint16_t DataLength) 00436 { 00437 HAL_StatusTypeDef status = HAL_OK; 00438 00439 status = HAL_SPI_Transmit(&hnucleo_Spi, DataIn, DataLength, SpixTimeout); 00440 00441 /* Check the communication status */ 00442 if(status != HAL_OK) 00443 { 00444 /* Execute user timeout callback */ 00445 SPIx_Error(); 00446 } 00447 } 00448 00449 /** 00450 * @brief SPI Write a byte to device 00451 * @param Value: value to be written 00452 * @retval None 00453 */ 00454 static void SPIx_Write(uint8_t Value) 00455 { 00456 HAL_StatusTypeDef status = HAL_OK; 00457 uint8_t data; 00458 00459 status = HAL_SPI_TransmitReceive(&hnucleo_Spi, (uint8_t*) &Value, &data, 1, SpixTimeout); 00460 00461 /* Check the communication status */ 00462 if(status != HAL_OK) 00463 { 00464 /* Execute user timeout callback */ 00465 SPIx_Error(); 00466 } 00467 } 00468 00469 /** 00470 * @brief SPI error treatment function 00471 * @retval None 00472 */ 00473 static void SPIx_Error (void) 00474 { 00475 /* De-initialize the SPI communication BUS */ 00476 HAL_SPI_DeInit(&hnucleo_Spi); 00477 00478 /* Re-Initiaize the SPI communication BUS */ 00479 SPIx_Init(); 00480 } 00481 00482 /****************************************************************************** 00483 LINK OPERATIONS 00484 *******************************************************************************/ 00485 00486 /********************************* LINK SD ************************************/ 00487 /** 00488 * @brief Initialize the SD Card and put it into StandBy State (Ready for 00489 * data transfer). 00490 * @retval None 00491 */ 00492 void SD_IO_Init(void) 00493 { 00494 GPIO_InitTypeDef gpioinitstruct = {0}; 00495 uint8_t counter = 0; 00496 00497 /* SD_CS_GPIO Periph clock enable */ 00498 SD_CS_GPIO_CLK_ENABLE(); 00499 00500 /* Configure SD_CS_PIN pin: SD Card CS pin */ 00501 gpioinitstruct.Pin = SD_CS_PIN; 00502 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00503 gpioinitstruct.Pull = GPIO_PULLUP; 00504 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00505 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00506 00507 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00508 gpioinitstruct.Pin = LCD_CS_PIN; 00509 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00510 gpioinitstruct.Pull = GPIO_NOPULL; 00511 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00512 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00513 LCD_CS_HIGH(); 00514 /*------------Put SD in SPI mode--------------*/ 00515 /* SD SPI Config */ 00516 SPIx_Init(); 00517 00518 /* SD chip select high */ 00519 SD_CS_HIGH(); 00520 00521 /* Send dummy byte 0xFF, 10 times with CS high */ 00522 /* Rise CS and MOSI for 80 clocks cycles */ 00523 for (counter = 0; counter <= 9; counter++) 00524 { 00525 /* Send dummy byte 0xFF */ 00526 SD_IO_WriteByte(SD_DUMMY_BYTE); 00527 } 00528 } 00529 00530 /** 00531 * @brief Set the SD_CS pin. 00532 * @param pin value. 00533 * @retval None 00534 */ 00535 void SD_IO_CSState(uint8_t val) 00536 { 00537 if(val == 1) 00538 { 00539 SD_CS_HIGH(); 00540 } 00541 else 00542 { 00543 SD_CS_LOW(); 00544 } 00545 } 00546 00547 /** 00548 * @brief Write byte(s) on the SD 00549 * @param DataIn: Pointer to data buffer to write 00550 * @param DataOut: Pointer to data buffer for read data 00551 * @param DataLength: number of bytes to write 00552 * @retval None 00553 */ 00554 void SD_IO_WriteReadData(const uint8_t *DataIn, uint8_t *DataOut, uint16_t DataLength) 00555 { 00556 /* Send the byte */ 00557 SPIx_WriteReadData(DataIn, DataOut, DataLength); 00558 } 00559 00560 /** 00561 * @brief Write a byte on the SD. 00562 * @param Data: byte to send. 00563 * @retval Data written 00564 */ 00565 uint8_t SD_IO_WriteByte(uint8_t Data) 00566 { 00567 uint8_t tmp; 00568 00569 /* Send the byte */ 00570 SPIx_WriteReadData(&Data,&tmp,1); 00571 return tmp; 00572 } 00573 00574 /** 00575 * @brief Write an amount of data on the SD. 00576 * @param Data: byte to send. 00577 * @param DataLength: number of bytes to write 00578 * @retval none 00579 */ 00580 void SD_IO_ReadData(uint8_t *DataOut, uint16_t DataLength) 00581 { 00582 /* Send the byte */ 00583 SD_IO_WriteReadData(DataOut, DataOut, DataLength); 00584 } 00585 00586 /** 00587 * @brief Write an amount of data on the SD. 00588 * @param Data: byte to send. 00589 * @param DataLength: number of bytes to write 00590 * @retval none 00591 */ 00592 void SD_IO_WriteData(const uint8_t *Data, uint16_t DataLength) 00593 { 00594 /* Send the byte */ 00595 SPIx_WriteData((uint8_t *)Data, DataLength); 00596 } 00597 00598 /********************************* LINK LCD ***********************************/ 00599 /** 00600 * @brief Initialize the LCD 00601 * @retval None 00602 */ 00603 void LCD_IO_Init(void) 00604 { 00605 GPIO_InitTypeDef gpioinitstruct; 00606 00607 /* LCD_CS_GPIO and LCD_DC_GPIO Periph clock enable */ 00608 LCD_CS_GPIO_CLK_ENABLE(); 00609 LCD_DC_GPIO_CLK_ENABLE(); 00610 00611 /* Configure LCD_CS_PIN pin: LCD Card CS pin */ 00612 gpioinitstruct.Pin = LCD_CS_PIN; 00613 gpioinitstruct.Mode = GPIO_MODE_OUTPUT_PP; 00614 gpioinitstruct.Speed = GPIO_SPEED_FREQ_HIGH; 00615 HAL_GPIO_Init(SD_CS_GPIO_PORT, &gpioinitstruct); 00616 00617 /* Configure LCD_DC_PIN pin: LCD Card DC pin */ 00618 gpioinitstruct.Pin = LCD_DC_PIN; 00619 HAL_GPIO_Init(LCD_DC_GPIO_PORT, &gpioinitstruct); 00620 00621 /* LCD chip select high */ 00622 LCD_CS_HIGH(); 00623 00624 /* LCD SPI Config */ 00625 SPIx_Init(); 00626 } 00627 00628 /** 00629 * @brief Write command to select the LCD register. 00630 * @param LCDReg: Address of the selected register. 00631 * @retval None 00632 */ 00633 void LCD_IO_WriteReg(uint8_t LCDReg) 00634 { 00635 /* Reset LCD control line CS */ 00636 LCD_CS_LOW(); 00637 00638 /* Set LCD data/command line DC to Low */ 00639 LCD_DC_LOW(); 00640 00641 /* Send Command */ 00642 SPIx_Write(LCDReg); 00643 00644 /* Deselect : Chip Select high */ 00645 LCD_CS_HIGH(); 00646 } 00647 00648 /** 00649 * @brief Write register value. 00650 * @param pData Pointer on the register value 00651 * @param Size Size of byte to transmit to the register 00652 * @retval None 00653 */ 00654 void LCD_IO_WriteMultipleData(uint8_t *pData, uint32_t Size) 00655 { 00656 uint32_t counter = 0; 00657 00658 /* Reset LCD control line CS */ 00659 LCD_CS_LOW(); 00660 00661 /* Set LCD data/command line DC to High */ 00662 LCD_DC_HIGH(); 00663 00664 if (Size == 1) 00665 { 00666 /* Only 1 byte to be sent to LCD - general interface can be used */ 00667 /* Send Data */ 00668 SPIx_Write(*pData); 00669 } 00670 else 00671 { 00672 /* Several data should be sent in a raw */ 00673 /* Direct SPI accesses for optimization */ 00674 for (counter = Size; counter != 0; counter--) 00675 { 00676 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00677 { 00678 } 00679 /* Need to invert bytes for LCD*/ 00680 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *(pData+1); 00681 00682 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_TXE) != SPI_FLAG_TXE) 00683 { 00684 } 00685 *((__IO uint8_t*)&hnucleo_Spi.Instance->DR) = *pData; 00686 counter--; 00687 pData += 2; 00688 } 00689 00690 /* Wait until the bus is ready before releasing Chip select */ 00691 while(((hnucleo_Spi.Instance->SR) & SPI_FLAG_BSY) != RESET) 00692 { 00693 } 00694 } 00695 00696 /* Deselect : Chip Select high */ 00697 LCD_CS_HIGH(); 00698 } 00699 00700 /** 00701 * @brief Wait for loop in ms. 00702 * @param Delay in ms. 00703 * @retval None 00704 */ 00705 void LCD_Delay(uint32_t Delay) 00706 { 00707 HAL_Delay(Delay); 00708 } 00709 00710 #endif /* HAL_SPI_MODULE_ENABLED */ 00711 00712 #ifdef HAL_ADC_MODULE_ENABLED 00713 /******************************* LINK JOYSTICK ********************************/ 00714 /** 00715 * @brief Initialize ADC MSP. 00716 * @retval None 00717 */ 00718 static void ADCx_MspInit(ADC_HandleTypeDef *hadc) 00719 { 00720 GPIO_InitTypeDef gpioinitstruct; 00721 00722 /*** Configure the GPIOs ***/ 00723 /* Enable GPIO clock */ 00724 NUCLEO_ADCx_GPIO_CLK_ENABLE(); 00725 00726 /* Configure ADC1 Channel8 as analog input */ 00727 gpioinitstruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00728 gpioinitstruct.Mode = GPIO_MODE_ANALOG; 00729 gpioinitstruct.Pull = GPIO_NOPULL; 00730 gpioinitstruct.Speed = GPIO_SPEED_FREQ_MEDIUM; 00731 HAL_GPIO_Init(NUCLEO_ADCx_GPIO_PORT, &gpioinitstruct); 00732 00733 /*** Configure the ADC peripheral ***/ 00734 /* Enable ADC clock */ 00735 NUCLEO_ADCx_CLK_ENABLE(); 00736 } 00737 00738 /** 00739 * @brief DeInitializes ADC MSP. 00740 * @param None 00741 * @note ADC DeInit does not disable the GPIO clock 00742 * @retval None 00743 */ 00744 static void ADCx_MspDeInit(ADC_HandleTypeDef *hadc) 00745 { 00746 GPIO_InitTypeDef gpioinitstruct; 00747 00748 /*** DeInit the ADC peripheral ***/ 00749 /* Disable ADC clock */ 00750 NUCLEO_ADCx_CLK_DISABLE(); 00751 00752 /* Configure the selected ADC Channel as analog input */ 00753 gpioinitstruct.Pin = NUCLEO_ADCx_GPIO_PIN ; 00754 HAL_GPIO_DeInit(NUCLEO_ADCx_GPIO_PORT, gpioinitstruct.Pin); 00755 00756 /* Disable GPIO clock has to be done by the application*/ 00757 /* NUCLEO_ADCx_GPIO_CLK_DISABLE(); */ 00758 } 00759 00760 /** 00761 * @brief Initializes ADC HAL. 00762 * @retval None 00763 */ 00764 static HAL_StatusTypeDef ADCx_Init(void) 00765 { 00766 /* Set ADC instance */ 00767 hnucleo_Adc.Instance = NUCLEO_ADCx; 00768 00769 if(HAL_ADC_GetState(&hnucleo_Adc) == HAL_ADC_STATE_RESET) 00770 { 00771 /* ADC Config */ 00772 hnucleo_Adc.Init.DataAlign = ADC_DATAALIGN_RIGHT; 00773 hnucleo_Adc.Init.ScanConvMode = ADC_SCAN_DISABLE; 00774 hnucleo_Adc.Init.ContinuousConvMode = DISABLE; 00775 hnucleo_Adc.Init.NbrOfConversion = 1; 00776 hnucleo_Adc.Init.DiscontinuousConvMode = DISABLE; 00777 hnucleo_Adc.Init.NbrOfDiscConversion = 1; 00778 hnucleo_Adc.Init.ExternalTrigConv = ADC_SOFTWARE_START; 00779 00780 /* Initialize MSP related to ADC */ 00781 ADCx_MspInit(&hnucleo_Adc); 00782 00783 /* Initialize ADC */ 00784 if (HAL_ADC_Init(&hnucleo_Adc) != HAL_OK) 00785 { 00786 return HAL_ERROR; 00787 } 00788 00789 /* Run ADC calibration */ 00790 if (HAL_ADCEx_Calibration_Start(&hnucleo_Adc) != HAL_OK) 00791 { 00792 return HAL_ERROR; 00793 } 00794 } 00795 00796 return HAL_OK; 00797 } 00798 00799 /** 00800 * @brief Initializes ADC HAL. 00801 * @param None 00802 * @retval None 00803 */ 00804 static void ADCx_DeInit(void) 00805 { 00806 hnucleo_Adc.Instance = NUCLEO_ADCx; 00807 00808 HAL_ADC_DeInit(&hnucleo_Adc); 00809 ADCx_MspDeInit(&hnucleo_Adc); 00810 } 00811 00812 /******************************* LINK JOYSTICK ********************************/ 00813 00814 /** 00815 * @brief Configures joystick available on adafruit 1.8" TFT shield 00816 * managed through ADC to detect motion. 00817 * @retval Joystickstatus (0=> success, 1=> fail) 00818 */ 00819 uint8_t BSP_JOY_Init(void) 00820 { 00821 if (ADCx_Init() != HAL_OK) 00822 { 00823 return (uint8_t) HAL_ERROR; 00824 } 00825 00826 /* Select Channel 8 to be converted */ 00827 sConfig.Channel = ADC_CHANNEL_8; 00828 sConfig.SamplingTime = ADC_SAMPLETIME_71CYCLES_5; 00829 sConfig.Rank = 1; 00830 00831 /* Return Joystick initialization status */ 00832 return (uint8_t)HAL_ADC_ConfigChannel(&hnucleo_Adc, &sConfig); 00833 } 00834 00835 /** 00836 * @brief DeInit joystick GPIOs. 00837 * @note JOY DeInit does not disable the Mfx, just set the Mfx pins in Off mode 00838 * @retval None. 00839 */ 00840 void BSP_JOY_DeInit(void) 00841 { 00842 ADCx_DeInit(); 00843 } 00844 00845 /** 00846 * @brief Returns the Joystick key pressed. 00847 * @note To know which Joystick key is pressed we need to detect the voltage 00848 * level on each key output 00849 * - None : 3.3 V / 4095 00850 * - SEL : 1.055 V / 1308 00851 * - DOWN : 0.71 V / 88 00852 * - LEFT : 3.0 V / 3720 00853 * - RIGHT : 0.595 V / 737 00854 * - UP : 1.65 V / 2046 00855 * @retval JOYState_TypeDef: Code of the Joystick key pressed. 00856 */ 00857 JOYState_TypeDef BSP_JOY_GetState(void) 00858 { 00859 JOYState_TypeDef state = JOY_NONE; 00860 uint16_t keyconvertedvalue = 0; 00861 00862 /* Start the conversion process */ 00863 HAL_ADC_Start(&hnucleo_Adc); 00864 00865 /* Wait for the end of conversion */ 00866 if (HAL_ADC_PollForConversion(&hnucleo_Adc, 10) != HAL_TIMEOUT) 00867 { 00868 /* Get the converted value of regular channel */ 00869 keyconvertedvalue = HAL_ADC_GetValue(&hnucleo_Adc); 00870 } 00871 00872 if((keyconvertedvalue > 1800) && (keyconvertedvalue < 2090)) 00873 { 00874 state = JOY_UP; 00875 } 00876 else if((keyconvertedvalue > 500) && (keyconvertedvalue < 780)) 00877 { 00878 state = JOY_RIGHT; 00879 } 00880 else if((keyconvertedvalue > 1200) && (keyconvertedvalue < 1350)) 00881 { 00882 state = JOY_SEL; 00883 } 00884 else if((keyconvertedvalue > 10) && (keyconvertedvalue < 130)) 00885 { 00886 state = JOY_DOWN; 00887 } 00888 else if((keyconvertedvalue > 3500) && (keyconvertedvalue < 3760)) 00889 { 00890 state = JOY_LEFT; 00891 } 00892 else 00893 { 00894 state = JOY_NONE; 00895 } 00896 00897 /* Return the code of the Joystick key pressed*/ 00898 return state; 00899 } 00900 #endif /* HAL_ADC_MODULE_ENABLED */ 00901 00902 /** 00903 * @} 00904 */ 00905 00906 /** 00907 * @} 00908 */ 00909 00910 /** 00911 * @} 00912 */ 00913 00914 /** 00915 * @} 00916 */ 00917 00918 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Fri Mar 3 2017 19:17:51 for STM32F1xx_Nucleo BSP User Manual by
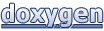