STM32F0xx_Nucleo_32 BSP User Manual
|
Functions | |
uint32_t | BSP_GetVersion (void) |
This method returns the STM32F0XX NUCLEO BSP Driver revision. | |
void | BSP_LED_Init (Led_TypeDef Led) |
Configures LED GPIO. | |
void | BSP_LED_On (Led_TypeDef Led) |
Turns selected LED On. | |
void | BSP_LED_Off (Led_TypeDef Led) |
Turns selected LED Off. | |
void | BSP_LED_Toggle (Led_TypeDef Led) |
Toggles the selected LED. | |
void | I2C1_Init (void) |
I2C Bus initialization. | |
void | I2C1_Write (uint8_t Addr, uint8_t Reg, uint8_t Value) |
Writes a single data. | |
uint8_t | I2C1_Read (uint8_t Addr, uint8_t Reg) |
Reads a single data. | |
HAL_StatusTypeDef | I2C1_ReadBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Reads multiple data on the BUS. | |
HAL_StatusTypeDef | I2C1_IsDeviceReady (uint16_t DevAddress, uint32_t Trials) |
Checks if target device is ready for communication. | |
HAL_StatusTypeDef | I2C1_WriteBuffer (uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) |
Write a value in a register of the device through BUS. | |
void | I2C1_Error (void) |
Manages error callback by re-initializing I2C. | |
void | I2C1_MspInit (I2C_HandleTypeDef *hi2c) |
I2C MSP Initialization. |
Function Documentation
uint32_t BSP_GetVersion | ( | void | ) |
This method returns the STM32F0XX NUCLEO BSP Driver revision.
- Return values:
-
version : 0xXYZR (8bits for each decimal, R for RC)
Definition at line 114 of file stm32f0xx_nucleo_32.c.
References __STM32F0XX_NUCLEO_32_BSP_VERSION.
void BSP_LED_Init | ( | Led_TypeDef | Led | ) |
Configures LED GPIO.
- Parameters:
-
Led Specifies the Led to be configured. This parameter can be one of following parameters: - LED3
- Return values:
-
None
Definition at line 126 of file stm32f0xx_nucleo_32.c.
References LED_PIN, LED_PORT, and LEDx_GPIO_CLK_ENABLE.
void BSP_LED_Off | ( | Led_TypeDef | Led | ) |
Turns selected LED Off.
- Parameters:
-
Led Specifies the Led to be set off. This parameter can be one of following parameters: - LED3
- Return values:
-
None
Definition at line 162 of file stm32f0xx_nucleo_32.c.
void BSP_LED_On | ( | Led_TypeDef | Led | ) |
Turns selected LED On.
- Parameters:
-
Led Specifies the Led to be set on. This parameter can be one of following parameters: - LED3
- Return values:
-
None
Definition at line 150 of file stm32f0xx_nucleo_32.c.
void BSP_LED_Toggle | ( | Led_TypeDef | Led | ) |
Toggles the selected LED.
- Parameters:
-
Led Specifies the Led to be toggled. This parameter can be one of following parameters: - LED3
- Return values:
-
None
Definition at line 174 of file stm32f0xx_nucleo_32.c.
void I2C1_Error | ( | void | ) |
Manages error callback by re-initializing I2C.
- Return values:
-
None
Definition at line 316 of file stm32f0xx_nucleo_32.c.
References I2C1_Init(), and nucleo32_I2c1.
Referenced by I2C1_Read(), I2C1_ReadBuffer(), I2C1_Write(), and I2C1_WriteBuffer().
void I2C1_Init | ( | void | ) |
I2C Bus initialization.
- Return values:
-
None
Definition at line 189 of file stm32f0xx_nucleo_32.c.
References BSP_I2C1, I2C1_MspInit(), I2C1_TIMING, and nucleo32_I2c1.
Referenced by BSP_DIGIT4_SEG7_Init(), and I2C1_Error().
HAL_StatusTypeDef I2C1_IsDeviceReady | ( | uint16_t | DevAddress, |
uint32_t | Trials | ||
) |
Checks if target device is ready for communication.
- Note:
- This function is used with Memory devices
- Parameters:
-
DevAddress Target device address Trials Number of trials
- Return values:
-
HAL status
Definition at line 283 of file stm32f0xx_nucleo_32.c.
References I2c1Timeout, and nucleo32_I2c1.
void I2C1_MspInit | ( | I2C_HandleTypeDef * | hi2c | ) |
I2C MSP Initialization.
- Parameters:
-
hi2c I2C handle
- Return values:
-
None
Definition at line 330 of file stm32f0xx_nucleo_32.c.
References BSP_I2C1_CLK_ENABLE, BSP_I2C1_FORCE_RESET, BSP_I2C1_GPIO_CLK_ENABLE, BSP_I2C1_GPIO_PORT, BSP_I2C1_RELEASE_RESET, BSP_I2C1_SCL_PIN, BSP_I2C1_SCL_SDA_AF, and BSP_I2C1_SDA_PIN.
Referenced by I2C1_Init().
uint8_t I2C1_Read | ( | uint8_t | Addr, |
uint8_t | Reg | ||
) |
Reads a single data.
- Parameters:
-
Addr I2C address Reg Register address
- Return values:
-
Read data
Definition at line 236 of file stm32f0xx_nucleo_32.c.
References I2C1_Error(), and nucleo32_I2c1.
HAL_StatusTypeDef I2C1_ReadBuffer | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) |
Reads multiple data on the BUS.
- Parameters:
-
Addr I2C Address Reg Reg Address RegSize The target register size (can be 8BIT or 16BIT) pBuffer pointer to read data buffer Length length of the data
- Return values:
-
0 if no problems to read multiple data
Definition at line 261 of file stm32f0xx_nucleo_32.c.
References I2C1_Error(), I2c1Timeout, and nucleo32_I2c1.
void I2C1_Write | ( | uint8_t | Addr, |
uint8_t | Reg, | ||
uint8_t | Value | ||
) |
Writes a single data.
- Parameters:
-
Addr I2C address Reg Register address Value Data to be written
- Return values:
-
None
Definition at line 216 of file stm32f0xx_nucleo_32.c.
References I2C1_Error(), and nucleo32_I2c1.
HAL_StatusTypeDef I2C1_WriteBuffer | ( | uint16_t | Addr, |
uint8_t | Reg, | ||
uint16_t | RegSize, | ||
uint8_t * | pBuffer, | ||
uint16_t | Length | ||
) |
Write a value in a register of the device through BUS.
- Parameters:
-
Addr Device address on BUS Bus. Reg The target register address to write RegSize The target register size (can be 8BIT or 16BIT) pBuffer The target register value to be written Length buffer size to be written
- Return values:
-
None
Definition at line 297 of file stm32f0xx_nucleo_32.c.
References I2C1_Error(), I2c1Timeout, and nucleo32_I2c1.
Referenced by BSP_DIGIT4_SEG7_Display(), and BSP_DIGIT4_SEG7_Init().
Generated on Wed Jul 5 2017 09:51:17 for STM32F0xx_Nucleo_32 BSP User Manual by
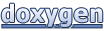