STM32F0xx_Nucleo_32 BSP User Manual
|
stm32f0xx_nucleo_32.c
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f0xx_nucleo_32.c 00004 * @author MCD Application Team 00005 * @brief This file provides set of firmware functions to manage: 00006 * - LED available on STM32F0XX-Nucleo Kit 00007 * from STMicroelectronics. 00008 * - Gravitech 7segment shield available separatly. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Includes ------------------------------------------------------------------*/ 00040 #include "stm32f0xx_nucleo_32.h" 00041 00042 /** @addtogroup BSP 00043 * @{ 00044 */ 00045 00046 /** @addtogroup STM32F0XX_NUCLEO_32 NUCLEO 32 00047 * @{ 00048 */ 00049 00050 /** @defgroup STM32F0XX_NUCLEO_32_Private_Constants Private Constants 00051 * @{ 00052 */ 00053 00054 /** 00055 * @brief STM32F0XX NUCLEO BSP Driver version number V1.0.4 00056 */ 00057 #define __STM32F0XX_NUCLEO_32_BSP_VERSION_MAIN (0x01) /*!< [31:24] main version */ 00058 #define __STM32F0XX_NUCLEO_32_BSP_VERSION_SUB1 (0x00) /*!< [23:16] sub1 version */ 00059 #define __STM32F0XX_NUCLEO_32_BSP_VERSION_SUB2 (0x04) /*!< [15:8] sub2 version */ 00060 #define __STM32F0XX_NUCLEO_32_BSP_VERSION_RC (0x00) /*!< [7:0] release candidate */ 00061 #define __STM32F0XX_NUCLEO_32_BSP_VERSION ((__STM32F0XX_NUCLEO_32_BSP_VERSION_MAIN << 24)\ 00062 |(__STM32F0XX_NUCLEO_32_BSP_VERSION_SUB1 << 16)\ 00063 |(__STM32F0XX_NUCLEO_32_BSP_VERSION_SUB2 << 8 )\ 00064 |(__STM32F0XX_NUCLEO_32_BSP_VERSION_RC)) 00065 00066 /** @defgroup STM32F0XX_NUCLEO_32_Private_Variables Private Variables 00067 * @{ 00068 */ 00069 GPIO_TypeDef* LED_PORT[LEDn] = {LED3_GPIO_PORT}; 00070 const uint16_t LED_PIN[LEDn] = {LED3_PIN}; 00071 00072 00073 /** 00074 * @brief BUS variables 00075 */ 00076 #if defined(HAL_I2C_MODULE_ENABLED) 00077 uint32_t I2c1Timeout = BSP_I2C1_TIMEOUT_MAX; /*<! Value of Timeout when I2C1 communication fails */ 00078 I2C_HandleTypeDef nucleo32_I2c1; 00079 #endif /* HAL_I2C_MODULE_ENABLED */ 00080 00081 /** 00082 * @} 00083 */ 00084 00085 /** @defgroup STM32F0XX_NUCLEO_32_Private_Function_Prototypes Private Function Prototypes 00086 * @{ 00087 */ 00088 00089 #if defined(HAL_I2C_MODULE_ENABLED) 00090 /* I2C1 bus function */ 00091 /* Link function for I2C peripherals */ 00092 void I2C1_Init(void); 00093 void I2C1_Error (void); 00094 void I2C1_MspInit(I2C_HandleTypeDef *hi2c); 00095 void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value); 00096 uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg); 00097 HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00098 HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length); 00099 HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials); 00100 #endif /* HAL_I2C_MODULE_ENABLED */ 00101 00102 /** 00103 * @} 00104 */ 00105 00106 /** @defgroup STM32F0XX_NUCLEO_32_Private_Functions Private Functions 00107 * @{ 00108 */ 00109 00110 /** 00111 * @brief This method returns the STM32F0XX NUCLEO BSP Driver revision 00112 * @retval version : 0xXYZR (8bits for each decimal, R for RC) 00113 */ 00114 uint32_t BSP_GetVersion(void) 00115 { 00116 return __STM32F0XX_NUCLEO_32_BSP_VERSION; 00117 } 00118 00119 /** 00120 * @brief Configures LED GPIO. 00121 * @param Led Specifies the Led to be configured. 00122 * This parameter can be one of following parameters: 00123 * @arg LED3 00124 * @retval None 00125 */ 00126 void BSP_LED_Init(Led_TypeDef Led) 00127 { 00128 GPIO_InitTypeDef GPIO_InitStruct; 00129 00130 /* Enable the GPIO_LED Clock */ 00131 LEDx_GPIO_CLK_ENABLE(Led); 00132 00133 /* Configure the GPIO_LED pin */ 00134 GPIO_InitStruct.Pin = LED_PIN[Led]; 00135 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00136 GPIO_InitStruct.Pull = GPIO_NOPULL; 00137 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00138 00139 HAL_GPIO_Init(LED_PORT[Led], &GPIO_InitStruct); 00140 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00141 } 00142 00143 /** 00144 * @brief Turns selected LED On. 00145 * @param Led Specifies the Led to be set on. 00146 * This parameter can be one of following parameters: 00147 * @arg LED3 00148 * @retval None 00149 */ 00150 void BSP_LED_On(Led_TypeDef Led) 00151 { 00152 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_SET); 00153 } 00154 00155 /** 00156 * @brief Turns selected LED Off. 00157 * @param Led Specifies the Led to be set off. 00158 * This parameter can be one of following parameters: 00159 * @arg LED3 00160 * @retval None 00161 */ 00162 void BSP_LED_Off(Led_TypeDef Led) 00163 { 00164 HAL_GPIO_WritePin(LED_PORT[Led], LED_PIN[Led], GPIO_PIN_RESET); 00165 } 00166 00167 /** 00168 * @brief Toggles the selected LED. 00169 * @param Led Specifies the Led to be toggled. 00170 * This parameter can be one of following parameters: 00171 * @arg LED3 00172 * @retval None 00173 */ 00174 void BSP_LED_Toggle(Led_TypeDef Led) 00175 { 00176 HAL_GPIO_TogglePin(LED_PORT[Led], LED_PIN[Led]); 00177 } 00178 00179 /****************************************************************************** 00180 BUS OPERATIONS 00181 *******************************************************************************/ 00182 #if defined(HAL_I2C_MODULE_ENABLED) 00183 /******************************* I2C Routines *********************************/ 00184 00185 /** 00186 * @brief I2C Bus initialization 00187 * @retval None 00188 */ 00189 void I2C1_Init(void) 00190 { 00191 if(HAL_I2C_GetState(&nucleo32_I2c1) == HAL_I2C_STATE_RESET) 00192 { 00193 nucleo32_I2c1.Instance = BSP_I2C1; 00194 nucleo32_I2c1.Init.Timing = I2C1_TIMING; 00195 nucleo32_I2c1.Init.OwnAddress1 = 0; 00196 nucleo32_I2c1.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT; 00197 nucleo32_I2c1.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE; 00198 nucleo32_I2c1.Init.OwnAddress2 = 0; 00199 nucleo32_I2c1.Init.OwnAddress2Masks = I2C_OA2_NOMASK; 00200 nucleo32_I2c1.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE; 00201 nucleo32_I2c1.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE; 00202 00203 /* Init the I2C */ 00204 I2C1_MspInit(&nucleo32_I2c1); 00205 HAL_I2C_Init(&nucleo32_I2c1); 00206 } 00207 } 00208 00209 /** 00210 * @brief Writes a single data. 00211 * @param Addr I2C address 00212 * @param Reg Register address 00213 * @param Value Data to be written 00214 * @retval None 00215 */ 00216 void I2C1_Write(uint8_t Addr, uint8_t Reg, uint8_t Value) 00217 { 00218 HAL_StatusTypeDef status = HAL_OK; 00219 00220 status = HAL_I2C_Mem_Write(&nucleo32_I2c1, Addr, (uint16_t)Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 100); 00221 00222 /* Check the communication status */ 00223 if(status != HAL_OK) 00224 { 00225 /* Execute user timeout callback */ 00226 I2C1_Error(); 00227 } 00228 } 00229 00230 /** 00231 * @brief Reads a single data. 00232 * @param Addr I2C address 00233 * @param Reg Register address 00234 * @retval Read data 00235 */ 00236 uint8_t I2C1_Read(uint8_t Addr, uint8_t Reg) 00237 { 00238 HAL_StatusTypeDef status = HAL_OK; 00239 uint8_t Value = 0; 00240 00241 status = HAL_I2C_Mem_Read(&nucleo32_I2c1, Addr, Reg, I2C_MEMADD_SIZE_8BIT, &Value, 1, 1000); 00242 00243 /* Check the communication status */ 00244 if(status != HAL_OK) 00245 { 00246 /* Execute user timeout callback */ 00247 I2C1_Error(); 00248 } 00249 return Value; 00250 } 00251 00252 /** 00253 * @brief Reads multiple data on the BUS. 00254 * @param Addr I2C Address 00255 * @param Reg Reg Address 00256 * @param RegSize The target register size (can be 8BIT or 16BIT) 00257 * @param pBuffer pointer to read data buffer 00258 * @param Length length of the data 00259 * @retval 0 if no problems to read multiple data 00260 */ 00261 HAL_StatusTypeDef I2C1_ReadBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00262 { 00263 HAL_StatusTypeDef status = HAL_OK; 00264 00265 status = HAL_I2C_Mem_Read(&nucleo32_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00266 00267 /* Check the communication status */ 00268 if(status != HAL_OK) 00269 { 00270 /* Re-Initiaize the BUS */ 00271 I2C1_Error(); 00272 } 00273 return status; 00274 } 00275 00276 /** 00277 * @brief Checks if target device is ready for communication. 00278 * @note This function is used with Memory devices 00279 * @param DevAddress Target device address 00280 * @param Trials Number of trials 00281 * @retval HAL status 00282 */ 00283 HAL_StatusTypeDef I2C1_IsDeviceReady(uint16_t DevAddress, uint32_t Trials) 00284 { 00285 return (HAL_I2C_IsDeviceReady(&nucleo32_I2c1, DevAddress, Trials, I2c1Timeout)); 00286 } 00287 00288 /** 00289 * @brief Write a value in a register of the device through BUS. 00290 * @param Addr Device address on BUS Bus. 00291 * @param Reg The target register address to write 00292 * @param RegSize The target register size (can be 8BIT or 16BIT) 00293 * @param pBuffer The target register value to be written 00294 * @param Length buffer size to be written 00295 * @retval None 00296 */ 00297 HAL_StatusTypeDef I2C1_WriteBuffer(uint16_t Addr, uint8_t Reg, uint16_t RegSize, uint8_t *pBuffer, uint16_t Length) 00298 { 00299 HAL_StatusTypeDef status = HAL_OK; 00300 00301 status = HAL_I2C_Mem_Write(&nucleo32_I2c1, Addr, Reg, RegSize, pBuffer, Length, I2c1Timeout); 00302 00303 /* Check the communication status */ 00304 if(status != HAL_OK) 00305 { 00306 /* Re-Initiaize the BUS */ 00307 I2C1_Error(); 00308 } 00309 return status; 00310 } 00311 00312 /** 00313 * @brief Manages error callback by re-initializing I2C. 00314 * @retval None 00315 */ 00316 void I2C1_Error(void) 00317 { 00318 /* De-initialize the I2C communication BUS */ 00319 HAL_I2C_DeInit(&nucleo32_I2c1); 00320 00321 /* Re-Initiaize the I2C communication BUS */ 00322 I2C1_Init(); 00323 } 00324 00325 /** 00326 * @brief I2C MSP Initialization 00327 * @param hi2c I2C handle 00328 * @retval None 00329 */ 00330 void I2C1_MspInit(I2C_HandleTypeDef *hi2c) 00331 { 00332 GPIO_InitTypeDef GPIO_InitStruct; 00333 RCC_PeriphCLKInitTypeDef RCC_PeriphCLKInitStruct; 00334 00335 /*##-1- Set source clock to SYSCLK for I2C1 ################################################*/ 00336 RCC_PeriphCLKInitStruct.PeriphClockSelection = RCC_PERIPHCLK_I2C1; 00337 RCC_PeriphCLKInitStruct.I2c1ClockSelection = RCC_I2C1CLKSOURCE_SYSCLK; 00338 HAL_RCCEx_PeriphCLKConfig(&RCC_PeriphCLKInitStruct); 00339 00340 /*##-2- Configure the GPIOs ################################################*/ 00341 00342 /* Enable GPIO clock */ 00343 BSP_I2C1_GPIO_CLK_ENABLE(); 00344 00345 /* Configure I2C SCL & SDA as alternate function */ 00346 GPIO_InitStruct.Pin = (BSP_I2C1_SCL_PIN| BSP_I2C1_SDA_PIN); 00347 GPIO_InitStruct.Mode = GPIO_MODE_AF_OD; 00348 GPIO_InitStruct.Pull = GPIO_PULLUP; 00349 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_HIGH; 00350 GPIO_InitStruct.Alternate = BSP_I2C1_SCL_SDA_AF; 00351 HAL_GPIO_Init(BSP_I2C1_GPIO_PORT, &GPIO_InitStruct); 00352 00353 /*##-3- Configure the Eval I2C peripheral #######################################*/ 00354 /* Enable I2C clock */ 00355 BSP_I2C1_CLK_ENABLE(); 00356 00357 /* Force the I2C peripheral clock reset */ 00358 BSP_I2C1_FORCE_RESET(); 00359 00360 /* Release the I2C peripheral clock reset */ 00361 BSP_I2C1_RELEASE_RESET(); 00362 } 00363 00364 #endif /*HAL_I2C_MODULE_ENABLED*/ 00365 00366 /** 00367 * @} 00368 */ 00369 00370 /** 00371 * @} 00372 */ 00373 00374 /** 00375 * @} 00376 */ 00377 00378 /** @addtogroup STM32F0XX_NUCLEO_32_GRAVITECH_4DIGITS 00379 * @{ 00380 */ 00381 00382 /** @addtogroup STM32_GRAVITECH_4DIGITS_Exported_Functions 00383 * @{ 00384 */ 00385 00386 #if defined(HAL_I2C_MODULE_ENABLED) 00387 00388 /** 00389 * @brief BSP of the 4 digits 7 Segment Display shield for Arduino Nano - Gravitech. 00390 init 00391 * @retval HAL_StatusTypeDef 00392 */ 00393 HAL_StatusTypeDef BSP_DIGIT4_SEG7_Init(void) 00394 { 00395 uint8_t control[1] = {0x47}; 00396 00397 /* Init I2C */ 00398 I2C1_Init(); 00399 00400 /* Configure the SAA1064 component */ 00401 return I2C1_WriteBuffer(0x70, 0, 1, control, sizeof(control)); 00402 } 00403 00404 /** 00405 * @brief BSP of the 4 digits 7 Segment Display shield for Arduino Nano - Gravitech. 00406 Display the value if value belong to [0-9999] 00407 * @param Value A number between 0 and 9999 will be displayed on the screen. 00408 * DIGIT4_SEG7_RESET will reset the screen (any value above 9999 will reset the screen also) 00409 * @retval HAL_StatusTypeDef 00410 */ 00411 HAL_StatusTypeDef BSP_DIGIT4_SEG7_Display(uint32_t Value) 00412 { 00413 const uint8_t lookup[10] = {0x3F,0x06,0x5B,0x4F,0x66, 00414 0x6D,0x7D,0x07,0x7F,0x6F}; 00415 00416 uint32_t thousands, hundreds, tens, base; 00417 HAL_StatusTypeDef status = HAL_ERROR; 00418 uint8_t d1d2d3d4[4]; 00419 00420 if (Value < 10000) 00421 { 00422 thousands = Value / 1000; 00423 hundreds = (Value - (thousands * 1000)) / 100; 00424 tens = (Value - ((thousands * 1000) + (hundreds * 100))) / 10; 00425 base = Value - ((thousands * 1000) + (hundreds * 100) + (tens * 10)); 00426 00427 d1d2d3d4[3] = lookup[thousands]; 00428 d1d2d3d4[2] = lookup[hundreds]; 00429 d1d2d3d4[1] = lookup[tens]; 00430 d1d2d3d4[0] = lookup[base]; 00431 00432 } 00433 else 00434 { 00435 d1d2d3d4[3] = 0; 00436 d1d2d3d4[2] = 0; 00437 d1d2d3d4[1] = 0; 00438 d1d2d3d4[0] = 0; 00439 } 00440 00441 /* Send the four digits to the SAA1064 component */ 00442 status = I2C1_WriteBuffer(0x70, 1, 1, d1d2d3d4, sizeof(d1d2d3d4)); 00443 00444 return status; 00445 } 00446 00447 #endif /*HAL_I2C_MODULE_ENABLED*/ 00448 00449 /** 00450 * @} 00451 */ 00452 00453 /** 00454 * @} 00455 */ 00456 00457 /** 00458 * @} 00459 */ 00460 00461 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Wed Jul 5 2017 09:51:17 for STM32F0xx_Nucleo_32 BSP User Manual by
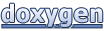