STM32F0xx_Nucleo_32 BSP User Manual
|
stm32f0xx_nucleo_32.h
Go to the documentation of this file.
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f0xx_nucleo_32.h 00004 * @author MCD Application Team 00005 * @brief This file contains definitions for: 00006 * - LED available on STM32F0xx-Nucleo_32 Kit from STMicroelectronics 00007 * - 7 segment display from Gravitech 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Define to prevent recursive inclusion -------------------------------------*/ 00039 #ifndef __STM32F0XX_NUCLEO_32_H 00040 #define __STM32F0XX_NUCLEO_32_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 /* Includes ------------------------------------------------------------------*/ 00047 #include "stm32f0xx_hal.h" 00048 00049 00050 /** @addtogroup BSP 00051 * @{ 00052 */ 00053 00054 /** @defgroup STM32F0XX_NUCLEO_32 NUCLEO 32 00055 * @brief This section contains the exported types, contants and functions 00056 * required to use the Nucleo 32 board. 00057 * @{ 00058 */ 00059 00060 /** @defgroup STM32F0XX_NUCLEO_32_Exported_Types Exported Types 00061 * @{ 00062 */ 00063 00064 typedef enum 00065 { 00066 LED3 = 0, 00067 LED_GREEN = LED3 00068 } Led_TypeDef; 00069 00070 /** 00071 * @} 00072 */ 00073 00074 /** @defgroup STM32F0XX_NUCLEO_32_Exported_Constants Exported Constants 00075 * @brief Define for STM32F0XX_NUCLEO_32 board 00076 * @{ 00077 */ 00078 00079 #if !defined (USE_STM32F0XX_NUCLEO_32) 00080 #define USE_STM32F0XX_NUCLEO_32 00081 #endif 00082 00083 /** @defgroup STM32F0XX_NUCLEO_LED LED Constants 00084 * @{ 00085 */ 00086 00087 #define LEDn 1 00088 00089 #define LED3_PIN GPIO_PIN_3 00090 #define LED3_GPIO_PORT GPIOB 00091 #define LED3_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00092 #define LED3_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00093 00094 #define LEDx_GPIO_CLK_ENABLE(__INDEX__) do {LED3_GPIO_CLK_ENABLE(); } while(0) 00095 #define LEDx_GPIO_CLK_DISABLE(__INDEX__) LED3_GPIO_CLK_DISABLE()) 00096 00097 /** 00098 * @} 00099 */ 00100 00101 /** @defgroup STM32F0XX_NUCLEO_32_BUS BUS Constants 00102 * @{ 00103 */ 00104 00105 #if defined(HAL_I2C_MODULE_ENABLED) 00106 /*##################### I2C2 ###################################*/ 00107 /* User can use this section to tailor I2Cx instance used and associated resources */ 00108 /* Definition for I2C1 Pins */ 00109 #define BSP_I2C1 I2C1 00110 #define BSP_I2C1_CLK_ENABLE() __HAL_RCC_I2C1_CLK_ENABLE() 00111 #define BSP_I2C1_CLK_DISABLE() __HAL_RCC_I2C1_CLK_DISABLE() 00112 #define BSP_I2C1_FORCE_RESET() __HAL_RCC_I2C1_FORCE_RESET() 00113 #define BSP_I2C1_RELEASE_RESET() __HAL_RCC_I2C1_RELEASE_RESET() 00114 00115 #define BSP_I2C1_SCL_PIN GPIO_PIN_6 /* PB.6 add wire between D5 and A5 */ 00116 #define BSP_I2C1_SDA_PIN GPIO_PIN_7 /* PB.7 add wire between D4 and A4 */ 00117 00118 #define BSP_I2C1_GPIO_PORT GPIOB /* GPIOB */ 00119 #define BSP_I2C1_GPIO_CLK_ENABLE() __HAL_RCC_GPIOB_CLK_ENABLE() 00120 #define BSP_I2C1_GPIO_CLK_DISABLE() __HAL_RCC_GPIOB_CLK_DISABLE() 00121 #define BSP_I2C1_SCL_SDA_AF GPIO_AF1_I2C1 00122 00123 /* Maximum Timeout values for flags waiting loops. These timeouts are not based 00124 on accurate values, they just guarantee that the application will not remain 00125 stuck if the I2C communication is corrupted. 00126 You may modify these timeout values depending on CPU frequency and application 00127 conditions (interrupts routines ...). */ 00128 #define BSP_I2C1_TIMEOUT_MAX 1000 00129 00130 /* I2C TIMING is calculated in case of the I2C Clock source is the SYSCLK = 32 MHz */ 00131 /* Set TIMING to 0x009080B5 to reach 100 KHz speed (Rise time = 50ns, Fall time = 10ns) */ 00132 //#define I2C1_TIMING 0x009080B5 00133 #define I2C1_TIMING 0xB0420F13 00134 00135 #endif /* HAL_I2C_MODULE_ENABLED */ 00136 00137 /** 00138 * @} 00139 */ 00140 00141 /** 00142 * @} 00143 */ 00144 00145 /** @defgroup STM32F0XX_NUCLEO_32_Exported_Functions Exported Functions 00146 * @{ 00147 */ 00148 00149 uint32_t BSP_GetVersion(void); 00150 void BSP_LED_Init(Led_TypeDef Led); 00151 void BSP_LED_On(Led_TypeDef Led); 00152 void BSP_LED_Off(Led_TypeDef Led); 00153 void BSP_LED_Toggle(Led_TypeDef Led); 00154 00155 /** 00156 * @} 00157 */ 00158 00159 /** 00160 * @} 00161 */ 00162 00163 /** @defgroup STM32F0XX_NUCLEO_32_GRAVITECH_4DIGITS GRAVITECH 4 DIGITS 00164 * @brief This section contains the exported functions 00165 * required to use Gravitech shield 7 Segment Display 00166 * @{ 00167 */ 00168 00169 /** @defgroup STM32_GRAVITECH_4DIGITS_Exported_Constants Exported Constants 00170 * @{ 00171 */ 00172 00173 #define DIGIT4_SEG7_RESET 10000 00174 /** 00175 * @} 00176 */ 00177 00178 /** @defgroup STM32_GRAVITECH_4DIGITS_Exported_Functions Exported Functions 00179 * @{ 00180 */ 00181 00182 HAL_StatusTypeDef BSP_DIGIT4_SEG7_Init(void); 00183 HAL_StatusTypeDef BSP_DIGIT4_SEG7_Display(uint32_t Value); 00184 00185 /** 00186 * @} 00187 */ 00188 00189 /** 00190 * @} 00191 */ 00192 00193 /** 00194 * @} 00195 */ 00196 00197 #ifdef __cplusplus 00198 } 00199 #endif 00200 00201 #endif /* __STM32F0XX_NUCLEO_32_H */ 00202 00203 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00204
Generated on Wed Jul 5 2017 09:51:17 for STM32F0xx_Nucleo_32 BSP User Manual by
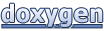